31 Push Item To Beginning Of Array Javascript
Mar 02, 2017 - The spread operator (...) is to spread out all items from a collection. ... 1) The push() method adds one or more elements to the end of an array and returns the new length of the array. ... 2) The unshift() method adds one or more elements to the beginning of an array and returns the new length ... In computer science, this means an ordered collection of elements which supports two operations: push appends an element to the end. shift get an element from the beginning, advancing the queue, so that the 2nd element becomes the 1st. Arrays support both operations. In practice we need it very often.
Using Array.unshift () The easiest way to add elements at the beginning of an array is to use unshift () method. It adds the elements of your original array. Sometime you wish not to alter the original array, and assign it to a new variable instead. In Javascript, you can do this in multiple ways in a single statement.
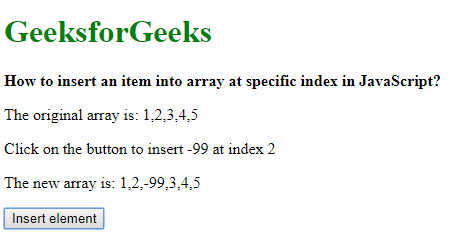
Push item to beginning of array javascript. JavaScript | Add new elements at the beginning of an array Last Updated : 01 Apr, 2019 Adding new elements at the beginning of existing array can be done by using Array unshift () method. This method is similar to push () method but it adds element at the beginning of the array. Each element of the first array is compared with the second array using the indexOf() method. The arr2.indexOf(x) method searches arr2 and returns the position of the first occurrence of arr1. If the value cannot be found, it returns -1. All the elements that are in both arrays are returned by the filter() method. Last Updated : 06 Mar, 2019. There are several methods for adding new elements to a JavaScript array. push (): The push () method will add an element to the end of an array, while its twin function, the pop () method, will remove an element from the end of the array. If you need to add an element or multiple elements to the end of an array, the ...
I notice that you wish to keep the array the same length as the original, even if the supplied item is prepended to the array. You also speak like scaling is going to be an issue, so I will add some tests that will use a reasonably large data set. Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. There are various ways to add or append an item to an array. We will make use of push, unshift, splice, concat, spread and index to add items to an array. Let's discuss all the 6 different methods one by one in brief. The push() method. This method is used to add elements to the end of an array. This method returns the new array length.
In this tutorial, we will demonstrate how to add single, multiple items (elements) into an array, and how to add one array to another array using the array push () and Concat () method of javaScript with examples. javaScript push () Method The javaScript push () method is used to add new elements to the end of an array. start → The index at which to start changing the array. deleteCount(optional) → An integer indicating the number of elements in the array to remove from start. (elem1, elem2 …) → The elements to add to the array, beginning from start. If you do not specify any elements, splice() will only remove elements from the array. Aug 11, 2017 - Assuming I have an array that has a size of N (where N > 0), is there a more efficient way of prepending to the array that would not require O(N + 1) steps? In code, essentially, what I current...
Nov 26, 2011 - FYI, just like there's .push() ... for the beginning of the array. ... Елин Й. ... Well as a programmer it is always very important to have options, sometimes the easy solutions dont always work in certain situations, and splice is a good way to remove items from arrays, ... Dec 29, 2009 - Most other online resources will ... because javascript is case sensitive. Beginners may not know this. I recommend changing "Push()" to "push()" in your titles. ... Thank you, it's really helpful. ... Thanks for such helpful post. But i would also like to see the performance of the shift and unshift method if i have very large array... Aug 24, 2014 - Learn how to insert an item into an array at a specified index with JavaScript!
In this tutorial, we will learn about how to add or remove items from the beginning or end of the arrays by using four different methods in JavaScript. Push() method. The push() method is used to add the new elements to the end of an array. Example: The unshift method inserts the given values to the beginning of an array-like object. unshift is intentionally generic. This method can be called or applied to objects resembling arrays. How to use JavaScript push, concat, unshift, and splice methods to add elements to the end, beginning, and middle of an array.
The push () method in javascript returns the number of the elements in the current array after adding the specified elements in its parameters. This is always equal to the length of the array before adding the elements plus the number of the elements which are pushed. Let's verify this with the help of an example. In this tutorial, you'll learn how to insert element to front/beginning of an array in JavaScript. Code Handbook Newsletter Contact How To Insert Element To Front/Beginning Of An Array In JavaScript ... For adding an element to an array, you use push which inserts the new element to the end of the array. JavaScript arrays have a push () function that lets you add elements to the end of the array, and an unshift () function that lets you add elements to the beginning of the array. The splice () function is the only native array function that lets you add elements to the middle of an array.
6 days ago - In this example, we will see pop, push, shift, and unshift array methods one by one in Javascript Node.js with an example. In addition, JavaScript gives us four methods to add or remove items from the beginning or end of arrays. Pop Push Shift and Unshift Array Methods in JavaScript You can use the javascript push () method to add the elements or items from the last or end of an array. push () method javascript Definition: - The javaScript push () method is used to add a new element to the last or end of an array. Syntax of push () method is TL;DR. When you want to add an element to the end of your array, use push (). If you need to add an element to the beginning of your array, try unshift (). And you can add arrays together using concat (). There are certainly many other options for adding elements to an array, and I invite you to go out and find some more great array methods!
Definition and Usage The unshift () method adds new items to the beginning of an array, and returns the new length. unshift () overwrites the original array. Tip: To add new items at the end of an array, use push (). Definition and Usage The push () method adds new items to the end of an array. push () changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift (). Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Mar 16, 2016 - Does Javascript have any built-in functionality that does this? The complexity of my method is O(n) and it would be really interesting to see better implementations. ... FYI: If you need to continuously insert an element at the beginning of an array, it is faster to use push statements followed ... Using the Last Index of the Array. To add an element to the end of an array, we can use the fact that the length of an array is always one less than the index. Say, the length of an array is 5, then the last index at which the value will be 4. So, we can directly add the element at the last+1 index. Let us take a look: 5 Way to Append Item to Array in JavaScript. Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍.
unshift () Return Value. Returns the new (after adding arguments to the beginning of array) length of the array upon which the method was called. Notes: This method changes the original array and its length. To add elements to the end of an array, use the JavaScript Array push () method. Pop, Push, Shift and Unshift Array Methods in JavaScript JavaScript gives us four methods to add or remove items from the beginning or end of arrays: pop() : Remove an item from the end of an array You can use the unshift () method to easily add new elements or values at the beginning of an array in JavaScript. This method is a counterpart of the push () method, which adds the elements at the end of an array. However, both method returns the new length of the array.
Inserting an item into an existing array is a daily common task. You can add elements to the end of an array using push, to the beginning using unshift, or to the middle using splice. Those are known methods, but it doesn't mean there isn't a more performant way. The unshift () method adds one or more items to the beginning of an array and returns the new length of the modified array. Here's what it looks like in a code example: Say you want to add an item to an array, but you don't want to append an item at the end of the array. You want to explicitly add it at a particular place of the array. That place is called the index. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on.
The push method appends values to an array. push is intentionally generic. This method can be used with call () or apply () on objects resembling arrays. The push method relies on a length property to determine where to start inserting the given values. 1 week ago - The javascript array push() method is used to add items at the end of an array. The javascript array unshift() method is used to add items at the beginning of an array.
Javascript Typed Arrays Javascript Mdn
How To Declare An Empty Array In Javascript Quora
Push An Item To The Beginning Of An Array In Javascript
Javascript Pushing An Object In An Array Is What By
How To Add An Item At The Beginning Of An Array In Javascript
How To Add Object In Array Using Javascript Javatpoint
Array Prototype Splice Javascript Mdn
Javascript Add Item To Array First
Javascript Array Methods Declaration Properties Cronj
The Beginner S Guide To Javascript Array With Examples
Insert An Element In Specific Index In Javascript Array By
How To Manage React State With Arrays
Dynamic Array In Javascript Using An Array Literal And
Pop Push Shift And Unshift Array Methods In Javascript
Debugging In Visual Studio Code
Array Unshift Codesnippet Io Javascript Tips Amp Tricks
Momentjs Add Moment Object Into Array Stack Overflow
How To Insert An Item Into Array At Specific Index In
How To Add An Object To An Array In Javascript Geeksforgeeks
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Push How To Add Element In Array
Useful Javascript Array And Object Methods By Robert Cooper
Building A Stack Vs Building A Queue In Javascript By Kenny
How To Add Remove And Replace Items Using Array Splice In
Angularjs Push Item To First Or 0 Index Of Scope Array
Essential Vanilla Javascript Methods Cheat Sheet By Bytecut
Javascript Array Push Vs Unshift Methods Explained With 4
What Is The Best Way To Publish A List Of Objects To
How To Create Array In Javascript Code Example
All About Javascript Arrays In 1 Article By Rajesh Pillai
0 Response to "31 Push Item To Beginning Of Array Javascript"
Post a Comment