32 Fetch Api Javascript Tutorial
Fetch is a promise based HTTP request API built in to JavaScript. It's fairly well supported and is promise based for ease of use and is a perfect fit for those wanting to use out of the box solutions for HTTP.. Let's take a look at a few different ways we can use fetch to access data from the JSON Placeholder API:. GET Below is the data . Fetch API. It is the newest standard for dealing with HTTPRequest, it is part of the window object, and we can easily fetch data from an external API as well.Fetch returns Promises I'll be demonstrating in the code below how to get data from Github API, an external API with Fetch API.
Fetch Api Introduction To Promised Based Data Fetching In
Next up, we're going to use the fetch() method to get the data from the API. When you console.log the fetch(), this is what you see in the console👇 The fetch() method creates a JavaScript ...
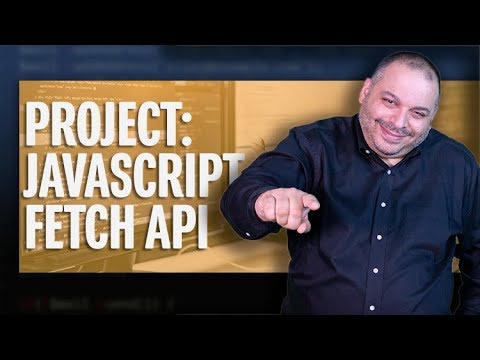
Fetch api javascript tutorial. The Fetch API was introduced around the same time as JavaScript ES6. It is a much cleaner promised-based API as opposed the the old XMLHttpRequest (XHR). Fet... 22/8/2020 · Fetch API. The Fetch API is a simpler, easy-to-use version of XMLHttpRequest to consume resources asynchronously. Fetch lets you work with REST APIs with additional options like caching data, reading streaming responses, and more. The major difference is that Fetch … The fetch () method is modern and versatile, so we'll start with it. It's not supported by old browsers (can be polyfilled), but very well supported among the modern ones. The basic syntax is: let promise = fetch( url, [ options]) url - the URL to access. options - optional parameters: method, headers etc.
Summary: in this tutorial, you'll learn about the JavaScript Fetch API and how to use it to make asynchronous HTTP requests. The Fetch API is a modern interface that allows you to make HTTP requests to servers from web browsers. If you have worked with XMLHttpRequest (XHR) object, the Fetch API can perform all the tasks as the XHR object does. The Fetch API is a promise-based JavaScript API for making asynchronous HTTP requests in the browser similar to XMLHttpRequest (XHR).Unlike XHR, it is a simple and clean API that uses promises to provides a more powerful and flexible feature set to fetch resources from the server. The main difference is that the Fetch API uses Promises, which enables a simpler and cleaner API, avoiding callback hell and having to remember the complex API of XMLHttpRequest. The Fetch API has been available in the Service Worker global scope since Chrome 40, but it'll be enabled in the window scope in Chrome 42. There is also a rather ...
In this tutorial, you'll learn about the JavaScript Fetch API and how to use it to make asynchronous HTTP or network requests similar to XMLHttpRequest (XHR) requests. And as well as, you will learn how to use the JavaScript fetch API to get json, text, html Data from apis. Compared to XMLHttpRequest and the libraries built around it, like JQuery.ajax, the fetch API defines a more modern and cleaner way of performing asynchronous requests, based on the use of promises.In this article we will see some of the interfaces provided by the API, like Request and Response, and we will learn how to use the fetch method to perform various types of asynchronous requests. Passo 2 — Usando Fetch para buscar dados de uma API. As amostras de código a seguir baseiam-se na Random User API. Usando a API, você irá buscar dez usuários e os exibirá na página usando o JavaScript puro. A ideia é obter todos os dados da Random User API e exibÃ-los em itens de lista dentro da lista de autores.
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch () method that provides an easy, logical way to fetch resources asynchronously across the network. This kind of functionality was previously achieved using XMLHttpRequest. Fetch can also be used in other environments, but for the purposes of this Fetch API tutorial, I'm going to focus on using the Fetch API in the browser. The fetch() method takes two arguments, but only one is mandatory: the location of the requested resource. # fetch() - An Introduction. If you want to send Http requests from (client-side) JavaScript, modern JavaScript offers the fetch() API - a powerful tool for sending Http requests. In the above video, we'll explore the fetch() API and understand how it works. You can dive deeper with the help of MDN and its fetch() API Docs.
Vanilla JS Fetch works with promises, so we can return a promise chain as we learned on 20-03-2020: Promise chains in JavaScript. The first API response will return an object, not an actual response, so we need to tell the promise that we want to return the response as JSON data. You can play around with this codepen. 15/6/2020 · The Fetch API provides an interface for fetching resources similar to XMLHttpRequest (XHR). The main difference is that the Fetch API uses Promises which make working with request and response easier, avoiding the callback hell. The Fetch API provides fetch() method defined on window object which can be used to make network request. Syntax The Fetch API interface allows web browser to make HTTP requests to web servers. ... JavaScript Tutorial How To Tutorial SQL Tutorial Python Tutorial W3.CSS Tutorial Bootstrap Tutorial PHP Tutorial Java Tutorial C++ Tutorial jQuery Tutorial. Top References HTML Reference CSS Reference
The Fetch API is used to make Ajax requests, such as calling an API or fetching a remote resource or HTML file from a server. In the past, I've been very vocal about my preference for XHR over Fetch. I was wrong. Fetch is objectively superior. It does everything I need it to with a simpler syntax. Let's look at how it works. The Basic Fetch API Syntax For today's article, we'll use ... fetch-api-tutorial. A guide to using JavaScript's simple Fetch API interface with typicode's JSON placeholder as the fake API to play with. GET, POST, PUT, PATCH, and DELETE are five most common HTTP methods for retrieving from and sending data to a server. The Fetch API provides a JavaScript interface that enables users to manipulate and access parts of the HTTP pipeline such as responses and requests. Fetch API has so many rich and exciting options like method, headers, body, referrer, mode, credentials, cache, redirect, integrity and few more.
By default, fetch requests make use of standard HTTP-caching. That is, it respects the Expires and Cache-Control headers, sends If-Modified-Since and so on. Just like regular HTTP-requests do. The cache options allows to ignore HTTP-cache or fine-tune its usage: "default" - fetch uses standard HTTP-cache rules and headers, Since the ES7, Fetch is now fully implemented in Chrome. You can even use the async-await and completely get rid of promises. How to use Fetch API? The fetch() method is available in the global window scope, with the first parameter being the URL you want to call. By default, the Fetch API makes a GET request. #Which is better old-time AJAX vs. Fetch. As you learned in this tutorial, the Fetch method is more friendly and modern than the old AJAX, and thus the fetch() method will eventually replace the old code, but it will take years. Until that happens, you need to overcome the problem of older browsers which don't support the new standard, especially the explorers and mobile browsers.
Now, JavaScript has its own built-in way to make API requests. This is the Fetch API, a new standard to make server requests with promises, but includes many other features. In this tutorial, you will create both GET and POST requests using the Fetch API. Prerequisites. To complete this tutorial, you will need the following: In this tutorial, I will be explaining how to fetch data asynchronously from an external API using the Fetch API. I believe in learning by practice, so here's the application we are going to ... bewoksatukosong | Hallo semuanya balik lagi dengan om bewok, kali ini om bewok akan share ke kalian cara menggunakan fetch api. Fetch ini di gunakan untuk mengambil data dan menampilkan data ke browser. Fetch API ini ada pada saat es 6 tiba. Kalau teman - teman belum belajar javascript, kalian bisa liat daftar tutorialnya dibawah ini.
The Fetch API provides a fetch() method defined on the window object, which you can use to perform requests. This method returns a Promise that you can use to retrieve the response of the request. Fetch API Demo - JavaScript Tutorial Approach: First make the necessary JavaScript file, HTML file and CSS file. Then store the API URL in a variable (here api_url). Define a async function (here getapi ()) and pass api_url in that function. Define a constant response and store the fetched data by await fetch () method. Define a constant data and store the data in JSON form by ...
Javascript Get Data From Fetch Api Example Tuts Make
React Fetch Http Post Request Examples Jason Watmore S Blog
How To Use The Javascript Fetch Api To Get Data Geeksforgeeks
How To Fetch Data In React Cheat Sheet Examples
How To Use Api With React Reactjs Api Call Example
Talking To Python From Javascript Flask And The Fetch Api
Advance Javascript Fetch Api Tutorial
Javascript Fetch Api Complete Guide To Javascript Fetch Api
How To Update Data Using Javascript Fetch Api Code Example
Javascript Fetch Api Tutorial With Js Fetch Post And Header
Fetch Api For Beginners Make Your First Application Using The
Javascript Fetch Api Get Data From External Rest Api
Fetch Api Introduction To Promised Based Data Fetching In
Get And Post Method Using Fetch Api Geeksforgeeks
Api Tutorial For Beginners With Google Sheets And Apps Script
Javascript Fetch Api Example Tutorial From Scratch Is Today S
Javascript Fetch Api Tutorial With Js Fetch Post And Header
How To Use Amp Integrate Apis Beginners Guide W Tutorial
Beginners Guide To Fetching Data With Ajax Fetch Api
React Native Tutorial 26 How To Submit Form And Fetch Api
Simplest Way To Use Axios To Fetch Data From An Api In
How To Use The Javascript Fetch Api To Get Data
Fetch Api Introduction To Promised Based Data Fetching In
Javascript Fetch Api Tutorial Creating A Beer Pairing App
How To Fetch Rest Api By Using Tools And Javascript By
Consuming Rest Apis In React With Fetch And Axios Smashing
Fetching Data From The Server Learn Web Development Mdn
Learn Fetch Api In 6 Minutes Youtube
Javascript Fetch Api Tutorial With Js Fetch Post And Header
0 Response to "32 Fetch Api Javascript Tutorial"
Post a Comment