29 How To Remove Item From Array Javascript
Summary: in this tutorial, you will learn how to remove duplicates from an array in JavaScript. 1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Use Array.filter () to Remove a Specific Element From JavaScript Array The filter methods loop through the array and filter out elements satisfying a specific given condition. We can use it to remove the target element and keep the rest of them. It helps us to remove multiple elements at the same time.
Javascript Remove The Last Item From An Array Geeksforgeeks
20/5/2020 · JavaScript provides many ways to remove elements from an array. You can remove an item: By its numeric index. By its value. From the beginning and end of the array. Removing an element by index. If you already know the array element index, just use the Array.splice() method to remove it from the array. This method modifies the original array by removing or replacing existing elements and returns the removed elements …
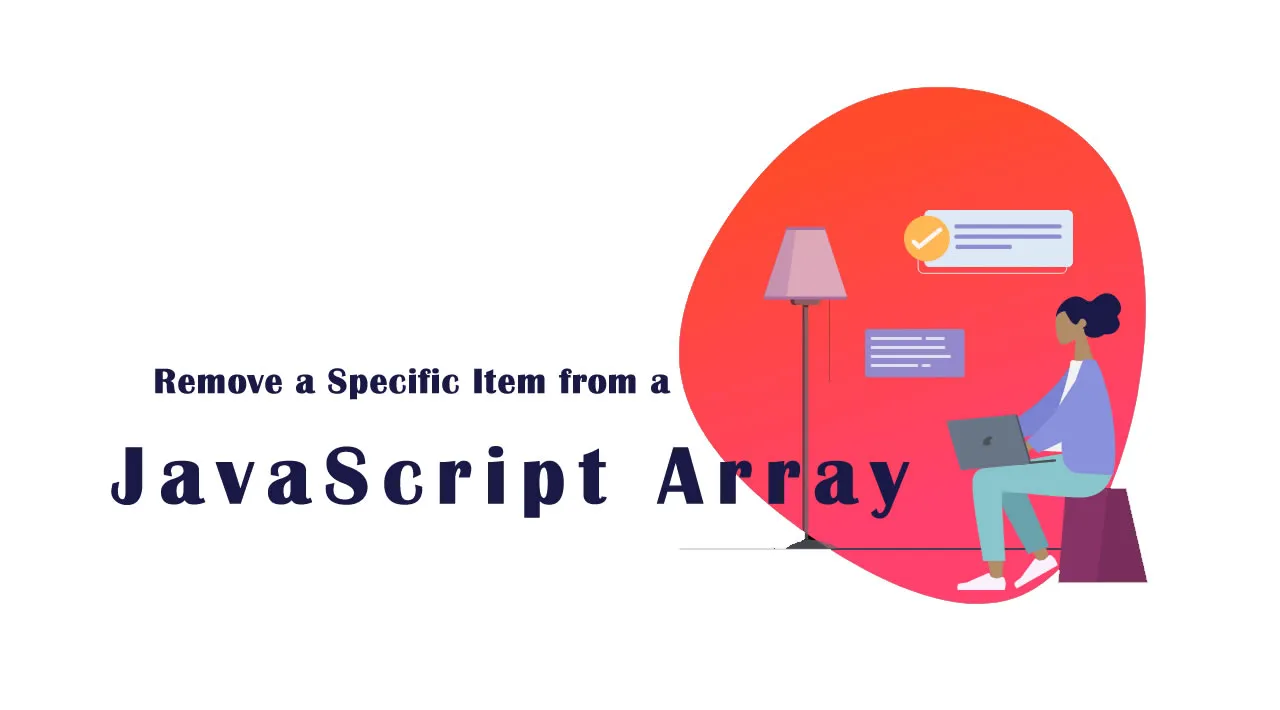
How to remove item from array javascript. 4/8/2009 · Array.prototype.remove = Array.prototype.remove || function() { this.splice(0, this.length); }; and you can simply call it like this to empty any array in your javascript application: arr.remove(); //[] The JavaScript is a very powerful programming language and provides a great support for arrays. There are many different language constructs available that you can use to remove item from an array. This article contains description of only the most popular ways to modify an array, but you can extend and improve them to meet your specific needs. JavaScript Array splice () method This method adds/deletes items to/from the array, and returns the deleted item (s).
To remove an element from a JavaScript array using the Array.prototype.splice () method, you need to do the following: Pass the number of elements you wish to remove as the second argument to the method. Please be aware that the Array.prototype.splice () method will change/mutate the contents of the original array by removing the item (s) from it. 20/4/2020 · Removing an item from an Array One way to solve this problem is using Array.prototype.indexOf () to find the index of the value, then Array.prototype.splice () to remove that item: Note that.indexOf returns -1 if the index is not found, but.splice interprets an index of -1 as the last item in the array, just like.slice. 20. Two solutions, one evolve creating new instance and one changes the instance of your array. Filter: idToRemove = DESIRED_ID; myArr = myArr.filter (function (item) { return item.Id != idToRemove; }); As you can see, the filter method returns new instance of the filtered array. Second option is to find the index of the item and then remove it ...
17/7/2021 · filter (): In a given JavaScript array, when we need to remove one or more than one item based on some condition then we use arr.filter () method to perform the operation. For example, lets take a numbers array is numArray = [4,33,42,7,52,84,11,8,]. Now from this array if we want values of more than 10 then we will use filter () method. In JavaScript, the Array.splice () method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice () returns the removed elements (if any) as an array. @David you can compare the length of the two arrays to get how many items were deleted (i.e. arr.length - result.length). And to delete them from the original array you can just replace it arr = result; - Ahmad Ibrahim Feb 17 '17 at 1:09
push () / pop () — add/remove elements from the end of the array unshift () / shift () — add/remove elements from the beginning of the array concat () — returns a new array comprised of this array joined with other array (s) and/or value (s) Found a problem with this page? The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: Array.splice (position,num); To delete elements from an array, we have to pass two arguments within the splice function, the two arguments are: The position argument specifies the position of the first item to delete The num argument specifies the number of elements to delete.
9/1/2021 · JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. var ar = [1, 2, 3, 4, 5, 6]; ar.length = 4; console.log( ar ); To remove an item from an array by value, we can use the splice () function in JavaScript. The splice () function adds or removes an item from an array using the index. To remove an item from a given array by value, you need to get the index of that value by using the indexOf () function and then use the splice () function to remove the value ... There are many cases when it needs to remove some unused values from the Array which no longer needed within the program. Inbuilt functions are available in JavaScript to do this. Some functions auto-arrange the Array index after deletion and some just delete the value and not rearrange the index.
25/7/2018 · How to Remove an Element from an Array in JavaScript JavaScript suggests several methods to remove elements from existing Array . You can delete items from the end of an array using pop() , from the beginning using shift() , or from the middle using splice() functions. To remove elements or items from any position in an array, you can use the splice() array method in JavaScript. Jump to full code; Consider this array of numbers, // number array const numArr = [23, 45, 67, 89]; What if we need to remove the element at index 1 in the array, in our case 45. We can do that with the splice() array method. The ... Here are a few ways to remove an item from an array using JavaScript. All the method described do not mutate the original array, and instead create a new one. If you know the index of an item. Suppose you have an array, and you want to remove an item in position i. One method is to use slice():
One operation that seems to be more difficult than it should be in every programming language is removing a value from an array. It's such an easy concept mentally that it skews our programmatic view of the task. In JavaScript the splice method is of huge help in removing an item from an array. JavaScript Splice Here are a few ways to remove an item from an array using JavaScript. All the method described do not mutate the original array, and instead create a new one. If you know the index of an item. Suppose you have an array, and you want to remove an item in position i. One method is to use slice(): Removing Elements from End of a JavaScript Array The simpl e st way to remove one or more elements from the end from an array of elements is by setting the length property to a value less than the...
Definition and Usage The splice () method adds and/or removes array elements. splice () overwrites the original array. Whatever you do, don't use delete to remove an item from an array. JavaScript language specifies that arrays are sparse, i.e., they can have holes in them. Using delete creates these kinds of holes. It removes an item from the array, but it doesn't update the length property. This leaves the array in a funny state that is best avoided. To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. To remove null or undefined values do the following:
To remove an object from the array in Javascript, use one of the following methods. array.pop () - The pop () method removes from the end of an Array. array.splice () - The splice () method deletes from a specific Array index. array.shift () - The shift () method removes from the beginning of an Array. To remove a particular element from an array in JavaScript we'll want to first find the location of the element and then remove it. Finding the location by value can be done with the indexOf() method, which returns the index for the first occurrence of the given value, or -1 if it is not in the array. 01 Remove Array Item by Using splice () An array splice () is used for two purposes in JavaScript. The first use is to remove array items and the second use is to add array items. Syntax: 1. array.splice (item-index, number-of-item-remove, add-item1, ....., add-itemX)
The indexOf () method returns the index of the given element. If the element is not in the array, indexOf () returns -1. The if condition checks if the element to remove is in the array. The splice () method is used to remove the element from an array.
How To Remove An Element From A Javascript Array By Eniola
Remove Element From Array Javascript First Last Value
Javascript Array Merge Two Arrays And Removes All Duplicates
Remove Item From List Javascript Code Example
Javascript Array Remove Element From Array Tuts Make
Java67 How To Remove An Element From Array In Java With Example
How To Remove Item From Array By Value
5 Way To Append Item To Array In Javascript Samanthaming Com
Javascript Array Splice Delete Insert And Replace
How To Remove A Specific Item From An Array In Javascript
How To Remove Commas From Array In Javascript
9 Ways To Remove Elements From A Javascript Array
Vue Js Remove Item From Array Example Tuts Make
How To Remove A Specific Item From A Javascript S Array Tl
The Fastest Way To Remove A Specific Item From An Array In
How To Remove Array Duplicates In Es6 By Samantha Ming
C Program To Delete An Element From An Array
Js How To Remove Item From Array Code Example
Lodash Remove Item From Array Pakainfo
How To Remove A Specific Item From A Javascript Array
Delete A Specific Item Of An Array In Javascript Debajit S
How To Remove Duplicate Objects From An Array In Javascript
Remove Multiple Item From An Array In Javascript Dev Community
Javascript Remove Object From Array
5 Ways To Remove Items From An Array In Javascript Laptrinhx
How To Remove A Property From A Javascript Object
How Can I Remove A Specific Item From An Array Stack Overflow
Remove Item From An Array Of Objects By Obj Property Renat
0 Response to "29 How To Remove Item From Array Javascript"
Post a Comment