33 Difference Between Variable And Object In Javascript
As a general rule, anything that is not a primitive type in JavaScript is an object of type 'object'. You are probably wondering why the type null is not in the list. This is because null is an object type. To get the type of a variable in JavaScript you can use the typeof operator. 9/11/2014 · 1. The difference lies in the type of the argument you pass. typeof apple //"string" typeof orange //"object". When passing string literals, you're passing immutable data, and it is passed by value, hence it cannot change. EDIT: That is, the data that window.apple points to cannot change.
How To Create A Custom Numeric Javascript Variable Displayr
In this article we'll look at a few examples to better understand how variables are treated and what the difference is between "reference" and "value". Passing Primitives Primitive data types in JavaScript are things like number , string , boolean , or undefined .
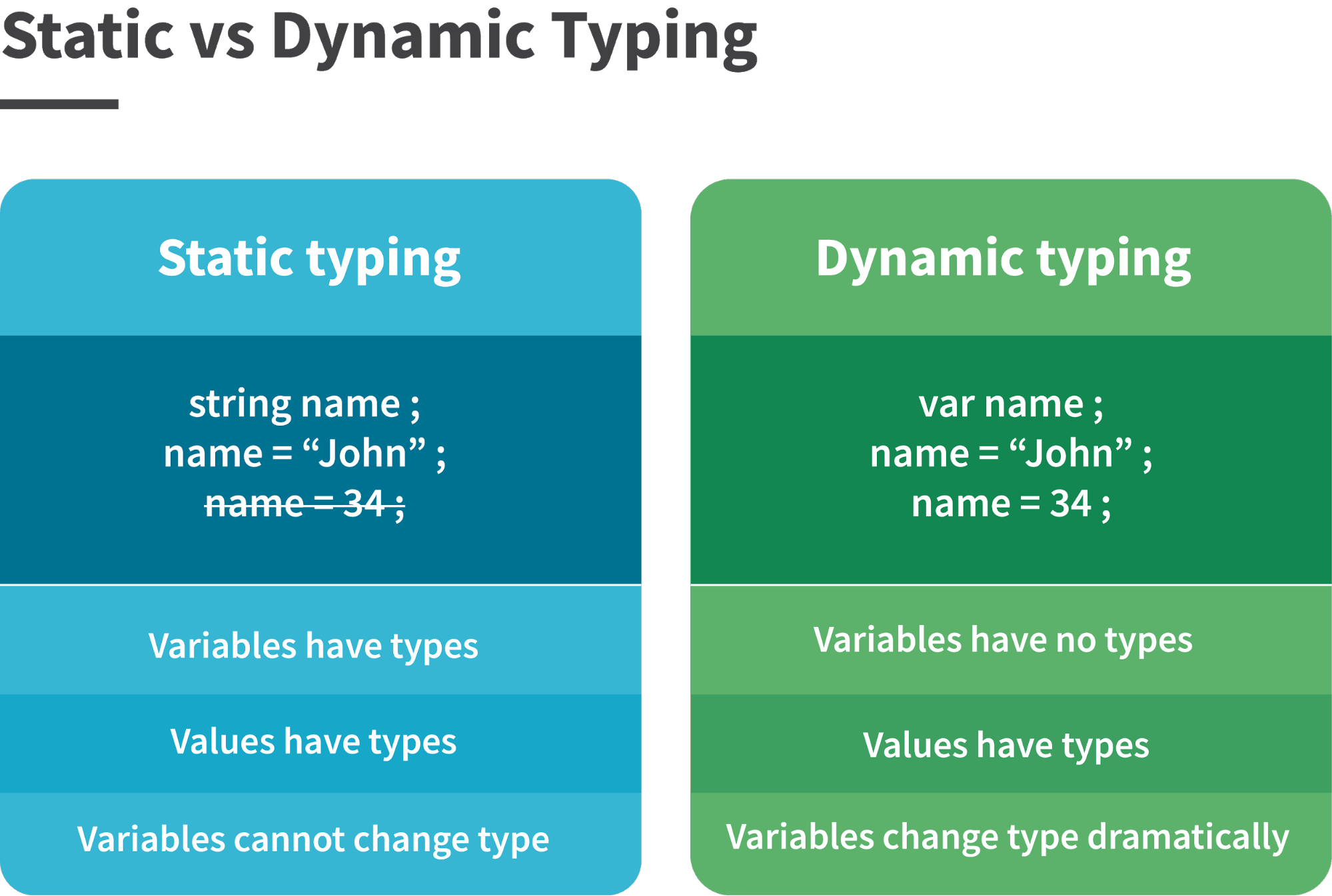
Difference between variable and object in javascript. In JavaScript variables were declared using the var keyword up until ECMAScript 6 (ES6). Before ES6, we used to declare variable using the var keyword where variables had global scope by default or in other words we can say the variables declared using the var keyword are available throughout the program and not just within a block.. While on the other hand, if we want a variable to exist only ... The scope determines the accessibility of variables and other resources in the code, like functions and objects. JavaScript function scopes can have two different types, the locale and the global scope. Local variables are declared within a function and can only be accessed within the function. And since the String is also an object, under name, a reference points out to the actual String value ("Krishna"). In short, object is an instance of a class and reference (variable) points out to the object created in the heap area.
The difference between private variable, public variable and static variable? How we achieve this in JS? How to add/remove properties to object in run time? How to achieve inheritance ? How to extend built-in objects? What is Object in JavaScript? What is the Prototype object in JavaScript and how it is used? What is "this"? What is its value? The let variables have the same execution phase as the var variables. The temporal dead zone starts from the block until the let variable declaration is processed. In other words, it is the location where you cannot access the let variables before they are defined. In this tutorial, you have learned about the differences between var and let ... Unlike JavaScript Object, a JSON Object has to be fed into a variable as a String and then parsed into JavaScript. A framework like jQuery can be very helpful when doing parsing. There are quite ...
In JavaScript, you can pass by value and by reference. The main difference between the two is that passing by value happens when assigning primitives while passing by reference when assigning objects. Let's discuss values and references in more detail in this post. 1. Understanding primitive and objects. Introduction. An object in JavaScript is a data type that is composed of a collection of names or keys and values, represented in name:value pairs.The name:value pairs can consist of properties that may contain any data type — including strings, numbers, and Booleans — as well as methods, which are functions contained within an object.. Objects in JavaScript are standalone entities that ... 24/10/2016 · I think a variable is just storing data like putting something in a box, think of the box as a variable. The object is a created thing from it's class template, it has behaviours and attibutes and it can interact with things. the variable on the other hand can just store data. correct me if I'm wrong :)
JavaScript variables can be objects. Arrays are special kinds of objects. Because of this, you can have variables of different types in the same Array. You can have objects in an Array. Both objects and arrays are considered "special" in JavaScript. Objects represent a special data type that is mutable and can be used to store a collection of data (rather than just a single value). Arrays are a special type of variable that is also mutable and can also be used to store a list of values. Object.is isn't "looser" than double equals or "stricter" than triple equals, nor does it fit somewhere in between (i.e., being both stricter than double equals, but looser than triple equals). We can see from the sameness comparisons table below that this is due to the way that Object.is handles NaN.
All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs. So , in nutshell , a variable is child of class object just like an object of user-defined class. In JavaScript, variables are objects that are "containers" for storing information. Objects are with all of their properties, methods, and event handlers. In JavaScript everything is an object. Being able to explain the differences between these 3 variables declarations in a javascript interview is essential for passing it. I've had this question asked in almost every tech interview I have had so far. I hope this post helps in the understanding of var, let, and const a little bit better.
Another key difference between JavaScript and Java is that JavaScript is a dynamically typed language, while Java is a statically typed language. This means, variables are declared with type at compile-time, and can only accept values permitted for that type, other hand variables are declared using var keyword in JavaScript, and can accept ... ***Note- Key differences between rest parameter and spread operator: Rest parameter is used to take a variable number of arguments and turns into an array while the spread operator takes an array or an object and spreads it ; Rest parameter is used in function declaration whereas the spread operator is used in function calls. All global JavaScript objects, functions, and variables automatically become members of the window object. The window is the first thing that gets loaded into the browser . This window object has the majority of the properties like length, innerWidth, innerHeight, name, if it has been closed, its parents, and more.
In JavaScript, variables are objects that are "containers" for storing information. Objects are with all of their properties, methods, and event handlers. In JavaScript everything is an object. JavaScript: Differences Between Using var, let, and const Keywords for Variable Declaration ... you can change the properties of an object. ... Difference between variables declared with "var ...
However, after the launch of ES6 in 2015, JavaScript also allowed use of the let keyword to initialize any variable. So, both keywords essentially help in declaring a variable in JavaScript. var a = 1; let b = 2; However, there are many differences between both the keywords in terms of functionality and behavior. …But we don't recommend doing that. Normally, one uses null to assign an "empty" or "unknown" value to a variable, while undefined is reserved as a default initial value for unassigned things.. Objects and Symbols. The object type is special.. All other types are called "primitive" because their values can contain only a single thing (be it a string or a number or whatever). Variable A variable is the name given to a memory location. It is the basic unit of storage in a program. The value stored in a variable can be changed during program execution. Each variable has a type, such as int, double or Object, and a scope. Class variable may be instance variable, local variable or constant.
Functions are objects, too. We can set properties and method on functions. typeof will return 'function' but the Function constructor derives from the Object constructor. The big differences between primitive types and objects are. primitive types are immutable, objects only have an immutable reference, but their value can change over time The Difference Between a Value and a Reference Primitive values and object references are stored in JavaScript programs in different ways. When a primitive value is assigned to a variable (eg let foo = 'bar'), the variable is set to that value directly. When the variable is assigned with an object, however, things are different. The difference is that the values stored in both variables now are the address of the actual object stored on the heap. As a result, both variables are referencing the same object. Consider the following example. First, declare a variable x that holds an object whose name property is 'John'.
Javascript Difference Between And Mycplus C And
What 39 S The Difference Between Using Let And Var Stack
Advanced Javascript Play With Object In Javascript
What Are The Differences Between A Variable And An Object In
Javascript Archives Codeforbetter
Creating Objects The Java Tutorials Gt Learning The Java
Objects In Javascript Geeksforgeeks
Javascript Typeof How To Check The Type Of A Variable Or
Class Javascript Instance Variable
The Difference Between Values And References In Javascript
Javascript Lesson 25 Difference Between Arrays And Objects
What Are The Different Data Types In Javascript Edureka
Seven Javascript Quirks I Wish I Had Known About
Javascript Interview Questions And Answers 2021 Interviewbit
C Class And Object With Example
Difference Between Object And Class Object Vs Class
Es6 Map Vs Object What And When By Maya Shavin
Difference Between Var And Let In Javascript Geeksforgeeks
Storing The Information You Need Variables Learn Web
Object Oriented Javascript For Beginners Learn Web
Difference Between Object Create Amp New Keyword In Javascript
Understanding Classes And Objects
3 Ways To Check If An Object Has A Property In Javascript
Identify If A Variable Is An Array Or Object In Javascript
Javascript Performance Lookups Through Prototype Amp Scope
Understanding Classes And Objects
Object Oriented Javascript For Beginners Learn Web
Methods For Deep Cloning Objects In Javascript Logrocket Blog
How To Use Object Destructuring In Javascript
0 Response to "33 Difference Between Variable And Object In Javascript"
Post a Comment