32 Dynamically Create Object Javascript
# How to Set Dynamic Property Keys with ES6 🎉. Previously, we had to do 2 steps - create the object literal and then use the bracket notation. With ES6, you can now directly use a variable as your property key in your object literal. YAY! 👏 Method 2: using the Window object. In the browser, the window object is the global object. Using it also we can create a dynamic variable which will run on our browser. Without wasting any time, let's see below is the example of generating dynamic variables using the JavaScript window object:
Building Html Tables With Javascript By Chris Webb
Dynamic Array in JavaScript means either increasing or decreasing the size of the array automatically. JavaScript is not typed dependent so there is no static array. JavaScript directly allows array as dynamic only. We can perform adding, removing elements based on index values.
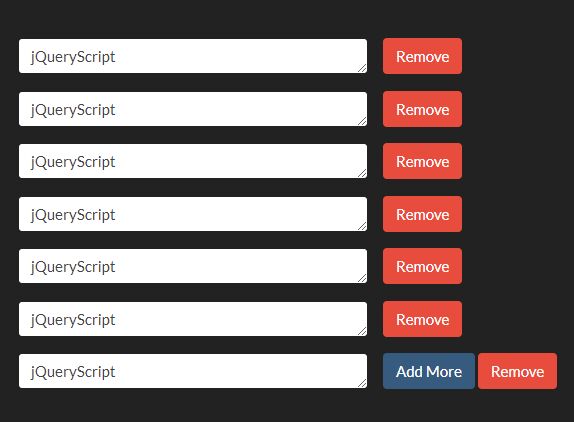
Dynamically create object javascript. Create or Add Dynamic key to Object. So, till this point, we get a rough idea about how we can create and access the objects in javascript. Let's now make it a step further and discuss how we can achieve dynamic key behavior in javascript objects. So, there are 3 ways that can be used to create a Dynamic key to an existing object. 1. The Object.create() method creates a new object, using an existing object as the prototype of the newly created object. ... JavaScript reference. Standard built-in objects. Object. Object.create() Change language ... but also dynamically via whatever the order any such property-adding code-branches actually get executed at runtime as depends on ... In earlier JavaScript specifications (ES5 and below), the key in an object literal is always interpreted literally, as a string. To use a "dynamic" key, you have to use bracket notation: var obj = {};
Creating objects dynamically with factory pattern in javascript Creating objects dynamically with factory pattern in javascript When creating features, we often need to create instances based on parameters coming from an endpoint or a similar dynamic source. 29/3/2021 · To create an object with dynamic keys in JavaScript, you can use ES6's computed property names feature. The computed property names feature allows us to assign an expression as the property name to an object within object literal notation. Here is an example: You can create most any shape element. Give it a try with polygons, polylines and even ellipses. You can dynamically create curves and paths too, but that's a bit more involved so I'll save it for another tutorial. I hope you picked up a few pixels of information about creating dynamic SVG elements. Until next time, keep your pixels movin'.
In this tutorial, we will review how to create an object, what object properties and methods are, and how to access, add, delete, modify, and loop through object properties. Creating an Object. An object is a JavaScript data type, just as a number or a string is also a data type. As a data type, an object can be contained in a variable. How to create dynamic values and objects in JavaScript ? Last Updated : 23 Feb, 2021 In JavaScript, you can choose dynamic values or variable names and object names and choose to edit the variable name in the future without accessing the array. To do, so you can create a variable and assign it a particular value. Dynamically create an object in mootools or javascript. Asked 2012-06-12 08:58:34. Active 2012-06-12 09:32:35. Viewed 117 times. ... I would like to convert an entire form of data to a javascript object. would convert to a javascript object... 2 answers JavaScript, MooTools - Variable scope in Class/overwrite global variable ...
In our index.js, we used document.createElement() to dynamically create a new div.Then, we added a dog class to the div we created earlier.. The classList property is used to add, remove, and toggle CSS classes on an element.. Next, we created an image tag with src and alt attributes.. We also created the h2 tag and added text content in it. Additionally, we added a new p element with a class ... In JavaScript, dynamic variable names can be achieved by using 2 methods/ways given below. eval (): The eval () function evaluates JavaScript code represented as a string in the parameter. A string is passed as a parameter to eval (). If the string represents an expression, eval () evaluates the expression. Inside eval (), we pass a string in ... Dynamically select a method of an object in JavaScript Learn how to access a method of an object dynamically in JavaScript. Published Jan 12, 2019. Sometimes you have an object and you need to call a method, or a different method, depending on some condition.
Defining a dynamic property like an Array on the Javascript Object. Let us take the same example as above: var obj = { property1: '', property2: '' }; To create a dynamic property on the object obj we can do: obj['property_name'] = 'some_value'; what this does is, it creates a new property on the object obj which can be accessed as. Creating Objects Dynamically There are two ways to create objects dynamically from JavaScript. You can either call Qt.createComponent() to dynamically create a Component object, or use Qt.createQmlObject() to create an object from a string of QML. Creating a component is better if you have an existing component defined in a QML document and you ... Dynamically create json object using javascript. Dynamically Add Variable Name Value Pairs To Json Object 3 Ways To Add Dynamic Key To Object In Javascript Codez Up Build Dynamic Json Stack Overflow Convert And Loop Through Json With Php And Javascript Arrays Perform Operations On Data Azure Logic Apps Microsoft Docs
so instead {…} you just create an empty object and then assign stuff to it, and use another if statement to check if there's a next property, case in which you'd assign a recursive call object to the terms sub-object. So you kinda need a nested if statement: the outer one to check if any of the lists have any elements left and the inner ... So I thought I could create objects for every tab , but I have to name them , I use something like this : Object. var step = function(params){ this.id = randID(); this.titolo = null; this.descrizione = null; this.dmax = null; $.extend(this,params); // this.render(); } When the button is clicked , it instance a new object 4/6/2020 · New elements can be dynamically created in JavaScript with the help of createElement () method. The attributes of the created element can be set using the setAttribute () method. The examples given below would demonstrate this approach. Example 1: In this example, a newly created element is added as a child to the parent element.
A JavaScript object is syntactically defined as a function, which is itself a first instance and that is cloned to create more instances. In addition, this structure is dynamic, methods (in fact inner functions) and attributes may be added during the execution of the script. In JavaScript everything that is defined in the global scope is defined as a property of the global window object. So the func_abc function defined about is defined as window.func_abc. Also in JavaScript properties of objects can be accessed using an associative array syntax e.g. window.abc and window["abc"] are equivalent. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
The last (but not the least) way to create a JavaScript object is using the Object.create () method. It's a standard method of JavaScript's pre-built Object object type. The Object.create () method allows you to use an existing object literal as the prototype of a new object you create. Learn how to dynamically create HTML elements with plain JavaScript. ... So the benefit of the bookmarks array is to create objects (a state of information), ... In JavaScript, an object is a standalone entity, with properties and type. Compare it with a cup, for example. A cup is an object, with properties. The syntax for creating a function from Function Object is as follows: const myFunction = new Function (arg1, arg2, …argN, body); args1, args2,.. argsN are the arguments accepted by the function and body is the string consisting of JavaScript statements that will be executed when the function is called.
How to dynamically create nested object in vanilla JavaScript. { Player1: [ {cardName: "test", balanceNum: null, ability:""} ], Player2: [ {cardName: "test", balanceNum: null, ability:""} ], . . . } At the moment I am currently using this attempt to loop through and create this dynamically. Player gets update through the loop and I want each ... Creating Objects Dynamically There are two ways to create objects dynamically from JavaScript. You can either call Qt.createComponent()to dynamically create a Componentobject, or use Qt.createQmlObject()to create an object from a string of QML. In this tutorial, you'll learn how to create JSON Array dynamically using JavaScript. This is one of most common scenarios and you'll see two ways of creating JSON array dynamically.
This tutorial will teach you how to create a dynamic HTML table through the use of Javascript and Document Object Model (DOM) manipulation. The number of table rows and cell content will vary depending on your data. In this tutorial, I will be creating a scoreboard for a Javascript video game and the data will be coming from a fetch request.
How To Create Dynamic Controls In C
How To Dynamically Create Javascript Elements With Event
Dynamically Creating Components With Angular By Netanel
Dynamically Add Elements Using Jquery And Save Data To Database
How To Code Adobe Javascript How To Code Pdf Javascript
Dynamically Select A Method Of An Object In Javascript
Vue Dynamic Forms Made With Vue Js
Working With Dynamic Objects Beyond The Basics With
Dynamically Create Html Form Fields Jquery Dynamic Forms
Translating Content Dynamically By Using Amazon S3 Object
How To Create An Image Element Dynamically Using Javascript
How To Generate Dynamic Pdfs Using React And Nodejs By
Dynamic V Model Name Binding In V For Loop Vuejs 5 Balloons
How To Create A Form Dynamically With The Javascript
How To Dynamically Add Properties In A Javascript Object Array
Rules For Dynamically Populated Groups Membership Azure Ad
Dynamic Array In Javascript Using An Array Literal And
Dynamically Creating A Json Object Structure Team Abap
How To Create Dynamic Forms With Custom Validation In
Create Ul And Li Elements Dynamically Using Javascript
Github Asigloo Vue Dynamic Forms Easy Way To Dynamically
How To Dynamically Create Reactive Properties In Vue
How To Make A Dynamic Dropdown With React And Fetch Matt
Call A Javascript Function Within Dynamically Created Html
Adding And Removing Vue Js Watchers Dynamically
Understanding Dynamic Routing And Api Routes In Next Js
Dynamic Array In Javascript Using An Array Literal And
Add Or Remove Input Fields Dynamically With Reactjs Clue
0 Response to "32 Dynamically Create Object Javascript"
Post a Comment