24 Javascript Clone File Object
Summary: Copying or cloning an array in Javascript is often confusing for beginners. This article explains different ways to copy or clone array in Javascript and some hidden facts about them. There are some fundamental concepts like mutable and immutable objects in Javascript which you must need to know. Mar 31, 2016 - Observed TypeError - Illegal invocation exception when deep watching an object containing FileList object. #13924
34 Javascript Clone File Object Javascript Nerd Answer
Copy an Object from the WebKit Inspector as Code. To copy an object from the WebKit inspector as code, we can right-click the variable and then click Store as Global Variable from the menu. There's also the copy function in Chrome to copy the object into our clipboard. We can also combine copy with the JSON.stringify to copy it as a string.
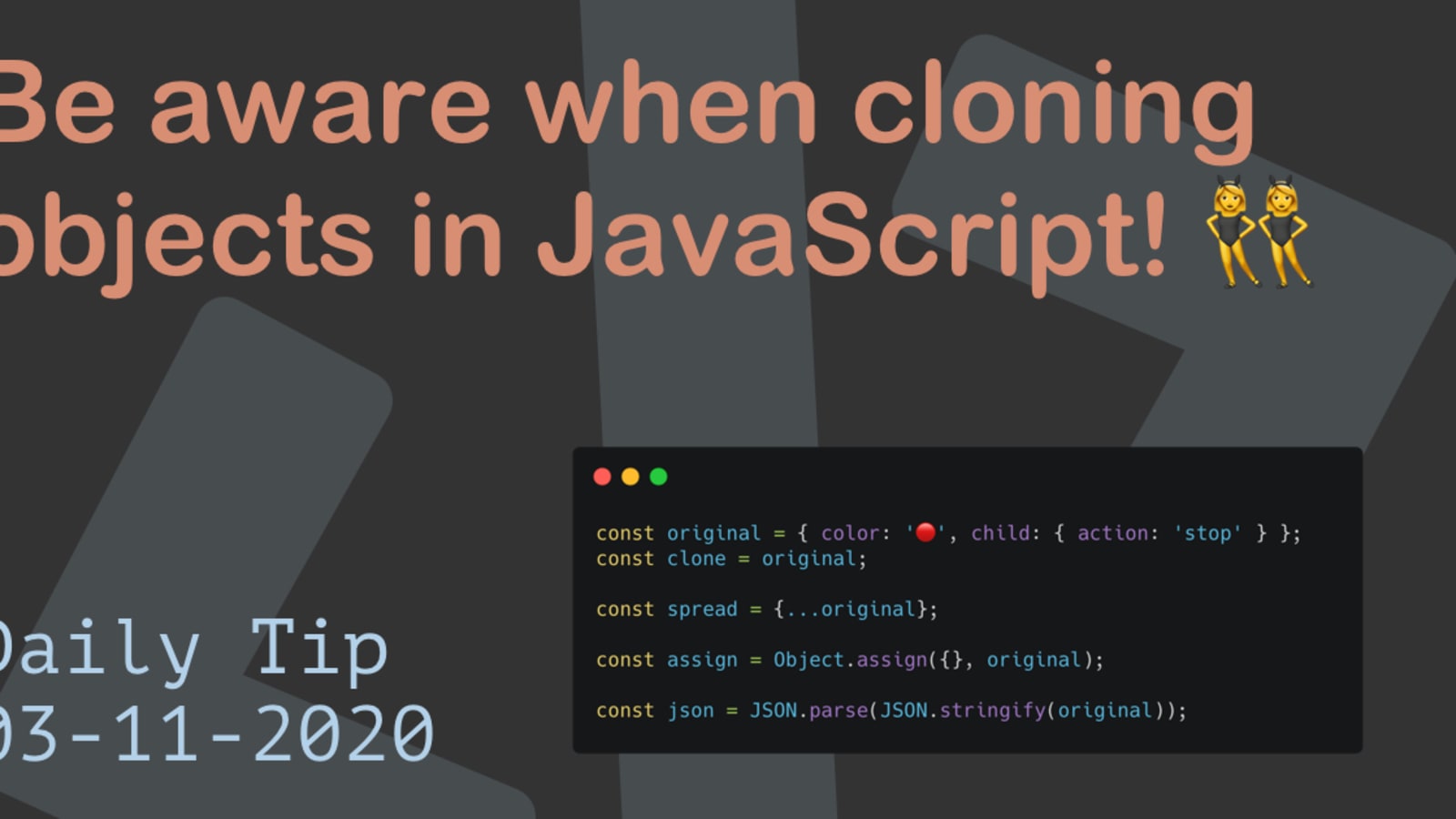
Javascript clone file object. Deep Copying Objects. A deep copy will duplicate every object it encounters. The copy and the original object will not share anything, so it will be a copy of the original. Here's the fix to the problem we encountered using Object.assign(). Let's explore. Using JSON.parse(JSON.stringify(object)); This fixes the issue we had earlier. Cloning an object in JavaScript a task that is almost always used in any project, to clone everything from simple objects to the most complicated ones. As it may seem simple for not seasoned… In case a property inside the original object is an object, it can be shared between the copy and the original one. Notice that by nature, JavaScript objects are changeable and are stored as a reference. So, when assigning the object to another variable, you assign the memory address of the object to the variable.
Oct 02, 2013 - I had a form with plenty of inputs and one (hidden) file input. File had to be selected in modal window. So I cloned the file input in modal into the form. It worked prefectly for Firefox and Opera but not for Chrome for security reasons. I have read plenty of similar solutions but none… The Object.assign () method only copies enumerable and own properties from a source object to a target object. It uses [ [Get]] on the source and [ [Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties. If you're on Windows, right click your file > select properties > copy the Location value.. Here's a screenshot: So your New File would look something like this based on my example: var file = New File("C:\Users\<insert-your-local-computer-name>\Desktop\New Text Document.txt");
what do you mean by file object? you access the input element by id, so the filename is: $('#files').val(). of course in modern browsers this is read only. if you meant the file contents, then with a html 5 browser (safari, chrome, firefox, IE 10+), you can use the file api to read the file. There are an idea to clone an object(or array) by JSON method without deep traversal: function clone (src) { return JSON.parse(JSON.stringify(src)); } But it only supports to clone Object and need JSON support. Dec 08, 2016 - Not the answer you're looking for? Browse other questions tagged javascript file copy clone or ask your own question.
Cloning a JavaScript object is a task that is used mostly because we do not want to create the same object if the same object already exists. There are few ways. By iterate through each property and copy them to new object. Using JSON method as the source object MUST be JSON safe. AngularJS is what HTML would have been, had it been designed for building web-apps. Declarative templates with data-binding, MVC, dependency injection and great testability story all implemented with pure client-side JavaScript! It's important to understand how to clone an object in JavaScript correctly. It is possible to create a shallow copy and a deep copy of an object. A shallow copy of an object references the original. So any changes made to the original object will be reflected in the copy.
Jul 24, 2021 - The File interface provides information about files and allows JavaScript in a web page to access their content. The cloned object is completely independent of the original object. JavaScript offers many ways to create shallow and deep clones of objects. You can use the spread operator (...) and Object.assign () method to quickly create a shallow object duplicate. the same way as any other object - not any other object but plain object. The reason is clear, JSON.stringify reads only own enumerable properties, this is documented. It's unclear which file you're talking about, but as for browser File, file objects may contain size information for starters which would be unreachable if they wouldn't be aware of file contents.
23/11/2019 · In es6, we have spread operator which is also used to clone an object. const obj = {a:1,b:2,c:3}; const clone = {...obj}; console.log(clone); Note: These above two methods are only used for shallow copying of objects but not for deep copying. As you can see, the only data that was altered by our method were the ones were Date objects, which have now been turned in to Unix timestamps. Underscore. The Underscore clone() method works much the same way as Lodash's clone() method. It only provides a shallow copy of the given object, with any nested objects being copied by reference. Javascript object is the collection of properties, and the property is an association between the key-value pair. Javascript objects are reference values, you can't simply just copy using the = operator. Clone Object In Javascript JavaScript offers many ways to copy an object, but not all provide a deep copy.
Cloning a JavaScript object is a task that is used mostly because we do not want to create the same object if it already exists. As we are now aware, objects are assigned and copied by reference. In other words, a variable stores not the object value, but a reference. Create Copy Of JavaScript Object Except For Certain Properties. May 15, 2020 by Andreas Wik. Here's how to create a copy of an object in JavaScript, but without certain properties. Let's say I want to make a copy of this statueObj object below, BUT I don't want the company property in my new object. javascript - Copy/Clone files from File Input to another Input - Stack Overflow If i open a file select, select a file, then click file select again and then click cancel, it forgets the originally selected file
Mar 12, 2021 - Create an independent copy, a clone? That’s also doable, but a little bit more difficult, because there’s no built-in method for that in JavaScript. But there is rarely a need – copying by reference is good most of the time. But if we really want that, then we need to create a new object and ... 1. Shallow copy. To shallow copy, an object means to simply create a new object with the exact same set of properties. We call the copy shallow because the properties in the target object can still hold references to those in the source object.. Before we get going with the implementation, however, let's first write some tests, so that later we can check if everything is working as expected. In general, cloning means copying one value to another. In JavaScript, we do cloning i.e. copying one value to another using JavaScript. To be more precise they are two types of cloning in JavaScript. As a programmer, it might be a beginner or veteran he/she should be able to know the differences between Deep clone and shallow clone.
JavaScript provides several methods to copy, clone, or duplicate arrays and objects. In this tutorial, we would like to share with you the simplest, different and easiest way to copy, clone or duplicate an array and objects. 14/8/2014 · const SIZE = blob.size; var start = 0; var end = BYTES_PER_CHUNK; while (start < SIZE) { if ('mozSlice' in blob) { var chunk = blob.mozSlice(start, end); } else if ('webkitSlice' in blob) { var chunk = blob.webkitSlice(start, end); }else{ var chunk = blob.slice(start, end); } upload(chunk); start = end; end = start + BYTES_PER_CHUNK; } p = ( j = file.length - 1) ? true : false; self.postMessage(blob.name + " Uploaded … Apr 14, 2020 - Learn how JavaScript handles mutable data, such as objects and arrays, and understand how shallow cloning and deep cloning work.
Nov 25, 2020 - There are several ways to shallow clone an object in vanilla JavaScript, and a couple of ways to deep clone an object. Here's what you need to know. Object.assign() performs a shallow copy of an object, not a deep clone. const copied = Object. assign ({}, original ) Being a shallow copy, values are cloned, and objects references are copied (not the objects themselves), so if you edit an object property in the original object, that's modified also in the copied object, since the referenced ... This file was generated by verb-generate-readme, v0.8.0, on November 21, 2018. ... Recursively (deep) clone JavaScript native types, like Object, Array, RegExp, Date as well as primitives. Used by superstruct, merge-deep, and many others!
JavaScript provides 3 good ways to clone objects: using spread operator, rest operator and Object.assign () function. Aside from just cloning objects, using object spread and Object.assign () lets you add or updated properties when creating the clone. Rest operator also gives the benefit of skipping certain properties when cloning. const cloneFood = Object.assign({}, food); {} is the object that is modified. The target object is not referenced by any variable at that point, but because Object.assign returns the target object, we are able to store the resulting assigned object into the cloneFood variable. We could switch our example up and use the following: javascript html object file-upload cloning. Share. Follow asked Jan 21 '13 at 12:17. kingmaple kingmaple. 3,942 5 5 gold badges 28 28 silver badges 43 43 bronze badges. Add a comment | 1 Answer Active Oldest Votes. 3 If you're using jQuery you can ...
1 week ago - Cloning objects is one of the most commonly used operations in the JavaScript universe. In this... Code language: CSS (css) The reason is that the address is reference value while the first name is a primitive value. Both person and copiedPerson references different objects but these objects reference the same address objects.. Deep copy example. The following snippet replaces the Object.assign() method by the JSON methods to carry a deep copy the person object: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Introduction to JavaScript Clone Object The object which does not exist but that reference is to be used in another new object that is the same object is copied through another new object the instance will not be created again is known as cloning object. Jul 18, 2010 - When clicked, it lets a user select ... the element's change event is fired. You can access the list of files from event.target.files, which is a FileList object. Each item in the FileList is a File object.... Everything in the JavaScript world is an Object. We often need to clone an Object, and when working with TypeScript, preserving the object type may also be required. This article will explore the options of deep clone an Object with TypeScript. The implementations of the clone are not depended on external libraries. Shallow copy
Clone the Object Using Object.assign () // program to clone the object // declaring object const person = { name: 'John', age: 21, } // cloning the object const clonePerson = Object.assign ( {}, person); console.log (clonePerson); // changing the value of clonePerson clonePerson.name = 'Peter'; console.log (clonePerson.name); console.log (person.
Clone Javascript Object With Json Stringify By Mayank Gupta
Super Clone Pro Luminositycrm Llc Appexchange
What Is The Most Efficient Way To Deep Clone An Object In
The Node Js Fs Module Changing Ownership And Copying Files
Java Copy File Directory New Name Rename With Example
How To Transfer Files And Data Between Angular Clients And
An Example Of How To Create And Clone A Gitlab Repository
Uploading To Amazon S3 Directly From A Web Or Mobile
How To Build A Drag Amp Drop Form With Fastapi Amp Javascript
Python Copy File Using Shutil Copy Shutil Copystat
Github Cubiclesoft Js Fileexplorer A Zero Dependencies
Be Aware When Cloning Objects In Javascript Dev
Javascript Clone Object Code Example
3 Ways To Clone Objects In Javascript Samanthaming Com
How To Copy Files In Sas Enterprise Guide The Sas Dummy
Upload Image To Blob Storage With Vscode App Service Cosmos
How To Clone Object In Javascript Example Tutorial
Astrea Clone Astrea It Services Pvt Ltd Appexchange
Copy Files To Oracle Oci Cloud Object Storage From Command
How To Transfer Files And Data Between Angular Clients And
How To Create Your Very Own File Transfer Program In Node Js
Javascript Write To Text File Code Example
How To Deep Clone Javascript Object Red Stapler
0 Response to "24 Javascript Clone File Object"
Post a Comment