29 How To Override Css Class In Javascript
Given an HTML document and the task is to override the function, either predefined function or user-defined function using JavaScript. Approach: When we run the script then Fun () function called. After clicking the button the GFG_Fun () function called and this function contains another function which will run. Often times it makes sense to define sensible default styles for mixins. This way, the user can just make a call to the mixin and pass in any explicit styles that they want to override. In this lesson, you will learn how to convert arguments with default values as well as how to use default values with objects destructuring.
Js Classname Replace Code Example
Adding a CSS class to an element using JavaScript. Now, let's add the CSS class "newClass" to the DIV element "intro". For the purpose of this example, I have added a delay using the setTimeout() method so that you can see the style changing: //Delay the JS execution by 5 seconds //by using setTimeout setTimeout(function(){ //Add the ...
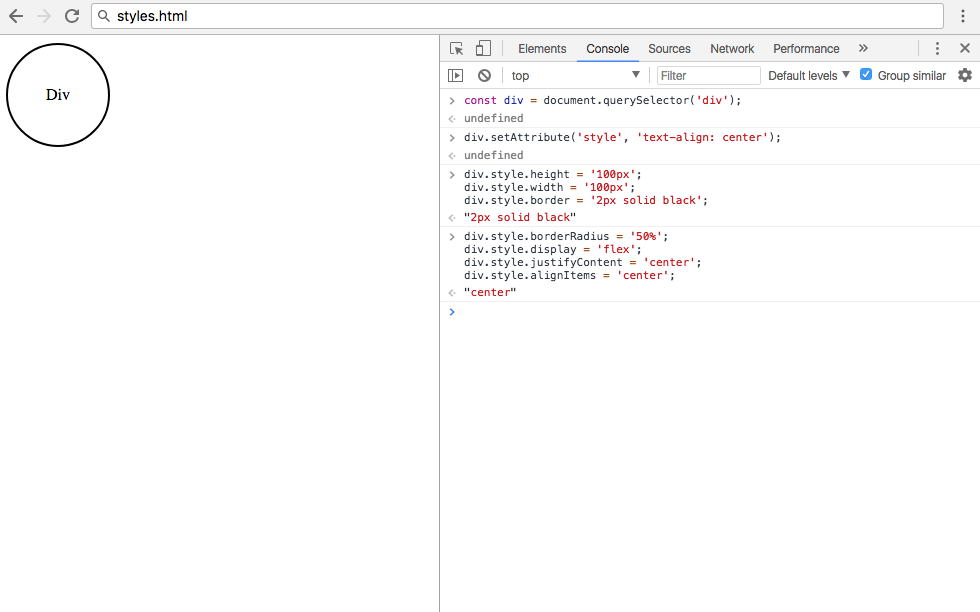
How to override css class in javascript. The constructor () method is a special method for creating and initializing objects created within a class. The constructor () method is called automatically when a class is initiated, and it has to have the exact name "constructor", in fact, if you do not have a constructor method, JavaScript will add an invisible and empty constructor method. Let’s now see how we can make one class override another. If that class has a background-color of blue, and you want your <div> to have a red background instead, try to change the color from blue to red in the class itself. You could also create a new CSS class that defined a background-color property with a value of red and let your <div> reference that class. Example of making one style override another:¶ It usually starts with finding a CSS selector affecting a given set of properties. Next, you need to define your CSS selector which is simply the one that you have found prepended with a sort of...
Javascript to Override CSS. JScript and Scripting > JScript Native. ... In your code you're trying to access all elements by class name. If you want to change the class names of all child elements then get the parent elements id and iterate through all child elements and change class name. If you want the overrideclass to override the yesand noclasses define your overrideclass after the other ones in your CSS file and redefine the same rules with different values. The first thing to do is inspect the element and make sure that your new CSS is actually being applied to the nav. If you can see your new CSS in the Styles pane, but your new CSS is crossed out, it means that there's some other CSS that is overriding your new CSS. In CSS terminology this concept is called Specificity.
In the above example, we have added style attribute and container class to the div element but we will still see green color. To override the inline styles we need to use !important rule in our external css file. Example: < Now, we'll present the ways of overriding the !important rule. Add another CSS rule having !important. Then give a selector with a higher specificity (adding a tag, id, or class to the selector) or add a CSS rule having the same selector at a later point than the existing one. In a specificity tie, the last defined rule wins. And then, simply add the class to the element using JavaScript: const btn = document. querySelector ('.btn'); // add CSS class btn. classList. add ('btn'); Take a look at this guide to learn more about setting CSS styles using vanilla JavaScript. The purpose of these tutorials is not to discourage the use of CSS classes.
This post will discuss how to add a CSS class to an HTML element using JavaScript and jQuery. 1. Using JavaScript className property. The className property is commonly used to set the value of the class attribute of an element in plain JavaScript. The following code demonstrates this by setting the class attribute for the div element and ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. You can set the inline style of your container's scroll to auto, then reverting the change by altering the value of html.style.scrollBehavior before and after scrolling programmatically. JS's ScrollToOptions's behavior key-value pair cannot override CSS's scroll-behavior.The CSSOM draft mentions this:. If the user agent honors the scroll-behavior property and one of the following are true:
The classList property allows greater performance and functionality to alter your HTML elements and their CSS classes within JavaScript. For additional reading, check out the article How To Modify Attributes, Classes, and Styles in the DOM and the ebook Understanding the DOM — Document Object Model . To completely override the existing inline style, you set the cssText property of the style object. New in dash v0.22.0! Learn how to add custom CSS and JS to your application with the `assets` directory. Also, learn how to customize the HTML template that Dash serves on page load in order to add custom meta tags, customize the page's title, and more.
Yes and no, it depends on what you'd like to change or add. In this answer I will provide a working example, I'll show a methode to add some CSS to the Iframe that will make changes to the color.. If you don't control the content in the Iframe: Yo... How to override the !important CSS style attribute using Javascript Posted on May 2, 2011 September 9, 2018 by ellen I've worked on a few projects where a CSS style on some page element has been specified as "!important" and it interferes with the way the page functions. 9/2/2017 · document.getElementsByClassName("nav-questions").style.maxHeight="40%";document.getElementsByClassName("nav-questions").style.maxWidth="40%"; This line of code automatically override the global css as priority of JS applied css are always higher(even then inline css). Share.
Sometimes we want the variables to change only in a specific section of the page. Assume we want a different color of blue for button elements. Then, we can re-declare the --blue variable inside the button selector. When we use var (--blue) inside this selector, it will use the local --blue variable value declared here. Using the style.setProperty() method we can override/update global or local scoped CSS variables. It has the following syntax: style.setProperty(propertyName, value, priority); Where propertyName in our case would be the CSS variable we wish to update, and the value would be whatever we want the new value to be. Optionally, you can specify the third argument as well to set the priority (e.g ... The following table defines the first browser version with full support for Classes in JavaScript: Chrome 49. Edge 12. Firefox 45. Safari 9. Opera 36. Mar, 2016.
CSS Specificity Another important point to keep in mind when overriding the bootstrap styles is CSS specificity. We will need to use selectors that are as specific as the ones in the bootstrap.css. Using selectors that are not specific will not give you desired results, as the component will end up taking the default bootstrap styles even though we have written the custom styles in custom.css ... CSS classes aren't aliased to variables like element ids are, so you can't just reference "state" like you're doing. Rather than trying to change the definition of a class (not sure you can do that), I'd simply apply a different class to the element (foo.className = "myNewClass";, where "foo" is some element with an id of "foo" that initially had ... Another alternative is to remove existing CSS class and add new CSS class with expected style using JavaScript. Here is an example, that remove existing CSS class and add new using JavaScript:
To override the CSS properties of a class using another class, we can use the !important directive. In CSS, !important means "this is important", and the property:value pair that has this directive is always applied even if the other element has higher specificity. 25/1/2017 · There is no need to change the class itself. To override the behaviour, simply add another class to the existing one or change it entirely. I'll show the first option:.myclass { font-size: 14px; color: #aab5f0; } .myclass.override { font-size: 12px; } And then all you need to do from javascript is to toggle the override class. How to override !important A) Add another CSS rule with !important, and either give the selector a higher specificity (adding a tag, id or class to the selector), or add a CSS rule with the same selector at a later point than the existing one. This works because in a specificity tie, the last rule defined wins.
Welcome to a quick tutorial on how to change or override CSS with Javascript. Want to dynamically update or change some styles on a web page? The common ways to change or override CSS in Javascript are: document.getElementById ("ID").style.PROPERTY = "VALUE"; CSS class names can be removed or added using the classList method, or they can modified straight with the className property. Using the classList method. classList is pretty smart and the most modern system of manipulating CSS class names in elements via JavaScript. Remove class names. Here's how to remove a single class name:
White Labeling The Application Administration
Freecodecamp Basic Css Walk Through And Solutions This
How To Override Css In A Shadow Dom Web Component By Cesar
Override Css Style In Html Inline Or External Css Eyehunts
The 10 Most Common Bootstrap Mistakes That Developers Make
Use Custom Html Css And Javascript Code Sitejet Help
5 Ways To Change Override Css With Javascript Simple Examples
Supercharging Your Css Custom Properties
What Is Element Style And Why Is It Overriding My Css
How To Edit Customize And Override Bootstrap Css To Suit
Custom Css For Shortpoint Design Elements Shortpoint Support
Css Style Override In Html Fails Stack Overflow
Intellisense For Css Class Names In Html Visual Studio
Overriding Css Properties Of Third Party Components In
Embedding A Custom Css Style Class To A Sapui5 Control Sap
Supercharging Your Css Custom Properties
Internal Css Not Override External Css Api Google Why
Override Styles In Css All The Rules At A Glance
Css Modules React And Overriding Css Classes Stack Overflow
The 10 Most Common Bootstrap Mistakes That Developers Make
Overriding Child Element Styling Based On Parent With Css
10 Best Tools For Formatting And Optimizing Css Files
How To Modify Attributes Classes And Styles In The Dom
Css Modules React And Overriding Css Classes Stack Overflow
View And Change Css Chrome Developers
Is Possible Overwrite A Css Class With Another Css Class
0 Response to "29 How To Override Css Class In Javascript"
Post a Comment