25 Javascript Sort Array Of Numbers
How to sort array of objects in ascending and descending order of React app How to find tomcat version installed| check java version used in tomcat Solution for com.fasterxml.jackson.databind.exc.InvalidDefinitionException: Java 8 date/time type Multiple ways to create a array with random values in javascript How to write unit testing for ... Oct 29, 2020 - Learn how to use the sort() method on the array object to sort an array of numbers in JavaScript.
Sort In Descending Order With Custom Method In Javascript
JavaScript Searching and Sorting Algorithm: Exercise-3 with Solution. Write a JavaScript program to sort a list of elements using Heap sort. In computer science, heapsort (invented by J. W. J. Williams in 1964) is a comparison-based sorting algorithm.
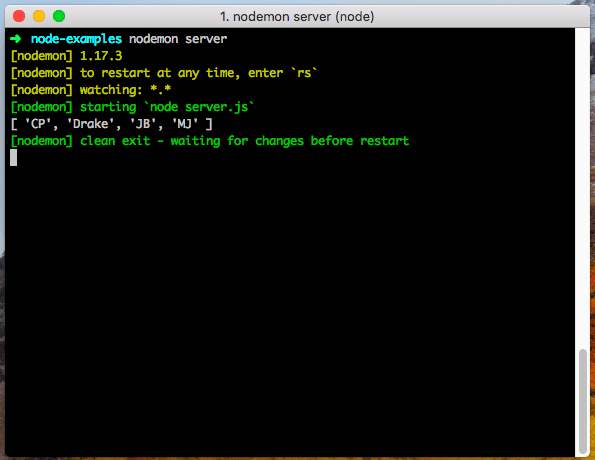
Javascript sort array of numbers. JavaScript has built-in sort () method by default it can only sort elements in ascending & alphabetical order, where it is converting elements into strings. For sorting numbers, we need to pass the compare function as an argument to the sort () method. Sorting array of numbers in Ascending order The sort () method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values. The time and space complexity of the sort cannot be guaranteed as it depends on the implementation. 7/7/2021 · The JavaScript Array.sort () method is used to sort the array elements in-place and returns the sorted array. This function sorts the elements in string format. It will work good for string array but not for numbers. For example: if numbers are sorted as string, than “75” is bigger than “200”.
The compare function compares all the values in the array, two values at a time (a, b). When comparing 40 and 100, the sort () method calls the compare function (40, 100). The function calculates 40 - 100 (a - b), and since the result is negative (-60), the sort function will sort 40 as a value lower than 100. In this short article we saw how to use the built-in .sort() method to easily sort arrays in JavaScript. This applies to any type of data, including strings, numbers, or even objects. The sort order is determined by the compareFun callback method, which is what allows you to determine the sort order or what object properties determine the sort ... Sort an array of objects in JavaScript dynamically. Learn how to use Array.prototype.sort() and a custom compare function, and avoid the need for a library.
The sort( ) method sorts the array elements in place and returns the sorted array. By default, the sort order is ascending, built upon converting the elements into strings and then comparing their sequences of UTF-16 code unit values. Number sorting can be incorrect as the sort( ) method sorts numbers in the following order: "35" is bigger than ... array.sort () method invoked without arguments sorts the elements alphabetically. That's why using array.sort () to sort numbers in ascending order doesn't work. But you can indicate a comparator function array.sort (comparator) to customize how the elements are sorted. I recommend numbers.sort ( (a, b) => a - b) as one of the shortest way ... Definition and Usage. The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana").
The sort( ) method accepts an optional argument which is a function that compares two elements of the array.. If the compare function is omitted, then the sort( ) method will sort the element based on the elements values.. Elements values rules: 1.If compare (a,b) is less than zero, the sort( ) method sorts a to a lower index than b.In other words, a will come first. A "basic sort" in Javascript is as simple as using ARRAY.sort (), but take extra note of the funky number sorting. Javascript is not wrong, that's just the way of how it works - All the elements will be converted and compared as strings. So in this case, "4" comes before "5". Thus the final result of 12, 41, 5, 97. JavaScript makes it easy for us, to sort an array. If we have an arbitrary array, it is enough to use the function sort () in the following way to sort the array: var ArrA = ['D','A','C','B']; ArrA.sort (); alert (ArrA); However, there may occur problems, if we have an array of numbers. What happens if we apply the function from above to an ...
May 08, 2020 - Get code examples like "how to sort numbers in array in javascript" instantly right from your google search results with the Grepper Chrome Extension. Write a function named sortBackwards that takes in an array of numbers and returns the same array, with the numbers sorted, highest to smallest. ... write a JavaScript function that takes 1 argument : an array of numbers. This function sorts the elements of input array in ascending order. The sort() method sorts the items of an array. It can sort either alphabetic or numeric values. By default, the sort() method sorts the items in ascending order (up). However, if we to sort array ...
The sort () method used above is helpful in sorting array alphabetically and does not produce desired results when it is used with numbers because the sort () method converts numbers into strings which means 30 becomes "30" and 45 becomes "45". To fix this problem, we can use compare formula in sort () method as shown below. @Web_Designer Counting sort is linear regarding the number range, not the array. For example, sorting [1,1000000] will take more than 2 steps, since the algorithm will have to scan each array index between 1 to 1000000 to see which cell's value is greater than 0.- ytersFeb 13 '18 at 15:05 2 Apr 30, 2020 - Using .sort() on arrays without providing a compare function will convert each item to a string. Then, JavaScript uses the string representation of each item and compares them in UTF-16 code units order. And here’s the catch. You want the number 9 come before the number 80 when sorting data.
To customize the sort order, you pass it a function to compare two items in the array. sort will pass to your function two items in the array (conventionally the parameters a and b in your function). sort looks for one of three different outcomes from your function: If your function returns a value less than 0, a comes first. The arr.sort () method is used to sort the array in place in a given order according to the compare () function. If the method is omitted then the array is sorted in ascending order. Get code examples like"sort array of numbers in array in javascript". Write more code and save time using our ready-made code examples.
I am trying to sort an array which contains strings, numbers, and numbers as strings (ex. '1','2'). I want to sort this array so that the sorted array contains numbers first and then strings that contain a number and then finally strings. We need to sort the numbers of an array in descending order, for that we are using sort() method like .sort(function(a, b) {return b-a}) in the <script> block. Onclick of the button " Click " in the HTML code fires the function myFunction() in the <script> block, at the same time .sort(function(a, b) {return b-a}) method sorts and sets the ... May 04, 2020 - Okey dokey, the binary tree hiatus continues, because my node deletion post got out of hand and I nee...
4/9/2020 · TL;DR — Sort an array of numbers in ascending order using: myArray.sort((a, b) => a - b); Arrays in JavaScript are data structures consisting of a collection of data items. Because Javascript is not a typed language, Javascript arrays can contain different types of elements - strings, numbers, undefined, etc. It’s most often a good idea to have all items in an array be of the same type however. One of the many operations that can be performed on an array … Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: How to sort javascript array of numbers. April 24, 2021 May 1, 2021 AskAvy . sort() method it will not work If you simply try to sort a numeric array, because the sort() method sorts the array elements alphabetically. one can still sort an array of numbers correctly through utilizing a compare function.
Use.sort ((a,b) => a-b) In order to sort a list in numerical order, the Array.prototype.sort () method needs to be passed a comparison function. To sort the array numerically in ascending order,... Apr 28, 2021 - In JavaScript, we can sort the elements of an array easily with a built-in method called the sort( ) function. However, data types (string, number, and so on) can differ from one array to another. This means that using the sort( ) method alone is not always an appropriate solution. In this If you simply try to sort a numeric array using the sort () method it will not work, because the sort () method sorts the array elements alphabetically. But, you can still sort an array of integers correctly through utilizing a compare function, as shown in the following example:
Code language: JavaScript (javascript) To sort an array of numbers numerically, you need to pass into a custom comparison function that compares two numbers. The following example sorts the scores array numerically in ascending order. Jan 04, 2021 - The JavaScript sort() method reads the values in an array and returns those values in either ascending or descending order. A string containing either numbers or strings can be sorted. Home» Sort an Array of Objects in JavaScript Sort an Array of Objects in JavaScript Summary: in this tutorial, you will learn how to sort an array of objects by the values of the object’s properties. To sort an array of objects, you use the sort()method and provide a comparison function that determines the order of objects.
May 11, 2020 - JavaScript has its own build-in sorting methods and in this article, we will cover how to sort an array in JavaScript. Let’s start with the Sorting Algorithm concept. ... In computer science, a sorting algorithm is an algorithm that puts elements of a list in a certain order.
Sort An Array According To The Order Defined By Another Array
Sorting Arrays And Collections In Javascript Experts Exchange
Sort Arrays With Javascript Methods
Javascript Not Sorting Numeric Arrays Correctly Learn Web
Implementing Counting Sort With Javascript Dev Community
How To Sort Arrays In Javascript
Median Of Two Sorted Arrays With Different Sizes In O Log Min
Algorithms With Javascript Median Of Two Sorted Arrays By
How Does Sort Works In Javascript Stack Overflow
Java67 How To Sort An Array In Descending Order In Java
Sort Array In Ascending Order In C
Ozenero Mobile Amp Web Programming Tutorials
Sorting Objects In Javascript E C How To Get Sorted Values
How To Sort Array With Numbers Alphanumeric Alphabets In
Javascript Fundamental Es6 Syntax Get The Median Of An
Javascript Array Sort How To Sort Array In Javascript
Javascript How To Sort An Array From Big Number To Small
Javascript Sort Array Of Objects Code Example
The Fastest Way To Find Minimum And Maximum Values In An
Sort An Array With A Javascript Do While Loop Using Bubble Sort
Javascript Sorts An Array Of Numbers Using The Bubble Sort
How To Sort An Array In Javascript By Bahadir Mezgil Itnext
How To Sort Arrays In Javascript
0 Response to "25 Javascript Sort Array Of Numbers"
Post a Comment