31 Check If Int Javascript
The Number.isInteger() method in JavaScript is used to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it returns false. Syntax: Number.isInteger(value) Parameters: This method accepts a single parameter value which specifies the number which the user wants to check for ... The parseInt function converts its first argument to a string, parses that string, then returns an integer or NaN. If not NaN, the return value will be the integer that is the first argument taken as a number in the specified radix.
Javascript Function Check A Number Is Prime Or Not W3resource
Javascript: Check if a string contains only digits without using Regex. In the below solution, we are using the function charCodeAt (index), which returns an integer representing the UTF-16 code value of the index passed. Function:-. function checkIfStringHasOnlyDigits(_string) {. for (let i = _string.length - 1; i >= 0; i--) {.
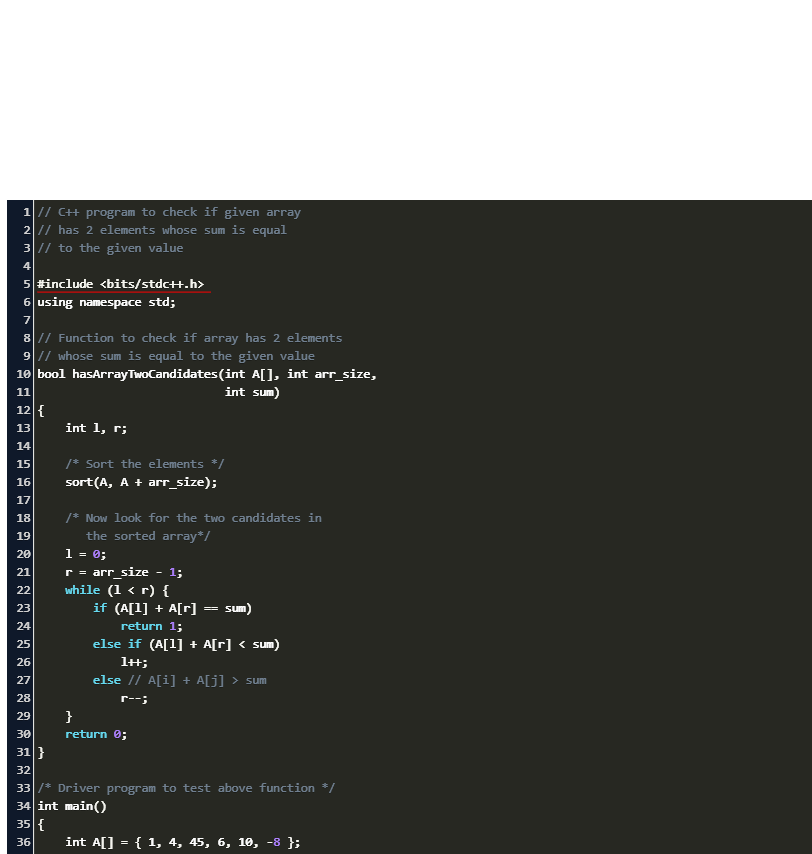
Check if int javascript. 17/1/2018 · The Number.isInteger() method in JavaScript is used to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it returns false. Syntax: Number.isInteger(value) Parameters: This method accepts a single parameter value which specifies the number which the user wants to check for integer. JavaScript Math: Exercise-15 with Solution. Write a JavaScript function to check whether a value is an integer or not. Test Data: console.log (is_Int (23)); console.log (is_Int (4e2)); console.log (is_Int (NaN)); Jun 04, 2020 - ActionScript queries related to “js check if string is integer” ... function that accepts a string and returns `true` if the string is a valid number, or `false` if it is not in javascript
In JavaScript, the best way to check for NaN is by checking for self-equality using either of the built-in equality operators, == or ===. Because NaN is not equal to itself, NaN != NaN will always... Definition and Usage The Number.isInteger () method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false. JavaScript isInteger () is an inbuilt method that resolves whether the passed value is an integer. The isInteger () method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise, it returns False. The isInteger () method is not supported in Internet Explorer 11 and earlier versions.
In JavaScript, there are two ways to check if a variable is a number : isNaN () - Stands for "is Not a Number", if variable is not a number, it return true, else return false. typeof - If variable is a number, it will returns a string named "number". Note. After some searches, I found that parseInt () and isNaN () can be used to do the job. The return value of text input field is string. First try parseInt () to convert the value to integer. If the type casting fails, it parseInt () will return NaN, so we can use isNaN () to check the result of conversion. If decimal part is greater than 0.5, then number is rounded to next integer which has greater absolute value. Absolute value refers to the magnitude value of the number regardless of its sign (for example 12 has greater absolute value than 11, -10 has greater absolute value than -9).
Jul 20, 2021 - A Boolean indicating whether or not the given value is an integer. ... If the target value is an integer, return true, otherwise return false. If the value is NaN or Infinity, return false. The method will also return true for floating point numbers that can be represented as integer. Front End Technology Javascript Object Oriented Programming To convert a string to an integer parseInt () function is used in javascript. parseInt () function returns Nan (not a number) when the string doesn't contain number. If a string with a number is sent then only that number will be returned as the output. Jan 23, 2016 - I mean, JavaScript already has the method to check for integerhood. No need to write a new one. isNaN() tests for numericity, not integerhood. ... @globewalldesk Maybe it answers the question "how to check if a number is integer" but not "if a variable is integer".
From time to time you have to check whether a variable is defined in JavaScript. For example, to determine if an external script has been successfully loaded into the web page, or to determine if the browser supports a Web API ( IntersectionObserver , Intl ). How to check if a value is a number in JavaScript How is it possible to determine if a variable value is a number? Published Jun 21, 2020. We have various ways to check if a value is a number. The first is isNaN(), a global variable, assigned to the window object in the browser: Jan 18, 2020 - JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... how to write a function that checks the number of strings in the string and returns it in javascript
javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". Javascript Web Development Front End Technology Object Oriented Programming. Let's say we have the following variables −. var value1 = 10; var value2 = 10.15; Use the Number () condition to check that a number is float or integer −. Number (value) === value && value % 1 !== 0; } Nov 25, 2009 - I’ve read that the == comparison ... to use === which will return true if both the left/right side are truly identical. ... Generally, it’s best practice to use === but in this example, value % 2 is guaranteed to return an integer or NaN, which allows us to absolutely know ...
Write a javascript function named is_integer which checks if the passed argument is an integer. You can use any mathematical operator or functions defined in the Math object. ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript ... In this chapter, you will learn about how to check variable is an integer or not in Javascript., here I am listing the possible ways to check if the value is an integer or not. Solution 1: Number.isInteger (): To check if the variable is an integer or not, use the method Number.isInteger (), which determines whether the passed value is an integer. Definition and Usage The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false.
NOTE: the above function uses WEAK VARIABLE TYPING - if you need to check is_int() using strong variable typing, you will need to use a different approach. Write a javascript function named is_integer which checks if the passed argument is an integer. You can use any mathematical operator or functions defined in the Math object. ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript ... In javaScript Number.isInteger () function to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it will return false. If you have any questions or thoughts to share, use the comment form below to reach us.
indexOf () Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf () method. This method searches the array for the given item and returns its index. If no item is found, it returns -1. Example 6: javascript check if a value is a integer number Learn, how to find if a given variable is a string or not in JavaScript. The typeof operator. We can use the typeof operator to check if a given variable is a string. The typeof operator returns the type of a given variable in string format. Here is an example:
The parseInt() function parses a string and then returns an integer. It returns NaN when it is unable to extract numbers from the string. For example, console.log(parseInt('195')) console.log(parseInt('boo')) Output: 195 NaN Use the Number() Function to Check Whether a Given String Is a Number or Not in JavaScript Output. Enter a number: 27 The number is odd. In the above program, number % 2 == 0 checks whether the number is even. If the remainder is 0, the number is even. In this case, 27 % 2 equals to 1. Hence, the number is odd. The above program can also be written using a ternary operator. May 26, 2014 - In the ECMAScript specification, ... JavaScript”). In this blog post, I explain how to check whether a value is an integer. ... There are many ways in which you could implement this check. At this moment, you may want to take a break and try to write your own solution: a function isInteger(x) that returns true if x is an integer ...
// program to check if a number is a float or integer value function checkNumber(x) { let regexPattern = /^-?[0-9]+$/; // check if the passed number is integer or float let result = regexPattern.test(x); if(result) { console.log(`${x} is an integer.`); } else { console.log(`${x} is a float value.`) } } checkNumber(44); checkNumber(-44); checkNumber(3.4); checkNumber(-3.4); javascript check if string is integerjavascript is floatjquery is integeris integer javajavascript check if number is decimalnumber.isfloat javascriptisintegercheck if number is integer javajavascript check if string contains only numbersjavascript check if string contains numbertypescript ... Nov 19, 2020 - It is useful to know how to check if a value is of the primitive type number, since JavaScript is a dynamically typed language. ... One example when a number might show up as another type, indicating a bug in my code, would be in parsing currency: In this case, I would know that I forgot to parse the string into ...
3/2/2018 · How to check if a variable is an integer in JavaScript? Javascript Web Development Front End Technology To check if a variable is an integer, check the value using parseInt () and check … javascript check if a value is an int; javascript check if number; javascript check if a value is a integer number; javascript check if variable is number; check if input is a number javascript; integer check in javascript; check if a string contains digits js; js is variable int; how to check if a string is an integer javascript; check if ... JavaScript exercises, practice and solution: Write a JavaScript program to check whether three given integer values are in the range 50..99 (inclusive). Return true if one or more of them are in the said range.
Sep 04, 2020 - JavaScript is a dynamically typed language, meaning that the interpreter determines the type of the variable at runtime. In practice, this allows us to use the same variable to store different types of data in the same code. It also means that without documentation and consistency, we do not ...
Javascript To Find Odd Or Even Number Tutorialsmade
Check For Pair In An Array With A Given Sum Interview Problem
Math Sign How To Check If A Number Is Positive Or Negative
Python Modulo In Practice How To Use The Operator Real
3 Ways To Check If A Variable Is Defined In Javascript
Java Program To Check Whether Number Is Divisible By 5 And 11
Check Number Integer Or Float In Java
Cognitive Complexity Vs Cyclomatic Complexity An Example
C Program To Check For Perfect Square C Programs Studytonight
4 Ways To Convert String To Character Array In Javascript
C Conditional Statement If If Else And Nested If Else With
How To Check If A String Contains At Least One Number Using
Python Program To Check A Number Or String Is Palindrome
Check If The Sum Of 2 Numbers Is Equal To The Input
Javascript Check If Empty Code Example
How To Check If Two Strings Have Same Characters Javascript
How To Draw A Flowchart To Check If A Number Is Positive Or
2 Ways To Convert Values To Boolean In Javascript
Working With Javascript In Visual Studio Code
How To Check If Array Includes A Value In Javascript
3 Ways To Check If Variable Is A Number In Javascript Dev
Parseint Check For Nan Isn T Working Properly Stack Overflow
How To Check If A Javascript Object Property Is Undefined
C Program To Check The Number Is Divisible By 5 And 11
Javascript Code To Check Number Is Armstrong Or Not
Python Program To Separate Even And Odd Numbers In Array
Marcus Mengs On Twitter Adding The Realopen Declaration
The 10 Most Common Mistakes Javascript Developers Make Toptal
How To Draw A Flowchart To Check If A Number Is Positive Or
Javascript Program To Check If A Number Is Multiple Of 3
0 Response to "31 Check If Int Javascript"
Post a Comment