32 Draw 3d Shapes Javascript
Because as all the following animation steps were plain 2D, I couldn't use a 3D renderer such as Three.js. And so I had to figure out how to render a 3D shape using only the Canvas 2D API. In this article, I'll show you how I finally did it. I'll first explain how to render a basic shape from a 3D scene using the JavaScript Canvas 2D API. How to draw shapes in javascript. Currently Enrolling S Example: Python3 Don't press . Markers are placed at each waypoint along the route widgets import DrawingWidget import hyperbolic We can manually draw shapes by consecutive commands giving coordinates. Rectangle functions You can draw three different types of rectangles: clearRect(x, y, w ...
Lesson 6 Drawing 3d Shapes Earth 801 Special Topics In
Next we define a uniform variable uColor. As opposed to attributes, uniform variables are constant. When the shader is executed for each vertex and pixel on the screen, the uniform value will remain the same at every call. We use it to define the colour of the shape we will be drawing. We will pass a value for this colour from JavaScript.
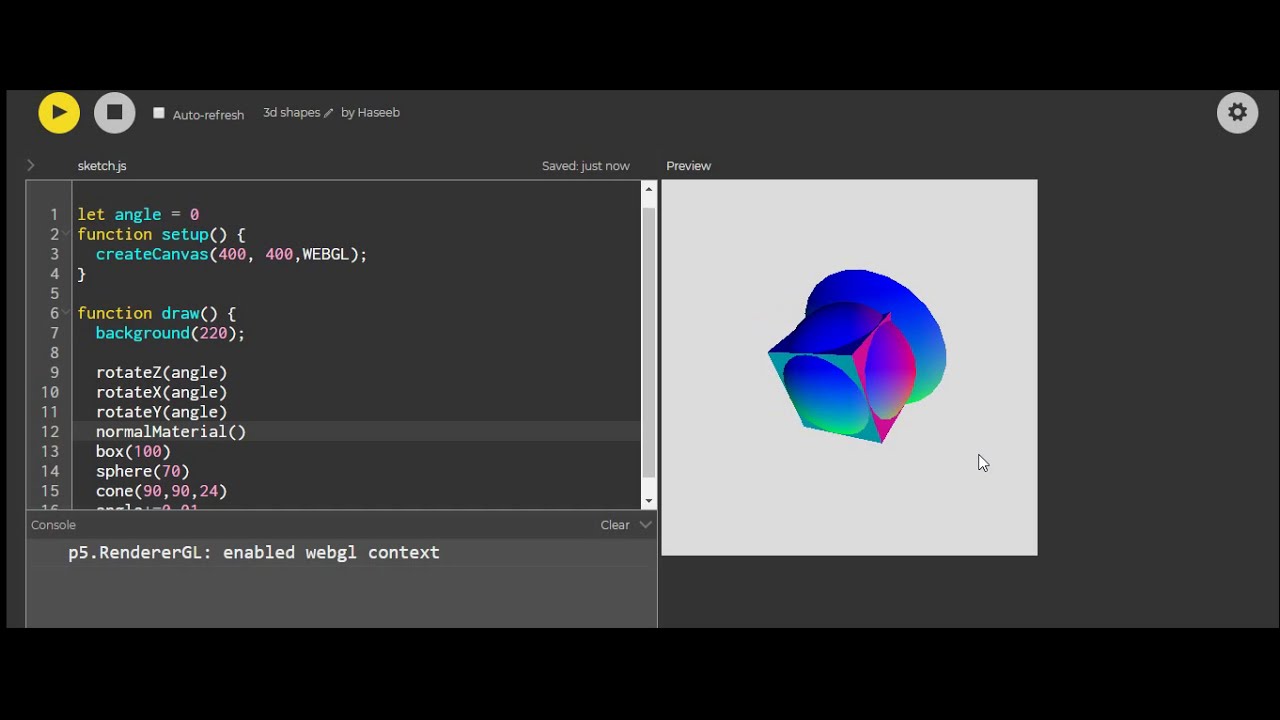
Draw 3d shapes javascript. Drawing Shapes with the JavaScript Canvas API JavaScript. By Joshua Hall. ... To keep things simple we'll stick with 2D shapes, but with the webgl context, 3D is also possible. For our example we'll need our canvas to be fullscreen but setting the size using CSS creates a strange blurry effect, which we obviously don't want, so we'll ... Now that we have set up our canvas environment, we can get into the details of how to draw on the canvas. By the end of this article, you will have learned how to draw rectangles, triangles, lines, arcs and curves, providing familiarity with some of the basic shapes. Working with paths is essential when drawing objects onto the canvas and we will see how that can be done. Using these properties, Fabric.js will draw the shape in such a way that its top-left corner of the shape is at placed this point. Except for the fabric.Line class, all other shapes need these ...
Cango3D. Cango3D provides methods for creating, rendering and animating 3D outline paths, filled shapes and stroke text on a 2D canvas. Complex 3D objects can be constructed by maneuvering shapes, acting as panels, forming the 3D object. The current version of Cango3D is 11v00, and the source code is available at Cango3D-11v00.js. I am learning WebGL from few weeks and I've got a problem with drawing some 3D shapes. Guess I am corectly counting vertices and indices, as well as a color for each triangle, but it does not work. ... javascript 3d webgl. Share. Improve this question. Follow edited Oct 30 '16 at 10:35. gman. Javascript Drawing Viewer (Firefox 2+, Safari 3+, IE is known to fail) Full Dataset: line_study.zip (241MB) Version 1.0, May 6, 2008. This archive contains the initial and registered drawings, prompt and drawing pages, instruction page, models, camera views, and lighting environments. Gauge Settings
Let's learn how to draw shapes with JavaScript and create fabulous Fibonacci flowers all from scratch with just plain vanilla JavaScript. In this HTML5 Canva... Creating 3D objects using WebGL. « Previous. Next ». Let's take our square plane into three dimensions by adding five more faces to create a cube. To do this efficiently, we're going to switch from drawing using the vertices directly by calling the gl.drawArrays () method to using the vertex array as a table, and referencing individual ... Canvas allows drawing and pixel manipulation using JavaScript API. This drawing has to be performed on canvas' context. Think of context as a page to paint and API as available tools (or brushes). The context can either be "2d" or "webgl"(3d). For the purpose of this introduction we will always assume "2d" context.
Create a Drawing App with HTML5 Canvas and JavaScript. by William Malone. This tutorial will take you step by step through the development of a simple web drawing application using HTML5 canvas and its partner JavaScript. The aim of this article is to explore the process of creating a simple app along the way learn: See the Pen javascript-drawing-exercise-1 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: javascript Drawing Exercises. Next: Write a JavaScript program to draw a circle. Standard Draw 3D is a Java library with the express goal of making it simple to create three-dimensional models, simulations, and games. If you have not used StdDraw3D before, make sure to go through the basic tutorial before coming here. This document is the official reference manual for Standard Draw 3D. Listed below is every method
Learn how to draw 3D shapes like a cube, cone, cylinder and torus using Corel Painter and other digital art software. Create accurate primitive shapes withou... You can add various shapes to your map. A shape is an object on the map, tied to a latitude/longitude coordinate. The following shapes are available: lines, polygons, circles and rectangles. You can also configure your shapes so that users can edit or drag them. Polylines. To draw a line on your map, use a polyline. Now, getting back to drawing the cube. First, create an html file with any name you like, say "cube.html" and put the html template I've provided above in it. Since most of the code we'll use to draw the cube and other shapes is based on javascript, our code will go into the script tags. I shall avoid multiple files for the sake of ...
Drawing Shapes. In this lesson, we will learn how to draw simple shapes using HTML5 canvas. We are going to draw circles, rectangles and polygons in different colours. Setting up. First of all, create a new folder where you will store all your code. If you have trouble coming up with a name, I named mine LunarLander. Draw 3d shapes javascript. 4 Graphics And Rendering In Three Js Programming 3d. An Introduction To Three Js The Humaan Blog. 3d Shapes Drawing Art. Ideas For Teaching 3d Shapes In Kindergarten You Clever Monkey. Create A 3d Product Landing Page With Threejs And React. Here then is some of the code that draws the editing display with a 3D projection in a 2D context. The fundamental things going on here are sortFacets() and drawCubes(). This is what produces the 3D projection that gives the illusion of a solid shape. The other code here has to do with updating the WebGL view and drawing elements of the editing UI.
The CanvasRenderingContext2D interface provides basic methods to draw straight lines and curved lines to draw complex shapes. But as the rectangle is a basic shape, Canvas API gives us dedicated ... Afterwards we add these shape objects to the stage and call stage.update() which will do the actual drawing in the canvas. Below you can see JavaScript code for creating and drawing circle shape which is then added to the stage. For code used to draw other shapes, please refer to the codepen for this post. However, when it comes to displaying 3D shapes things become less easy, as 3D geometry is more complex than 2D geometry. To do that, you can use dedicated technologies and libraries, like WebGL ...
jQuery dRawr is a jquery and HTML5 canvas based drawing plugin that lets users to draw shapes with mouse. The plugin comes with a lot of useful tools and brushes. The drawing board wrap around HTML5 canvas element and generates all necessary tools (i.e pencil, pen, eraser, spray paint, color picker, mover etc.) to draw shapes. How to create Animated 3D canvas object in HTML5. Custom drawn 3d object on canvas (html5). Today's lesson very interesting, we'll learn how to create 3D objects using HTML5. This is our first lesson on the practice HTML5. In this tutorial we will make a quadrangular star. Which will rotate around its axis. Drawing 3D shapes. Rotating 3D shapes. Generating 3D shapes. This is the currently selected item. Next lesson. Advanced development tools. Sort by: Top Voted. Rotating 3D shapes. Our mission is to provide a free, world-class education to anyone, anywhere. Khan Academy is a 501(c)(3) nonprofit organization. Donate or volunteer today! Site ...
A few primitive shapes can be drawn directly onto the graphics context in JavaScript. The most common shapes for HTML5 and CSS3 programming that most web developers use are rectangles and text. Drawing 3D shapes. So now that we have a representation of our cube, we need to find a way to draw it. To draw a 3D shape on a 2D surface, we need to "project" it. Imagine shining a light behind the cube and projecting its shadow onto a screen. The simplest form of projection is an orthogonal projection, which is what you would get if the beams ...
How To Render 3d In 2d Canvas Base Design
Create A 3d Product Landing Page With Threejs And React
Creating Custom 3d Objects For Use With Three Js
4 Graphics And Rendering In Three Js Programming 3d
Webgl And Javascript Drawing Simple 3d Shapes Using Three Js
Creating 3d Cube A Practical Guide To Three Js With Live Demo
Creating 3d Cube A Practical Guide To Three Js With Live Demo
Learning 3d Graphics With Three Js Dynamic Geometry Steemit
10 Javascript Libraries To Draw Your Own Diagrams 2020 Edition
Building A 3d Engine With Javascript Sitepoint
Draw Dimension Lines Along With 3d Cube Using Three Js
Generic 3d Shape To Add Any Svg Image On Surface Issue 339
Molecular Drawing Questions Marvinsketch
Let S Build 3d Procedural Landscape With React And Three Js
How To Write A 3d Modeling Application In Javascript
How To Draw In 3d The Complete Guide For Beginners Improve
How To Export A 3d Ggb Model To Use In P5 Js Geogebra
5 Ways To Draw 3d Shapes Wikihow
What Are The Names Of 2d And 3d Shapes Theschoolrun
How To Build A Color Customizer App For A 3d Model With Three
Developing 3d Web Apps With Three Js By Tom Castagna
3d Data Visualization With React And Three Js By Peter
Html5 Render Open Data On A 3d World Globe With Three Js
Ideas For Teaching 3d Shapes In Kindergarten You Clever Monkey
Create A Basic 3d Shapes In Html5 With Threejs Linux Tutorial
What Are Different 3d Shapes Definitions And Examples
How To Create 3d Geometries In Webgl And P5 Js Geeksforgeeks
Make A Rotatable 3d Product Boxshot With Three Js
0 Response to "32 Draw 3d Shapes Javascript"
Post a Comment