22 Javascript Error Handling Best Practices
The above example demonstrates that accessing: an uninitialized variable number; a non-existing object property movie.year; or a non-existing array element movies[3]; are evaluated to undefined.. The ECMAScript specification defines the type of undefined value:. Undefined type is a type whose sole value is the undefined value.. In this sense, typeof operator returns 'undefined' string for an ... We can handle this in two ways: We call thisThrows () in an async function and await the thisThrows () function. We chain the thisThrows () function call with a .catch () call. The first solution would look like this: And the second one: Both solutions work fine, but the async/await one is easier to reason about (at least in my personal opinion).
Best Practices For Node Js Error Handling Toptal
The first step in handling errors is to provide a client with a proper status code. Additionally, we may need to provide more information in the response body. 3.1. Basic Responses. The simplest way we handle errors is to respond with an appropriate status code. Here are some common response codes:
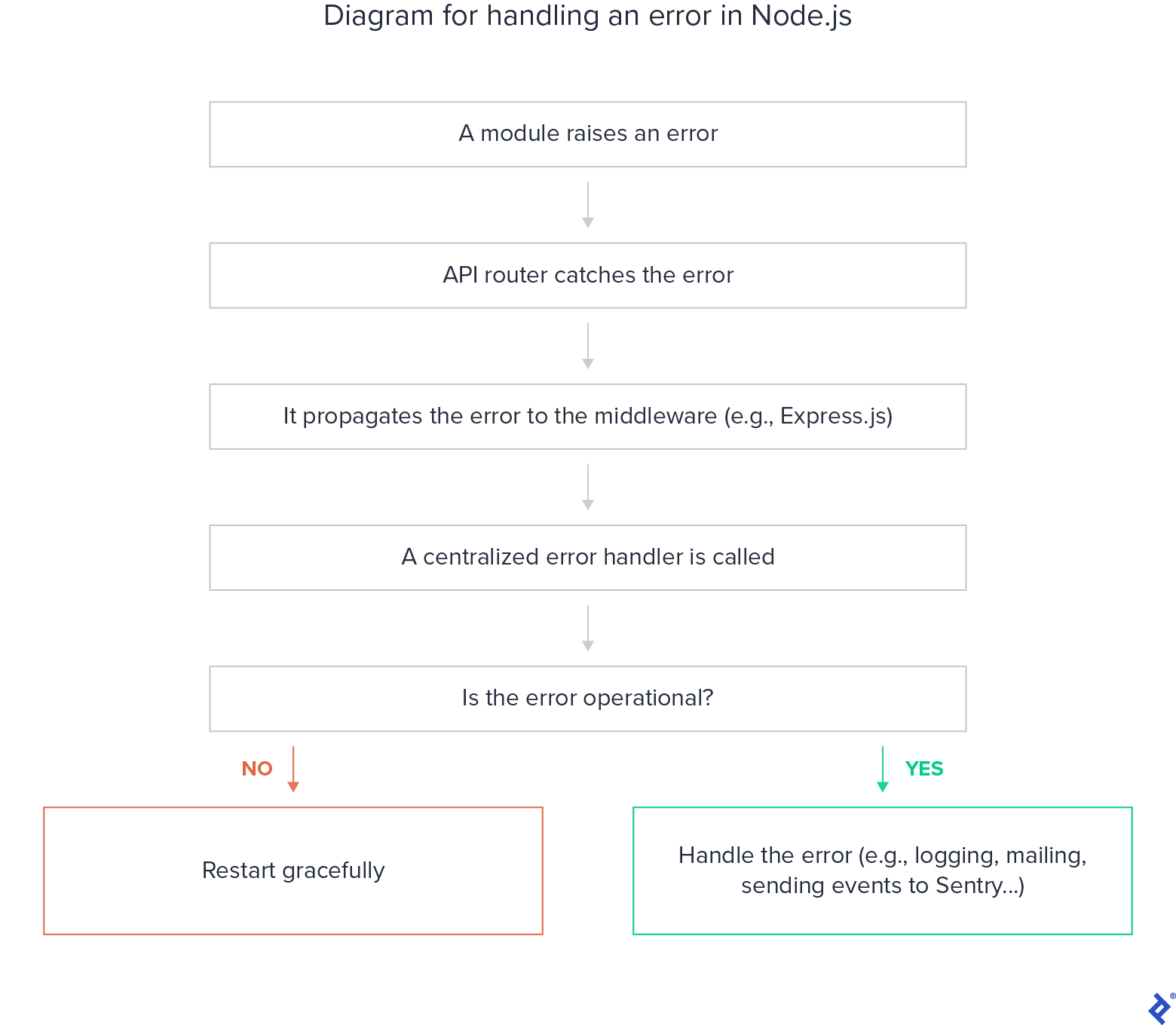
Javascript error handling best practices. When creating large web applications in JavaScript it is critical to implement a robust error handling process, whether coding on the server or in the browser. Apr 12, 2019 - Every modern programming language has the ability to handle and throw exceptions, and JavaScript is no exception. In this column, I discuss why, how, and when you should make use of JavaScript’s try catch statement as well as throwing your own custom errors. This playlist/video has been uploaded for Marketing purposes and contains only selective videos. For the entire video course and code, visit [http://bit.ly/2...
I believe that much of the reason that try..catch is rare in JavaScript is because the language has a pretty high tolerance for error. The vast majority of situations can be handled by using code checks, good defaults, and asynchronous events. In some cases, simply using a pattern will prevent issues: Using Node.js built-in Error object is a good practice because it includes intuitive and clear information about errors like StackTrace, which most developers depend on to keep track of the root of an error. And additional meaningful properties like HTTP status code and a description by extending the Error class will make it more informative. Jan 29, 2019 - Medium is an open platform where readers find dynamic thinking, and where expert and undiscovered voices can share their writing on any topic.
25/6/2011 · An immensely interesting set of slides on Enterprise JavaScript Error Handling can be found at https://web.archive /web/20140126104824/http://www.devhands /2008/10/javascript-error-handling-and-general-best-practices/ In short it summarizes: Assume your code will fail; Log errors to the server; You, not the browser, handle errors Jan 31, 2018 - Instead, we need a more novel method to handle the exception, report the error gracefully and then continue with the program execution from that point where the error was caught. In this article, I am going to discuss different errors you might encounter in JavaScript, the best practices to ... To learn more, read Custom Database Action Script Execution Best Practices. If recovery from an error condition is not possible (or probable) within this time period, then an error condition should be explicitly returned; this is as simple as completing rule execution by returning an instance of a Node Error object, as in:
Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things. Example In this example we misspelled "alert" as "adddlert" to deliberately produce an error: Example: As in runtime error, there are exceptions and this exception is correct by the help of the try and catch method. try ___ catch method: JavaScript uses the try catch and finally to handle the exception and it also used the throw operator to handle the exception. try have the main code to run and in the catch, give the exception ... Best practice: When logging errors to the console inside a catch block, using console.error () rather than console.log () is advised for debugging. It formats the message as an error, and adds it to the list of error messages generated by the page.
JavaScript - Errors & Exceptions Handling, There are three types of errors in programming: (a) Syntax Errors, (b) Runtime Errors, and (c) Logical Errors. Building robust Node.js applications requires dealing with errors properly. The onerror () method was the first event handler to facilitate and handle errors in JavaScript. It is often used with the syntax window.onerror. This enables the error event to be fired on the window object whenever an error occurs during runtime. Below is an example showing how to use the onerror () method:
Photo by vnwayne fan on Unsplash. JavaScript is a very forgiving language. It's easy to write code that runs but has issues in it. In this article, we'll look at how to handle errors in ... 27/11/2017 · An immensely interesting set of slides on Enterprise JavaScript Error Handling can be found at http://www.devhands /2008/10/javascript-error-handling-and-general-best-practices/ In short it summarizes: Assume your code will fail; Log errors to the server; You, not the browser, handle errors; Identify where errors might occur; Throw your own errors Logging is an essential part of development. While working on React projects, logging provides a way to get feedback and information about what's happening within the running code.
8/6/2017 · In a multi-layered solution with deep call stacks, it is impossible to figure out where it went wrong. As far as error handling, this is pretty bad. A fail-silent strategy will leave you pining for... Spread the love Related Posts Cloning Arrays in JavaScriptThere are a few ways to clone an array in JavaScript, Object.assign Object.assign allows us… Using the Javascript Conditional OperatorThe JavaScript conditional operator is a basic building block of JavaScript programs. The conditional operator… JavaScript Best Practices — ClassesCleaning up our JavaScript code is easy with ... non-critical errors that can occur in user-defined functions. That must depend on the purpose of the page being displayed. For an "advertising" page, the code should probably do its best to
May 01, 2021 - This is post # 20 of the series, dedicated to exploring JavaScript and its building components. In the process of identifying and describing the core elements, we also share some rules of thumb we… I recently wrote a detailed tutorial about Node.js logging best practices you should check out. The gist of it is to use structured logging to print errors in a formatted way and send them for safekeeping to a central location, like Sematext Logs, our log management tool. Launching Visual Studio Code. Your codespace will open once ready. There was a problem preparing your codespace, please try again.
¶ Sometimes, when something strange occurs, it would be practical to just stop doing what we are doing and immediately jump back to a place that knows how to handle the problem. I've got some good news and some bad news. If you're handling errors as best you can in your application, awesome! That's the good news. The bad news is that you're only halfway there. What do I mean? Well, handling errors in your program deals only with the things you know about. Oct 10, 2019 - There is no such thing as perfect code. Even if there was, our users will always find surprising ways to break what once seemed like perfectly watertight code and attempt to do things that we don’t…
Aug 18, 2020 - Learn how to deal with errors and exceptions in synchronous and asynchronous JavaScript code. As explained in best practice #4, the exception message should describe the exceptional event. And the stack trace tells you in which class, method, and line the exception was thrown. If you need to add additional information, you should catch the exception and wrap it in a custom one. But make sure to follow best practice number 9. Handling errors well can be tricky. How Error() historically worked in JavaScript hasn't made this easier, but using the Error class introduced in ES6 can be helpful
Apr 26, 2019 - Handling errors well can be tricky. How Error() historically worked in JavaScript hasn’t made this easier, but using the Error class introduced in ES6 can be helpful Error handling in Javascript isn't complex, but when we are writing React components we may have some difficulties dealing with uncaught… Anything else I ought to know, regarding trapping errors in my application? I'm also completely game for hearing of books that have great chapters or in-depth explanations of error-handling. Eloquent JavaScript touches on the matter, but isn't very prescriptive or opinionated about the issue. Thanks for any advice you can give!
The common practice is to use $.ajaxSetup to specify a generic callback handler for errors during the non- $.ajax functions. TL;DR: When an unknown error occurs (a developer error, see best practice number #3)- there is uncertainty about the application healthiness. A common practice suggests restarting the process carefully using a 'restarter' tool like Forever and PM2 Jan 07, 2019 - Coders often default to log errors. This is a bad habit. Learn how to build a beautiful structure to bulletproof your Error handling.
Error Handling In Node Javascript Sucks Solution The Best
Node Js Architecture And 12 Best Practices For Node Js
Error Handling With Angular 8 Tips And Best Practices Rollbar
Proper Error Handling In Javascript Scotch Io
Node Js Error Handling Best Practices Ship With Confidence
Best Practices To Control Your Errors Jsmanifest
Node Js Error Handling Best Practices Ship With Confidence
Node Js Best Practices Error Handling And Logging By John
Javascript Error And Exceptional Handling With Examples
C Exception Handling Best Practices Elmah Io
Proper Error Handling In Javascript Scotch Io
Best Practices In Error Handling Ta Digital Labs
Node Js Best Practices Error Handling By John Au Yeung
How To Implement Error Handling In Sql Server
Javascript Best Practices Error Handling Techniques By
A Mostly Complete Guide To Error Handling In Javascript
Error Messages Examples Best Practices Amp Common Mistakes Cxl
Node Js Error Handling Err Arg Not Iterable
Exception Filters Nestjs A Progressive Node Js Framework
Node Js Error Handling Explained
Error Handling With Angular 8 Tips And Best Practices Rollbar
0 Response to "22 Javascript Error Handling Best Practices"
Post a Comment