33 Compare Two Dates Javascript
I have two string dates in the format of m/d/yyyy. For example, "11/1/2012", "1/2/2013". I am writing a function in JavaScript to compare two string dates. So, isLater("1/2/2013", "11/1/2012") should return true. Compare Two Dates, Ignoring Time, in JavaScript Dec 4, 2020 To compare two dates, ignoring differences in hours, minutes, or seconds, you can use the toDateString() function and compare the strings :
Javascript Difference Between Two Dates Code Example
Javascript Web Development Front End Technology. To compare two dates with JavaScript, create two dates object and get the recent date. You can try to run the following code to compare two dates.
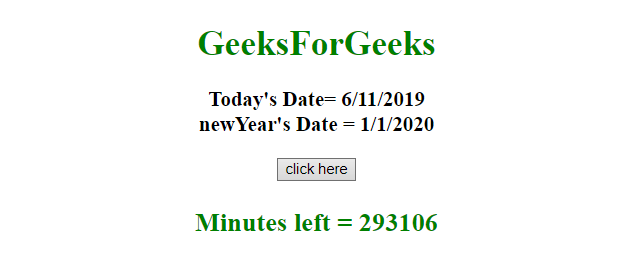
Compare two dates javascript. 21/7/2021 · Compare two dates using JavaScript. In JavaScript, we can compare two dates by converting them into numeric value to corresponding to its time. First, we can convert the Date into a numeric value by using getTime () function. By converting the given dates into numeric value we can directly compare them. The easiest way to compare dates in javascript is to first convert it to a Date object and then compare these date objects. Below three functions are used to find an object: 1. dates pare (a,b) There are a few common ways to compare dates in Javascript: Parse the date strings into date objects and compare them directly using operators. var dateA = new Date("1 Feb 2003"); var dateB = new Date("2 Mar 2004"); ... Compare two dates with JavaScript - StackOverflow ...
Checks if a date is the same as another date. Use Date.prototype.toISOString () and strict equality checking (===) to check if the first date is the same as the second one. Jun 10, 2020 - Get code examples like "compare two date objects javascript" instantly right from your google search results with the Grepper Chrome Extension. As you have seen above, date and date1 variables are initialized at different time and that's why getTime() is returning different values for both. This is a numeric value and if we want to compare two Date, we can simply do it by comparing the values returning by the getTime() method. Javascript program :
Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... javaScript Age in Dog years //write a function that takes your age and returns it to you in dog years - they say that 1 human year is equal to seven dog ... Dates can be compared easily in javascript. The dates can belong to any frame i.e past, present and future. Past dates can be compared to future or future dates can be compared to the present. 23/10/2020 · JavaScript Compare Two Dates With Comparison Operators. We can directly compare two dates in JavaScript with comparison operators like <, <=, > and >=. JavaScript. javascript Copy. var date1 = new Date('2020-10-23'); var date2 = new Date('2020-10-22'); console.log(date1 > date2); console.log(date1 >= date2); console.log(date1 < date2); console.
Compare two dates without time Here, we will learn to compare dates using new Date () operators that creates a new date object with the current date and time. Assume that, we have two JavaScript Date objects like: 2. Define two date variables in JavaScript Initialize them by creating the date objects using new Date () After defining the dates, calculate the time difference between them by subtracting one date from another date using date2.getTime () - date1.getTime (); Now next step is to calculate the days between the dates. compare two dates javascript without time code example
Aug 13, 2019 - Can someone suggest a way to compare the values of two dates greater than, less than, and not ... ? The values will be coming from text boxes. In this article, we're going to have a look at how to compare two dates in JavaScript. Quick solution: Copy. xxxxxxxxxx. 1. var date1 = new Date(2020, 10, 15); 2. var date2 = new Date(2020, 10, 15); 3. In JavaScript we can compare two dates by converting them to a numeric value to match the times, for this we use the getTime () function. By converting the specified dates into numeric values, we can compare them directly. We can use the relational operators below to compare two dates: < > <= >=. We cannot apply these operators below on the ...
Most programming languages (including JavaScript) compare dates as numbers, so a later date is a larger number, and an earlier date is a smaller number. They usually can combine dates and times, as well, treating each date + time combination as a number, so 3:31 AM on January 17, 1832 is a smaller number than 7:15 PM on that same date. Comparing Two Dates in JavaScript We can use comparison operators like < and > two compare two Date objects, and under the hood, their time counters are effectively compared. You're effectively comparing two integer counters: However, what if we need to compare these two dates using JavaScript? The getTime () method. The getTime () method allows us to get the Unix Timestamp of a Date object. This method will return the number of milliseconds that have passed between the "Unix Epoch" and the date in question.
Javascript Compare dates. In the previous section, we discussed the date methods as well as the constructors. Here, with the help of those methods, we will learn to compare dates. Basically, there are different ways by which we can compare dates, such as: Comparing two dates with one another. Comparing date with time. Comparing dates using getTime() 28/1/2009 · To compare two date we can use date.js JavaScript library which can be found at : https://code.google /archive/p/datejs/downloads. and use the Date pare( Date date1, Date date2 ) method and it return a number which mean the following result:-1 = date1 is lessthan date2. 0 = values are equal. 1 = date1 is greaterthan date2. Jun 10, 2020 - Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... how to write a program that displays a message “It’s Fun day” if it's Saturday or Sunday today in javascript
In this article, you will learn how to compare two dates in javascript. Date comparison is required any time you are using Date in your code or program. You can create the new Date objects using the Date () Constructor. You can compare two dates with JavaScript, you compare them using the >, <, <= or >= operators. Comparing two dates in JavaScript can be said like this: Compare two dates in terms of which is greater and which is smaller. In order to do that we can use these below relational operators: < > <= >= But unfortunately, we can not use these below operators: == != !== === You can’t use these to compare the value of the date. Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... how to write a program that displays a message “It’s Fun day” if it's Saturday or Sunday today in javascript
Today, We want to share with you compare two dates in javascript.In this post we will show you convert the Date into a numeric value by using getTime () function, hear for By converting using js the given dates into numeric value we can directly compare we will give you demo and example for implement.In this post, we will learn about Calculating the Difference between Two Dates in JavaScript with an example. Firstly to compare two dates in JavaScript, let's take a look at how we can compare two dates to see if they are equal. In JavaScript, it is well known that we cannot compare two different objects, even if they are the same because they are different instances which means they will always fail when comparing them. var date1 = new Date('December 25, 2017 01:30:00'); var date2 = new Date('June 18, 2016 02:30:00'); //best to use .getTime() to compare dates if(date1.getTime() === date2.getTime()){ //same date } if(date1.getTime() > date2.getTime()){ //date 1 is newer } ... using javascript when i ' m iterate ...
To compare the values of two dates, we need to check the first date is greater, less or equal than the second date. Using the Date object we can compare dates in JavaScript. Different ways to compare two dates in JavaScript. Compare two dates without time; Compare dates with time; Compare dates Using getTime() function; 1. Compare two dates ... Apr 28, 2021 - This post will discuss how to compare two date strings in JavaScript. The solution should determine whether the first date string is greater than, less than, or equal to the second date string. The easiest way to compare dates in javascript is to first convert it to a Date object and then compare these date-objects. Below you find an object with three functions: dates pare(a,b) Returns a number:-1 if a < b; 0 if a = b; 1 if a > b; NaN if a or b is an illegal date; dates.inRange (d,start,end) Returns a boolean or NaN:
Jul 23, 2019 - Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... how to write a program that displays a message “It’s Fun day” if it's Saturday or Sunday today in javascript Comparing dates in JavaScript The following describes how to compare two dates: I have two dates. Date one is 15-01-2010 and another date is 15-01-2011. In the above example, first we are constructing the dates with delimiter -then we are comparing the first date with second date, if both dates are equal it returns true else it returns false if it is not equal.. Second way using toDateString() method. Similarly, we can also compare two dates by using the toDateString() method which returns the date in English format "Mon Dec 16 2019".
Mar 18, 2020 - Although neither == nor === can compare whether two dates are equal, surprisingly both < and > work fine for comparing dates: d1 < d2; // false d1 < d3; // false d2 < d1; // true · So to check if date a is before date b, you can just check a < b. Another neat trick: you can subtract dates in JavaScript... Example: Compare Value of Two Dates. // program to compare value of two dates // create two dates const d1 = new Date(); const d2 = new Date(); // comparisons const compare1 = d1 < d2; console.log (compare1); const compare2 = d1 > d2; console.log (compare2); const compare3 = d1 <= d2; console.log (compare3); const compare4 = d1 >= d2; console.log ...
How To Store All Dates In An Array Present In Between Given
Calculate The Difference Between Two Dates In Javascript
3 2 3 6 Datetime Control Documentation Processmaker
How To Get Differences Between Two Dates Js Practice House
How To Compare Two Datetime Fields Microsoft Dynamics Crm
How To Get Difference Between Two Dates In Hours In Javascript
How To Find Unique Dates In An Array In Javascript By Dr
How To Compare Two Dates In Javascript Atomized Objects
How To Calculate The Difference In Days Between Two Calendar
How To Calculate Minutes Between Two Dates In Javascript
Comparing Difference Between Two Dates Or Times Zapier
Javascript Difference Between Two Dates In Days Hours And
How To Compare Two Dates Using Javascript Code Example How
Compare The Data Difference Between Two Dates Js Time Diff
Find All Date Between Two Dates In Javascript
Get Difference Between Two Dates Javascript Code Example
Custom Javascript In Cognos Analytics Date Prompts And
How To Compare Two Dates In Javascript Code Premix
How To Compare Two Dates In Java
Compare Two Dates With Javascript Stack Overflow
How To Calculate The Difference Between Two Dates In Javascript
How To Compare Two Dates Monthname Yearnumber In Javascript
Javascript Difference Between Two Dates In Days W3resource
How To Calculate Minutes Between Two Dates In Javascript
Comparing Difference Between Two Dates Or Times Zapier
How To Compare Two Dates In Javascript
How To Compare Two Dates Monthname Yearnumber In Javascript
Compare Two Dates In Javascript Clue Mediator
Javascript Comparison Between Two Dates W3resource
How To Work With Date And Time In Javascript Using Date
Php Difference Between Two Dates In Years Months And Days
Javascript How To Compare Dates Code Example
0 Response to "33 Compare Two Dates Javascript"
Post a Comment