27 Javascript Object Dot Notation
Sep 10, 2020 - Dot-Object makes it possible to transform javascript objects using dot notation. 9/1/2020 · Dot notation is simply the act of reading or assigning values to an object by using a dot (. ). Let’s say you have an object called dog: const dog = { name: 'Naya', age: 2 } Now we want to read one of its values, e.g. the age of the dog: console.log( dog. age) That’s reading values from an object with dot notation.
Seven Javascript Quirks I Wish I Had Known About
13/6/2021 · This makes code more readable. Dot notation is used most frequently. Syntax: obj.property_name. var user = {name : "Mark"}; user.name ; // "Mark" When not to use dot notation
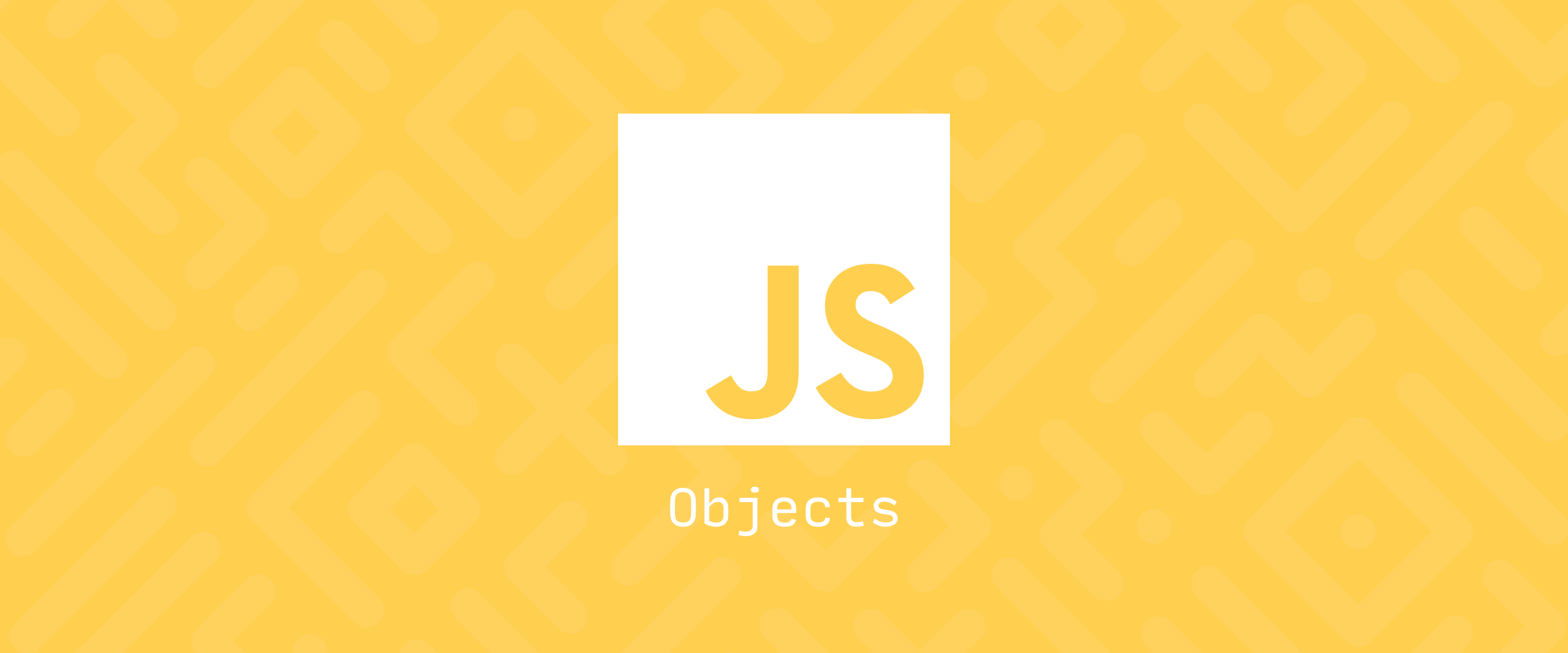
Javascript object dot notation. Convert a JavaScript string in dot notation into an object reference. Ask Question Asked 10 years, 2 months ago. Active 4 days ago. Viewed 159k times ... If you want to convert a string dot notation into an object, I've made a handy little helper than can turn a string like a.b.c.d with a value of e with dotPathToObject("a.b.c.d", ... In JavaScript, one can access properties using the dot notation (foo.bar) or square-bracket notation (foo["bar"]). However, the dot notation is often preferred because it is easier to read, less verbose, and works better with aggressive JavaScript minimizers. foo["bar"]; Rule Details. This rule is aimed at maintaining code consistency and ... Lets see some of the important points of dot and bracket notations (obj.x <---- here x is a property identifier) To access javascript object properties using dot notation, the Property identifiers can only be alphanumeric (and _ and $) Property identifiers cannot start with a number. Property identifiers cannot contain variables.
Spread syntax can be used when all elements from an object or array need to be included in a list of some kind. In the above example, the defined function takes x, y, and z as arguments and returns the sum of these values. An array value is also defined. When we invoke the function, we pass it all the values in the array using the spread syntax ... This is an example of dot notation. You can access properties on an object by specifying the name of the object, followed by a dot (period) followed by the property name. This is the syntax: objectName.propertyName;. When working with dot notation, property identifies can only be alphanumeric (and _ and $). JSON, short for JavaScript Object Notation, is a data-interchange format. It has a special syntax that looks a lot like the syntax for JavaScript objects. Sometimes, keys in JSON might be more than one word.
Properties of a JavaScript object can be accessed using a dot notation (.) or bracket notation [ ]. For Example, objectName.propertyName or objectName ['propertyName'] will give us the value. All members of an object literal in JavaScript, both properties and functions, are public. function changeObjectToDotNotationFormat(inputObject, current, prefinalObject) { const result = prefinalObject ? prefinalObject : {}; // This allows us to use the most recent result object in the recursive call for (let key in inputObject) { let value = inputObject[key]; let newKey = current ? `${current}.${key}` : key; if (value && typeof value === "object") { changeObjectToDotNotationFormat(value, newKey, … May 01, 2013 - JavaScript provides two notations for accessing object properties. The first, and most common, is known as dot notation. Under dot notation, a property is accessed by giving the host object’s name, followed by a period (or dot), followed by the property name.
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. Dot notation In the object.property syntax, the property must be a valid JavaScript identifier. (In the ECMAScript standard, the names of properties are technically "IdentifierNames", not "Identifiers", so reserved words can be used but are not recommended). For example, object.$1 is valid, while object.1 is not. Javascript : Dot (.) notation vs Bracket [ ] notation. For most of the developers, Dot (.) or Bracket [] does not matter. They randomly use any one of these and once they ran into a strange problem they start to believe that "Javascript is such a mess". Believe me, Javascript is not the mess but yes it's a different and unique programming ...
Safely sets object properties with dot notation strings in JavaScript. That is to say, it allows setting a nested property on an object using a string such as "a.b.c". For example: set (obj, "a.b.c", value) would be equivalent to a.b.c = value Some notes on intended behavior 7/12/2014 · Dot notation is always preferable. If you are using some "smarter" IDE or text editor, it will show undefined names from that object. Use brackets notation only when you have the name with like dashes or something similar invalid. And also if the name is stored in a variable. We can access nested properties of an object or nested objects using the dot notation (.) or the bracket notation []. Nested properties of an object can be accessed by chaining key or properties names in the correct sequence order. Example: JavaScript Objects, access Nested Properties of an object using dot & bracket notation
Jul 20, 2021 - There are two ways to access properties: dot notation and bracket notation. ... In the object.property syntax, the property must be a valid JavaScript identifier. (In the ECMAScript standard, the names of properties are technically "IdentifierNames", not "Identifiers", so reserved words can ... Accessing Object Properties with Dot Notation There are two ways to access the properties of an object: dot notation (.) and bracket notation ([]), similar to an array. Dot notation is what you use when you know the name of the property you're trying to access ahead of time. Feb 11, 2020 - Dot Notation vs. Bracket Notation Syntax ... JavaScript is an interpreted, object-oriented language that has two main data types: primitives and objects. This data within JavaScript is contained as fields (properties or variables) and code (procedures or methods).
JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ... Feb 07, 2017 - Well dot notation is a little bit easier to read. however, it has to do with the way JS “unboxes” statements. If you use dot notation, javascript goes till this first dot and then starts to unbox the property after the dot. For instance, let’s say we have the following object: However, the advantage of the literal or initializer notation is, that you are able to quickly create objects with properties inside the curly braces. You notate a list of key: value pairs delimited by commas.. The following code creates an object with three properties and the keys are "foo", "age" and "baz".The values of these keys are a string "bar", the number 42, and another object.
If you used the Dot notation, it will just assume you're trying to access the property with a valid JavaScript identifier. Because it's returning something, you might think everything is working... Safely setting object properties with dot notation strings in JavaScript Safely setting object properties with dot notation strings in JavaScript Javascript Front End Technology Object Oriented Programming You can use lodash's set method to set properties at any level safely. As it turns out, this dot notation is how JavaScript grants access to the data inside an object. The dot (.) is simply an operator that sits between its operands, just like + and -. By convention, the variables stored in an object that we access via the dot operator are generically called properties.
The dot notation and bracket notation both are used to access the object properties in JavaScript. The dot notation is used mostly as it is easier to read and comprehend and also less verbose. The main difference between dot notation and bracket notation is that the bracket notation allows us to access object properties using variable. Mar 23, 2019 - Convert Javascript string in dot notation into an object reference - string-dot-notation-to-object Dot notation Above, you accessed the object's properties and methods using dot notation. The object name (person) acts as the namespace — it must be entered first to access anything encapsulated inside the object.
The "Object Dot Notation" Lesson is part of the full, JavaScript: The Hard Parts, v2 course featured in this preview video. Here's what you'd learn in this lesson: Will provides a scenario in order to make the argument for storing data and functionality together in one place. Dot notation is introduced as a way to allow users to assign and ... Get a nested object property by passing a dot notation string as the property name. Raw. getProperty.js. /**. * A function to take a string written in dot notation style, and use it to. * find a nested object property inside of an object. *. * Useful in a plugin or module that accepts a JSON array of objects, but. After you create an object and define its properties in JavaScript, you’ll want to be able to retrieve and change those properties. The two ways to access object properties are by using dot notation or square brackets notation. Dot notation In dot notation, the name of an object is followed ...
Nov 17, 2011 - where arguments(arg1 to argN) must ... functionBody is a string containing the javaScript statements comprising the function definition. In our case we take the advantage of string function body to retrieve object member with dot notation.... JavaScript Algorithms and Data Structures. Object Oriented Programming. Use Dot Notation to Access the Properties of an Object. The last challenge created an object with various properties. Now you'll see how to access the values of those properties. Here's an example: Objects are one of the most valuable things you can learn in JavaScript. You can use them to take your programs to the next level. An object is a collection of data - or key value pairs - which consist of variables and functions that you can access using dot notation.. Now that's a bunch of words that might not mean anything to you at the moment, so let's break it down.
Oct 03, 2020 - How to unflatten a JavaScript object in a daisy-chain/dot notation into an object with nested objects and arrays? How to turn a JSON object into a JavaScript array in JavaScript ? How to turn a String into a JavaScript function call? Safely setting object properties with dot notation strings ... Accessing Object Properties. There are 2 ways to access object properties are Dot and Bracket in JavaScript. const obj = {. name: 'value'. }; // Dot Notation. obj.name; // 'value' // Bracket ... Objects are key data structures in Javascript (pun intended) and are widely used, but there are some details which we often overlook. One of them is the difference between the dot and bracket notations used to access the object properties. In this article, we'll try to understand both the notations and the differences between them. Let's begin!
How To Access An Object Having Spaces In The Object S Key
Dot Vs Bracket Notation In Accessing Javascript Object By
Dot And Bracket Notation Javascript Basics
The Chronicles Of Javascript Objects By Arfat Salman Bits
How To Set Dynamic Property Keys With Es6 Samanthaming Com
Javascript Altering An Object Where Dot Notation Is Used
What Is A Javascript Object Key Value Pairs And Dot Notation
How To Create Objects In Javascript
Learn Object Dot Notation Javascript The Hard Parts V2
Dot Notation Vs Bracket Notation To Access Object Properties
เธเธงเธฒเธกเนเธเธเธ เธฒเธเธฃเธฐเธซเธง เธฒเธเธเธฒเธฃเนเธ Dot Notation เนเธฅเธฐ Bracket Notation เนเธ
Javascript Object Creation Using New Object Method Dot Net
Rules For Unquoted Javascript Object Literal Keys Stack
How To Add A Key Value Pair To An Object In Javascript
Why Do You Need To Use Bracket Notation Then Dot Notation To
Introduction To Java Script Java Script 2 Xingquan
Javascript Objects Explore The Different Methods Used To
Definition Of Object Field Amp Dot Notation Issue 676 Jagi
Everything About Javascript Objects By Deepak Gupta
Object Access Bracket Notation Vs Dot Notation With
What Is An Object In Javascript Part I Dev Community
Bracket Notation Works But Dot Notation Does Not Javascript
0 Response to "27 Javascript Object Dot Notation"
Post a Comment