21 Convert Text To Date In Javascript
24/3/2015 · Date Input - Parsing Dates. If you have a valid date string, you can use the Date.parse() method to convert it to milliseconds. Date.parse() returns the number of milliseconds between the date and January 1, 1970: The task is to convert a JSON result to JavaScript Date with the help of JavaScript. There are few two methods which are discussed below: Approach 1: Use substr() method to get the integer part of string. Use the parseInt() method followed by Date() to get the JavaScript date. Example: This example implements the above approach.
Date Manipulation In Javascript A Complete Guide
The new Date () gives the current date and time. const d1 = new Date(); console.log (d1); // Mon Nov 09 2020 10:52:32 GMT+0545 (Nepal Time) To convert the name to a number, we use the getTime () method. The getTime () method returns the number of milliseconds from midnight of January 1, 1970 (EcmaScript epoch) to the specified date.
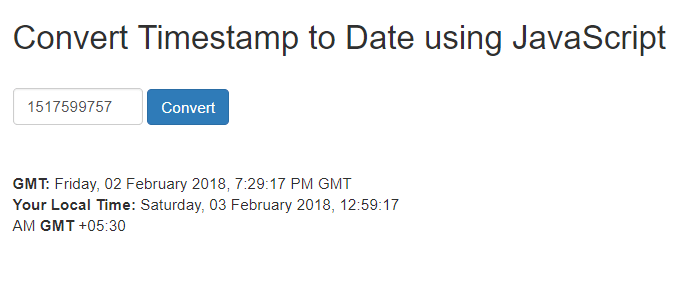
Convert text to date in javascript. The Javascript date can be converted to UTC by using functions present in Javascript Date object. The toUTCString () method is used to convert a Date object into a string, according to universal time. The toGMTString () returns a string which represents the Date based on the GMT (UT) time zone. By default, JavaScript uses the browser's time ... Below is the example of Date toISOString () method. The date.toISOString () method is used to convert the given date object's contents into a string in ISO format (ISO 8601) i.e, in the form of (YYYY-MM-DDTHH:mm:ss.sssZ or ±YYYYYY-MM-DDTHH:mm:ss.sssZ).The date object is created using date () constructor. The .NET JSON date format part contains two parts - i.e., /Date(Milliseconds + Timezone offset)/. Converting JSON Date string to Local Date: If you are interested in just the Date part - Milliseconds and doesn't care about the timezone offset, then you can use following simple script to convert as JavaScript Date;
You can convert the date string to the date object and then you extract the month as a number, zero based value starting with January as 0, or use the "util.printd" method to create the formatted output for the date. var cDateField = "WkEnd"; // name of date field JavaScript Date Time Format. The above shown date time is the default format. It's not so straight to change JavaScript date time format. Change Date Format dd-mmm-yyyy. To convert date to format dd-mmm-yyyy you need to extract the date, month and year from the date object. 10/8/2019 · Converting a string to a date in JavaScript Javascript Front End Technology Object Oriented Programming The best format to represent a date is yyyy-mm-dd as this causes no confusion and is pretty straight forward. In order to parse dates from this string format to Date objects in JS, all you need to do is pass this string to the Date constructor.
Converting a string in ANSI/ISO and US date format to a datetime Both CONVERT () and TRY_CONVERT () function can recognize ANSI/ISO and US formats with various delimiters by default so you don't have to add the style parameter. This example shows how to use the CONVERT () function to convert strings in ISO date format to datetime values: JavaScript only has a Date object, which is misnamed since it is really a date+time. Which means it cannot parse a time string HH:mm:ss without a date, but it can parse a date string. There are a few options. Convert time to a datetime string and parse using Date (). Use Momentjs String + Format parsing function. 1. How to Convert a String into Point Number¶. Use parseFloat() function, which purses a string and returns a floating point number. The argument of parseFloat must be a string or a string expression. The result of parseFloat is the number whose decimal representation was contained in that string (or the number found at the beginning of the string).
Convert time into date object and store it into new variable date. Convert the date object's contents into a string using date.toString() function. Example 1: This example first gets the milliseconds of the current date and time, Then using that value to get the date by Date() method. How can I convert a string to a date in JavaScript? var st = "date in some format" var dt = new date(); var dt_st= //st in date format same as dt ... If you need to check the contents of the string before converting to Date format: ... How to deal with a boss who keeps changing his mind and doesn't reply to text/calls but when I get stuck due ... How to Convert a String into a Date in JavaScript The best format for string parsing is the date ISO format with the JavaScript Date object constructor. But strings are sometimes parsed as UTC and sometimes as local time, which is based on browser vendor and version. It is recommended is to store dates as UTC and make computations as UTC.
To increment a date in javascript, we're going to discuss few techniques. First few methods to know: JavaScript getDate () method. This method returns the day of the month (from 1 to 31) for the defined date. Syntax: Date.getDate () Return value: It returns a number, from 1 to 31, denoting the day of the month. JavaScript setDate () method. The standard string representation of a date time string is a simplification of the ISO 8601 calendar date extended format. (See the section Date Time String Format in the ECMAScript specification for more details.). For example, "2011-10-10" (date-only form), "2011-10-10T14:48:00" (date-time form), or "2011-10-10T14:48:00.000+09:00" (date-time form with milliseconds and time zone) can be ... 19/11/2011 · CodeProject, 20 Bay Street, 11th Floor Toronto, Ontario, Canada M5J 2N8 +1 (416) 849-8900
A Typescript function to convert an excel serial date to a js date is. public static SerialDateToJSDate (excelSerialDate: number): Date { return new Date (Date.UTC (0, 0, excelSerialDate - 1)); } And to extract the UTC date to use. Where Date is called as a constructor with more than one argument, if values are greater than their logical range (e.g. 13 is provided as the month value or 70 for the minute value), the adjacent value will be adjusted. E.g. new Date(2013, 13, 1) is equivalent to new Date(2014, 1, 1). The global method Number () can be used to convert dates to numbers. d = new Date (); Number (d) // returns 1404568027739 The date method getTime () does the same.
20/6/2020 · Now, we need to convert the above date string to an actual date object with JavaScript. Note: The date string should be in ISO format (YYYY-MM-DD or MM/DD/YYYY or YYYY-MM-DDTHH:MM:SSZ) Using new Date() constructor. The new Date() constructor takes the date string as an argument and creates the new date object. Example: JavaScript does not have a date data type. However, you can use the Date object and its methods to work with dates and times in your applications. The Date object has a large number of methods for setting, getting, and manipulating dates. It does not have any properties. 26/4/2021 · In LWC Instead converting from datettime to date why can't we convert our date to datetime using default JS functionalites like, (var newStartDate = new Date(this.newStartdatefield). So now we can do all our computations easily
In SQL Server, converting string to date implicitly depends on the string date format and the default language settings (regional settings); If the date stored within a string is in ISO formats: yyyyMMdd or yyyy-MM-ddTHH:mm:ss(.mmm), it can be converted regardless of the regional settings, else the date must have a supported format or it will throw an exception, as an example while working ... Definition and Usage. The toString () method converts a Date object to a string. Note: This method is automatically called by JavaScript whenever a Date object needs to be displayed as a string. How to convert a date in format 23/10/2015 into a JavaScript Date format: Fri Oct 23 2015 15:24:53 GMT+0530 (India Standard Time)
Date objects don't have a timezone, they are just an offset from 1970-01-01T00:00:00Z. So when you call toDate, the returned object has lost the timezone information you attached to the moment object with .tz("MST7MDT").That's why libraries like moment.tz exist, to supplement the bare bones capability of the built-in Date object which only understands UTC and the host timezone, that's it. How to get the Highlighted/Selected text in JavaScript? Next. Django - Dealing with warnings. Recommended Articles. ... How to convert a JavaScript date to UTC? 18, Apr 19. How to convert date to another timezone in JavaScript ? 19, Nov 19. How to Convert CFAbsoluteTime to Date Object and vice-versa in JavaScript ? Use the Date.parse () Function to Convert String to Date in JavaScript Date.parse () is an alternate option to convert the string date. It returns a numeric value instead of a date object. Hence it will require further processing if you expect a date object.
Javascript Date Formatting Net Style Waldek Mastykarz
How Do I Convert A Firestore Date Timestamp To A Js Date
Javascript Date Format Function Convert Datetime Like Php
How To Convert Text Dates In Excel Displayr
How To Convert Timestamps To Human Readable Dates In
Date Validation As Text Format In Javascript
Convert Custom Date Format Lt Day Gt Lt Hour Gt Lt Minute Gt Lt Second Gt To
Build Timestamp Converter To Date With Javascript Coders Zine
Everything You Need To Know About Date In Javascript Css Tricks
How To Get Current Formatted Date Dd Mm Yyyy In Javascript
Converting A String To A Date In Javascript Stack Overflow
Sql Convert Date Functions And Formats
How To Convert Text Dates In Excel Displayr
Javascript Date Formatting Net Style Waldek Mastykarz
Convert Mysql Data Type Datetime To Javascript With Php
Js Date Format Amp Validate Library Moment Js Free Jquery
Javascript Convert Date Time String To Epoch Stack Overflow
Java Convert Date To Timestamp Javatpoint
How To Convert Date To Iso String In Javascript Code Example
3 Ways Of How To Change Date Format In Excel 14 Date Formulas
0 Response to "21 Convert Text To Date In Javascript"
Post a Comment