29 How To Trim Whitespace In Javascript
This JavaScript tutorial explains how to use the string method called trim() with syntax and examples. In JavaScript, trim() is a string method that is used to remove whitespace characters from the start and end of a string. Whitespace characters include spaces, tabs, etc. If you want to to implement something like ruby's string.strip method, which returns returns a copy of the string with leading and trailing whitespace removed, this should work: x = " Many spaces before and after "y = x.replace(/(^\s+|\s+$)/g, "") The ^\smeans whitespace after the beginning of the string, \s$ means whitespace at the end of the string, the | is for either / or in the in the ...
str.trim()only trims the trailing spaces at the beginning and end. The other solution strips out all the spaces from everywhere in the string. - Cute_NinjaJul 30 '18 at 21:16 Ah, so silly of me!
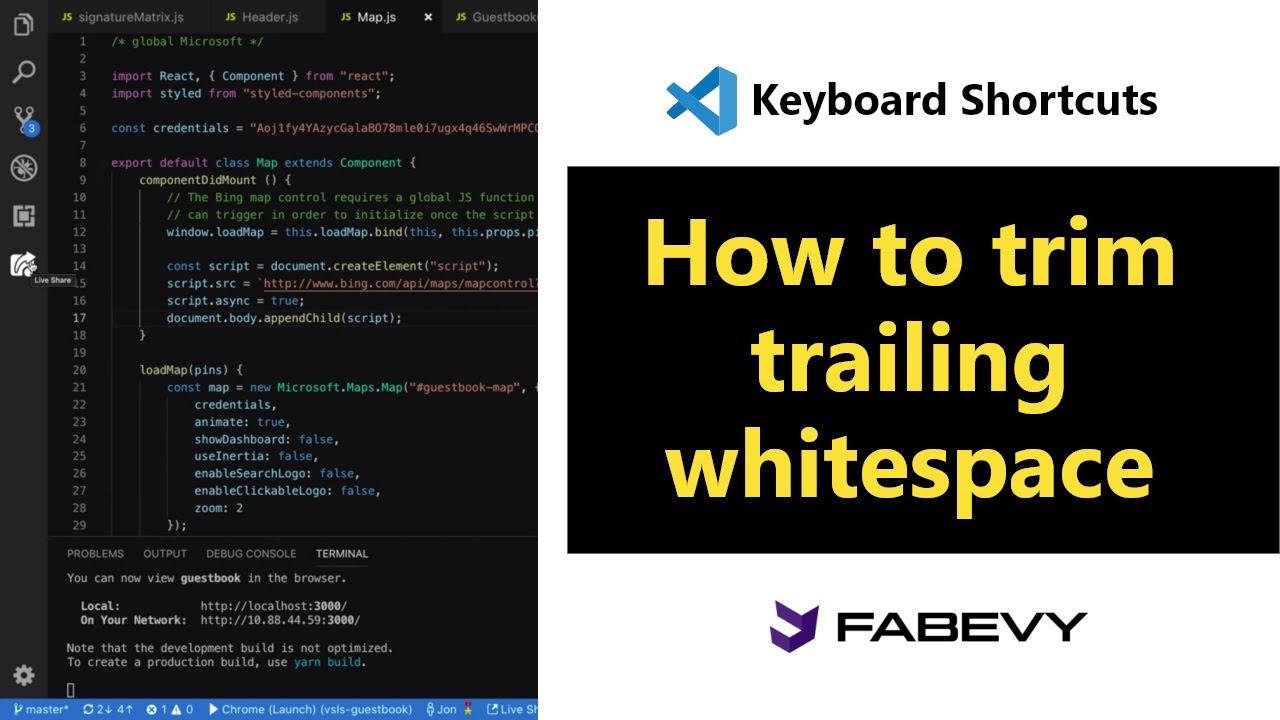
How to trim whitespace in javascript. Use a replace () and trim () method to Remove whitespace of string in JavaScript (). The trim () method do not support in browsers, you can remove whitespaces from both sides of a string or between words using replace () method with a regular expression: Methods in JS to remove string whitespace replace () - Used with regular expression. Description: Remove the whitespace from the beginning and end of a string. ... The string to trim. 1. Remove extra white spaces with StringUtils. This approach, using StringUtils.normalizeSpace () is most readable and it should be preferred way to remove unwanted white spaces between words. This function returns the argument string with whitespace normalized by -. using trim (String) to remove leading and trailing whitespace, and then.
JavaScript trim () method. The javascript String.trim () is a string method that is used to remove whitespace characters from the beginning and from the end of a string. 1. let res = str.trim (); Here, The trim () method doesn't change the original string. if you want to remove only starting whitespace or ending whitespace from the given ... So trim removes whitespace. So that is the white space created by: ... In JavaScript, you can easily create multi-line strings using the Template Literals. So if you're wondering if trim works with that, you bet it does 👍 Jan 14, 2020 - Remove all the whitespaces and find it's length in javascript ... Removing whitespace from strings. js ... remove the whitespace from the beginning and end of the string and make the string lowercase. nodejs ... // How to create string with multiple spaces in JavaScript var a = 'something' ...
Apr 01, 2020 - Today, we’re going to look at a few different ways to remove whitespace from the start or end of a string with vanilla JavaScript. Let’s dig in. The String.trim() method You can call the trim() method on your string to remove whitespace from the beginning and end of it. 3 weeks ago - The trim() method removes whitespace from both ends of a string. Whitespace in this context is all the whitespace characters (space, tab, no-break space, etc.) and all the line terminator characters (LF, CR, etc.). The whitespace characters are space, tab, no-break space, etc. Note that the trim () method doesn't change the original string. To remove whitespace characters from the beginning or from the end of a string only, you use the trimStart () or trimEnd () method. JavaScript trim () example
JavaScript String trim() The trim() is a built-in string function in JavaScript, which is used to trim a string. This function removes the whitespace from both the ends, i.e., start and end of the string. As the trim() is a string method, so it is invoked by an instance of the String class. Jun 22, 2017 - The trim() method is used to remove white space and from both ends of a string (white space meaning characters like space, tab, no-break space and so on). It also removes line terminator characters… String replacements in JavaScript are a common task. It still can be tricky to replace all appearances using the string.replace() function. Removing all whitespace can be cumbersome when it comes to tabs and line breaks. Luckily, JavaScript's string.replace() method supports regular expressions. This tutorial shows you how to remove all ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Trim. Trim is nothing but removing, and it is a string method which is used to remove the white space from both the sides of a string. White space is nothing but the space, tab, no-break space, etc.., This method does not affect to the value of a string. Trim Functions. The concept Trim produces three functions/methods in JavaScript, they are ... The left trim should remove all leading whitespace characters, and the right trim should remove all trailing whitespace characters from a string. 1. Using JavaScript native methods. JavaScript provides a native trimStart() method, which can be used to remove whitespace from
# Using String.prototype.trimEnd () Introduced in ES10, you can use the String.prototype.trimEnd () method to remove whitespace characters from the end of a string. Please note that the String.prototype.trimEnd () method returns a new string with stripped out whitespace characters from the right-most side of a given string. Mar 22, 2021 - When you need to strip whitespace from a JavaScript string, you can use the String.trim() method, which allows you to remove whitespaces before and after the string. Apr 10, 2020 - Connect and share knowledge within a single location that is structured and easy to search. ... I've read this question about javascript trim, with a regex answer.
Trim white space means removing white spaces from the text input from both start and end. The following example shows how to trim white space from text input in ReactJS. Creating React Application And Installing Module: Step 1: Create a React application using the following command: npx create-react-app foldername The trim() method removes whitespace from both ends of a string. Whitespace in this context is all the whitespace characters (space, tab, no-break space, etc.) and all the line terminator characters (LF, CR, etc.). Jul 31, 2018 - @0x48piraj you misread OP question. he wanted to strip ALL whitespace – Andre Figueiredo May 11 at 15:38 ... Not the answer you're looking for? Browse other questions tagged javascript string trim or ask your own question.
Therefore, we should trim them before doing anything with them. In this article, we'll look at how to trim strings in JavaScript. What are Whitespaces? White spaces include the space, tab, no-break space, and line end characters. They are all trimmed by the JavaScript string methods that trim whitespaces. trimStart. String instances come with the In JavaScript we can trim the whitespace from the string just by calling the String.prototype.trim function which will do this for us! Here is how it looks when using trim to remove the whitespace from the beginning and end of a string in JavaScript: The JavaScript trim function is used to remove whitespace or blank space from inside any string. The whitespace can be removed from the front, back, and middle of the string. For this, you will have to use the trim function "trim ()". Let's know how to use trim in JavaScript.
At the click event of the button, a JS function is called where the trim function is used to delete the spaces before and after the string. To see the difference, the source string is displayed as web page loads. As you click the button, the trimmed string is shown by using the document.getElementById ("strtrimmed").innerHTML statement. In JavaScript, the trim () method is used to trim the white spaces from the beginning and end of the string but not anywhere in between string. Struggling with JavaScript frameworks? Codementor has 10,000+ vetted developers to help you. Experienced JavaScript experts are just one click away. We split the string into an array, using whitespaces as the breakpoint - split (" "). Then join the array back into a string (which is devoid of whitespaces now) - join (""). An interesting way to remove whitespaces, but has bad performance, and not practical at all.
If you want to remove leading whitespace from a JavaScript string, the trimStart() function is what you're looking for. Equivalently, you can call trimLeft(), which is an alias for trimStart(). let example = ' Hello World'; example.trimStart(); // 'Hello World' example.trimLeft(); // 'Hello World'. The trimStart() function is a relatively recent addition to JavaScript, so you need a polyfill ... Remove leading and trailing whitespace from JavaScript String: Using trim () method which works only in higher version browsers Using replace () method which works in all browsers 1. 1 week ago - To trim String in JavaScript, use the String.trim() method. The trim() method is a built-in JavaScript method that removes whitespace from both sides of the String. Whitespace, in this context, is all of the whitespace characters, including space, tab, no-break space, and all the line terminator ...
Nov 18, 2011 - Steven Levithan analyzed many different implementation of trim in Javascript in terms of performance. Nov 14, 2012 - String trimming is one of the most common tasks that programmers deal with. Trimming functions remove whitespace from the beginning and/or end of a string. Native support for trimming was introduced in JavaScript 1.8.1, meaning that they were not part of Internet Explorer prior to IE9. Sep 02, 2014 - Note that none of this works with other types of whitespace, for instance   (thin space) or (non-breaking space). You can also trim strings from the front or back. ... Frontend Masters is the best place to get it. They have courses on all the most important front-end technologies, ...
Whitespace is any whitespace character like tab, space, LF, no-break space, etc. In this tutorial, we will learn how to trim from the start, end and both sides of a string in Javascript with examples. Remove whitespace from both sides of a string : In Javascript, String.trim method is used to remove whitespaces from both sides of a string. This ... JavaScript trim () String. The JavaScript trim () method removes any white spaces from the start and end of a string. The trim () method creates a new string. trim () does not accept any arguments. This method is added to the end of a string. Here is the syntax for the trim () method: var ourString = "Test " : ourString.trim (); Jul 10, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Remove Whitespace Characters with trim () Method The trim () method in JavaScript can be used to remove whitespace characters from both ends of a string. This is useful for stripping out any formatting that might be used in conjunction with spaces or tabs. After entering text with leading and/or trailing whitespaces click the button " show trim " that will trigger Javascript function. It will execute JS trim method and show an alert with the entered text after trim string function is executed. See example by clicking the link below: Experience this example online Jul 22, 2017 - Read writing from Raphael Ugwu on Medium. Writer, Software Engineer and a lifelong student. Every day, Raphael Ugwu and thousands of other voices read, write, and share important stories on Medium.
In JavaScript, you can use the trim() method to remove whitespace characters from the beginning and end of the string. It returns a new string stripped of whitespace characters. The whitespace characters are space, tab, no-break space, and all the line terminator characters (LF, CR, etc.).
How To Trim White Spaces From Input In Reactjs Geeksforgeeks
Trim Leading Whitespace From Html Output Issue 7884
About Basic String Strip Function Knime Analytics Platform
Trim Space Is Not Working Automation Cloud Community
Strip White Space From All Columns In Table Knime Analytics
Js Split String On Whitespace And Add To Array Code Example
Remove Trailing Spaces Automatically Or With A Shortcut
How To Remove White Spaces From String In Java
How To Trim String In Javascript Dev Community
Trim White Spaces From A String In Javascript
Basics Of Javascript String Trim Method By Jakub
How To Auto Remove Trailing Whitespace In Eclipse Stack
How To Remove The Whitespace From Both Sides Of The String By
Google Sheets Remove Leading And Trailing Spaces
Trim Is Not A Function Error When Trying To Remove The Space
Python Trim String How To Trim A String In Python Datagy
Visual Studio Code Shortcut 18 How To Trim Trailing
Trim Method For Strings In Javascript Codesource Io
How To Trim String In Javascript Samanthaming Com
Pandas Strip Whitespace From Entire Dataframe Geeksforgeeks
How To Trim String In Javascript Samanthaming Com
Fastest Method To Remove All Whitespace From Strings In Net
Remove Leading And Trailing Whitespace From Javascript String
Remove White Space From Text That I Scraped From Website
Regex Harvest Variables And Trim Trailing White Space
Java Exercises Trim Any Leading Or Trailing Whitespace From
Github Fvsch Php Trim Whitespace Trim Whitespace
0 Response to "29 How To Trim Whitespace In Javascript"
Post a Comment