30 Javascript Text Selection Event
An event is something that happens when user interact with the web page, such as when he clicked a link or button, entered text into an input box or textarea, made selection in a select box, pressed key on the keyboard, moved the mouse pointer, submits a form, etc. Each element in this list is a DOM <option> element — so you can use the value and text property to get the value and inside text of the option. // For a normal select (and not multi-select) the list would contain only a single element var text = document.querySelector('#choose-fruit').selectedOptions[0].text; var value = document ...
Detect Hover On Selected Text Stack Overflow
Apr 28, 2021 - Click, drag, release: you’ve just selected some text on a webpage — probably to copy and paste it somewhere or to share it. Wouldn't it be cool if
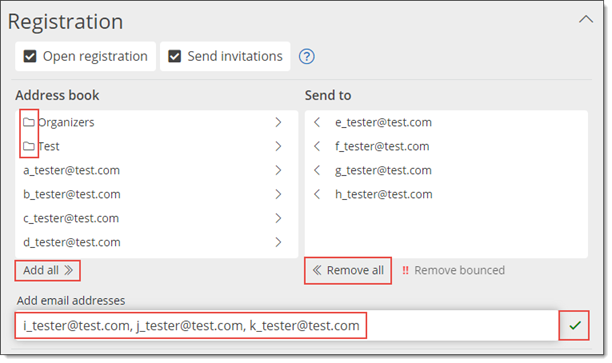
Javascript text selection event. A Selection object represents the range of text selected by the user or the current position of the caret. To obtain a Selection object for examination or manipulation, call window.getSelection (). A user may make a selection from left to right (in document order) or right to left (reverse of document order). Sep 04, 2018 - A disadvantage of this approach is that it doesn't work when a user selects text using the keyboard (Shift+→, etc.). ... @Marcel Korpel: In some sort of textarea or input, yes, but you could use the onselect event for those. The select event fires when some text has been selected.
The onSelect event occurs when the user selects text by dragging the mouse across a certain part of the text. We can write JavaScript code for this event. In Example 16.2, when the user types some characters into the text area and then select a part of the text, the selected will be shown to the user via a pop-up dialog. Example 16.2 2 weeks ago - The change event triggers when the element has finished changing. For text inputs that means that the event occurs when it loses focus. The HTML onchange event occurs when an element's value is changed. For example when you change/select a value in dropdown or enter or remove text in a textbox, the onchange event occurs. You can attach JavaScript to perform some actions as the onchange event occurs.
Jul 07, 2019 - Selection change event notes. GitHub Gist: instantly share code, notes, and snippets. How it works: First, select the <button> and <select> elements using the querySelector () method. Then, attach a click event listener to the button and show the selected index using the alert () method when the button is clicked. Feb 09, 2012 - This is not just for google chrome extension but also for javascript I am writing a chrome extension in which when a text is highlighted and a context menu is shown i display my item in the context
Disabling text selection, cut, copy, and right-click on a Page with jQuery and JavaScript This post will discuss how to stop content theft from your website simply by disabling text selection, cut, copy, and right-click function via mouse and keyboard. Introduction to JavaScript onchange onchange event in JavaScript is an important event which is used for the event changes occurring at the time of performing the event. onchange event occurs in full fledge as soon as the state of the value gets changed while performing the event. To select all text inside an element such as DIV, we can simply use JavaScript document.createRange () method to create a range, and than using range.selectNodeContents () we can set node range (start and end), after which use selection.addRange () to select the range of the element.
There may be a need to find out the text selected/highlighted by the user. It can be done very easily using the window and document objects and their properties. Handling selected text is different for different browsers. The ways to get selected text are shown below: Example-1: By using window.getSelection property Document: selectionchange event. The selectionchange event of the Selection API is fired when the current text selection on a document is changed. Bubbles. Javascript Form Events : Event handler onClick. Using the event handler onClick is the most frequently used in form, or elsewhere to trigger event handler function on click events. A click event is set to take place when the button within a form, radio or checkbox is pressed or when a selection is made. Example: Javascript DOM for Event Handling.
Listening for events. All public events are relayed using the jQuery event system, and they are triggered on the <select> element that Select2 is attached to. You can attach to them using the .on method provided by jQuery: $('#mySelect2').on('select2:select', function (e) { // Do something }); JavaScript - Difference between Var, Let, and Const Keyword; In this article, we are discussing how to handle the dropdown selected index change event in HTML using JavaScript. We saw how to show or hide textbox based on dropdown selected index change event in HTML form. Using JavaScript change event for the select element The <select> element fires the change event once the selection has completed. The following example shows how to handle the change event of the <select> element. The <p> element with the id result will display the selected item:
Mar 15, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. For text inputs that means that the event occurs when it loses focus. For instance, while we are typing in the text field below - there's no event. But when we move the focus somewhere else, for instance, click on a button - there will be a change event: <input type="text" onchange="alert (this.value)"> <input type="button" value="Button"> The onselect event occurs after some text has been selected in an element. The onselect event is mostly used on <input type="text"> or <textarea> elements.
Type the starting character number and end character number. Then click on "Set Selection" button to set selection. How to get caret position or selection Click on "Get Position/Selection". How onfocus Event work in JavaScript? The text box is the one situation where onfocus can be used. In addition to that, when a user tries to stroll down the list of items in a particular box and the person has moved the cursor on each and every option, at a particular time, that option present in the select box is at the focus. The dispatchEvent delivers an Event at the specified EventTarget, synchronously executing the affected EventListeners in an appropriate order. Unlike native events that are fired by the DOM and call event handlers asynchronously with the help of the event loop, the dispatchEvent execute event handlers synchronously.
var cbobject= document.testform.rb1; </script>. Here are the events, dom properties and method associated with Select Box element. Event Handlers: Associated with Form type SelectBox: All the examples below use a javascript function output. <script language=javascript>. function output () {. alert ("testing Select Option events"); event | select event. Occurs after some text has been selected in an element. Use the onselect event on the textarea, input:password and input:text elements for cross-browser functionality. Support for the onselect event on other elements is browser-dependent. - JavaScript - Get selected value from dropdown list. About Mkyong . Mkyong is providing Java and Spring tutorials and code snippets since 2008.
Occurs after some text has been selected in an element. Use the onselect event on the textarea, input:password and input:text elements for cross-browser functionality. Support for the onselect event on other elements is browser-dependent. Event bubbling is an approach to listening for events that's better for performance and gives you a bit more flexibility. Instead of adding event listeners to specific elements, you listen to all events on a parent element (often the document or window). Events within that element "bubble up," and you can check to see if the element that triggered the event (the event.target) matches the ... Select and Deselect Text Inside an Element Using JavaScript By The Web Flash · November 3, 2015 It is pretty easy to select all text inside an <input> or a <textarea> by using select () method like so: document.querySelector ('#exampleInput').select ();
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. 5/5/2019 · There is a new experimental API that deals with this: The selectionchange event of the Selection API is fired when the selection object of the document is modified, or when the selection associated with an <input> or a <textarea> changes. The selectionchange event is fired at the document in the first case, on the element in the second case. Event Handler : Description : Example : onMouseOver: ... The following are the list of DOM (Dynamic Object Model) methods that can be used to do dynamic changes like dynamic text area selection using javascript. DOM Method : Description : Example : select() Used to dynamically select a text area : To Select: document.form1.textn.select();
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Input Events. onblur - When a user leaves an input field onchange - When a user changes the content of an input field onchange - When a user selects a dropdown value onfocus - When an input field gets focus onselect - When input text is selected onsubmit - When a user clicks the submit button onreset - When a user clicks the reset button onkeydown ... The event delegation is a useful pattern because you can listen for events on multiple elements using one event handler. Making the event delegation work requires 3 steps: Determine the parent of elements to watch for events. Attach the event listener to the parent element. Use event.target to select the target elements.
27/3/2021 · "select" – the newly inserted text will be selected. "start" – the selection range collapses just before the inserted text (the cursor will be immediately before it). "end" – the selection range collapses just after the inserted text (the cursor will be right after it). "preserve" – attempts to preserve the selection. This is the default. The following program shows how to get selected index value or selected text from a Javascript dropdownlist ... The following program shows how to dropdown list onchange event in Javascript. While you change the selection from javascriptcombobox you can see the color of Div element is changing. The change event is fired for <input>, <select>, and <textarea> elements when an alteration to the element's value is committed by the user. Unlike the input event, the change event is not necessarily fired for each alteration to an element's value.
5 days ago - The Window.getSelection() method returns a Selection object representing the range of text selected by the user or the current position of the caret · When cast to string, either by appending an empty string ("") or using Selection.toString(), this object returns the text selected
Javascript Text Selection Events Stack Overflow
Trumba Help Set Up Event Registration
Javascript By Sergio Forastieri Issuu
Floating Share Search Translate Buttons For Text Selection
Dom And Events Document Object Model Dom Events
Javascript Scheduler Select A Time Range Over Multiple Rows
Gh 6083 Selected Text In Cell Loses Text Selection When You
D3 S Selection Text Is Expensive Stack Overflow
Dom And Events Document Object Model Dom Events
How To Get The Highlighted Selected Text In Javascript
Calculating Xy Position Of Text Selection Stack Overflow
Ho To Catch Text Selection Event In The Pdf Viewer Issue
Chapter 4 1 Interacting With App From Console Simple
Trumba Help Set Up Event Registration
Javascript Detect Right Click Code Example
Properties Shown In Case Of Multiple Selection Autodesk Forge
Event Listener Works In On Context But Not Another Code
How To Captured Selected Text Range In Ios After Text
Drop Down Selection Populate Text Field Javascript
Angular 10 9 8 Ngselect With Single Multiple Selection And
Excel Tablecollection Add Triggers Redundant Workbook
Question Event Listener Selection For Itext Elements
Reference Block Elements Slack
How To Get The Highlighted Selected Text In Javascript
Lightweight Text Selection Javascript Library Text Select
0 Response to "30 Javascript Text Selection Event"
Post a Comment