32 Array String To Number Javascript
Jan 15, 2021 - Let's learn how to convert JavaScript array to string with or without commas. Example code included. Using JavaScript's sort Method for Sorting Arrays of Numbers The sort method available on the Array prototype allows you to sort the elements of an array and control how the… alligator.io
How To Convert A Nested Php Array To A Css Sass Less Rule
JavaScript has a built-in parseInt () method that converts a string into a corresponding integer. It takes two arguments. The first argument is the string to convert and the second argument is the numeral base. Go back to the convert.js file and add the following code:
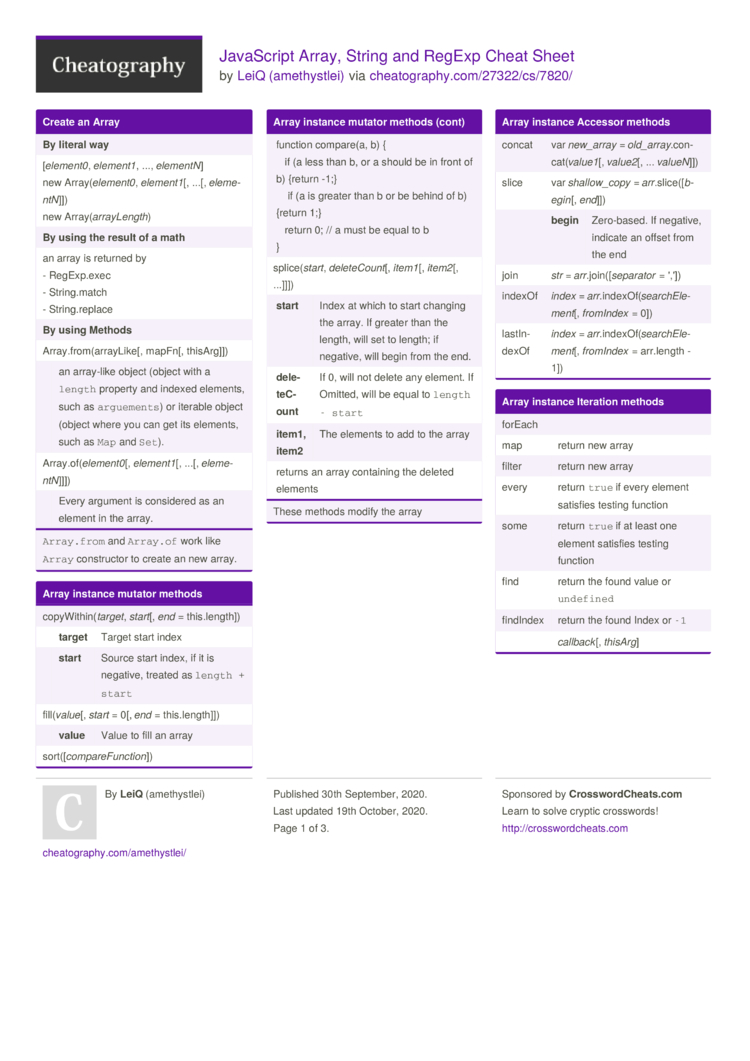
Array string to number javascript. The JavaScript Array.sort () method is used to sort the array elements in-place and returns the sorted array. This function sorts the elements in string format. It will work good for string array but not for numbers. For example: if numbers are sorted as string, than "75" is bigger than "200". Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... Mar 01, 2018 - You can also take advantage of JavaScript’s type coercion: the + operator can either add two numbers or concatenate two strings. But what happens when you try to join an object (an array [] is also an object) with something else? JavaScript is forgiving so it won’t crash our program, instead ...
There is a built-in function called Number which can be used in JavaScript to convert string to number. This function can convert both integers and float values inside the string to a number. For instance, Lets take a look at some of the examples here. console.log (Number ('123')); // output: 123 Nov 06, 2017 - In JavaScript, you can represent a number is an actual number (ex. 42), or as a string (ex. '42'). If you were to use a strict comparison to compare the two, it would fail because they’re two different types of objects. 1 week ago - Since an array's length can change ... array, JavaScript arrays are not guaranteed to be dense; this depends on how the programmer chooses to use them. In general, these are convenient characteristics; but if these features are not desirable for your particular use, you might consider using typed arrays. Arrays cannot use strings as element ...
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Sep 03, 2020 - How to generate a string out of an array in JavaScript Method 1. This code will set the array variable " arr " to a new empty array. It will be perfect if you don't have references to the original array. arr = []; Method 2. By set the array length to zero. arr.length = 0. Method 3. Using .splice (), the .splice () function will return an array with all the removed items.
We call split with the comma to separate the string into an array with the number strings in it. Then we call map with a callback that converts each entry to a number with the unary + operator. Therefore, nums is [1, 2, 3, 4] as a result. Instead of using the unary + operator, we can also use the parseInt function. The following example defines a function that splits a string into an array of strings using separator. After splitting the string, the function logs messages indicating the original string (before the split), the separator used, the number of elements in the array, and the individual array elements. The toString () method returns a string with all array values separated by commas. toString () does not change the original array.
An array in JavaScript can hold different types of value in a single variable. The value can be a string or a number. Sometimes you might have to separate the numbers and strings from the array. I'll show how easily you can filter out numbers in an array using JavaScript. Home » Javascript » Converting an Array to string in JavaScript. Converting an Array to string in JavaScript. Last updated on July 31, 2021 by Yogesh Singh. Sometimes required to convert an Array to string format for displaying on the page, send to AJAX request, or further processing. This is what's called grapheme clusters - where the user perceives it as 1 single unit, but under the hood, it's in fact made up of multiple units. The newer methods spread and Array.from are better equipped to handle these and will split your string by grapheme clusters 👍 # A caveat about Object.assign ⚠️ One thing to note Object.assign is that it doesn't actually produce a pure array.
A primitive value in JavaScript is a string, number, boolean, symbol, and special value undefined. The easiest way to determine if an array contains a primitive value is to use array.includes() ES2015 array method: All objects in JavaScript have a toString method available for converting their values to strings. It may be the toString method inherited from the generic Object, or it may be specific to the particular type of object. For example, array objects, date objects, regular expression objects, and ... Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Strings in the console are symbolized by wrapping them in quotes. By that fact, we can assume that i is a string. Convert it to an integer and it will no longer be a string and no longer have those quotes. endFlowArray.push (+i); Your "numbers" in flowEnd and dateFlow are actually strings, not numbers. Share. Mar 01, 2018 - What if you actually want to iterate over an array of strings with map() and change it to numbers? Use Number! 4 weeks ago - The join() method creates and returns a new string by concatenating all of the elements in an array (or an array-like object), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator.
Separators make Array#join() a very flexible way to concatenate strings. If you want to join together a variable number of strings, you should generally use join() rather than a for loop with +. String#concat() JavaScript strings have a built-in concat() method. The concat() function takes one or more parameters, and returns the modified string. Use parseInt () function, which parses a string and returns an integer. The first argument of parseInt must be a string. parseInt will return an integer found in the beginning of the string. Remember, that only the first number in the string will be returned. number of occurrences of a number in an array javascript; how to count the frequency of elements in a list javascript; getting the frequency of elements in an array javascript; count occurrences of a number in another number javascript -array -string; count occurrences of a number in number javascript -array -string
In this tutorial, you will learn, how to convert strings to arrays & split string by a comma separated array in javascript. If you want to convert array or object array to comma separated string javascript or convert object array to a comma-separated string javascript. Type of Array in JavaScript with Example. There are two types of string array like integer array or float array. 1. Traditional Array. This is a normal array. In this, we declare an array in such a way the indexing will start from 0 itself. 0 will be followed by 1, 2, 3, ….n. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
String.split () Method The String.split () method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as an argument. You can also optionally pass in an integer as a second parameter to specify the number of splits. Jul 02, 2020 - Get code examples like "javascript Convert an array of strings to numbers" instantly right from your google search results with the Grepper Chrome Extension. The split () Method in JavaScript The split () method splits (divides) a string into two or more substrings depending on a splitter (or divider). The splitter can be a single character, another string, or a regular expression. After splitting the string into multiple substrings, the split () method puts them in an array and returns it.
Jul 20, 2021 - For Array objects, the toString method joins the array and returns one string containing each array element separated by commas. JavaScript calls the toString method automatically when an array is to be represented as a text value or when an array is referred to in a string concatenation. ARRAY.toString() Convert array to string, elements will be separated by commas. Click Here: ARRAY.JOIN(SEPARATOR) Convert array to string, elements will be separated by defined SEPARATOR. Click Here: ARRAY.flat(LEVEL) Flatten the array to the specified LEVEL. Click Here: JSON.stringify(ARRAY) JSON encode an array or object into a string. Click ... JavaScript array to string (with and without commas) Let's learn how to convert JavaScript array to string with or without commas. Example code included. Posted on January 14, 2021. To convert a JavaScript array into a string, you can use the built-in Array method called toString.
Using join() method to convert an array to string in javascript. If you want to form the string of the array values with custom separator then join(separator) is the method you are looking for. Oct 01, 2020 - To run the above program, you need to use the following command − ... Here, my file name is demo212.js. ... PS C:\Users\Amit\JavaScript-code> node demo212.js The integer array value= [ 101, 50, 70, 90, 110, 90, 94, 68 ] The string array value= [ 101, 50, 70, 90, 110, 90, 94, 68 ] In JavaScript, you'll often need to iterate through an array collection and execute a callback method for each iteration. And there's a helpful method JS devs typically use to do this: the forEach() method.. The forEach() method calls a specified callback function once for every element it iterates over inside an array. Just like other array iterators such as map and filter, the callback ...
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. In this article, we have given an array of strings and the task is to convert it into an array of numbers in JavaScript. There are two methods to do this, which are given below: Method 1: Array traversal and typecasting: In this method, we traverse an array of strings and add it to a new array of numbers by typecasting it to an integer using ... The arr.map(Number) simply runs over all items in array, executes the Number function on every item and adds the result into a new array. Just like as shown in the previous example. On the same basis, you can convert to String, Date, RegExp. However, not a Boolean, since Boolean("false") is true.
Javascript queries related to "javascript Convert an array of strings to numbers" string array to numbersjs; convert all string to number in javascript array Jul 02, 2020 - Turn an array of numbers into a long string of all those numbers. how to convert list of numbers to string in javascript To convert a number into an array, we can use the from() method from the global Array object in JavaScript. TL;DR // random number const num = 123456; // use Array.from() method to convert numbers into an array // Pass the string version of number "123456" as the first argument // and the Number constructor as the second argument const numsArr ...
Javascript Array To String With And Without Commas
Convert An Array To String In Javascript Clue Mediator
Difference Between Array And String Difference Between
How To Sort Alphabetically An Array Of Objects By Key In
In Java How To Convert String To Char Array Two Ways
Javascript Array Push How To Add Element In Array
Javascript Convert String Array To Array
How To Compare String And Number In Javascript Code Example
11 Ways To Check For Palindromes In Javascript By Simon
How To Convert A Byte Array To String With Javascript
5 Ways To Convert A Value To String In Javascript By
How To Convert Integer Array To String Array Using Javascript
Javascript Math Calculate The Sum Of Values In An Array
String Split Method Archives Js Startup
Using Powershell To Split A String Into An Array
Dynamic Array In Javascript Using An Array Literal And
Convert Iterables To Array Using Spread In Javascript By
How To Check If A Variable Is An Array In Javascript
How To Convert A String To A Number In Javascript
Convert Array Into String If Array Is Within An Array Using
Convert String To Number Or Array In Javascript With React
How To Construct Caml Query For An Array Of Strings Ishir
How To Remove Commas From Array In Javascript
C Convert Int To String Javatpoint
Jquery Convert Json String To Array Sitepoint
Javascript Array String And Regexp Cheat Sheet By
Javascript Typed Arrays Javascript Mdn
Convert Comma Separated String To Array Using Javascript
Parsing A Query String Into An Array With Javascript A
Type Conversion Between Node Js And C Alibaba Cloud
0 Response to "32 Array String To Number Javascript"
Post a Comment