25 Get Length Of Object Javascript
Jul 22, 2019 - Get code examples like "how to change length of object in javascript" instantly right from your google search results with the Grepper Chrome Extension. Object.Keys() method return's an array with all the keys present in the object. As array is essentially an object in javascript. It returns its indexes as the keys. let arr = []; arr[0] = 1; arr[99] = 10; let indexes = Object.keys(arr); console.log(indexes); //["0", "99"] Now we can get the length of this array of keys or indexes to find the ...
How To Check If Object Is Empty In Javascript Samanthaming Com
Jul 02, 2019 - Something an Object should have built in is the `.length` property. Which im sure in future specs of JavaScript it might — that is why we do a check first with the if statement to see if the length property doesnt exist. As a note, Object.keys has strong browser support down to IE 9.
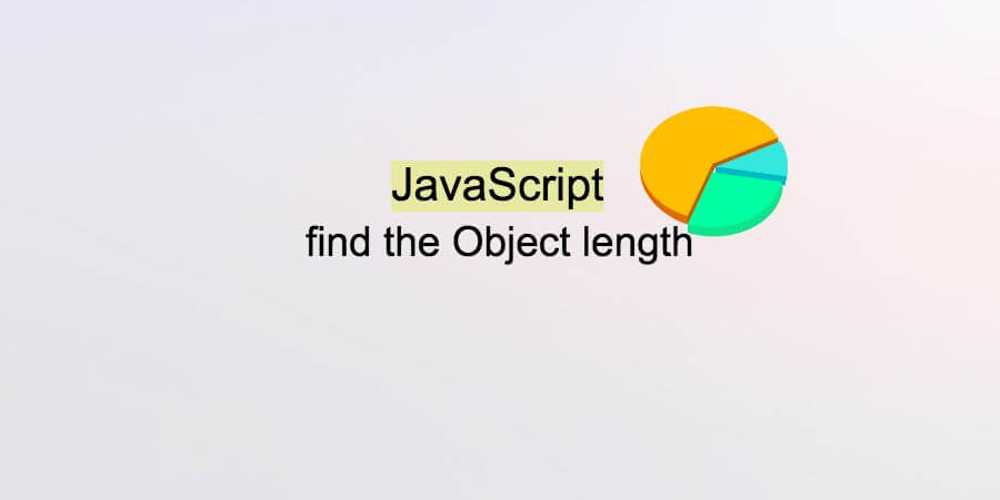
Get length of object javascript. Associative Array) it is not a accurate value javascript - How to get object length JavaScript - Get Object Size JavaScript Array length Property javascript object empty javascript object keys values javascript object length undefined javascript object size bytes JavaScript: get the number of properties in an object jquery object length ... While this certainly works, it would be nice to avoid using a hand-rolled method just to count properties. Surely JavaScript has something better available? Fortunately, it does. While it's not quite as simple as finding the length of an array, it is awfully close. In modern browsers, we can ... The Object.keys () method accepts the object as an argument and returns the array with enumerable properties. We know that array has a length property so that by using the Object.keys () method returned array we can get the Object size.
Learn JavaScript - Getting set length. Get monthly updates about new articles, cheatsheets, and tricks. 18/6/2019 · Method 1: Using the Object.keys() method: The Object.keys() method is used to return the object property name as an array. The length property is used to get the number of keys present in the object. It gives the length of the object. Syntax: objectLength = … javascript create variable containing an object that will contain three properties that store the length of each side of the box
Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object.The ordering of the properties is the same as that given by looping over the properties of the object manually. One of simplest and perhaps the quickest way to get the length of a given object in JavaScript is by using the length property of Object.keys () method. The Object.keys () method returns an array of object properties. For example, I have an array with few properties in it. Jul 26, 2010 - Description: The number of elements in the jQuery object. ... The number of elements currently matched. The .size() method will return the same value.
Finding the length of a JavaScript object; Sorting JavaScript object by length of array properties. How to get the length of an object in JavaScript? Best way to find length of JSON object in JavaScript; Length of a JavaScript associative array? Get the length of a StringBuffer Object in Java; What happens when length of object is set to 0 ... Create an object variable and assign values to it Create an variable which shows the size Call the Object.keys (object-name).length and assign it to the variable (in step 2). Example-1: To get size of javascript object by Object.keys () method. This means we can use the length property of the returned array to get the length of an object. This method has been available since ES6, so we can use it safely anywhere. For instance, we can write: const obj = { a: 1, b: 2 } const size = Object.keys (obj).length; console.log (size) We call Object.keys with the obj object to get an array of ...
javascript create variable containing an object that will contain three properties that store the length of each side of the box The length property sets or returns the number of elements in an array. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Learn how to find the length of a string in JavaScript. String Length. The length property returns the length of a string:
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: javascript create variable containing an object that will contain three properties that store the length of each side of the box Apr 29, 2020 - Get code examples like "how to find length of javascript object" instantly right from your google search results with the Grepper Chrome Extension.
Use the Object.keys() Method to Get the Length of an Object in JavaScript. The Object.keys() method returns an array of properties of the Object. We use the length property to get the number of keys. Example: const getLengthOfObject = (obj) => { let lengthOfObject = Object.keys(obj).length; console.log(lengthOfObject); } getLengthOfObject({ id: 1, name: 'Mark', age: 30 } ); Output: 3 In this article we will address how to get length of an object in JavaScript Length of an object means number of properties present in the object Object has Object.keys (object) method which returns keys present in the object. Number of keys in an object is the length of an object Apr 29, 2020 - javascript create variable containing an object that will contain three properties that store the length of each side of the box
Object.keys () method is used to return an array whose elements are strings corresponding to the enumerable properties found directly upon an object. The ordering of the properties is the same as that given by the object manually in a loop is applied to the properties. Object.keys () takes the object as an argument of which the enumerable own ... Here's an update as of 2016 and widespread deployment of ES5 and beyond. For IE9+ and all other modern ES5+ capable browsers, you can use Object.keys() so the above code just becomes:. var size = Object.keys(myObj).length; This doesn't have to modify any existing prototype since Object.keys() is now built-in.. Edit: Objects can have symbolic properties that can not be returned via Object.key ... calculate memory size of javascript object, it is not a accurate value! - memorySizeOfObject.js. calculate memory size of javascript object, it is not a accurate value! - memorySizeOfObject.js ... try to get size of this object. with your edit size = ~1.2kb but real size is ~300-400bytes. This comment has been minimized. Sign in to view.
26/2/2020 · //Write a JavaScript program to get the length of a JavaScript object. Object.objsize = function(Myobj) { var osize = 0, key; for (key in Myobj) { if (Myobj.hasOwnProperty(key)) osize++; } return osize; }; var student = { name : "David Rayy", sclass : "VI", rollno : 12 }; var objsize = Object.objsize(student); console.log('Size of the current object : '+objsize); May 03, 2020 - In JavaScript, almost everything is an object. Objects are used to store the information in key-value pairs, where a value can be any other data type or function. Unlike arrays and strings, objects… Array.prototype.length The length property of an object which is an instance of type Array sets or returns the number of elements in that array. The value is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array.
23/1/2021 · Code: Javascript. 2021-01-23 08:26:15. var person= { "first_name": "Harry" , "last_name": "Potter" , "age": 14 }; var personSize = Object .keys (person).length; //gets number of properties (3) 13. Andrew Bennett. Code: Javascript. 2021-05-20 07:48:43. //length of this object is 4 let object … I know you can get the length OUTSIDE of the object by creating a new variable var length = data.fields.length; However, I need the length to be inside of the object. ECMAScript 2016 (ed. 7) established a maximum length of 2^53 - 1 elements. Previously, no maximum length was specified. In Firefox, strings have a maximum length of 2**30 - 2 (~1GB). In versions prior to Firefox 65, the maximum length was 2**28 - 1 (~256MB). For an empty string, length is 0.
Apr 29, 2020 - javascript create variable containing an object that will contain three properties that store the length of each side of the box How to get the size of a JavaScript object? Ask Question Asked 12 years ago. Active 7 months ago. Viewed 302k times ... yet it've helped me a few times in the past to get the approx object file size: Write your object/response to the console or a new tab, copy the results to a new notepad file, save it, and check the file size. ... Javascript get array length Example. Let's see the simple example of How to JavaScript check array length. On Clicking a button "myFunction()" function will call. In a function, the first script will create an array and assigning value to the count variable. And in last show this value in a p element.
Object.keys(empty).length === 0 evaluates to true, which means that empty has no properties. 2. Object.values() returns values. Object.values(object) is the JavaScript utility function that returns the list of values of object. Let's use this function to get the values of hero object: To get the number of keys in a JSON object in javascript you can use one of the following 2 methods. Using Object.keys() The Object.keys() method returns an array of a given object's own enumerable property names, in the same order as we get with a normal loop. Feb 18, 2021 - Learn how to find the length of an object in JavaScript
As you can see Object.getOwnPropertyNames will return ALL property keys, whereas Object.keys will just return the enumerable property keys. As I mentioned before, enumerable attributes are maybe hidden for a reason, so you might not want to access that. Therefore, Object.getOwnPropertyName might not be the method you want to use to get the length of an object. 5/7/2019 · In javascript, we have Object.keys () property, which checks whether there are any properties or not. If we use the length property with Object.keys () then the number of properties will be displayed which is nothing but the length of the object. This method can also be used to limit or set the length of the array, by using the syntax 'Array_name.length = N', where N represents the user's desired number to be set as the length of the array.
How To Get The Length Of A Javascript Object By John Au
Samantha Ming On Twitter Checking Empty Object Here 39 S A
Proful Sadangi On Twitter Javascript Basics Length Of A
Debugging Quot Cannot Read Property Length Of Undefined
Fastest Javascript Key Value Pair Object Length
Measuring Size Of Objects In An Image With Opencv Pyimagesearch
Javascript Objects Explore The Different Methods Used To
Pivot A Javascript Array Convert A Column To A Row Techbrij
How To Find Array Length In Java Javatpoint
How Can I Calculate Length On The Object Observer In The
Javascript Object Find The Length Of An Object W3resource
Find Distance From Camera To Object Using Python And Opencv
Javascript Array Distinct Ever Wanted To Get Distinct
How To Find Object Length In Javascript Reactgo
Group Array Objects Using Javascript
Typescript String Length Example
Dynamic Array In Javascript Using An Array Literal And
Hacks For Creating Javascript Arrays
Interfaces In Typescript What Are They And How Do We Use
Javascript String Length Examples To Implement String Length
Javascript Array Methods How To Use Map And Reduce
Hacks For Creating Javascript Arrays
Trouble Getting Size Of An Array In A Js Object Inside
0 Response to "25 Get Length Of Object Javascript"
Post a Comment