29 Aws Sdk Javascript S3 Download File
Uploading Files in AWS S3 Bucket through JavaScript SDK with Progress Bar. Shruti Shresth. Nov 19, 2018 · 4 min read. Amazon S3 Bucket is a cloud storage resource by Amazon Web Services (AWS ... This exercise has the code which uses AWS SDK for JavaScript v3, which you would have got after finishing Exercise1: backend performs create, delete, get, list and update operations on DynamoDB. frontend does put, get, delete operations using an S3 browser client.
Nodejs Aws S3 File Upload Download Example
The other day I needed to download the contents of a large S3 folder. That is a tedious task in the browser: log into the AWS console, find the right bucket, find the right folder, open the first file, click download, maybe click download a few more times until something happens, go back, open the next file, over and over.
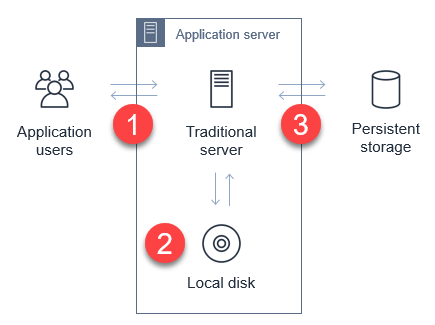
Aws sdk javascript s3 download file. On December 15th, 2020, we announced the general availability of the AWS SDK for JavaScript, version 3 (v3). This blog shows you how to generate a presigned URL for an Amazon S3 bucket using the modular AWS SDK for JavaScript. Motivation A presigned URL gives you access to the object identified in the URL, provided […] To install aws-sdk, you can simply use npm package manager to do below. npm install aws-sdk And here is the simple code to generate the upload URL. First, you need to create S3 bucket object. const s3bucket = new AWS.S3({ accessKeyId: process.env.AWS_ACCESS_KEY, secretAccessKey: process.env.AWS_SECRET_KEY, signatureVersion: 'v4', region ... // Attempt to get the object from S3 let data = await S3.getObject(params).promise()
26/6/2021 · AWS SDK with Javascript: Multi-Files Download from S3 # aws # javascript # zip # download DEV Community – A constructive and inclusive social network for software developers. Examples of how to access various services using the SDK for JavaScript. Step 6. Upload File Via React S3. If you are not using other AWS services then you don't need the full aws-sdk which will unnecessarily increase your bundle size. We will use another library named react-s3. First, install it. yarn add react-s3. Then create a new component named UploadImageToS3WithReactS3 and add the following code
11/9/2018 · AWS SDK JavaScript S3 Managed Download. The JavaScript SDK Managed Download can be used for custom S3 downloads. The client can configure options for the Managed Download such as chunk size and number of concurrent transfers. Installing In Node.js. This package only works in Node.js versions 6+ currently. Amazon Simple Storage Service (Amazon S3) is a web service that provides highly scalable cloud storage. Amazon S3 provides easy to use object storage, with a simple web service interface to store and retrieve any amount of data from anywhere on the web. The JavaScript API for Amazon S3 is exposed through the AWS.S3 client class. In this example, a series of Node.js modules are used to obtain a list of existing Amazon S3 buckets, create a bucket, and upload a file to a specified bucket. These Node.js modules use the SDK for JavaScript to get information from and upload files to an Amazon S3 bucket using these methods ...
May 21, 2019 - Policy document with S3 permissions to specific folder in bucket ... Once we click on Allow button AWS cognito Identity poolId will be created and wizard will be moved to dashboard. Click on Sample code and select need Platform to get IdentityPoolId. Following image shows AWS SDK for javascript ... 12/8/2018 · mkdir nodeS3 npm init -y npm install aws-sdk touch app.js mkdir data Next, you need to create a bucket for uploading a file (after configuring your AWS CLI). Let’s create a bucket with the s3 command. aws s3 mb s3://your.bucket.name Uploading File. First of all, you need to import the aws-sdk module and create a new S3 object. Use AWS Lambda to encapsulate proprietary logic that you can invoke from browser scripts without downloading and revealing your intellectual property to users. ... You can browse the SDK for JavaScript examples in the AWS Code Sample Catalog.
For more information, see the Amazon SDK for JavaScript v3 Developer Guide ... How to create the package.json manifest for your project. How to install and include the modules that your project uses. How to create an Amazon Simple Storage Service (Amazon S3) service object from the AWS.S3 client ... The AWS SDK for JavaScript enables you to directly access AWS services from JavaScript code running in the browser. Authenticate users through Facebook, Google, or Login with Amazon using web identity federation. Store application data in Amazon DynamoDB, and save user files to Amazon S3. Step 2: Backend using S3 sdk, calling S3API to generate a pre-signed URL. Step 3: Return the pre-signed URL to frontend web applicatoin. S t ep 4: Frontend use HTTP call to upload the file to S3. In this flow, frontend application doesn't need to set up the AWS credential. The presigned-URL should be expired in certain time (maybe 1 second ...
Get started quickly using AWS with the AWS SDK for JavaScript in Node.js. The SDK helps take the complexity out of coding by providing JavaScript objects for AWS services including Amazon S3, Amazon EC2, DynamoDB, and Amazon SWF. The single, downloadable package includes the AWS JavaScript ... This section explains how to download objects from an S3 bucket. 31/12/2018 · Is there a javascript code to download a file from Amazon S3? I couldn't find anything useful resources on the internet. So, if any of you have done something similar to this can you post the code? Well, is it possible to write a javascript code to download a file from S3?
The AWS SDK for .NET enables .NET developers to easily work with Amazon Web Services and build scalable solutions with Amazon S3, Amazon DynamoDB, Amazon Glacier, and more. AWS S3/S3 Bucket Amazon simple storage service (Amazon S3) is used as storage for the internet. Setting a Simple Bucket Policy. Create a Node.js module with the file name s3_setbucketpolicy.js. The module takes a single command-line argument that specifies the bucket whose policy you want to apply. Configure the SDK as previously shown. Create an AWS.S3 service object. Bucket policies are specified in JSON. Download an object from an Amazon S3 bucket to a file using this AWS SDK for Ruby code example.
28/6/2021 · If you want to download multiple files as zipped from AWS S3, and you have your server to take care of the file metadata handlings, then this article may help you understand how the process works. AWS doesn't provide the default multi-files download, so in order to achieve you may want to add lambda function or to use your own implemented service. To Download using AWS S3 CLI : aws s3 cp s3://WholeBucket LocalFolder --recursive aws s3 cp s3://Bucket/Folder LocalFolder --recursive To Download using Code, Use AWS SDK .. To Download using GUI, Use Cyberduck .. For a more detailed explanation, check this out! // Attempt to get the object from S3 let data = await S3.getObject(params).promise()
I'm trying to download a pdf file from S3, but everytime I download it to my local machine, I receive the error, "Error: Failed to load PDF". It seems I am still downloading a blob instead of a file, but have tried everything and looked through too many stackoverflow solutions. Downloading files. ¶. The methods provided by the AWS SDK for Python to download files are similar to those provided to upload files. The download_file method accepts the names of the bucket and object to download and the filename to save the file to. import boto3 s3 = boto3.client('s3') s3.download_file('BUCKET_NAME', 'OBJECT_NAME', 'FILE_NAME') Use encryption keys managed by Amazon S3 or customer master keys (CMKs) stored in Amazon Web Services Key Management Service (Amazon Web Services KMS) - If you want Amazon Web Services to manage the keys used to encrypt data, specify the following headers in the request. x-amz-server-side-encryption. x-amz-server-side-encryption-aws-kms-key-id
I came here looking for away to download a s3 file on the client side. Here is how I solved it: As, I can not store my s3 auth keys on client side, I used my server-side scripts to generate a pre-signed url and send it back to client like: const AWS = require ('aws-sdk') const s3 = new AWS.S3 () AWS.config.update ( {accessKeyId: 'your access ... Step 1 : In the head section of your page include javascript sdk and specify your keys like this: Step 2 : Now create a simple html form with a file input. alert ("File uploaded successfully."); To upload the file successfully, you need to enable CORS configuration on S3. Generally, it is not advisable to display your keys directly on page, so ... To download files from S3, you will need to add the AWS Java SDK For Amazon S3 dependency to your application. Here is the Maven repository for Amazon S3 SDK for Java. Gradle Dependency. Add the following dependency to the build.gradle file: implementation group: 'com.amazonaws', name: 'aws-java-sdk-s3', version: '1.11.1009' Maven Dependency
How to use the AWS SDK for Java's TransferManager class to upload, download, and copy files and directories using Amazon S3. AWS Documentation AWS SDK for Java Developer Guide Upload Files and Directories Download Files or Directories Copy Objects Wait for a Transfer to Complete Get Transfer Status and Progress More Info Menu AWS S3: how to download file instead of displaying in-browser 25 Dec 2016 on aws s3. As part of a project I've been working on, we host the vast majority of assets on S3 (Simple Storage Service), one of the storage solutions provided by AWS (Amazon Web Services). Feb 13, 2015 - Hi, I can get the data from my S3 bucket. However, the file is in memory. I'm wondering how to download the file. I don't see any documentation on this. s3.getObject(params, functio...
Use requests with a Node.js stream object for asynchronous calls with the SDK for JavaScript. Step 3: Download or Read Pre Signed URL from AWS S3. Once you have received the pre signed URL from S3, you can download the file or open the document in document viewer. In my use case, I download the PDF file and draw it in canvas using PDF.js. In that case my document is being secure and not shareble from one environemnt to other environment. With Amazon S3 Select, you can create SQL expressions to select a subset of CSV or JSON records from files stored in Amazon S3. The AWS SDK for JavaScript provides the tools to use this API to process events asynchronously in Node.js, or synchronously in browser environments. Let us know what you think about this feature in the comments below!
Also, create two additional files: aeeiee-s3.js and aeeiee-s3-views.php. The JavaScript file will hold all the JS code to handle uploading files to our S3 bucket while the aeeiee-s3-views.php file will handle displaying HTML content on the page. We will create a plugin file with the information below. May 27, 2020 - Before using this code snippet please make sure that you have your own s3 bucket (if you dont – see our tutorial: How to create bucket). You also have to have file called “test.txt” in your bucket. Check this article to learn how to upload files. 'use strict' const AWS = require('aws-sdk') ... AWS Java SDK 2 - S3 File upload & download. In this tutorial, we will walk through new AWS SDK 2.0 for doing object level operations on S3 bucket. We will specifically cover PutObject, GetObject and GetUrl operation on S3 Objects using AWS SDK 2.0.
Example AWS S3 Multipart Upload with aws-sdk for Node.js - Retries to upload failing parts - aws-multipartUpload.js ... Download ZIP. Example AWS S3 Multipart Upload with aws-sdk for Node.js - Retries to upload failing parts ... how would I tell it to extract all the files in the folder rather than individual files?
Browser Based Uploads Using Post Aws Signature Version 2
Cloud Object Storage Store Amp Retrieve Data Anywhere
Getting Started With Amazon S3 And Python
How To To Upload Files To S3 With The Aws Javascript Sdk And
Upload Files To Aws S3 In Node Js By Soni Pandey Medium
How To Manage Amazon S3 Files From Your Server Side Code
Aws Sdk With Javascript Multi Files Download From S3 Dev
Upload A File To An S3 Bucket Using The Aws Sdk Lagu Mp3
Angular 6 Nodejs Express Restapis Upload File Download File
Do Load Testing Upload Amp Download From Aws S3 Via Jmeter
Uploading To Amazon S3 Directly From A Web Or Mobile
Use Presigned Url To Upload Files Into Aws S3 By Kulasangar
Nodejs Express Restapis Download File From Amazon S3 Using
Aws Sdk For Javascript Developer Guide For Sdk V2
How To Handle Big Data With Node Js Aws Kinesis Aws S3 And
How To Copy File Across Buckets Using Aws S3 Or Aws Sdk Gem
Uploading Files To Aws S3 With Node Js
Amazon S3 Bucket Image Upload Using Node Js By Edison
Using The Aws Javascript Sdk In The Browser Modern Web
Cannot Download A Larger Than 2gb From S3 Issue 2916 Aws
Move File From One Folder To Another In Aws S3 Nodejs Code
Download Amp Upload From To Aws S3 Using Node Js By Tojo
Ozenero Mobile Amp Web Programming Tutorials
What Is Aws S3 Pre Signed Url How To Share Your S3 Bucket
Developer Preview Aws Sdk For Javascript In The Browser
How To Read Or Download Or Stream Document From S3 Using Aws
Writing Javascript Applications With The Aws Sdk Tls303
Php Download File From S3 Bucket And Save Locally Using Api
0 Response to "29 Aws Sdk Javascript S3 Download File"
Post a Comment