26 Javascript Async Map Function
Async functions Any Async function returns a Promise implicitly, and the resolved value of the Promise will be whatever returns from your function. Our function has an async keyword on its... As we can see, the structure is similar to regular iterators: To make an object asynchronously iterable, it must have a method Symbol.asyncIterator (1).; This method must return the object with next() method returning a promise (2).; The next() method doesn't have to be async, it may be a regular method returning a promise, but async allows us to use await, so that's convenient.
How To Use Async And Await With Array Prototype Map
# javascript # async # typescript # map May 2, 2019 ・Updated on Jul 10, 2019 ・2 min read MDN Web Docs: The map () method creates a new array with the results of calling a provided function on every element in the calling array. Today I found myself in a situation where I had to use an asynchronous function inside of an Array.map.
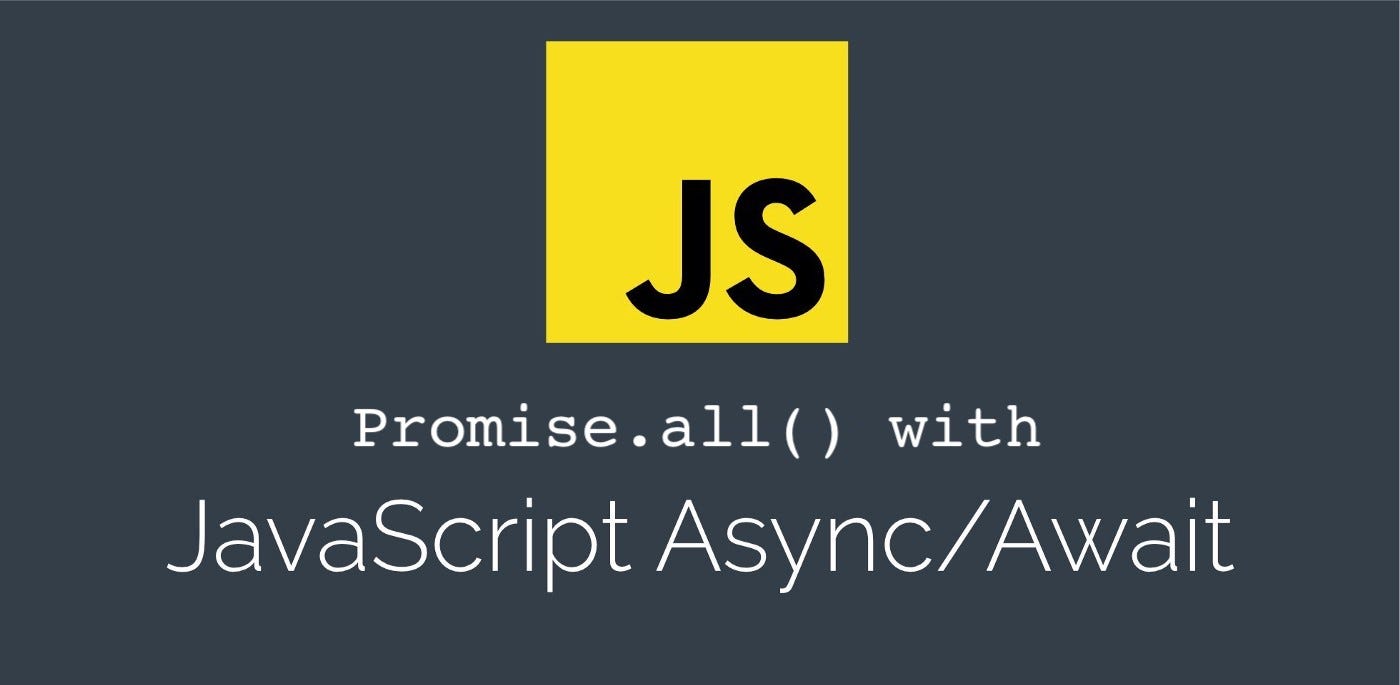
Javascript async map function. May 20, 2021 - I'm interested in that myself, I currently use callbacks for mapping over an array with asynchron requests, store the amount of requests + requests done and execute the finishing function (which is the callback for all requests) only if all requests are done. 25/2/2020 · It runs each element through an iteratee function and returns an array with the results. The synchronous version that adds one to each element: const arr = [1, 2, 3]; const syncRes = arr.map( (i) => { return i + 1; }); console.log(syncRes); // 2,3,4. An async version needs to do two things. First, it needs to map every item to a Promise with ... An async function expression is very similar to, and has almost the same syntax as, an async function statement.The main difference between an async function expression and an async function statement is the function name, which can be omitted in async function expressions to create anonymous functions. An async function expression can be used as an IIFE (Immediately Invoked Function ...
Callback based functions don't return a value. That means we have to use a special tool again - namely async.map The async map functions will be running at the same time. Although JavaScript is normally a single-threaded language, it means the resources allocated (like memory and ports) for each function will be occupied until the promise is resolved or rejected. For huge arrays, however, we are going to run a huge number of map functions at the same time. In javascript, the async array map method is a map method that uses async before the map function. A simple array map method modifies the object based on condition. But async array map method return the promise object instead of modified value. So, you need to pass async map array to promise.all to give the same result as map method.
The async and await keywords are a great addition to Javascript. They make it easier to read (and write) code that runs asynchronously. But they can still be confusing. Asynchronous programming is hard. Anyone who tells you differently is either lying or selling something. But there are some simple patterns you can learn that will make life easier. async. map (files, function (directory, callback) { fs.readFile(path.join(__dirname, ... A light-weight module that brings window.fetch to node.js. handlebars. Handlebars provides the power necessary to let you build semantic templates effectively with no frustration. crypto; colors. To declare an async class method, just prepend it with async: class Waiter { async wait() { return await Promise.resolve(1); } } new Waiter() .wait() .then( alert); The meaning is the same: it ensures that the returned value is a promise and enables await.
The function (the function name) is passed to setTimeout() as an argument. 3000 is the number of milliseconds before time-out, so myFunction() will be called after 3 seconds. When you pass a function as an argument, remember not to use parenthesis. Nov 10, 2020 - In this guide, we will specifically talk about how to use async/await syntax with the Array.map function available to all arrays in JavaScript. You will learn how to easily execute many asynchronous pieces of code in parallel using nothing but pure JavaScript. Nice post! Thanks for sharing. Just my 2 cents, in the function ParallelMapFlow the async await code in the map is not necessary: should be this let results = jobs.map(job => doJob(job,job)); instead of let results = jobs.map(async (job) => await doJob(job,job)); And, in the function ParallelPromiseAllFlow, the await in line number 7 the same ...
From the first steps with asynchronous programming in Javascript to an intricate understanding of how Promises and async functions work, the Asynchronous Programming Patterns in Javascript is a book that helps you write and reason about code easier. While working with Asynchronous JavaScript, people often write multiple statements one after the other and slap an await before a function call. This causes performance issues, as many times one statement doesn't depend on the previous one — but you still have to wait for the previous one to complete. async function. Việc tạo hàm với câu lệnh async function sẽ định nghĩa ra một hàm không đồng bộ (asynchronous function) - hàm này sẽ trả về một object AsyncFunction (en-US) Các hàm không đồng bộ sẽ hoạt động trong một thứ tự tách biệt so với phần còn lại của đoạn code thông ...
Apr 18, 2019 - You can't, but you can do something similar. This always trips me up so I thought I'd better write... Jul 18, 2021 - Hi, in this tutorial, we are going ... or use async-await inside the array map iterator in javascript. ... Last week, I was working on a client project to add one new feature, there is a situation where I need to call an API and pass data from every element of the array to that API. But the problem is the API function is returning ... Only when JavaScript is done running all its synchronous code, and is good and ready, will the event loop start picking from the queues and handing the functions back to JavaScript to run. So let's take a look at an example:
The sensible thing to do here would be to make the function passed to map asynchronous. This means that map would return an array of promises. We can then use Promise.all to get the result when all the promises return. As Promise.all itself returns a promise, the outer function does not need to be async. May 31, 2021 - TL:DR - Asynchronous, high order array-functions return an array of promises. In order to resolve... Async filter with map. The async version is a bit more complicated this time and it works in two phases. The first one maps the array through the predicate function asynchronously, producing true/false values. Then the second step is a synchronous filter that uses the results from the first step.
One useful thing to realise is that every time you mark a function as async, you're making that function return a promise. So of course, a map of async returns an array of promises :) - Anthony Manning-Franklin Oct 29 '18 at 6:52 | 27/3/2019 · Array.prototype.map() is a function that transforms Arrays. It maps one Array to another Array.The most important part of its function signature is the callback. The callback is called on each item in the Array and what that callback returns is what is put into the new Array returned by map.. It does not do anything special with what gets returned. I found myself stuck on using the map function with async and await. It took me relearning how to work with promises to figure it out, but once I figured it out, the syntax turned out to be pretty nice and readable. JavaScript's async and await syntax is new as of ES2017.
Jul 13, 2020 - This post will discuss using JavaScript's map method when dealing with async/await and promises. I'll be using a real-world example from my most recent project, viewable here. ... To start, I first had to fetch data from The Covid Tracking Project's API, so I did it using async/await, like so: async function ... 11/10/2018 · The main thing to notice is the use of Promise.all(), which resolves when all its promises are resolved.. list.map() returns a list of promises, so in result we’ll get the value when everything we ran is resolved. Remember, we must wrap any code that calls await in an async function.. See the promises article for more on promises, and the async/await guide. Jul 20, 2020 - Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance:
Jul 20, 2020 - using javascript when i ' m iterate localstorage current input value my DOM its Add multiple value or perivous value of localstorage ? ... Call the web api from $.ajax() function. Because of this, async collection processing requires some effort, and it's different depending on what kind of function you want to use. An async map works markedly differently than an async filter or and async reduce.. In this series, you'll learn how each of them works and how to efficiently use them. May 04, 2019 - I was writing some API code recently using Node 8, and came across a place where I needed to use Array.prototype.map with an async function on each item. Async/Await is one of my favorite new features in javascript as it makes asynchronous javascript code much more readable.
Sep 21, 2020 - Promises can be really tricky to understand in Javascript. Should we use Promises or the combo async/await? It can be a tough choice. But when it gets to doing functional programming, it can start to… Array.map() is a synchronous operation and runs a function on each element in the array resulting in a new array with the updated items. There are situations where you want to run asynchronous functions within map, e.g. updating a list of models and push the changed information back to the database or request information from an API that you want to use for further operations. async function An async function is a function declared with the async keyword, and the await keyword is permitted within them. The async and await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains. Async functions may also be defined as expressions.
ECMAScript 2017 introduced the JavaScript keywords async and await. The following table defines the first browser version with full support for both: Chrome 55. Edge 15. Firefox 52. Safari 11. Opera 42. Javascript async map function. Francesco Marassi. Executing Arrays Of Async Await Javascript Functions In. Javascript Es8 Introducing Async Await Functions By Ben. A Guide To Asynchronous Array Iterator Functions. Async Programming In Js Evolution Jobs Uk. Async Await Prompt Ui Beginner Javascript Wes Bos. P.S. The task is technically very simple, but the question is quite common for developers new to async/await. That's the case when knowing how it works inside is helpful. Just treat async call as promise and attach .then to it: async function wait () { await new Promise ( resolve => setTimeout ( resolve, 1000)); return 10; } function f ...
The Async Map Function Introduction By Preda W Medium
Async Await Vs Promises A Guide And Cheat Sheet By Kait
Array Map Async Await Dev Community
Node Hero Understanding Async Programming In Node Js
Javascript Async Await Serial Parallel And Complex Flow
A Problem With Fetch Api And Map Function In React Js Please
Async Await In Node Js Or Es6 With Loops Amp Github Api
Async Await In Typescript Logrocket Blog
How To Use Async Functions With Array Map In Javascript
Async Programming In Js Evolution Jobs Uk
Executing Arrays Of Async Await Javascript Functions In
Javascript Loops How To Handle Async Await
Node Js Workshop Javascript Async
Multiple Ways Of Async Await Fetch Api Call With Hooks Map
Nodejs Logging Made Right Habr
Calling An Async Function From Another Async Function Js Code
Async Map With Limited Parallelism In Node Js By Alex
Save Time And Money With Aws Lambda Using Async A Cloud Guru
How To Deal With Async Await In The Array Functions By
Promise All And Map With Async Await By Example
Callback Vs Promises Vs Async Await Loginradius Engineering
How To Use Async And Await In Javascript
Using Async Await With A Foreach Loop Stack Overflow
Node Js Tips Async And Map Async And Mongodb And Copying
Using Map Apply Bind And Sequence In Practice F For Fun
0 Response to "26 Javascript Async Map Function"
Post a Comment