26 Javascript Array Not Includes
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ... In JavaScript, you'll often need to iterate through an array collection and execute a callback method for each iteration. And there's a helpful method JS devs typically use to do this: the forEach() method.. The forEach() method calls a specified callback function once for every element it iterates over inside an array. Just like other array iterators such as map and filter, the callback ...
How To Get Last Element Of An Array In Javascript
Working with arrays in JavaScript is a common activity. Sometimes we get a variable in JavaScript that we need to be an array, but we aren't sure that it is. Non-primitive data types in JavaScripts are all objects (functions have their own type, but they too are objects).
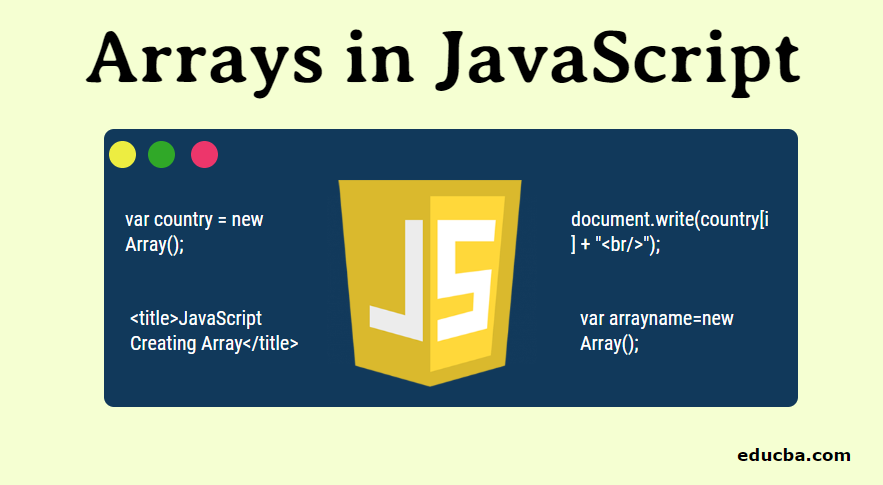
Javascript array not includes. To know whether an array contains a particular element or not, we use includes a method in javascript. Array.prototype.includes() is the method provided in javascript that helps us to determine whether any given array contains the specified value element considering all its entries and elements and this method returns a boolean value either true or false depending on the appropriate result and scenario. 5 Way to Append Item to Array in JavaScript. Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍. In javascript we can check whether a variable is array or not by using three methods. 1) isArray() method. The Array.isArray() method checks whether the passed variable is array or not. If the variable is an array it displays true else displays false.
As discussed above, JavaScript array is an object that represents a collection of similar type of elements. Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals. Second, it's declaration and initialization using JavaScript Array directly (new keyword). Use the lodash Library to Sum an Array in a JavaScript Array This tutorial teaches how to get the sum of an array of numbers in JavaScript. Use the for Loop to Sum an Array in a JavaScript Array. The for loop is used to iterate an array. We can use it to add all the numbers in an array and store it in a variable. JavaScript Array Methods. The Array object has many properties and methods which help developers to handle arrays easily and efficiently. You can get the value of a property by specifying arrayname.property and the output of a method by specifying arrayname.method().
Javascript Numeric Array Sorting. Sorting a Javascript array as numbers requires a comparison function to specify an alternative sort order. This function compares two parameters and a number denoting the relationship between the parameters; a zero, a positive value, or a negative value. The general form of a numeric comparison function is as ... JavaScript provides three methods pop, shift and splice that can remove entries from the array and which therefore reduce the length of the array. In each case the value (or values) removed are ... Chunking an array in JavaScript. We are required to write a function chunk () that takes in an array arr of string / number literals as the first argument and a number n as second argument. We are required to return an array of n subarrays, each of which contains at most arr.length / n elements.
For-each over an array in JavaScript. 1409. From an array of objects, extract value of a property as array. Hot Network Questions What is wrong with my two fair dice probability question reasoning? Deploying code without unit tests to give testers more time WCAM speaker invitation: scam, and how does it work? ... This method is another popular way to copy an array in Javascript. const originalArray = [1,2,3,4,5] const clone = [].concat(originalArray) Concat is a very useful method to merge two iterable. In this way, we take an empty array and concatenate the original array into it. It creates a fresh copy of the array. Second Method - javaScript sum array values using reduce () method. Next, we will use the reduce method of javascript for the sum of array values in javascript. You can see the example the below: 1. 2. 3. var numArr = [10, 20, 30, 40] // sums to value = 100. var sum = numArr.reduce (function(a, b) {return a+b;})
25/3/2020 · js array string includes. javascrpt include. check if name exists in array javascript. typescript check value in array list. typescript check value array list. array to contain. Typescript best way to check if an array exists. check value exist in array typescript. typescript if string in array. Make a human readable list from an array in JavaScript. ... Perhaps German speakers can clear up for us why the combo of narrow and unit for de behaves more like long and conjunction because I have no idea.. There's also a lesser-known localeMatcher option which can be configured to either 'lookup' or 'best fit' (defaults to 'best fit').As far as I can tell from mdn, its purpose is to tell the ... JavaScript arrays have the sort( ) method, which sorts the array elements into alphabetical order. The sort( ) method accepts a function that compares two items of the Array.sort([comparer]) . Watch a video course JavaScript - The Complete Guide (Beginner + Advanced)
JavaScript offers several ways to add, remove, and replace items in an array - but some of these ways mutate the array, and others are non-mutating; they produce a new array.. Below, I have outlined how to accomplish these three tasks using both mutating and non-mutating practices. This post concludes by showing how to iterate over an array and transform each item using the non-mutating ... JavaScript: Printing array elements. Learn how to print array elements to the console or the web page with JavaScript. Posted on March 15, 2021. When you need to print array elements, you can loop over the array and print each element to the console as follows: Definition and Usage. The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive.
JavaScript: Check if array is empty 11 min read You might find this useful when you want to execute a particular script if the array is empty - like enabling or disabling buttons based on if there is any input in the required field, etc. 14/3/2019 · Javascript array contains. To check if a JavaScript array contains a value or not, use the array includes () method. The array includes () method checks whether an array contains the item or specified an element or not. We can also determine if an array consists of an object in JavaScript. In JavaScript, array and object copy change the original values, so a deep copy is the solution for this. A deep copy means actually creating a new array and copying over the values, since whatever happens to it will never affect the origin one. JSON.parse and JSON.stringify is the best and simple way to deep copy.
3/12/2020 · In this article, we've gone over the few ways to check whether an array contains a value or not, in JavaScript. We've covered the includes () function, which returns a boolean value if the value is present. The indexOf () function returns the index of a value if it's present, and -1 if it isn't. There are multiple ways one can iterate over an array in Javascript. The most useful ones are mentioned below. Using for loop. Using while loop. This is again similar to other languages. using forEach method. The forEach method calls the provided function once for every array element in the order. The array will not be searched. let arr = [ 'a' , 'b' , 'c' ] arr . includes ( 'c' , 3 ) // false arr . includes ( 'c' , 100 ) // false Computed index is less than 0
2/2/2018 · What is the correct way of removing objects that have a certain field in JavaScript? I can include fields with the following: filteredResult = filteredResult.filter(e => e.selectedFields.includes("Red")); But if I wanted to remove all properties that have this field what would I do? There doesn't seem to be a "Remove" from the documentation. JavaScript associative array iteration. The example code seen till now uses a simple input JavaScript array that has a default numeric index. Now, we are going to see how to iterate an associative array with the JavaScript forEach loop. The below code uses JavaScript Map to create an associative array. JavaScript - Parse CSV data into an array. Learn how to use JavaScript to convert CSV data into an array. Posted on April 18, 2021. This tutorial will help you learn how to receive a CSV file using HTML <input> element and parse the content as a JavaScript array. For example, a CSV file with the following content:
The JavaScript filter array function is used to filter an array based on specified criteria. After filtering it returns an array with the values that pass the filter. The JavaScript filter function iterates over the existing values in an array and returns the values that pass. The search criteria in the JavaScript filter function are passed ... JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through. This article discusses how to create and work with objects ... Return value: This method returns the reference of the reversed original array. Below examples illustrate the JavaScript Array reverse () method: Example 1: In this example the reverse () method reverses the sequence of the array elements of arr. var arr = [34, 234, 567, 4]; print (arr); var new_arr = arr.reverse (); print (new_arr);
Replace the array with a new array. This is the fastest way to clear an array, but requires that you don't have references to the original array elsewhere in your code. For example, let's say your array looks like this: let a = [1,2,3];. To assign a to a new empty array, you'd use: a = []; The JavaScript array class is used in the construction of arrays, which are high-level and list-like objects. Arrays can be used for storing several values in a single variable. An array can be described as a unique variable that is capable of holding more than one value at the same time. Learn how to create a key value array in javascript. Arrays in javascript are not like arrays in other programming language. They are just objects with some extra features that make them feel like an array. It is advised that if we have to store the data in numeric sequence then use array else use objects where ever possible.
The returned promise is fulfilled with an array containing all the resolved values (including non-promise values) in the iterable passed as the argument.. If an empty iterable is passed, then the promise returned by this method is fulfilled synchronously. The resolved value is an empty array. If a nonempty iterable is passed, and all of the promises fulfill, or are not promises, then the ...
Javascript Indexof Method Explained With 5 Examples To
How To Check If A Javascript Array Contains A Specific Value
Javascript Foreach 10 Javascript Array Methods You Should
How Not To Sort An Array In Javascript Phil Nash
Javascript Array Distinct Ever Wanted To Get Distinct
How To Remove Commas From Array In Javascript
Data Structures Objects And Arrays Eloquent Javascript
3 Ways To Detect An Array In Javascript
Array Prototype Includes Javascript Mdn
How To Check If Array Includes A Value In Javascript
Geek97 On Twitter Javascript Tips Array 39 S Slice And Splice
Javascript Group Array By Key Code Example
Arrays In Javascript How To Create Arrays In Javascript
Hacks For Creating Javascript Arrays
Multikey Indexes Mongodb Manual
Understanding The Slice Method In Javascript The Basics The
To Mutate Or Not To Mutate The Javascript Array Methods
Indexed Collections Javascript Mdn
Algorithms 101 Find The Difference Between Two Arrays In
How To Implement Array Like Functionality In Sql Server
How To Remove Array Duplicates In Es6 By Samantha Ming
A List Of Javascript Array Methods By Mandeep Kaur Medium
How Do I Check If An Array Includes A Value In Javascript
Javascript Array Contains A Step By Step Guide Career Karma
0 Response to "26 Javascript Array Not Includes"
Post a Comment