34 Factorial Program In Javascript Using For Loop W3schools
Factorial of any number in Javascript - Using javascript you can find factorial of any number only use for loop concept. HOME C C++ DS Java AWT Collection Jdbc JSP Servlet SQL PL/SQL C-Code C++-Code Java-Code Project Word Excel. Sitesbay - Easy to Learn . ... Factorial Program in Javascript. Advertisements Find Factorial of number in JavaScript. Using javascript you can find factorial of any number, here same logic are applied like c language. you only need to write your javascript code inside <script> ..... </script> tag using any editor like notepad or edit plus. Save below code with .html or .htm extension.
C Program to Print String C Program to Add n Number of Times C Program to Generate Random Numbers C Program to Check whether the Given Number is a Palindromic C Program to Check whether the Given Number is a Prime C Program to Find the Greatest Among Ten Numbers C Program to Find the Greatest Number of Three Numbers C Program to Asks the User For a Number Between 1 to 9 C Program to Check ...
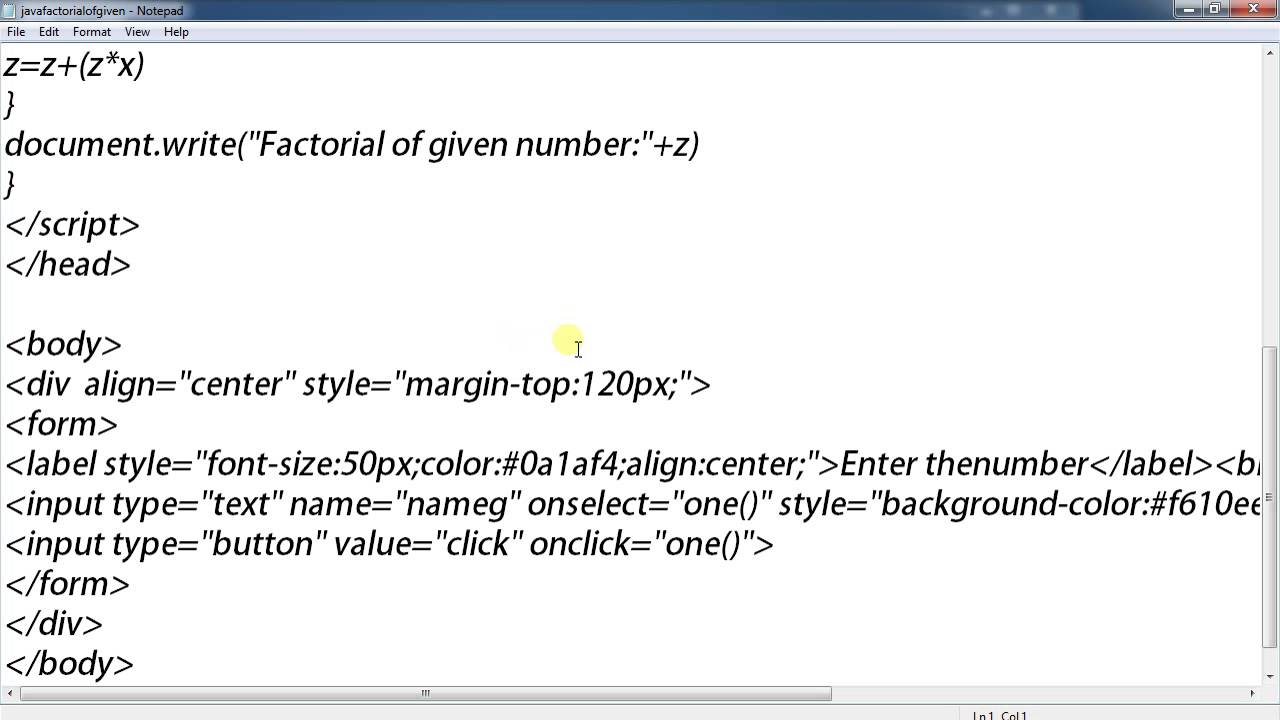
Factorial program in javascript using for loop w3schools. Do not use for in over an Array if the index order is important.. The index order is implementation-dependent, and array values may not be accessed in the order you expect. It is better to use a for loop, a for of loop, or Array.forEach() when the order is important. Javascript for loop factorial. airasyaqub August 3, 2017, 1:42pm #1. function FirstFactorial(num) ... Hey guys i am trying to find the factorial of a number using for loop and a function. Even though i skimmed and rechecked the algo of the program I couldnt understand why It is generating wrong results. please help here. 18/9/2020 · We are required to write a simple JavaScript function that takes in a Number, say n and computes its factorial using a for loop and returns the factorial. For example −. factorial(5) = 120, factorial(6) = 720. Maintain a count and a result variable, keep multiplying the count into result, simultaneously decreasing the count by 1, until it reaches 1
JavaScript Function: Exercise-1 with Solution. Write a JavaScript program to calculate the factorial of a number. In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. For example, 5! = 5 x 4 x 3 x 2 x 1 = 120. Pictorial Presentation: Sample Solution:-. So what is factorial? (if you don't know already) Multiplying given integer with the sequence of descending positive integers. For example, The value of 5! is 120. which is, 5 x 4 x 3 x 2 x 1 = 120. So, In this tutorial you are going to see you how to write a PHP program to find the n! using for loops! See the program here, JavaScript for Loop. Functions in JavaScript. JavaScript Objects. Arrays in JavaScript. Popular Examples. JavaScript "Hello World" Program. ... JavaScript Program to Find the Factorial of a Number. In this example, you will learn to write a JavaScript program to calculate the factorial of a number.
Now, a loop has to be implemented (here for loop) and within thisloop, the county variable 'i' is initialized as number-1, and the loop will continue till (i>1). Then the statement factorial =factorial * i; is given which calculates the factorial taking one value of 'i' at a time within loop and storing them back in the 'factorial' variable. 1. Fibonacci Series using for loop. Fibonacci Series can be considered as a list of numbers where everyone's number is the sum of the previous consecutive numbers. The list starts from 0 and continues until the defined number count. It is not any special function of JavaScript and can be written using any of the programming languages as well. The simplest way or logic to calculate the factorial program is by traversing each number one by one and multiplying it to the initial result variable. i.e. to find out the factorial of 5 we will traverse from 5 to 1 and go on multiplying each number with the initial result. In JavaScript, we can either traverse from 5 to 1 or from 1 to 5.
Java Factorial Program using For Loop. This program for factorial allows the user to enter any integer value. By using this value, this Java program finds Factorial of a number using the For Loop. The Factorial of number is the product of all the numbers less than or equal to that number & greater than 0. It is denoted with a (!) symbol. This article is based on Free Code Camp Basic Algorithm Scripting "Factorialize a Number" In mathematics, the factorial of a non-negative integer n can be a tricky algorithm. In this article, I'm going to explain three approaches, first with the recursive function, second using a while loop and third using a for loop. Write a program to count 5 to 15 using PHP loop: Write a factorial program using for loop in php: Factorial program in PHP using recursive function: Write a program to create Chess board in PHP using for loop: Write a Program to create given pattern with * using for loop
Factorial Program. The factorial of a number n is defined by the product of all the digits from 1 to n (including 1 and n). It is denoted by n! and is calculated only for positive integers. Factorial of 0 is always 1. The simplest way to find the factorial of a number is by using a loop. There are two ways to find factorial in PHP: Now, we will see how to calculate the factorial using recursive method in JavaScript. Using Recursive approach. In this approach, we are using recursion to calculate the factorial of a number. Here, we call same function again and again to get the factorial. Using recursion, we have to code less than the iterative approach. Now, we will see an ... 11/10/2019 · Examples: Input : 4 Output : 24 Input : 5 Output : 120. Approach 1: Iterative Method In this approach, we are using a for loop to iterate over the sequence of numbers and get the factorial.
factorial is initialized with 1. factorialNumber will get the result of the prompt (a number is expected) and then, using a cycle, in each step, factorial is multiplied with the index of the step, which is represented by i. When successfully calculated, we show the result using alert. Jobs Programming & related technical career opportunities; ... I need to use a loop to find the factorial of a given number. ... Browse other questions tagged javascript factorial or ask your own question. The Overflow Blog The strange domain names that developers bought ... how to factorize the number in javascript; calculate factorial using loop javascript; Write a program to find factorial of a given number using javascript; factorial of number js; factoral in javascript; javascrtipt factorial; factorial using while loop in js; javascript function to calculate the factorial of a number; factorial javascript for loop
Where: declaration: is used to declare the new variable. expression: is the array or collection object to be iterated. Program to use enhanced for loop example in java. The factorial of a number is the product of all the integers from 1 to that number. For example, the factorial of 6 is 1*2*3*4*5*6 = 720. Factorial is not defined for negative numbers, and the factorial of zero is one, 0! = 1. Source Code # Python program to find the factorial of a number provided by the user. JavaScript is one of mostly used scripting language all over the world. Today in this post we are going to see how to write a program to print Factorial values of a number using plain JavaScript. Lets' see the example… Continue Reading →
PHP program to print factorial of a number: The below program prints factorial of a number using a loop. The PHP echo statement is used to output the result on the screen. The factorial of a number can be calculated for positive integers only and is represented by n!. n! = n*(n-1)*(n-2)*.....*1 0! = 1 Example <! 2. Iterative Java Program to Find Factorial using for loop. In the Iterative approach, Running a for loop for n times where n is the given number. 3. Iterative Java Program to Find Factorial using while loop. The above program is implemented using for loop. Now, we will see the same using a while loop. We represent factorial with ! symbol. For example, 4 factorial is written as 4! = 4 x 3 x 2 x 1. In this example, we'll write a factorial program in JavaScript using a while loop. To find the factorial of a number using while loop, all we need to iterate the loop starting from 1 till the number that is given.
In the first example, using var, the variable declared in the loop redeclares the variable outside the loop. In the second example, using let, the variable declared in the loop does not redeclare the variable outside the loop. When let is used to declare the i variable in a loop, the i variable will only be visible within the loop. Learn HTML and CSS with W3Schools; Learn JavaScript and Ajax with W3Schools; HTML5 Black Book: Covers Css3, Javascript,XML, XHTML, Ajax, PHP And Jquery; HTML5 Application Development Fundamentals: MTA Exam 98-375.NET 4.0 Programming 6-In-1, Black Book; SQL Server 2008 R2 Black Book; Asp 4.0 Projects Covers: net 3.5 And 4.0 Codes, Black ...
C For Loop Learn Its Purpose With Flowchart And Example
Factorial Of A Number In Php Using For Loop Amp Recursion
Javascript Programming What Is Javascript The Most Popular
Patterns In Javascript 3 Amazing Types Of Patterns In
Javascript Tutorial 66 Find Factorial Of A Number Using For Loop In Javascript
Python Extract Manipulate And Analyze Data With 5 Projects
C For Loop Learn Its Purpose With Flowchart And Example
W3schools Python Classes Code Example
Problem Solving In Javascript Today We Ll Discuss About Some
Problem Solving In Javascript Today We Ll Discuss About Some
Simple User Registration Form Example In Angularjs W3adda
Factorial Of A Number Using Javascript Geeksforgeeks
C Switch Case Statement W3adda
Aaron Toponce Linux Gnu Freedom
Best Way To Find The Factorial Of A Number In Java Iterative
Factorial Program In Javascript
Net Fort Walton Beach High School
Find Factorial Of A Number Using Only Javascript Tutorialsmade
Python Program To Make Calculator
A Beginners Guide To Python 3 Programming
Problem Solving In Javascript Today We Ll Discuss About Some
35 Factorial Program In Javascript Using For Loop W3schools
Oracle Pl Sql While Loop With Example
Javascript Build A Factorial Function Using Loop And
Javascript Recursion With Examples
11 Ways To Check For Palindromes In Javascript By Simon
35 Factorial Program In Javascript Using For Loop W3schools
0 Response to "34 Factorial Program In Javascript Using For Loop W3schools"
Post a Comment