27 Javascript Document Methods And Properties
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The DOM or Document Object Model is the API that becomes available when an HTML page loads in a browser. This object model contains more than 50 methods and properties which can be accessed using the document keyword in JavaScript. One example of utilising the DOM API is to get the title of an HTML document using the document.title property:
Javascript Chapter 12 Document Object Model
To retrieve an element in an HTML document, you can use collections (all, anchors, applets, etc.), the getElementById, getElementsByName and getElementsByTagName methods and some other properties and methods. If you want to access the html or the body element, use the document.documentElement ...
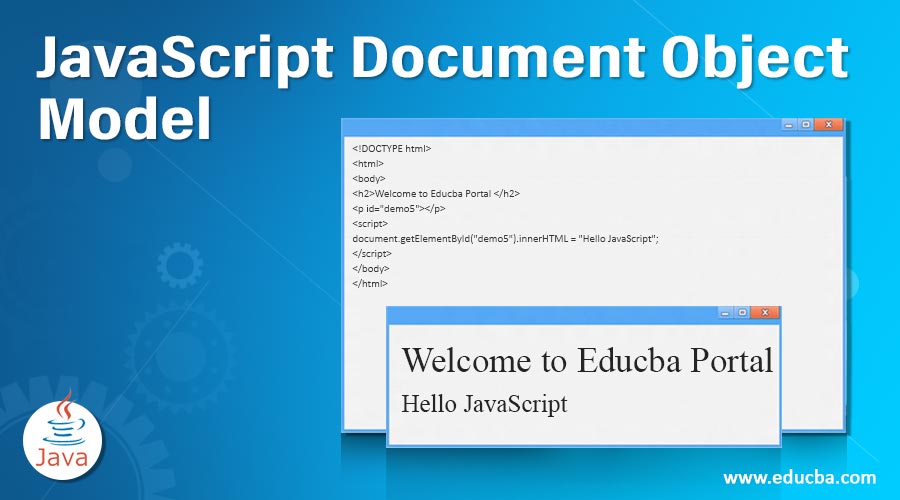
Javascript document methods and properties. A property holds some setting of an object. For example, the document.linkcolor property holds the color of hyperlinks in the current web page, and by changing the document.linkcolor property, you can change that color. Here are some example properties and the objects to which they belong: The document object represents the whole html document. When html document is loaded in the browser, it becomes a document object. It is the root element that represents the html document. It has properties and methods. By the help of document object, we can add dynamic content to our web page. As mentioned earlier, it is the object of window. So Document Object Model (DOM) is programming API For HTML and XML documents. When a web page is loaded, the browser creates a Document Object Model of the page.
JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ... In ES3 browsers (IE 8 and lower), the properties of built-in objects aren't enumerable. Objects like window and document aren't built-in, they're defined by the browser and most likely enumerable by design.. From ECMA-262 Edition 3:. Global Object There is a unique global object (15.1), which is created before control enters any execution context. Document that this is no longer the preferred way. @description: Describe a symbol. @enum: Document a collection of related properties. @event: Document an event. @example: Provide an example of how to use a documented item. @exports: Identify the member that is exported by a JavaScript module. @external: Document an external class/namespace ...
HTML DOM methods are actions you can perform (on HTML Elements). HTML DOM properties are values (of HTML Elements) that you can set or change. ... The HTML DOM can be accessed with JavaScript (and with other programming languages). List of Methods of Document Object. Here is the list of methods of document object in JavaScript: open() close() write() writeln() getElementById() getElementsByName() getElementsByTagName() JavaScript open() Method. The JavaScript open() method opens an HTML document to display the output. Here is the general form of open() method: Usually, the properties and methods are for objects, however, JavaScript has properties and methods for class also. There are some properties and methods that only belong to class. For example: counting the number of objects created by the class
JavaScript Document Object Properties. The document object provides various properties that are related to an HTML document such as title property, url property, etc. The property is accessed by using the notation given here: Here, objectName is the name of the object and the propertyName is the name of its property. Both are case-sensitive. 26/2/2020 · JavaScript Prototype Property: Function Object . Prototype is used to add new properties and methods to an object. Syntax. myobj.prototype.name = value. myobj: The name of the constructor function object you want to change. name: The name of the property or method to be created. value: The value initially assigned to the new property or method. The following properties and methods can be used on HTML documents: Property / Method. Description. activeElement. Returns the currently focused element in the document. addEventListener () Attaches an event handler to the document. adoptNode () Adopts a node from another document.
The references describe the properties and methods of each HTML object, along with examples. a abbr address area article aside audio b base bdo blockquote body br button canvas caption cite code col colgroup datalist dd del details dfn dialog div dl dt em embed fieldset figcaption figure footer form head header h1 - h6 hr html i iframe img ins ... A Few JavaScript Properties. href (url string in the browser that determines the page your browser loads) bgColor (background color of the document) value (the text - or lack thereof - in a form text field) src (path and filename of an image) Note: A document, although contained by the window and therefore a property of the window object, is ... Nov 10, 2020 - These methods operate exactly with what’s written in HTML. Also one can read all attributes using elem.attributes: a collection of objects that belong to a built-in Attr class, with name and value properties.
Forms and control elements, such as <input> have a lot of special properties and events.. Working with forms will be much more convenient when we learn them. Navigation: form and elements. Document forms are members of the special collection document.forms.. That's a so-called "named collection": it's both named and ordered.We can use both the name or the number in the document to get ... Element is the most general base class from which all element objects (i.e. objects that represent elements) in a Document inherit. It only has methods and properties common to all kinds of elements. More specific classes inherit from Element. For example, the HTMLElement interface is the base interface for HTML elements, while the SVGElement interface is the basis for all SVG elements. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
10/11/2020 · So, DOM properties and methods behave just like those of regular JavaScript objects: They can have any value. They are case-sensitive (write elem.nodeType, not elem.NoDeTyPe). DOM Node Methods. appendChild () — Adds a new child node to an element as the last child node. cloneNode () — Clones an HTML element. compareDocumentPosition () — Compares the document position of two elements. getFeature () — Returns an object which implements the APIs of a specified feature. The document object provides properties and methods to access all node objects, from within JavaScript. Tip: The document is a part of the Window object and can be accessed as window.document.
Dec 09, 2009 - This is a no-nonsense, easy to follow DOM reference for JavaScript. The first method is to access the property by using the dot(.) notation - object.property; The second method is by using square brackets - object[property] Lastly, we can store a property name, in the form of a string, in a variable and then use that variable to access the associated property. JavaScript Object Methods JavaScript Form Properties and Methods The method property is targeted at setting or returning the value of the method attribute in a form. The method attribute indicates the way of sending form-data. The latter is sent to the page, specified in the action attribute.
Here there is a list with useful properties and methods of the HTML objects that can be used in JS scripts. JavaScript code and examples that you can test directly on this page. Properties of the HTML objects elm.attributes - returns an object with properties ' name ', ' value ' with the assigned attributes of the corresponding HTML element. Private Methods ¶. In JavaScript, private methods have always been semi-possible due to the dynamic this and the Function prototype methods such as call and apply. Here is an example: function privateMethod () { this.doSomething (); } // The public method can call the above function // and retain the `this` context. JavaScript - Document Object Model or DOM, Every web page resides inside a browser window which can be considered as an object.
JavaScript has many document object and document object model with properties and methods associated. Data Object Model is a kind of programming interface that includes documents and objects. Documents in the programming interface are written using HTML (Hypertext Markup Language) or XML (Extensible Markup language). specialized kind of JavaScript object. Since functions are objects, they can have properties and methods, just like any other object. There is even a Function () constructor to create new function objects. The subsections that. follow document function properties and methods and the Function () constructor. JavaScript methods are written with parentheses that hold parameters. This can e.g. be kid.sleep("30 minutes") or kid.cry() . Then the kid has different properties as well, like weight, height, age etc.
DOM Selector Methods Adjust Element Properties In all browsers that use JavaScript we have access to a multitude of methods to affect what goes on the page. These methods will generally involve selecting and adjusting individual elements or accessing properties on the window. Jul 08, 2010 - This is a no-nonsense, easy to follow DOM reference for JavaScript. Methods of the document object Here there is a list with useful properties and methods of the document object, with Javascript code and examples. Properties of the document object document.body - returns the <body> HTML element.
The document object is the root node of the HTML document and the "owner" of all other nodes: (element nodes, text nodes, attribute nodes, and comment nodes). The document object provides properties and methods to access all node objects, from within JavaScript. Tip: The document is a part of the Window object and can be accessed as window ... 2 weeks ago - The Document Object Model (DOM) is the data representation of the objects that comprise the structure and content of a document on the web. This guide will introduce the DOM, look at how the DOM represents an HTML document in memory and how to use APIs to create web content and applications. tion considers DOM nodes in more detail, discussing methods and properties of DOM nodes that allow you to modify the DOM tree of a document using JavaScript. 10.3 Traversing and Modifying a DOM Tree The DOM gives you access to the elements of a document, allowing you to modify the contents of a page dynamically using event-driven JavaScript.
The HTML DOM Document Object. The document object represents your web page. If you want to access any element in an HTML page, you always start with accessing the document object. Below are some examples of how you can use the document object to access and manipulate HTML. JavaScript Document Object JavaScript Document object is an object that provides access to all HTML elements of a document. When an HTML document is loaded into a browser window, then it becomes a document object. The document object stores the elements of an HTML document, such as HTML, HEAD, BODY, and other HTML tags as objects. 1/9/2021 · Javascript document methods and properties. Javascript Document Object Model Top 5 Methods And Properties Element Getboundingclientrect Web Apis Mdn Js Document Object Model Javascript Document Object Methods Chapter 15 The W3c Dom And Javascript Javascript Document Object Model Top 5 Methods And Properties What Is A Node In Javascript Stack ...
In JavaScript, we use objects for declaring the value, property of the data which is a very important aspect of the programming. In JScript, each and everything is an object. Programmers use objects, properties of objects, and methods of objects to declare and use the objects in different concepts. In JavaScript, an object is a standalone entity with properties and methods. Consider the example of a cup of tea, where a cup is an object with properties. We can clearly say that the cup has a color, design, weight, material, etc. In the same vein, JavaScript objects can have properties, which define their characteristics.
Element Getboundingclientrect Web Apis Mdn
High Speed Dom Operations Javascript Unlocked
Javascript Dom Document Object Model Tutorial Koderhq
Javascript Objects Explore The Different Methods Used To
Html Dom The Document Object Model Is A Javascript Class That Defines Html Elements As Objects It Supports Properties Methods Events Image From Http Www W3schools Com Js Js Htmldom Asp
Lecture 5 Pdf Science Amp Amp Art Multimedia
Methods For Accessing Elements In The Dom File With
Javascript Dom Document Object Model Guide For Novice
Javascript Cheat Sheet For Design Junkies 2019
Javascript Document Object Model Or Dom
Javascript Document Object Model Top 5 Methods And Properties
Chapter 8 Client Side Pure Javascript Second Edition Book
Java Script O O A Highlevel Programming Language
What Is Dom In Javascript Dom Manipulation Web Api And Bom
Understanding The Document Object Node Js Mongodb And
What Is The Document Object Model
Working With Javascript In Visual Studio Code
Javascript Dom Tutorial With Example
Form Properties And Methods W3docs Javascript Tutorial
Javascript And Ajax Javascript Window Object Week 6 Web
Javascript Objects Methods And Properties 2 Html Element
0 Response to "27 Javascript Document Methods And Properties"
Post a Comment