34 Javascript Check If Object Has Property
18/8/2019 · JavaScript has no built-in.length or.isEmpty methods for objects to check if they are empty. So we have to create our own utility method or use 3rd-party libraries like jQuery or Lodash to check if an object has own properties. Here are some of the methods you can use to make sure that a given object does not have its own properties: Let me ask again. What kind of thing is userObj? I already know, i know the format…But i need to see if you know.
How To Check If Array Includes A Value In Javascript
The hasOwnProperty() method returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it). Note: Object.hasOwn() is recommended over hasOwnProperty() , in browsers where it is supported.
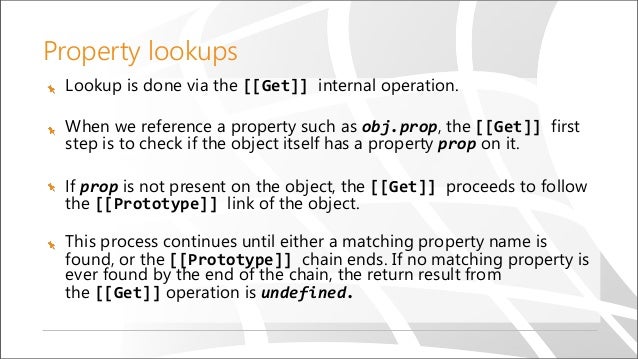
Javascript check if object has property. The Object.getOwnPropertyDescriptor() method returns an object describing the configuration of a specific property on a given object (that is, one directly present on an object and not in the object's prototype chain). The object returned is mutable but mutating it has no effect on the original property's configuration. Find Object In Array With Certain Property Value In JavaScript July 7, 2020 by Andreas Wik If you have an array of objects and want to extract a single object with a certain property value, e.g. id should be 12811, then find () has got you covered. 13/12/2020 · It tests whether at least one element in the array satisfies the test condition (which is implemented by the provided function). We can use this to test if a key in the object of arrays has a certain value in the following way: // ES5+ console.log(objs.some((obj) …
Browse other questions tagged javascript map associative-array is-empty object-property or ask your own question. The Overflow Blog Podcast 365: Fake your own voice with AI, podcasting has never been easier 8/8/2019 · Since JavaScript allows you to create dynamic objects, you have to be careful and check if an object’s property actually exists. There are at least 2 ways to do this. hasOwnProperty. hasOwnProperty is a function built into all objects. if (obj.hasOwnProperty('prop')) {// do something} var testObj = this.getView(); How can I check with DoJo (or just native JS) if testObj has callableFunction before I actually try to call callableFunction() and fail if it isn't there? I would prefer a native-DoJo solution as I need this to work on all browsers.
This is a type-safety check in JavaScript, and TypeScript benefits from that. However, there are some cases where TypeScript at the time of this writing needs a little bit more assistance from us. Let's assume you have a JavaScript object where you don't know if a certain property exists. The object might be any or unknown. In JavaScript ... If what you're looking for is if an object has a property on it that is iterable (when you iterate over the properties of the object, it will appear) then doing: prop in objectwill give you your desired effect. How to Check if a Value is an Object in JavaScript JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object".
The dot property accessor syntax object.property works nicely when you know the variable ahead of time. When the property name is dynamic or is not a valid identifier, a better alternative is square brackets property accessor: object[propertyName]. The object destructuring extracts the property directly into a variable: { property } = object. A Javascript object has normally the hasOwnProperty native method. The hasOwnProperty method returns a boolean indicating whether the object has the specified property as first parameter. Unlike the in operator, this method does not check down the object's prototype chain. If you want to know if the object physically contains the property @gnarf's answer using hasOwnProperty will do the work. If you're want to know if the property exists anywhere, either on the object itself or up in the prototype chain, you can use the in operator. if ('prop' in obj) { //...
In this best post for hasproperty in javascript, you'll read all about the 3 methods (hasOwnProperty () method, in operator, Comparing with undefined) to check if an object has a characteristic of an object : Compare with typeof and undefined. See the Pen javascript-object-exercise-17 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to get a copy of the object where the keys have become the values and the values the keys. Next: Write a JavaScript function to check whether a given value is a ... Javascript object provides the hasOwnProperty native method. The method returns a boolean indicating if the object has the specified property as a first parameter. The hasOwnProperty method does not check down the prototype chain of the object. let myObj = { welcome: "Welcome to W3Docs."
The hasOwnProperty () method in JavaScript is used to check whether the object has the specified property as its own property. This is useful for checking if the object has inherited the property rather than being it's own. Parameters: This method accepts single parameter prop which holds the name in the form of a String or a Symbol of the ... 3 Ways to Check If an Object Has a Property in JavaScript, The pickBy(object, predicate) methods creates an object composed of the input object properties for which values, predicate function return truthy value. If you do not provide the predicate function, the method will automatically check checked whether a value is truthy or not. The common ways to check if a property exists in an object are: The easiest is to use the hasOwnProperty() function - var exist = OBJECT.hasOwnProperty("PROPERTY");; Extract the keys from the object, then use the includes() function to check.. var keys = Object.keys(OBJECT);
How to Check for the Existence of Nested JavaScript Object Key To check for the existence of property nested objects you should do it step by step in order to avoid TypeError. It will be thrown if at least one of the members is null or undefined and you try to access a member. There are two ways of avoiding it. JavaScript Object hasOwnProperty () The JavaScript Object hasOwnProperty () method checks if the object has the given property as its own property. The syntax of the hasOwnProperty () method is: obj.hasOwnProperty (prop) Here, obj is an object. 30/6/2014 · Using myObject.hasOwnProperty('prop') is a great way of accessing the Object’s keys directly, which does not look into the Object’s prototype - hooray, this is great for specific use cases. hasOwnProperty returns a Boolean for us on whether a property exists. Examples
Use the hasOwnProperty()method to check if an property exists in the own properties of an object. Use the inoperator to check if a property exists in both own properties and inherited properties of an object. You can use the JavaScript some () method to find out if a JavaScript array contains an object. This method tests whether at least one element in the array passes the test implemented by the provided function. Here's an example that demonstrates how it works: It is important to use the hasOwnProperty() method, to determine whether the object has the specified property as a direct property, and not inherited from the object's prototype chain. Edit. From the comments: You can put that code in a function, and make it return false as soon as it reaches the part where there is the comment
The function has to check if the passed argument, users, contains the names: Alan, Jeff, Sarah and Ryan. And only returns true if the four names are in obj. A loop to check each name, accumulated in an array, to verify it exists in obj is fine. here is my function but saw a very nice and shorter alternative before. The in operator is another way to check the presence of a property in an object in JavaScript. It returns true if the property exists in an object. Otherwise, it returns false. Let us use the in operator to look for the cake property in the food object: @zzzzBov The question asks how to check if something is an object. ECMAScript defines what an object is, so I use that definition. I can't see any other reasonable interpretation. Answers that do other things (like excluding arrays) can be useful in some circumstances, but they don't check if something is an object. - Oriol Sep 2 '16 at 17:28
1. hasOwnProperty () method Every JavaScript object has a special method object.hasOwnProperty ('myProp') that returns a boolean indicating whether object has a property myProp. In the following example, hasOwnProperty () determines the presence of properties name and realName:
Object In Javascript Top Properties Methods
Check If Object Has Property Javascript On Joter Mahatours Fr
The Difference Between In And Hasownproperty In Javascript
How To Check If A Property Exists In An Object In Javascript
Javascript Find An Object In Array Based On Object S
Object Prototypes Learn Web Development Mdn
The Console Isn T Accepting My Solution Even Though I M
Javascript Check If Variable Exists Is Defined Initialized
Typescript Check If Array Contains Object With Property Value
Checking If A Key Exists In A Javascript Object Stack Overflow
Test Script Examples Postman Learning Center
How To Check If A Javascript Array Is Empty Or Not With Length
Objects In Javascript Geeksforgeeks
Different Ways To Check If An Object Is Empty In Javascript
How To Fix Eslint Error Do Not Access Object Prototype
Javascript Check If Object Has Method Code Example
How To Check If An Object Has A Specific Property In Javascript
Javascript Array Contains A Step By Step Guide Career Karma
How To Check If A Javascript Object Property Is Undefined
How To Check If An Object Has A Specific Property In
3 Ways To Check If An Object Has A Property In Javascript
Unquoted Property Names Object Keys In Javascript Mathias
The Right Way To Check If An Object Has A Property In
How To Check If An Object Is Empty In Javascript
Telmo Goncalves On Twitter Javascript Tip Use
2 Ways To Remove A Property From An Object In Javascript
Check If A Javascript Object Has A Property Tl Dev Tech
How To Check If An Object Has A Property Properly In
Typescript Check For Object Properties And Narrow Down Type
Github Jonschlinkert Get Value Use Property Paths A B C
Object Keys Function In Javascript The Complete Guide
0 Response to "34 Javascript Check If Object Has Property"
Post a Comment