34 Javascript Question Mark Operator
TypeScript question mark are used on variables to mark it as an optional parameter. When declaring a TypeScript variable, the declared variable becomes an optional parameter. This optional parameter will have undefined if not used. "Question mark" or "conditional" operator in JavaScript is a ternary operator that has three operands. The expression consists of three operands: the condition, value if true and value if false. The evaluation of the condition should result in either true/false or a boolean value.
Javascript Ternary Operator Without Else Condition Is It
The double question mark operator is called the nullish coalescing operator, and it's a new feature of JavaScript ES2020 that allows you provide a default value to use when a variable expression evaluates to null or undefined.
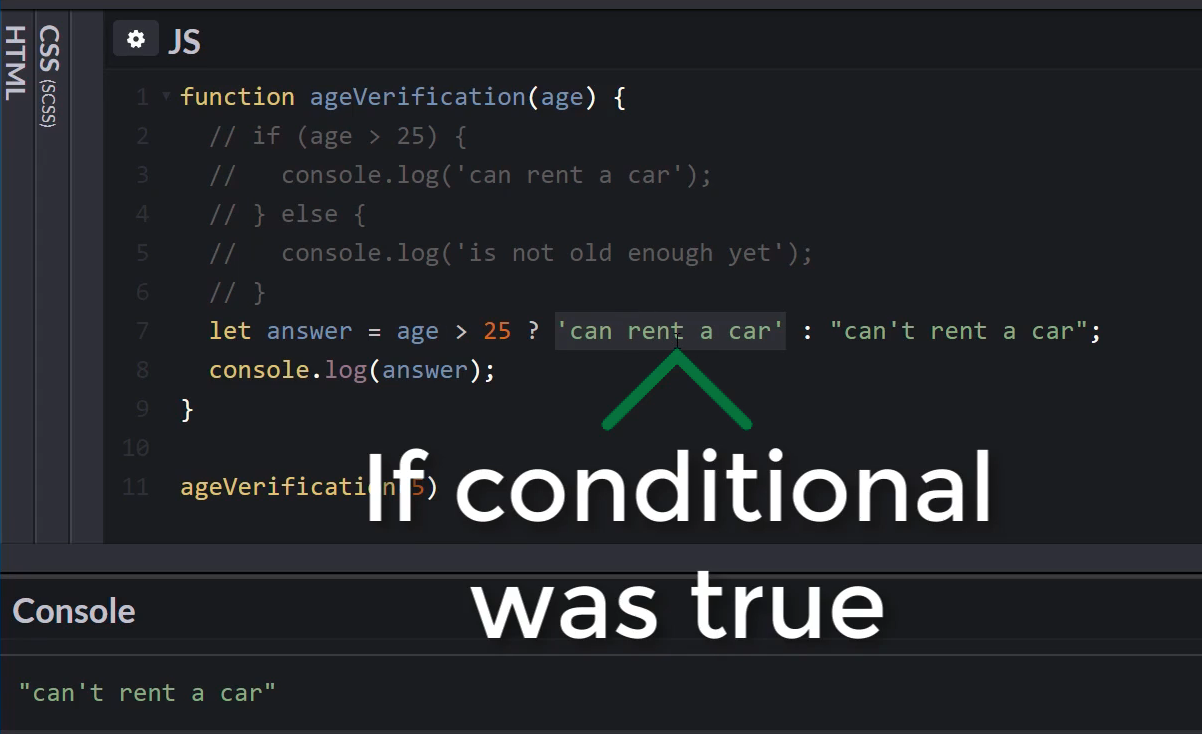
Javascript question mark operator. JavaScript string must be enclosed in double or single quotes (" " or ' '). String can be assigned to a variable using = operator. Multiple strings can be concatenated using + operator. A string can be treated as character array. Use back slash (\) to include quotation marks inside string. If you see double question mark (??) in typescript or javascript code and wonder what the hack is this? Well let me tell you that this is a is a logical operator being introduced in ECMAScript 2020 and new typescript version 3.7 One can think of an object as an associative array (a.k.a. map, dictionary, hash, lookup table).The keys in this array are the names of the object's properties.. It's typical when speaking of an object's properties to make a distinction between properties and methods.
Although not the most common use of a logical operator in JavaScript, the "double bang" or double exclamation mark "!!" recently caught my attention. Let's dive into its mechanics ... If you have ever noticed a double exclamation mark (!!) in someone's JavaScript code you may be curious what it's for and what it does. It's really simple: it's short way to cast a variable to be a boolean (true or false) value. Let me explain. typeof JavaScript != 'static' JavaScript is not a static language, rather it is a dynamic language. If you are new to JavaScript, the question mark after a variable may be confusing to you. Let's shed some light into it. The question mark in JavaScript is commonly used as conditional operator -- called ternary operator when used with a colon (:) and a question mark (?) -- to assign a variable name conditionally. const isBlack = false;
The nullish coalescing operator is written as two question marks ??.. As it treats null and undefined similarly, we'll use a special term here, in this article. We'll say that an expression is "defined" when it's neither null nor undefined.. The result of a ?? b is:. if a is defined, then a,; if a isn't defined, then b.; In other words, ?? returns the first argument if it's not ... The question mark here is a a ternary if operation, Simply put, it is a short, inline if statement. If the statement before the question mark evaluates to true, then the left-hand side of the colon is used, otherwise (if it is false) the right-hand side is used. You can also nest this, though it isn't always a good idea (for readability). The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as a shortcut for the if statement.
Aug 31, 2017 - The Null Propagation operator is a native solution to the problem, allowing us to handle these cases by sprinkling our code with question marks. The operator is all of ?., as we’ll see in a bit. es6 what is double exclamation operator. javascript is single threaded. javascript listen for double click. javascript multiples of 3 and 5. javascript multiplication. Javascript multiply a square matrix. javascript on double click. javascript operator double not. javascript operator double pipes. Nov 19, 2020 - The purpose of the question mark operator ? is to return one value or another depending on its condition. — JavaScript.info in August 2019 · “A ?” “What is a ? doing in my JavaScript code?” The ? character jumped off the screen when it showed up in my JavaScript programming. Thankfully, the question ...
It's the null conditional operator. It basically means: "Evaluate the first operand; if that's null, stop, with a result of null. Otherwise, evaluate the second operand (as a member access of the first operand)." “Question mark” or “conditional” operator in JavaScript is a ternary operator that has three operands. The requirements of this app are as follows: Grade of 90 and above is an A. Grade of 80 to 89 is a B. Grade of 70 to 79 is a C. Grade of 60 to 69 is a D. Grade of 59 or below is an F. Below we will create a simple set of if, else, and else if statements, and test them against a given grade. grades.js.
To be pedantic, it's a ternary operator, which happens to be the only one in most programming languages. Any operator that works on 3 parts is a ternary operator, just like addition is a binary operator that operates on the preceding and following expressions (e.g. 1+2 the plus operates on 1 and 2), or negation is a unary operator (e.g. -x where the value of x is negated). Jan 23, 2021 - The so-called “conditional” or “question mark” operator lets us do that in a shorter and simpler way. The operator is represented by a question mark ?. Sometimes it’s called “ternary”, because the operator has three operands. It is actually the one and only operator in JavaScript ... The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. This can be contrasted with the logical OR (||) operator, which returns the right-hand side operand if the left operand is any falsy value, not only null or undefined.
By using the ?. operator instead of just., JavaScript knows to implicitly check to be sure obj.first is not null or undefined before attempting to access obj.first.second. If obj.first is null or undefined, the expression automatically short-circuits, returning undefined. Feb 14, 2021 - In JavaScript the ? operator is used in conditional statements, and when paired with a :, can functio... Before JavaScript introduced default values for functions, everyone used || operator to assign default values to parameters e.g. But if you use || to provide a default value, you may encounter unexpected behavior if you consider some values as usable (e.g., '' or 0). Let's demonstrate with an example. Here, we have '0' and 1000 in variable ...
Apr 26, 2020 - ES2020 introduced the optional chaining operator denoted by the question mark followed by a dot: ... To access a property of an object using the optional chaining operator, you use one of the following: The so-called "conditional" or "question mark" operator lets us do that in a shorter and simpler way. The operator is represented by a question mark ?. Sometimes it's called "ternary", because the operator has three operands. It is actually the one and only operator in JavaScript which has that many. Introduction to the JavaScript nullish coalescing operator ES2020 introduced the nullish coalescing operator denoted by the double question marks (??
Learn how use optional chaining in JavaScript. We'll break down how to use the chain operator using a question mark (?.) and how you can use it to safely acc... Mar 02, 2021 - The conditional or question mark operator, represented by a ?, is one of the most powerful features in JavaScript. The ? operator is used in conditional statements, and when paired with a :, can function as a compact alternative to if...else statements. But there is more to it than meets the eye. Hi folks. I wanted to take this opportunity to talk about one of the new features of TypeScript 3.7 which, conveniently, will also be included in vanilla JavaScript. The feature is called Nullish Coalescing and it's summed up here by the good folks at TypeScript. But if you're a glutton for punishment, please read on.
The Null Propagation operator is a native solution to the problem, allowing us to handle these cases by sprinkling our code with question marks. The operator is all of ?., as we'll see in a bit. const firstName = person.profile ?. name ?. firstName Note that the ?. goes right before the property access. The conditional ternary operator in JavaScript assigns a value to a variable based on some condition and is the only JavaScript operator that takes three operands. The ternary operator is a substitute for an if statement in which both the if and else clauses assign different values to the same field, like so: The conditional or question mark operator, represented by a ?, is one of the most powerful features in JavaScript. The ? operator is used in conditional statements, and when paired with a :, can function as a compact alternative to if...else statements. But there is more to it than meets the eye.
Apr 17, 2018 - Not the answer you're looking for? Browse other questions tagged javascript syntax operators conditional-operator or ask your own question. Assignment operators are right-associative, so you can write: a = b = 5; Copy to Clipboard. with the expected result that a and b get the value 5. This is because the assignment operator returns the value that is assigned. First, b is set to 5. Then the a is also set to 5, the return value of b = 5, aka right operand of the assignment.
Typescript Question Mark Complete Guide To Typescript
Null Propagation Operator In Javascript
Null Propagation Operator In Javascript
Javascript Conditional Statements Become An Expert In Its
What S The Double Exclamation Mark For In Javascript Brian
Question Mark Postgres Operator Issue 1648 Vincit
Javascript Variable With Question Mark
What Does The Question Mark Mean In Javascript Code By
Top 50 Javascript Interview Questions And Answers For 2021
What Is The Question Mark Operator In Javascript
The Javascript Ternary Operator As A Shortcut For If Else
Typescript Question Mark Complete Guide To Typescript
How The Question Mark Operator Works In Javascript
Top 50 Javascript Interview Questions And Answers For 2021
Null Propagation Operator In Javascript
Javascript Conditional Statements Become An Expert In Its
Typescript Question Mark Complete Guide To Typescript
Javascript Double Question Mark Code Example
What Does The Question Mark Mean In Javascript Code By
Optional Chaining Amp Assertion Operator By Zohaib Codeburst
Javascript Conditional Statements Become An Expert In Its
What Does The Question Mark Mean In Javascript Code By
Javascript Ternary Operator Without Else Condition Is It
Which Equals Operator Vs Should Be Used In
Conditional Operator In C Javatpoint
Top 50 Javascript Interview Questions And Answers For 2021
What Does The Question Mark Mean In Javascript Code By
What Is The Javascript Ternary Operator By Aiman Rahmat
Ternary Operator Shorthand Javascript Code Example
How The Question Mark Operator Works In Javascript
Logical Operators In Javascript Devopsschool Com
0 Response to "34 Javascript Question Mark Operator"
Post a Comment