34 Javascript Decimal To Hex
Accurate hexadecimal to decimal conversion in JavaScript. Posted in javascript, programming at 4:05 pm by danvk. A problem came up at work yesterday: I was creating a web page that received 64-bit hex numbers from one API. But it needed to pass them off to another API that expected decimal numbers. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following: If the string begins with "0x", the radix is 16 (hexadecimal)
How To Convert Decimal To Hexadecimal In Javascript
Example 1: javascript convert string to 2 decimal var twoPlacedFloat = parseFloat(yourString).toFixed(2) Example 2: javascript convert number to hex yourNumber = par
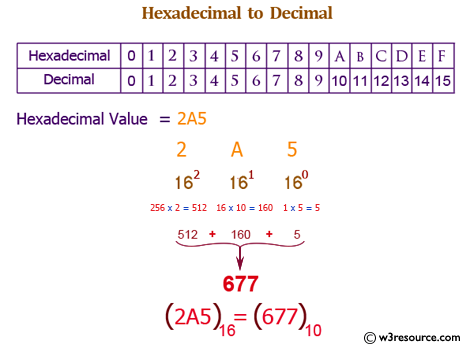
Javascript decimal to hex. Jan 30, 2021 - As decimal numbers include just 0 to 9, it is a little bit difficult to read big numbers while hexadecimal numbers are base-16 and it is easy to read hexadecimal numbers. Javascript code for Hexadecimal to decimal conversion: Get code examples like "convert number to hexadecimal in javascript" instantly right from your google search results with the Grepper Chrome Extension. Try our decimal to hex online converter tool. Hex to Dec in JavaScript Function parseInt() parses a string argument and returns an integer of the specified radix (the base in mathematical numeral systems).
JavaScript already offers a "native way" of doing it: // our decimal number const nr = 999999999; // convert to hex const hex = nr.toString(16); // back to dec: const decNr = parseInt(hex, 16); However, the example above can only handle numbers till Number.MAX_SAFE_INTEGER => 9007199254740991. In this tutorial, we are going to learn about how to convert decimal numbers to a binary, hexadecimal and octal in JavaScript. Number.toString. In JavaScript, we have a toString() method which takes the base as an argument and returns the string of the specified base. Let's see the examples. Converting decimal to binary Javascript provides a conversion method, and directly calls two functions to achieve conversion between the systems. Commonly used hexadecimal conversion: hex to decimal or decimal to hex, binary to decimal, hex, octal, octal to decimal, hex, etc. Then look at how to use javascript to realize these conversion between systems.
How to Convert Hexadecimal number to decimal in JavaScript? Reviewed by Bhaumik Patel on 7:37 PM Rating: 5 ... How to Convert Hexadecimal number to decimal in Ja... 11/7/2019 · How to convert decimal to hex in JavaScript ? Last Updated : 11 Jul, 2019. Given a number and the task is to convert the number from decimal to hex. This can be done by using toString () method. It takes the parameter which is the base of the converted string. In this case the base will be 16. Jul 17, 2014 - The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following:
Numbers in JavaScript are base 10 by default. Hexadecimal numbers usually start with 0x and octal numbers start with 0. To convert a decimal value to hexadecimal, pass the value 16 as a parameter to the number's toString () method. Similarly, pass the value 8 and 2 as parameters to toString () method to convert it to octal and binary format. Write a JavaScript code in a Web page dec2hex.html that enters a positive integer number num and converts and converts it to a hexadecimal form. The input should be entered as JavaScript prompt window. The output should be shown as browser popup window (alert). Decimal to Hexadecimal (n).toString(16))[see Number.prototype.toString]. Hexadecimal to Decimal. parseInt( hexadecimal_string, 16) [see Number.parseInt]
Read more: How to assign decimal, octal and hexadecimal values to the variables in JavaScript? In such cases, we can convert a decimal value to the hexadecimal string by using the number.toString (16) and hexadecimal string to the decimal by using hex_string.parseInt (16). In both cases, 16 is the base to the number system that says that target ... Related Searches to javascript tutorial - Decimal to hex decimal to hex table how to convert decimal to hexadecimal manually decimal to hexadecimal in c convert decimal to octal hexadecimal to binary converter 15 in hex text to hex converter 10 in hex decimal to hex table decimal to hexadecimal chart decimal to hexadecimal in c convert decimal ... convert decimal to hex js func. Convert Decimal To Hexa-Decimal including negative numbers react js. javascript change #ffffff to hex value. decimal to hex javascript without to string. jquery hex decoder to original converter. javascript decimal to hexidecimal. nodejs hex to int. javascript print number as hex.
The base is 16. In this tutorial, we will show you how simple can be converting decimal number to hexadecimal in JavaScript. ... The decimal number system has only ten digits from 0 to 9. Each value represents 0,1,2,3,4,5,6, 7,8 and 9 in this number system. The base is 10, as it has only ten digits. Questions: How do you convert decimal values to their hex equivalent in JavaScript? Answers: Convert a number to a hexadecimal string with: hexString = yourNumber.toString(16); and reverse the process with: yourNumber = parseInt(hexString, 16); Questions: Answers: If you need to handle things like bit fields or 32-bit colors, then you need to deal with signed ... Dec 11, 2018 - Now that JavaScript has native BigInt support it's finally easy to convert from hex strings to decimal strings.
The JavaScript function toString(16)will return a negative hexadecimal number which is usually not what you want. This function does some crazy addition to make it a positive number. How to Convert a Decimal to Binary. To understand how to convert a decimal to base, it's useful to first understand how to convert a decimal to binary. Binary is base 2. We have two different digits to count with, 0 and 1. (Or true and false). To calculate a binary value, we need to divide the decimal by 2 until the quotient is zero. We store ... Java Decimal to Hex conversion: Integer.toHexString () The Integer.toHexString () method converts decimal to hexadecimal. The signature of toHexString () method is given below: public static String toHexString (int decimal) public static String toHexString (int decimal) Let's see the simple example of converting decimal to binary in java.
As the accepted answer states, the easiest way to convert from dec to hex is var hex = dec.toString (16). However, you may prefer to add a string conversion, as it ensures that string representations like "12".toString (16) work correctly. // avoids a hard to track down bug by returning `c` instead of `12` (+"12").toString (16); Get code examples like "How to convert hexadecimal to decimal in JavaScript" instantly right from your google search results with the Grepper Chrome Extension. Convert Hexa to Decimal Using JS and fetch value in html, hexa to decimal canvert by javascript or jquery You can easily convert hexa to decimal by javascript and also show its value in the html page by id. You have a well explained below how you can convert hexa decimal to decimal by javascript You can show it in html page by converting id.
Convert Decimal Strings to Hex with JS BigInts With the introduction of native BigInts (also known as Big Nums or Arbirtrary-Precision Numbers), in JavaScript, it's now easy to convert from arbitrarily-large ints to hex (which is the gateway drug to typed arrays). However, there are some caveats, particulary with negative numbers. Jul 20, 2021 - This chapter introduces the concepts, objects and functions used to work with and perform calculations using numbers and dates in JavaScript. This includes using numbers written in various bases including decimal, binary, and hexadecimal, as well as the use of the global Math object to perform ... Jul 20, 2021 - The toString() method returns a string representing the specified Number object.
4 weeks ago - A value passed as the radix argument ... to NaN), JavaScript assumes the following: If the input string begins with "0x" or "0X" (a zero, followed by lowercase or uppercase X), radix is assumed to be 16 and the rest of the string is parsed as a hexadecimal number. If the input string begins with any other value, the radix is 10 (decimal)... A number with a radix of 16 indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. That is what we are going to use for this algorithm. return parseInt (hexString, 16); This is an extra bit of information about the parseInt method that I found interesting and didn't know existed. Divide the number by 16. Get the integer quotient for the next iteration. Get the remainder for the hex digit. Repeat the steps until the quotient is equal to 0.
We are going to code a rgbToHex() function that passes in RGB decimal values as inputs. Our goal for the function is to return the equivalent Hex codes. Hexadecimal (or Hex) is a base 16 system… By default, JavaScript displays numbers as base 10 decimals. But you can use the toString () method to output numbers from base 2 to base 36. Hexadecimal is base 16. Decimal is base 10. In this tutorial, we are going to learn about how to convert binary, hexadecimal and octal to decimal numbers in JavaScript. parseInt. In JavaScript, we have the parseInt() method by using that we can make conversions.
Mar 31, 2020 - Get code examples like "convert int to hex javascript" instantly right from your google search results with the Grepper Chrome Extension. Decimal to HexaDecimal. To convert a JavaScript Number to it's hexadecimal, toString() method is used. toString() method accepts a number parameter, ranging from 2 to 32, which specifies the base to use for representing the numeric values. (234). toString (16) // "ea" (-234). toString (16) // "-ea" Converting a negative number to hexadecimal ... To convert from RGB to HEX, we only need to convert the decimal number of each color from RGB to hexadecimal and then concatenate them together to create the equivalent hex color space. To change a value from decimal to hexadecimal, we can use the toString (16) function. If the length of the converted number is 1, we have to add a 0 before it.
Not a member? Sign Up · Demo of converting any decimal value to Hex 18/9/2019 · We can pass base 16(hex numbers) to get the desired number converted to hexadecimal string. Example console.log(Number(255).toString(16)) console.log(Number(17).toString(16)) Output ff 11. We can convert these numbers back to decimal using the parseInt function. The parseInt function available in JavaScript has the following signature −
How To Convert From Decimal To Hexadecimal 15 Steps
How To Convert From 4 Bit Hexadecimal To 7 Bit Ascii Stack
Decimal To Hex Converter To Convert Base 10 To Base 16
Convert Radix Number Bases With Node Red Decimal
Hex To Decimal Conversion Simplified Electrical Engineering 123
Use Hexadecimal Numbers In Calculation In Javascript
How To Convert Decimal To Hex In Javascript Geeksforgeeks
Github Tarekkhaled Js In Bits Convert Between Hexadecimal
Java Exercises Convert A Hexadecimal To A Decimal Number
5 27 6 1 Amp 6 3 Converting Decimal To Hexadecimal
Convert Hex To String Javascript Code Example
Javascript Rgb To Hex Code Example
How To Convert Hexadecimal To Decimal And Decimal To Hex
How To Convert Hexadecimal To Decimal And Decimal To Hex
Convert Number To Hexadecimal In Javascript Code Example
Best Hex To Decimal Converter Online
Decimal To Hex Converter Online Tool Coding Tools
Convert Decimal To Hex Knime Analytics Platform Knime
Converting To And From Hexadecimal By Karl Matthes Medium
Javascript Programming Tutorial 17 Converting Decimal Numbers To Binary Octal And Hexadecimal
Build A Hexadecimal Color Clock In Javascript By Sylvain
Convert Decimal To Hex In Javascript Github
C Program To Convert Hexadecimal To Decimal Aticleworld
C Sharp Exercises Convert A Number In Decimal To
Nodered How To Covert From Hex Number To Dec Number
Program For Decimal To Hexadecimal Conversion Geeksforgeeks
Javascript Numbers Get Skilled In The Implementation Of Its
How To Convert Hexadecimal To Binary Or Decimal 6 Steps
Hexadecimal To Decimal In C Javatpoint
Java Exercises Convert A Decimal Number To Hexadecimal
Javascript Algorithm Hex To Decimal By Erica N
Converting A String Type Hex Number To A Decimal Working
0 Response to "34 Javascript Decimal To Hex"
Post a Comment