33 Sort Array By Field Javascript
You want to sort it in Javascript, right? What you want is the sort () function. In this case you need to write a comparator function and pass it to sort (), so something like this: Get code examples like "javascript sort array of objects by key value ascending" instantly right from your google search results with the Grepper Chrome Extension.
Sorting Multiple Columns In A Table With React Dmc Inc
Sorting arrays in JavaScript is done via the method array.sort(). Passing in a function reference into array.sort() As touched on already, array.sort() accepts an optional parameter in the form of a function reference (lets call it sortfunction).
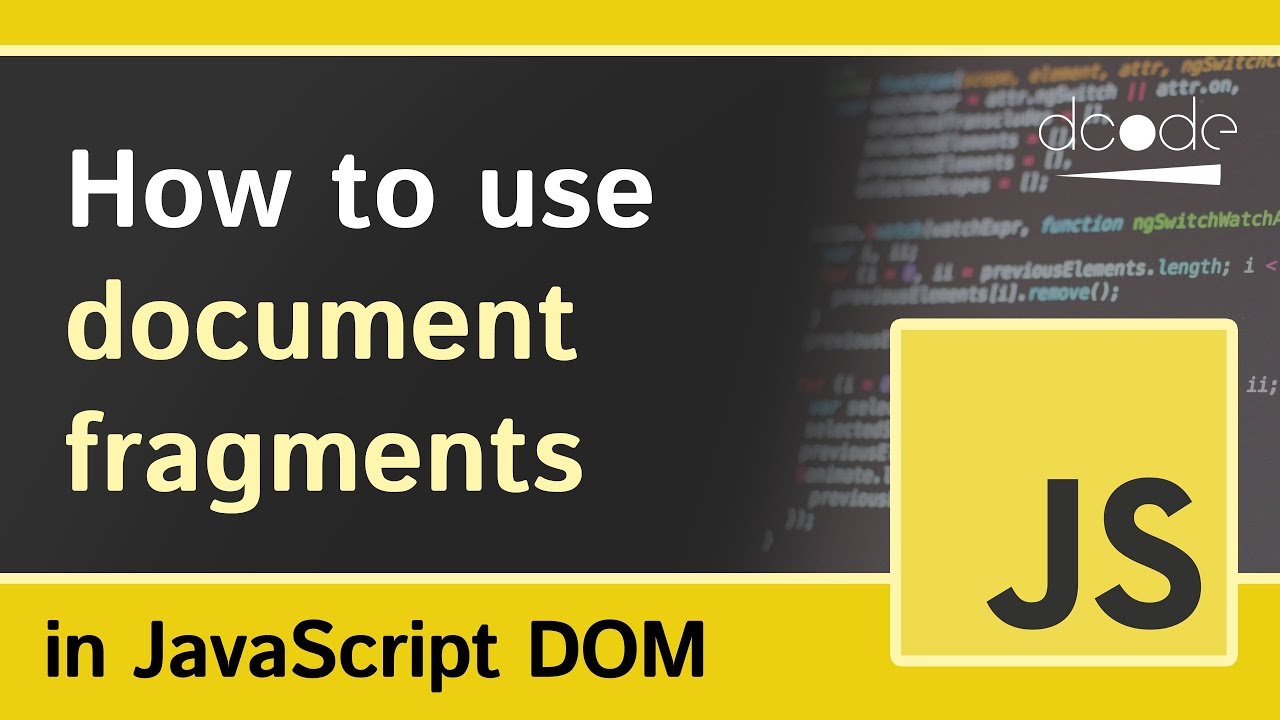
Sort array by field javascript. All Languages >> Javascript >> Next.js >> sort array of object by field javascript "sort array of object by field javascript" Code Answer's. javascript sort array by object property . javascript by Grepper on Jul 22 2019 ... Quick way to sort a JavaScript array by multiple fields How to avoid using if statements when sorting arrays by multiple keys in JavaScript. December 20, 2020 · 4 min read. Let's say we have an array of structured objects, like an array of data about the 30 NBA teams: May 20, 2020 - Get code examples like "sort array by field typescript" instantly right from your google search results with the Grepper Chrome Extension.
JavaScript arrays have the sort( ) method, which sorts the array elements into alphabetical order. The sort( ) method accepts a function that compares two items of the Array.sort([comparer]) . Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) Nov 26, 2019 - Sort an array of objects in JavaScript dynamically. Learn how to use Array.prototype.sort() and a custom compare function, and avoid the need for a library. The task is to sort the JavaScript array on multiple columns with the help of JavaScript. There are two approaches that are discussed below. Approach 1: Combine the multiple sort operations by OR operator and comparing the strings. For comparing the string, we will use the localeCompare () method.
The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana"). JavaScript objects sort by . field · how to sort the array of object depending upon the value inside the object node js you can call a.reverse () after the sort () if you want it sorted the other way.. EDIT: edited to reflect updated question of sorting an array of objects instead of an array of booleans.
Introduction to JavaScript Array sort () method The sort () method allows you to sort elements of an array in place. Besides returning the sorted array, the sort () method changes the positions of the elements in the original array. Nov 08, 2018 - The point here is that pure JavaScript with functional approach can take you a long way without external libraries or complex code. It is also very effective, since no string parsing have to be done ... /** * @description * Returns a function which will sort an * array of objects by the given key. JavaScript objects sort by . field ... Install and run react js project... ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0. ... Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control...
When we return 1, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning -1 would do the opposite.. The callback function could calculate other properties too, to handle the case where the color is the same, and order by a secondary property as well: Introduction to JavaScript- This tutorial introduces to you the very basics of JavaScript In the above program, the sort () method is used to sort an array by the name property of its object elements. The sort () method sorts its elements according to the values returned by a custom sort function ( compareName in this case). Here, The property names are changed to uppercase using the toUpperCase () method.
We also have a variable by the name of selfName that contains the name of a particular player −. const selfName = 'Arnav'; We are required to write a function that sorts the scorecard array in alphabetical order and makes sure the object with name same as selfName appears at top (at index 0). Therefore, let's write the code for this problem ... The JavaScript sort () method reads the values in an array and returns those values in either ascending or descending order. A string containing either numbers or strings can be sorted. Consider the following syntax: const values = [ 1, 2, 8, 9, 3 ]; values. sort (); This code sorts our "values" list. First, install syntax. sortBy (array or objects, [iteratekeys]) Input is array or objects iterate keys enables to sort. Return is sorted array. Following is an example for sort object with key values of an object array in ascending order. import all utilities using import keyword. an animal object has key-id,name and its values.
Array.sort(): The sort method takes the callback function as its argument and returns the sorted array. The return condition inside the callback function. if our return condition is a - b then it sorts the array in ascending order.. if our return condition is b - a then it sorts the array in descending order.. Let's sort our vegetables array in descending order. JavaScript sort multi dimensional array on specific columns. ... /** * Sorts an array of objects by column/property. * @param {Array} array - The array of objects. * @param {object} sortObject - The object that contains the sort order keys with directions (asc/desc). e.g. { age: 'desc', name: 'asc' } * @returns {Array} The sorted array. ... Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects.
This will sort your array by "one" and if they are the same (ie. 0) then it will sort by "two". It's simple, concise, readable, and best of all - works perfectly. When we return a positive value, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning a negative value will do the opposite. The sort() method returns a new sorted array, but it also sorts the original array in place. Thus, both the sortedActivities and activities arrays are now sorted. One option to protect the original array from being ... Apr 22, 2021 - Let's see how you can completely configure the sorting of an Array of objects. Let's say we have the...
Last week, my friend Andrew Borstein was playing around with a JavaScript upvoting system, and asked: I want to sort an array based on two properties. First sort by an integer, next sort by a string. So an array of objects might look like this: [ { title: 'Apple', votes: 1 }, { title: 'Milk', votes: 2 }, { title: 'Carrot', votes: 3 }, { title: 'Banana', votes: 2 } ] and then be sorted into this When you are working with an array of objects that contains a date field and we want to sort an array based on the date values then we can use the sort () method of an array. There are many ways to do sorting in JavaScript but in this article, we will go with the default function sort () of an array. Sort array based on presence of fields in objects JavaScript. ... We are required to sort this array so that the object with both firstName and lastName property appears first then the objects with firstName or lastName and lastly the objects with neither firstName nor lastName.
How to sort an array of object by two fields in JavaScript ? Last Updated : 13 Dec, 2019. Given an array of objects and the task is to sort the array elements by 2 fields of the object. There are two methods to solve this problem which are discussed below: Approach 1: Sort by field. We've got an array of objects to sort: The usual way to do that would be: Can we make it even less verbose, like this? So, instead of writing a function, just put byField (fieldName). Write the function byField that can be used for that. Open a sandbox with tests. Sorts array in place by values in ascending order. ... If two members compare as equal, they retain their original order. Prior to PHP 8.0.0, their relative order in the sorted array was undefined.
If you want to sort based on second column (which contains string value), then try this: arr.sort (function (a,b) { return a [1].charCodeAt (0)-b [1].charCodeAt (0) }) P.S. for the second case, you need to compare between their ASCII values. Hope this helps. "sort array by field" Code Answer's. javascript sort array of object by property . javascript by Friendly Hawkes on Feb 11 2020 Donate Comment Friendly Hawkes on Feb 11 2020 Donate Comment Jan 31, 2020 - In this tutorial, you have learned how to sort an array of objects by the values of the object’s properties. ... Primitive vs. Reference Values ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively.
Jul 08, 2019 - Arrays in JavaScript come with a built-in function that is used to sort elements in alphabetical order. However, this function does not directly work on arrays of numbers or objects. Instead a custom function, that can be passed to the built-in sort() method to sort objects based on the ... Underscore.js | sortBy () with Examples. The Underscore.js is a JavaScript library that provides a lot of useful functions that helps in the programming in a big way like the map, filter, invoke etc even without using any built-in objects. The _.sortBy () function is used to sort all the elements of the list in ascending order according to the ... Array.prototype.sort () The sort () method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values.
May 20, 2020 - JavaScript objects sort by . field · how to sort the array of object depending upon the value inside the object node js Numeric Sort By default, the sort () function sorts values as strings. This works well for strings ("Apple" comes before "Banana"). However, if numbers are sorted as strings, "25" is bigger than "100", because "2" is bigger than "1".
Javascript Fundamental Es6 Syntax Get The Median Of An
Underscore Js Sortby With Examples Geeksforgeeks
Sort Array Of Objects By Two Properties In Javascript By
How To Sort Array Arraylist String Map And Set In Java
How To Sort Arrays In Javascript
How To Sort Array By Groups With Javascript Stack Overflow
Javascript Array Sort The Specified Array Of Objects By
Powerful Js Reduce Function You Can Use It To Solve Any Data
Sort Array Of Objects By String Property Value In Javascript
How To Sort Alphabetically An Array Of Objects By Key In
Add Modify Verify And Sort Your Powershell Array
Sort Array Based On Conditions Using Javascript Sort Function
Sort Array Alphabetically Javascript Code Example
Top 50 Array Interview Questions Amp Answers 2021 Update
Javascript Array Sort How To Sort Array In Javascript
Search An Element In A Sorted And Infinite Array
Sort And Filter Dynamic Data In Table With Javascript By Ng
Javascript Array Sort How To Sort Array In Javascript
Javascript Sort Array By Object Property Code Example
Javascript Basic Sort The Strings Of A Given Array Of
Javascript Array Sort How Does Array Sort Work In Javascript
Use A Custom Sort Function On An Array In Javascript Egghead Io
Sorting Arrays In Javascript Array Prototype Sort Tutorial For Beginners
Javascript Sort The Items Of An Array W3resource
Solved Sorting In Array Solved Bugs Squidex Support
Js Sort An Array Of Objects On Multiple Columns Keys Dev
How Not To Sort An Array In Javascript Phil Nash
Sort An Array Of Objects With Javascript Array Prototype Sort
Indexed Collections Javascript Mdn
Javascript Sort Array Of Objects Code Example
Sort Javascript Object By Key Stack Overflow
How To Sort Array Of List Of Objects In Javascript Stack
0 Response to "33 Sort Array By Field Javascript"
Post a Comment