34 How To Write Javascript Code In Java Class
Java lets us separate the program into multiple files, each containing code for a different piece of the project. Classes in Java A class is a template, or a blueprint, from which Java objects are created. JSweet is an open transpiler from Java to TypeScript/JavaScript with more than 1000 well-typed JavaScript libraries.
Java Class Tools Read And Write Java Class Files In Node Js
Javadoc is a tool which comes with JDK and it is used for generating Java code documentation in HTML format from Java source code, which requires documentation in a predefined format. Following is a simple example where the lines inside /*….*/ are Java multi-line comments. Similarly, the line which preceeds // is Java single-line comment. Example
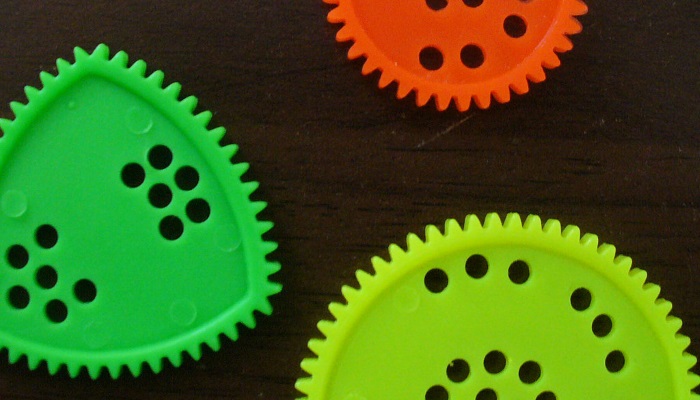
How to write javascript code in java class. Using Multiple Classes. You can also create an object of a class and access it in another class. This is often used for better organization of classes (one class has all the attributes and methods, while the other class holds the main() method (code to be executed)).. Remember that the name of the java file should match the class name. Java has grown to become one of the most popular programming languages in the world.It is versatile, relatively easy to use, and has a wide range of use cases.This makes it a great language to learn - for beginner and experienced programmers alike - and has resulted in a large number of people asking how to code in Java.. The purpose of this tutorial is to provide an introduction to Java ... There are following three ways in which users can add JavaScript to HTML pages. Embedding code; Inline code; External file; We will see three of them step by step. I. Embedding code:-To add the JavaScript code into the HTML pages, we can use the <script>.....</script> tag of the HTML
Java Inheritance (Subclass and Superclass) In Java, it is possible to inherit attributes and methods from one class to another. We group the "inheritance concept" into two categories: subclass (child) - the class that inherits from another class. superclass (parent) - the class being inherited from. To inherit from a class, use the extends keyword. Hence write your function between 10-15 lines focusing onto a single thing that the method does. 3. Be smart to reduce lines of code : Always try to think before even you start writing any piece ... Getting Started with Java in VS Code. This tutorial shows you how to write and run Hello World program in Java with Visual Studio Code. It also covers a few advanced features, which you can explore by reading other documents in this section. For an overview of the features available for Java in VS Code, see Java Language Overview.
If you use the scripting API to run JavaScript code from your servlet, then it's going to run on the server, and not the client. If your goal is to display a message box on the client's computer, then trying to run JavaScript code on the server doesn't make sense. This was the first simple JavaScript unit test from start to end. If you installed the Visual Studio Code extension, it will run tests automatically once you save a file. Let's try it by extending the test with this line: expect (getAboutUsLink ("cs-CZ")).toBe ("/o-nas"); You can create a Java object and assign it to a variable in JavaScript with the help of the new keyword. When you create an instance of a Java class, JavaScript automatically creates a JavaObject...
Nov 09, 2011 - This site is best viewed in a modern browser with JavaScript enabled. Something went wrong while trying to load the full version of this site. Try hard-refreshing this page to fix the error. ... Can you place java code in an external javascript file (.js). For example can I do the following ... : Progress OpenEdge is the complete development platform for building dynamic multi-language applications for secure deployment across any platform, any mobile device, and any Cloud. 1. Let us say your jsfunctions.js file has a function "display" and this file is stored in C:/Scripts/Jsfunctions.js. jsfunctions.js. var display = function (name) { print ("Hello, I am a Javascript display function",name); return "display function return" } Now, in your java code, I would recommend you to use Java8 Nashorn. In your java class,
In fact, the Javascript code on the browser will look like this: function getScreenDimension() { } Which is not valid Javascript code. The code in the JSP is run before the Javascript gets run on the browser. So to "run" Java code, you need to make a request to your server either by posting the form or with an AJAX call. Syntactically and semantically, this distinction between compile-time class expressions and runtime class objects makes JavaScript similar to Java code. However, there is no equivalent of the static property for a Class object in Java, because compile-time class expressions are never expressed ... Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically embedded into Java applications to provide scripting to end users. Update: Now Nashorn, which is more performant JavaScript Engine for Java, is available with jdk8.
Apr 20, 2009 - Most Java developers and JavaScript ... JavaScript and Java have very little in common other than some C-like heritage. Still, it can be useful at times to run a scripting language from within Java code and Java SE 6 allows this. The javax.script package was introduced with Java SE 6 and includes classes, interfaces, ... To write a JavaScript, you need a web browser and either a text editor or an HTML editor. Once you have the software in place, you can begin writing JavaScript code. To add JavaScript code to an HTML file, create or open an HTML file with your text/HTML editor. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Read on for some essential pointers that can help you write cleaner, more maintainable Java code. Keep classes small. So far you've created a few classes. After generating getter/setter pairs for even the small number (by the standards of a real-world Java class) of attributes, the Person class has 150 lines of code. Define a class in Javascript One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code.
Learn JavaScript and Javascript arrays to build interactive websites and pages that adapt to every device. Add dynamic behavior, store information, and handle requests and responses. This course can help marketers and designers upgrade their career and is a starting point for front-end engineers. In my next article, I'll talk about another fundamental building block of good JavaScript, which is MVC, Angular, TDD etc. TypeScript is the best way to write Angular 2 code. In fact, the alternative of writing it in plain JavaScript is so bad, I'd argue that it's the only way to write Angular 2 code. That and more in my next article. I managed to change that by writing my own CSS preprocessor. I stopped writing CSS and moved everything into the JavaScript world. This article is about AbsurdJS: a small Node.js module, which changed my workflow completely. The Concept. If you write a lot of CSS you probably use preprocessor. There are two popular - LESS and SASS.
JavaScript provides 3 places to put the JavaScript code: within body tag, within head tag and external JavaScript file. Let's create the first JavaScript example. Java Inner Classes. In Java, it is also possible to nest classes (a class within a class). The purpose of nested classes is to group classes that belong together, which makes your code more readable and maintainable. To access the inner class, create an object of the outer class, and then create an object of the inner class: A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {}
How to write a function in JavaScript. A JavaScript function is a block of code that consists of a set of instructions to perform a specific task. A function can also be considered as a piece of code that can be used over again and again in the whole program, and it also avoids rewriting the same code. Tip: To check if a JavaScript file is part of JavaScript project, just open the file in VS Code and run the JavaScript: Go to Project Configuration command. This command opens the jsconfig.json that references the JavaScript file. A notification is shown if the file is not part of any jsconfig.json project. Java User Input. The Scanner class is used to get user input, and it is found in the java.util package.. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read Strings:
Jan 30, 2018 - JavaScript cannot call java method directly since it is on the server. You need a Java framework like JSP to call when a request is received from JavaScript. Le ... Learn Python Learn Java Learn C++ Learn C# Learn R Learn Kotlin Learn Go. Server Side ... JavaScript Classes are templates for JavaScript Objects. JavaScript Class Syntax. Use the keyword class to create a class. Always add a method named constructor(): ... CODE GAME Play Game. Aug 26, 2017 - I am struggling to convert Java code into javascript. For that, for example, I am converting public static int primesolution into function primesolution. I do not have much idea whether I am on the...
Using classes and functions to wrap up code is basic modularity; Previewer: Writing my first JavaScript library. A image previewer for the web, which uses a Picasa inspired UI and is super light-weight. Getting rid of dependencies. Initially, I wrote the code heavily relying on jQuery selectors and functions cause I found them simplifying my ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Java Programming Forum - Learning Java easily
Oct 12, 2016 - I don't know how to call that java code from javascript - when clicking on the button. I have the code of calling the jar - it is java - code, but.
Spring Mvc How To Include Js Or Css Files In A Jsp Page
Javascript Programming With Visual Studio Code
Object Oriented Programming In Javascript Explained With
Object Oriented Javascript For Beginners Learn Web
Javascript Programming With Visual Studio Code
Scripting In Java Tutorial Call Javascript Function
Javascript Api Frida A World Class Dynamic
How To Pass Variable Values From Java To Javascript Stack
7 Concepts Of Object Oriented Javascript You Need To Know
Javascript Where To Write Javascript Code
Where To Write Javascript Where To Execute Javascript Code
Javascript Api Frida A World Class Dynamic
Navigate And Edit Java Source Code In Visual Studio Code
Javascript On Netbeans Scarce Code Support Features Stack
Add Javascript Scripting To Your Java Application Novixys
Java Script An Overview Sciencedirect Topics
Print Log To Javascript Console And Commenting Out Codes
Add Javascript Scripting To Your Java Application Novixys
Beta Basic Javascript Mistake In Example Code Issue
1 Writing Your First Javascript Program Javascript
A Main Class In Java Contains The Main Method
Hot Update Adding Your First Line Of Java Code
Abstract Classes In Javascript What Are Abstract Classes
The Complete Guide To Javascript Classes
Making Sense Of Es6 Class Confusion Toptal
Javascriptexecutor In Selenium Webdriver With Example
5 Tips To Organize Your Javascript Code Without A Framework
Inserting Custom Javascript Codes Wordpress Websites For
Java Script Functions Hi How Are You People Hope
Calling Java From Javascript And Vice Versa Why Stack Overflow
How To Write Javascript Code In Java Class
0 Response to "34 How To Write Javascript Code In Java Class"
Post a Comment