25 Javascript Add Element To Array
Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array. #2 unshift - Insert an element at the beginning of the array. #3 spread operator - Adding elements to an array using the new ES6 spread operator. #4 concat - This can be used to append an array to ... In JavaScript, by using push () method we can add an element/elemets to the end of an array. EXAMPLE: Adding element to the end of an array. In the above code snippet we have given Id as " myId "to the second <p> element in the HTML code. There is a function myFunction () in the <script> block which is connected to the onclick of the HTML ...
Javascript Tutorial Adding Elements To Array In Javascript
The size of the array cannot be changed dynamically in Java, as it is done in C/C++. Hence in order to add an element in the array, one of the following methods can be done: By creating a new array: Create a new array of size n+1, where n is the size of the original array. Add the n elements of the original array in this array.
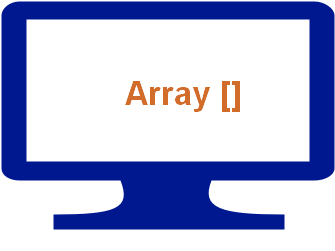
Javascript add element to array. In the above program, the splice () method is used to add an object to an array. The splice () method adds and/or removes an item. The first argument represents the index where you want to insert an item. The second argument represents the number of items to be removed (here, 0). The third argument represents the element that you want to add to ... Without Mutating. Actually, all unshift/push and shift/pop mutate the source array.. The unshift/push add an item to the existed array from begin/end and shift/pop remove an item from the beginning/end of an array.. But there are few ways to add items to an array without a mutation. the result is a new array, to add to the end of array use below code: The JavaScript Array.splice () method is used to change the contents of an array by removing existing elements and optionally adding new elements. This method accepts three parameters. The first parameter determines the index at which you want to insert the new element or elements. The second parameter determines the number of elements that you ...
18/6/2021 · JavaScript arrays have 3 methods for adding an element to an array: push () adds to the end of the array. unshift () adds to the beginning of the array. splice () adds to the middle of the array. Below are examples of using push (), unshift (), and splice (). There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end. ARRAYA.concat (ARRAYB) will join two arrays together. Method 1: push () method of Array. The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. The object is hence added to the end of the array.
For that purpose, I decided to write this article about some of the best methods we can use to add and remove elements in arrays in Javascript. In this article we'll be covering push , pop , shift , unshift , concat , splice , slice and also on how to use the ES6 spread operator for javascript arrays. push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... Output. In the above program, the splice () method is used to add a new element to an array. The first argument is the index of an array where you want to add an element. The second argument is the number of elements that you want to remove from the index element. The third argument is the element that you want to add to the array.
Say you want to add an item to an array, but you don't want to append an item at the end of the array. You want to explicitly add it at a particular place of the array. That place is called the index. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. Adding an element at a given position of the array. Sometimes you need to add an element to a given position in an array. JavaScript doesn't support it out of the box. So we need to create a function to be able to do that. We can add it to the Array prototype so that we can use it directly on the object. Arrays are considered similar to objects. The main similarity is that both can end with a comma. One of the most crucial advantages of an array is that it can contain multiple elements on the contrary with other variables. An element inside an array can be of a different type, such as string, boolean, object, and even other arrays.
The first and probably the most common JavaScript array method you will encounter is push(). The push() method is used for adding an element to the end of an array. Let's say you have an array of elements, each element being a string representing a task you need to accomplish. The push method appends values to an array.. push is intentionally generic. This method can be used with call() or apply() on objects resembling arrays. The push method relies on a length property to determine where to start inserting the given values. If the length property cannot be converted into a number, the index used is 0. This includes the possibility of length being nonexistent, in ... can some one help about: add element to end of array javascript Problem: can some one help about: add element to end of array javascript asked Mar 3 amir.zaib45 1.2k points
When you work with arrays, it is easy to remove elements and add new elements. This is what popping and pushing is: Popping items out of an array, or pushing items into an array. ... Since JavaScript arrays are objects, elements can be deleted by using the JavaScript operator delete: Example. To add an item to a specific index position in an array, we can use the powerful JavaScript array.splice () method. The splice () method can add or remove items from an array. The splice () syntax looks like this: The splice () method takes 3 or more arguments: Argument 1: the startIndex which is where (the position) you want to add something. To add guitar to the end of the instruments array, type the array's name 1:51. followed by a period, the word push, and a set of parentheses. 1:56. Inside the parentheses, 2:01. you include the element or value you wish to add to the end of the array. 2:02. You can think of the push method as pushing an item 2:08.
To add a new element to the HTML DOM, we have to create it first and then we need to append it to the existing element.. Steps to follow. 1) First, create a div section and add some text to it using <p> tags.. 2) Create an element <p> using document.createElement("p").. 3) Create a text, using document.createTextNode(), so as to insert it in the above-created element("p"). DOM Element. accessKey ... Previous JavaScript Array Reference Next ... Definition and Usage. The push() method adds new items to the end of an array. push() changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift(). Browser Support. Using Array.unshift () The easiest way to add elements at the beginning of an array is to use unshift () method. It adds the elements of your original array. Sometime you wish not to alter the original array, and assign it to a new variable instead. In Javascript, you can do this in multiple ways in a single statement.
JavaScript: Add an element to the end of an array. This is a short tutorial on how to add an element to the end of a JavaScript array. In the example below, we will create a JavaScript array and then append new elements onto the end of it by using the Array.prototype.push() method. See the Pen JavaScript: Add items in a blank array and display the items - array-ex-13 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: Write a JavaScript program to compute the sum and product of an array of integers. Let see the code in action. // create javascript array let array = [1, 2] // add element to array array.unshift(0) console.log(array) // result => [0, 1, 2] So we add 0 at the starting index without replacing another value because all value in the array gets shifted. you can also add multiple values in a single statement by passing a value in ...
In the same directory where the JavaScript file is present create the file named code.json. After creating the file to make the work easier add the following code to it: { "notes": [] } In the above code, we create a key called notes which is an array. The Main JavaScript Code. In the upcoming code, we will keep adding elements to the array. javascript has two inbuilt array methods name unshift() and push(), which is used to add elements or items at first or beginning and last or ending of an array in javascript. This tutorial has the purpose to explain how to add an element at the beginning and ending of an array in javascript using these unshift and push js methods. 5 Way to Append Item to Array in JavaScript Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍
Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. It is used to add the objects or elements in the array. Unlike the push () method, it adds the elements at the beginning of the array. " Note that you can add any number of elements in an array using the unshift () method."
How To Add New Elements To A Javascript Array
Javascript Add New Elements At The Beginning Of An Array
How To Remove Array Duplicates In Es6 By Samantha Ming
How To Add Remove And Replace Items Using Array Splice In
Data Structures Objects And Arrays Eloquent Javascript
Javascript Interview Question 49 Add A New Array Element By
Javascript Array A Complete Guide For Beginners Dataflair
Tools Qa Array In Javascript And Common Operations On
How To Insert Element Into Array At Specific Position Javascript
Javascript Array Push Key Value Code Example
How To Append Value To An Array In Javascript C Java Php
Javascript Append To Array A Js Guide To The Push Method
Understanding Array Splice In Javascript Mastering Js
Javascript Array A Complete Guide For Beginners Dataflair
How To Insert An Item Into Array At Specific Index In
Javascript Array Push How To Add Element In Array
Js Add Element To Array Middle Code Example
Javascript Array Distinct Ever Wanted To Get Distinct
C Program To Insert An Element In An Array
Working With Arrays In Javascript Learnbatta
Unshift Method To Add Element At The Beginning Of An Array
Use Push To Insert Elements Into An Array
Insert An Element In Specific Index In Javascript Array By
Create Ul And Li Elements Dynamically Using Javascript
0 Response to "25 Javascript Add Element To Array"
Post a Comment