29 Generate Random Number In Javascript Within A Range
Random Number Generated : 0.2894437916976895. The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive).This random number can then be scaled according to the desired range. Syntax: Math.random(); Parameters: This function does not accepts any parameter. In JavaScript, you can use the Math. random () function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive). If you want to get a random number between 0 and 20, just multiply the results of Math.random () by 20: To generate a random whole number, you can use the following Math methods along with Math.random ():
How To Generate 3 Random Whole Numbers Within A Given Range
Dec 28, 2020 - Math.random() is a function that returns a pseudo-random floating numbers between 0 and 1 (0 is inclusive, 1 is exclusive) We usually multiply the result with other numbers to scale the randomized value. However, there....
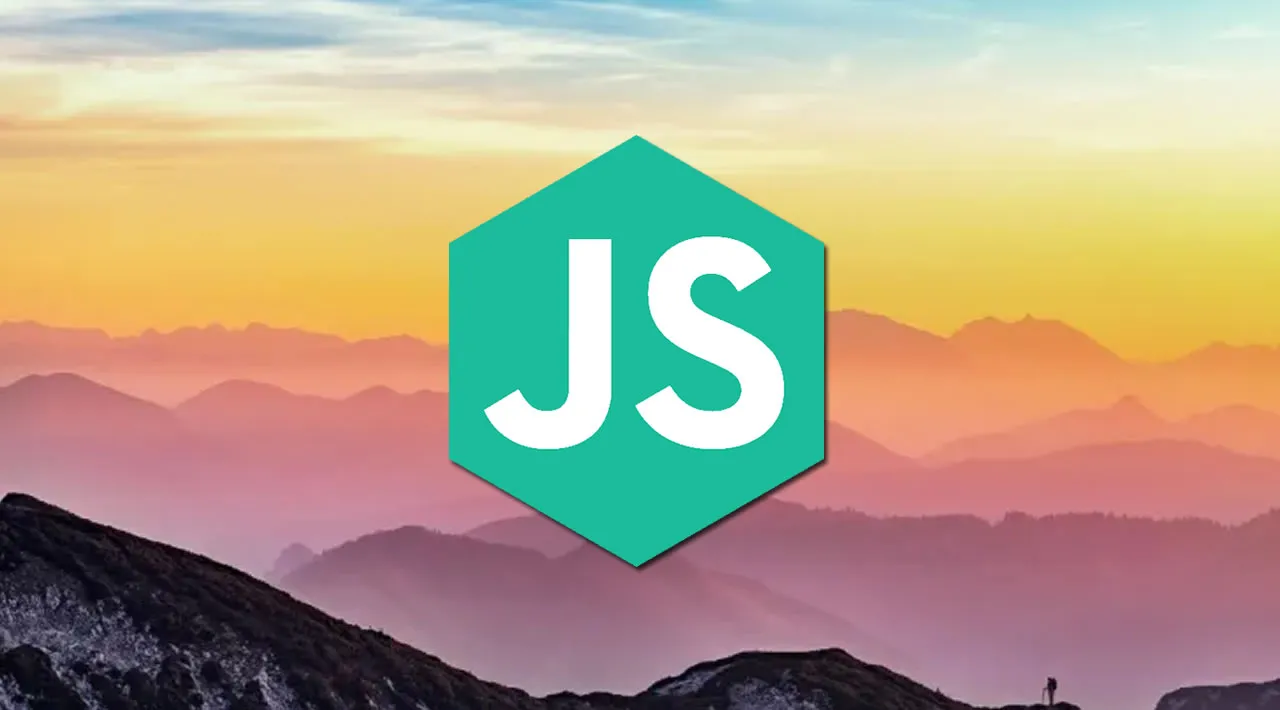
Generate random number in javascript within a range. Apr 16, 2021 - Javascript random numbers are essential for simulating random behavior in computing, but are never truly random and may not be cryptographically secure. To generate a random number in JavaScript, simply use the following code: ? 1. var randomnumber=Math.floor (Math.random ()*11) where 11 dictates that the random number will fall between 0-10. To increase the range to, say, 100, simply change 11 to 101 instead. Generating n random numbers between a range - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. ... How to create a random number between a range JavaScript; Generating desired pairs within a range using JavaScript; Sum of prime numbers between a range - JavaScript ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range. The implementation selects the initial seed to the random number generation algorithm; it cannot be chosen or reset by the user. Algorithm to Generate Random Number in a Specified Range in JavaScript. Generate a number between the range 0 and 1 and store it in a variable named rand. Calculate the total range range as max-min+1. Multiply range by rand and add it to min to get the random number inside the specified range.
Sometimes, we need to generate a random number between a range. Math.random() function generates floating numbers between 0 and 1. Method. Math.random() function: It returns a pseudo-random number between range 0 and 1. It can be scaled the random number between the desired range. Here, is a simple program to do so to generate the random number ... Photo by Kristopher Roller on Unsplash "The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range." — MDN Docs J avaScript has a useful built-in Math.random() function that generates pseudorandom floating ... function myRandomIntByMax(n){return Math.floor(Math.random() * n) + 1} myRandomIntByMax(15) // 14. Alright. We've got a general-purpose random number generator, and we know how to adjust the range, now comes the hard part. What I want is to be able to ask for some quantity of unique random numbers. So I want to be able to ask for, say, six ...
A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): 30/3/2021 · In this article, I’ll talk about how to use Math.random() to generate a random number between two numbers. “The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range.” Aug 28, 2018 - A short guide to .ceil, .floor, and .round. “Using Math.random() in JavaScript” is published by Joe Cardillo.
This post covers ways you can generate floats, integers and big integers (BigInts) within a certain range (min, max) using pure javascript (no dependencies). Floats Generate random float between 0 and 1 In this video, you will learn how to generate random numbers in javascript within range. How to create a random number between a range JavaScript Javascript Web Development Object Oriented Programming Our job is to create a function, say createRandom, that takes in two argument and returns a pseudorandom number between the range (max exclusive).
Question : Function to Generate Random Whole Numbers in JavaScript within a Range of 5 to 25. General Overview : (i) First convert it to the range - starting from 0. (ii) Then convert it to your desired range ( which then will be very easy to complete). So basically, if you want to generate Random Whole Numbers from 5 to 25 then : Oct 14, 2018 - Link to the challenge: https://learn.freecodecamp /javascript-algorithms-and-data-structures/basic-javascript/generate-random-whole-numbers-within-a-range JavaScript: get a random number from a specific range - random.js
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript Algorithms and Data Structures. Basic JavaScript. Generate Random Whole Numbers within a Range. Instead of generating a random whole number between zero and a given number like we did before, we can generate a random whole number that falls within a range of two specific numbers. Random Integer Between X and Y (Exclusive Y) Let's say you want to generate an integer in a range between X and Y. For example, a number larger than 1024, but no bigger than 49151. Create a new method called between which can be part of your app's utilities.
A formula for generating a random number within a given range using JavaScript is as follows: Math.floor(Math.random() * (UpperRange - LowerRange + 1)) + LowerRange; So to generate a random number between 25 and 75, the equation would be . Math.floor(Math.random() * (75-25+1)) + 25; i.e. Math.floor(Math.random() * 51) + 25; Here’s the entire code: Apr 01, 2018 - Learn how to generate a range of random numbers that fall within an upper and lower range you specify. Jan 06, 2017 - My code from the bootcamp. Contribute to Rafase282/My-FreeCodeCamp-Code development by creating an account on GitHub.
Math.random () = 0.8244326990411024. (myMax - myMin + 1) = 10 - 1 + 1 -> 10. a * b = 8.244326990411024. c + myMin = 9.244326990411024. Math.floor (9.244326990411024) = 9. randomRange should use both myMax and myMin, and return a random number in your range. You cannot pass the test if you are only re-using the function ourRandomRange inside ... I understand you can generate a random number in JavaScript within a range using this function: function getRandomInt (min, max) { return Math.floor(Math.random() * (max - min + … Let us see some of the examples to generate random numbers: 1. Use of Math.random () function. We have the Math. random () function in JavaScript to deal with the random numbers. This number always return less than 1 as a result. This will always give the result in the form of a decimal point. 2.
A way to randomly generate two numbers in a range. A lot of times you will need to create a random number in JavaScript within a given range. In fact, let's say you need to randomly calculate a basketball result, you know that score will be between 70 and 130 (for example ;)). So how do you generate numbers from a range. This article describes how to generate a random number using JavaScript. Method 1: Using Math.random () function: The Math.random () function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range. Task: Generate a random number within the following range (30-75) in TestComplete using a scripting language of your choice. This is a solution created for [TechCorner Challenge #9] The following Vb Script code demonstrates how to generate random integers that fall within a specified range from 30 to 75. Here, I have used 'Rnd' function
@sokras the method calls new Random (check the JavaDoc): "Creates a new random number generator. This constructor sets the seed of the random number generator to a value very likely to be distinct from any other invocation of this constructor." Very likely might just involve using the current time as seed. 10/11/2020 · Generating Random Numbers in JavaScript. Math.random() in JavaScript generates a floating-point (decimal) random number between 0 and 1 (inclusive of 0, but not 1). Let's check this out by calling: console.log(Math.random()) This will output a floating-point number similar to: 0.9261766792243478 2. Generating Random Numbers in a Range. 2.1. Math.random. Math.random gives a random double value that is greater than or equal to 0.0 and less than 1.0. Let's use the Math.random method to generate a random number in a given range [min, max): public int getRandomNumber(int min, int max) { return ( int) ( (Math.random () * (max - min)) + min); }
JavaScript code to generate random numbers within a range - In this article, we will learn how we can generate a random number using Math.random() and some mathematical manipulation between a range? Submitted by Abhishek Pathak, on October 02, 2017 JavaScript is a front-end scripting language. In this challenge we learn a formula in javascript that allows us to generate a random number within a range. To generate a random number within a range in ... Generating a random number. To generate a random number, Math.random () is used. We can even generate a number between specified numbers such as between 1 and 10, between 0 and 100, etc. Let's discuss it using an example.
May 17, 2020 - In JavaScript, we can generate random numbers using the Math.random() function. Unfortunately, this function only generates floating-point numbers between 0 and 1. In my experience, it's much more common to need a random integer within a certain range. For example, a random number between 10 and 20.
Create A Javascript Function Which Generates A Random
Generating Random Whole Numbers In Javascript In A Specific
Javascript Random Number Career Karma
Javascript Math Random Method Explained
Generate Useful Random Numbers In Javascript Javascript
How To Generate Random Human Readable Slugs In Javascript
Generate A Random Number In A Range In Javascript
Basic Javascript 105 111 Generate Random Whole Numbers Within A Range Freecodecamp
Random Number Generator And Checker
Generate Random Whole Numbers Within A Range Freecodecamp Basic Javascript
Python Math Generate A Series Of Unique Random Numbers
Generate Random Integer Between Two Numbers Using Javascript
How To Obtain Random Numbers Within A Range Using Javascript
Javascript Get A Random Number Within A Range Delightly Linux
Working With Random In Python Generate A Number Float In
React Native Generate Random Number Between 1 To 100 In
Working With Random In Python Generate A Number Float In
Python Random Randrange Javatpoint
How To Generate Float Range Of Numbers In Python
Random Number In Javascript Practical Example
Generate Random Whole Numbers Within A Range Dev Community
Math Random In Javascript Generating Random Numbers Copahost
Random Number Generator In Python Examples Of Random Number
Randomizing Program Execution With Random Number Generators
Randomizing Program Execution With Random Number Generators
Creating Javascript Random Numbers With Math Random Udacity
How To Generate Random Numbers Within A Specific Range
Java Random Generation Javabitsnotebook Com
0 Response to "29 Generate Random Number In Javascript Within A Range"
Post a Comment