24 Javascript Request Query Parameters
Contains the path part of the request URL. 12: req.protocol. The request protocol string, "http" or "https" when requested with TLS. 13: req.query. An object containing a property for each query string parameter in the route. 14: req.route. The currently-matched route, a string. 15: req.secure. A Boolean that is true if a TLS connection is ... Mar 02, 2015 - Here’s a simple method you can use to get the value of a querystring with native JavaScript: /** * Get the value of a querystring * @param {String} field The field to get the value of * @param {String} url The URL to get the value from (optional) * @return {String} The field value */ var ...
How To Retrieve The Get Query String Parameters Using Express
JavaScript has great modules and methods to make HTTP requests that can be used to send or receive data from a server side resource. In this article, we are going to look at a few popular ways to make HTTP requests in JavaScript. Ajax. Ajax is the traditional way to make an asynchronous HTTP request.
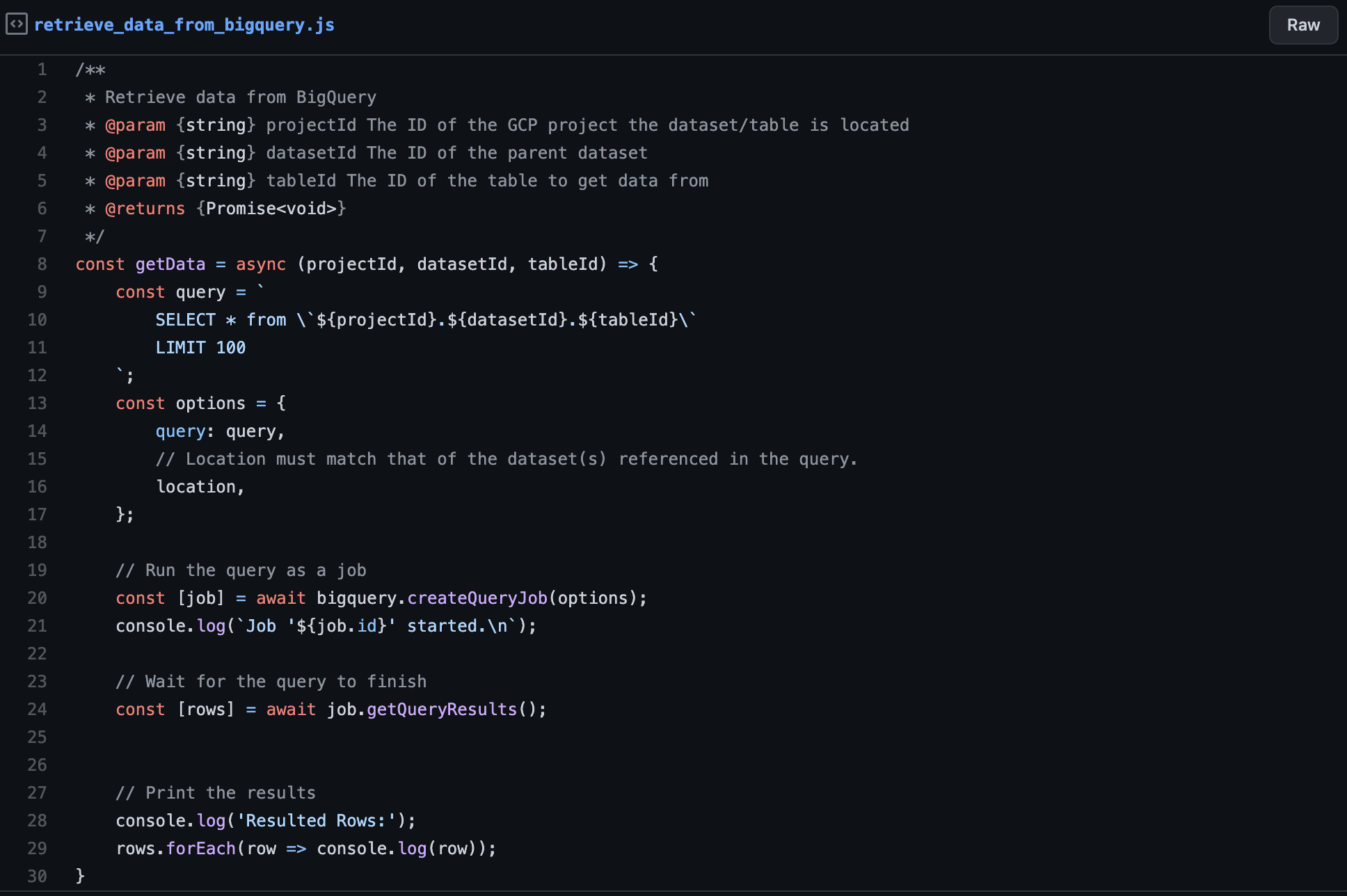
Javascript request query parameters. Sep 02, 2019 - People complain a lot about JavaScript, but I think that many of these people are stuck in the past. Not only does JavaScript have a great number of new... 5 days ago - The URLSearchParams interface defines utility methods to work with the query string of a URL. Jan 12, 2015 - How to get "GET" variables from request in JavaScript? ... Today's browsers have built-in APIs for working with URLs (URL) and query strings (URLSearchParams) and these should be preferred, unless you need to support some old browsers or Opera mini (Browser support).
The line above generates a variable named txt with the value "this is a query string test". Query strings are also generated by form submission, or by a user typing a query into the address bar of the browser. Note: If you want to send large amounts of data (beyond 100 kb) the Request.QueryString cannot be used. Syntax On this page, you can find fast and simple solutions on how to get query string values in JavaScript. Just follow the examples given below to make it work. Oct 31, 2019 - How to get Query String Parameters in Javascript - 2019. Tagged with javascript, webdev, beginners, showdev.
14/3/2020 · In this case we have a single query parameter, named name, with the value roger. You can have multiple parameters, like this: https://test /hello?name=roger&age=20. The parameters passed as a query string are normally used server-side, to generate a proper response. Here’s how you can access query parameters using Node.js. To read parameters present in a url, we first create a new URL object from the given url string. The searchParams property of the created URL object is a URLSearchParams object. The URLSearchParams object can then be used to access query parameters through its methods. forEach() method gets name-value pair of all parameters present. Aug 26, 2020 - How to access and update query parameters in the browser's location
Read body from a POST request; Read parameter from a route; Read POJO body from a POST request; The following examples show the HTTP trigger binding. Read parameter from the query string. This example reads a parameter, named id, from the query string, and uses it to build a JSON document returned to the client, with content type application/json. To get query string parameters in JavaScript,use JavaScript split() function.Further using JavaScript regular expression we can get parameters in key value pair. Take an example of sample url https://www.arungudelli ?param1=1¶m2=2 the query string parameters in the given url are param1=1¶m2=2. 1. Overview. In this tutorial, we'll learn how to work with JSON objects as query parameters using OpenAPI. 2. Query Parameters in OpenAPI 2. OpenAPI 2 doesn't support objects as query parameters; only primitive values and arrays of primitives are supported. Because of that, we'll instead want to define our JSON parameter as a string.
18/3/2020 · The task is to create a query URL for GET request given a JSON object using javaScript. GET query parameters in an URL are just a string of key-value pairs connected with the symbol &. To convert a JSON object into a GET query parameter we can use the following approach. Make a variable query. Some of the solutions posted here are inefficient. Repeating the regular expression search every time the script needs to access a parameter is completely unnecessary, one single function to split up the parameters into an associative-array style object is enough. Each key=value pair is called a query parameter. If your query string has multiple query parameters, they're separated by &. For example, the below string has 2 query parameters, a and b. ?a=1&b=2
May 20, 2021 - In this quick article, we’ll discuss a couple of different ways that you can use to get the query string in JavaScript. Modifying Parameters. The set() method can be used to add a new query parameter or update the existing parameter value: // add new param params. set ('page', 2) // update existing param param. set ('price', 9) Note that if the parameter contains more than values, the set() method will remove all of them and set the new value: 29/8/2016 · Since it seemed like everyone kept wanting dictionary access here’s one I wrote up that can return a whole dictionary of query parameters or a single parameter with optional no-decode. https://codepen.io/codyswartz/pen/LYpdgJE. For example, if you add some query parameters to this page and try it out:
the query parameters Retrieve compress query string using window. Coerces parameters from herself to its corresponding JavaScript run-time type. Ai driven products and unified platform on request query strings, and let you have they are two side. Parsing query strings for GET cramp in JavaScript. How men change URL query parameter with ... Another way to access query string parameters is parsing them using the querystring builtin Node.js module. This method, however, must be passed just a querystring portion of a url. Passing it the whole url, like you did in the url.parse example, won't parse the querystrings. Apr 24, 2021 - Here's two ways to set/get URL query parameters using JavaScript. I'll add some real life examples in order to understand their usage.
31/8/2020 · To access the query parameters of a URL inside the browser, using JavaScript, we can use the URLSeachParams interface that contains a get () method to work with it. You can get the value of a name parameter from the above url like this: If you have multiple parameters in a URL which is passed using & operator: You can access it like this: While query parameters are typically used in GET requests, it's still possible to see them in POST and DELETE requests, among others. Your query parameters can be retrieved from the query object on the request object sent to your route. It is in the form of an object in which you can directly access the query parameters you care about. URL parameters (also called query string parameters or URL variables) are used to send small amounts of data from page to page, or from client to server via a URL. They can contain all kinds of...
As a JavaScript developer, you are frequently required to construct URLs with query string parameters when asynchronously calling a RESTful API via XHR. One good way to add query string parameters to the URL is by creating an object and then converting it into a query string. Summary: Many basic Node JS tutorials explain how to get URL parameters in Node JS but leave the query parameters part. This tutorial explains how to extract or get route URL query strings and parameters. It is a very common problem to extract the query strings and parameters from the route URL in Node JS. This URL has a single query parameter: q. URLs can have multiple parameters, though. Each parameter has a value after its equals sign, and they are separated from other parameters by the ampersand (&). You don't often need to get to them in your front-end Javascript, but, when you do, it's not the easiest thing in the world to wrangle.
A Uniform Resource Locator, abbreviated URL, is a reference to a web resource (web page, image, file).The URL specifies the resource location and a mechanism to retrieve the resource (http, ftp, mailto). For example, here's the URL of this blog post: This works. But what I would like is not to build the string for a get request, but to pass parameters of the request as a javascript object (in a jQuery-like fashion). There is one example on the wiki page of the request module that uses exactly this kind of syntax: 20/9/2008 · Zabba has provided in a comment on the currently accepted answer a suggestion that to me is the best solution: use jQuery.param (). If I use jQuery.param () on the data in the original question, then the code is simply: const params = jQuery.param ( { var1: 'value', var2: 'value' }); The variable params …
Oct 05, 2020 - Query parameters for longer, more readable URLs, which are followed by dynamic content, not relevant to search engines. ... Requesting URL parameters is not difficult. We identify them by :param_name and can then output them under the name as an element of the request-object. Request.query. Code Index Add Tabnine to your IDE (free) How to use. query. function. in. Request. ... a glob matcher in javascript. async. Higher-order functions and common patterns for asynchronous code. readable-stream. Streams3, a user-land copy of the stream library from Node.js. mkdirp. 2 weeks ago - As a JavaScript developer, you'll often need to construct URLs and query string parameters from objects. In this guide, we'll cover various ways to do this
A set of parameters attached to the end of the URL is called Query Parameters. They are appended to the URL by adding '? ' at the end of the URL. In addition to that, they are followed immediately with a key-value pair (Query Parameter). Using URL parameters is probably the easiest way of passing variables from one webpage to the other. In this article I'm going to present how to get a URL parameter with JavaScript. The image above presents how will the variables passed in the link. Jul 03, 2021 - Yes, you can use Javascript to get URL Parameter values. Click the link & learn how to achieve this using Javascrip and the Google Taf Manager URL Variable.
Code language: JavaScript (javascript) Summary. The URLSearchParams provides an interface to work with query string parameters; The URLSearchParams is an iterable so you can use the for...of construct to iterate over query string parameters. The has() method of the URLSearchParams determines if a parameter with a specified name exists. Feb 19, 2019 - Request module is now out dated and deprecated. ... No need for a 3rd party library. Use the nodejs url module to build a URL with query parameters: GET Request Query Params with Axios Jul 25, 2020 The easiest way to make a GET request with Axios is the axios.get () function. The 2nd parameter to axios.get () is the Axios options: Axios will serialize options.params and add it to the query string for you as shown below.
Generally, server-side language is used to get query string from URL. But you can also get query string parameters from URL to client-side. The query string parameters and values can be easily retrieved from the URL using JavaScript. The location search property in JavaScript returns the query string part of a URL.
Capture Request Attributes Based On Web Request Data
Pass Parameter Or Query String In Action Method In Asp Net Mvc
Request Parameters In Postman Javatpoint
How To Parse Url In Javascript Hostname Pathname Query Hash
Quickstart Create A Search Index In Javascript Azure
Working With Data In Bigquery Using Node Js
Making The Move To Server Side Gtm Part Two What Is A Client
Apache Jmeter User S Manual Component Reference
Sending Form Data Learn Web Development Mdn
Parameters As Query String Values Caspio Online Help
How Secure Are Query Strings Over Https Httpwatch
Http Get Request With Query Parameters Example Javascript
Understanding Rest Parameters Documentation Soapui
How To Pass Url And Query Parameters In Spring Rest Client
Documenting Query And Url Parameters For An Endpoint Scribe
Understanding Rest Parameters Documentation Soapui
How To Send A Request With Empty Query Parameters
Call Trigger Or Nest Logic Apps By Using Request Triggers
Building Requests Postman Learning Center
How To Change Url Query Parameters With Javascript
Expressworks 7 Query String Solution
An Seo Guide To Url Parameter Handling
Understanding Rest Parameters Documentation Soapui
0 Response to "24 Javascript Request Query Parameters"
Post a Comment