35 Javascript Check If Value Is Function
Code Recipe to check if an array includes a value in JavaScript using ES6 In the above program, the passed value is checked if it is an integer value or a float value. The typeof operator is used to check the data type of the passed value. The isNaN () method checks if the passed value is a number. The Number.isInteger () method is used to check if the number is an integer value.
Learn About The Common Javascript Interface For Adobe Captivate
20/3/2015 · Checking strictly for undefined values: function test {if (a) {console. log ('a exists!');}} // The above function will throw a ReferenceError: a is not defined function test {if (typeof a!== 'undefined') {console. log ('a exists!');}} // This second function won't log anything, but it also won't throw an error! In the above example, a was never declared.
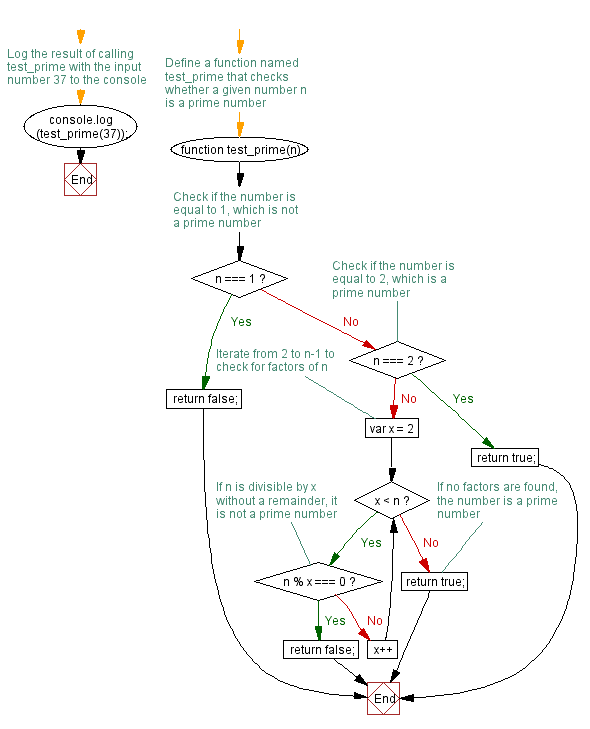
Javascript check if value is function. JavaScript Form Validation. HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted: Definition and Usage. The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed. The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions. I found that when testing native browser functions in IE8, using toString, instanceof, and typeof did not work. Here is a method that works fine in IE8 (as far as I know): function isFn(f){ return !!(f && f.call && f.apply); } //Returns true in IE7/8 isFn(document.getElementById); Alternatively, you can check for native functions using:
May 26, 2018 - In a JavaScript program, the correct way to check if an object property is undefined is to use the `typeof` operator. See how you can use it with this simple explanation In JavaScript obj.propName === undefined is true if either obj has a property 'propName' and the property's value is trictly equal undefined, or if obj does not have a property 'propName'. If you want to check whether obj has a property and that property is strictly equal to undefined, you should use the in operator. Since the very earliest versions of the isNaN function specification, its behavior for non-numeric arguments has been confusing. When the argument to the isNaN function is not of type Number, the value is first coerced to a Number.The resulting value is then tested to determine whether it is NaN.Thus for non-numbers that when coerced to numeric type result in a valid non-NaN numeric value ...
A promise describes a placeholder for an asynchronous computation that only returns the value to a given callback if you call .then (callback). You can use this behavior to determine whether a given value is a promise. Because promises implement the .then () method, you can check whether it's a function. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Jul 20, 2018 - A couple of days ago I shared a technique for checking if a function exists before trying to run it. Check if value is empty in JavaScript. Javascript Web Development Object Oriented Programming. Use the condition with "" and NULL to check if value is empty. Throw a message whenever ua ser does not fill the text box value. To get the state of a checkbox, whether checked or unchecked, you follow these steps: First, select the checkbox using the selecting DOM methods such as getElementById () or querySelector (). Then, access the checked property of the checkbox element. If its checked property is true, then the checkbox is checked; otherwise, it is not.
Variable is of function type Using Strict Equal (===) operator: In JavaScript, '===' Operator is used to check whether two entities are of equal values as well as of equal type provides a boolean result. In this example, we use the '===' operator. This operator, called the Strict Equal operator, checks if the operands are of the same type. Compared to typeof approach, the try/catch is more precise because it determines solely if the variable is not defined, despite being initialized or uninitialized.. 4. Using window.hasOwnProperty(). Finally, to check for the existence of global variables, you can go with a simpler approach. Each global variable is stored as a property on the global object (window in a browser environment ... This function is doing the calculation for us, and most importantly we are checking here if the checkboxes are checked using the JavaScript if else condition. function calculateCheckbox {// get beauty products checkboxes contianer's reference var el = document. getElementById ('beautyProducts'); // get beauty product input element reference in ...
Object.is () determines whether two values are the same value. Two values are the same if one of the following holds: both undefined. both null. both true or both false. both strings of the same length with the same characters in the same order. both the same object (meaning both values reference the same object in memory) Use the instanceof Function to Check Whether a Value Is an Object or Not in JavaScript We will check the value with the help of the instanceof method, which returns the type of object at run-time. In the following example, the function will return True if the value is of an object type. Definition and Usage The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false.
The isFinite () function determines whether a number is a finite, legal number. This function returns false if the value is +infinity, -infinity, or NaN (Not-a-Number), otherwise it returns true. How to Check if a Value is an Object in JavaScript JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object". The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf () method. This method searches the array for the given item and returns its index. If no item is found, it returns -1.
function isObject(value) { /* Requires ECMAScript 3 or later */ return new function() { return value; }() === value; } While a bit less simple than the previous example, this one does not rely on any global property, and thus might be the safest. this value. Old ECMAScript specifications required the this value to be an object. If the value is an Array, true is returned; otherwise, false is. See the article "Determining with absolute accuracy whether or not a JavaScript object is an array" for more details. Given a TypedArray instance, false is always returned. The isNaN () function to test whether the num value is not a number — if so, it returns true, and if not, it returns false. If the test returns false, the num value is a number. Therefore, a sentence is printed out inside the paragraph element that states the square, cube, and factorial values of the number.
21/6/2020 · We have various ways to check if a value is a number. The first is isNaN (), a global variable, assigned to the window object in the browser: const value = 2 isNaN(value) //false isNaN('test') //true isNaN({}) //true isNaN(1.2) //false If isNaN () returns false, the value is a number. AFAIK in JAVASCRIPT when a variable is declared but has not assigned value, its type is undefined. so we can check variable even if it would be an object holding some instance in place of value. create a helper method for checking nullity that returns true and use it in your API. 10/11/2020 · Many programming languages like Java have strict type checking. This means that if a variable is defined with a specific type it can contain a value of only that type. JavaScript, however, is a loosely typed (or dynamically typed) language. This means that a variable can contain a value of any type.
1 week ago - The typeof operator returns a string indicating the type of the unevaluated operand. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. 1 week ago - The if statement executes a statement if a specified condition is truthy. If the condition is falsy, another statement can be executed.
How to check if a JavaScript variable is a function or not. This is a simple guide on how to check if a JavaScript variable is a function or not. As you probably already know, JavaScript allows you to create and assign functions to variables. This makes it possible to pass functions as parameters to other functions. Oct 09, 2012 - I need to loop over the properties of a javascript object. How can I tell if a property is a function or just a value? This method is best suited for when you know you have a number and would to check if it is a NaN value, not for general number checking. Using the typeof() function. The typeof() function is a global function that accepts a variable or value as an argument and returns a string representation of its type. JavaScript has 9 types in total:
Jul 17, 2014 - Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In JavaScript Number.isInteger () method works with numbers, it will check whether a value an integer. This method returns true if the value is of the type Number and an integer. Otherwise, it will return false. If the target value is an integer, return true, otherwise, return false. If the value is NaN or Infinity, return false. JavaScript contains a few built-in methods to check whether an array has a specific value, or object. In this article, we'll take a look at how to check if an array includes/contains a value or element in JavaScript. Check Array of Primitive Values Includes a Value Array.includes() Function
CSS At-rules CSS Properties CSS ... Color Values CSS Color Names CSS Web Safe Fonts CSS Aural Properties ... PHP Array Functions PHP String Functions PHP File System Functions PHP Date/Time Functions PHP Calendar Functions PHP MySQLi Functions PHP Filters PHP Error Levels ... In JavaScript if a variable ... Sep 03, 2010 - Hello all, First of all I really hope someone can help, this is probably (I’m hoping) really simple but I just can’t get it! I appreciate all the help thank you! I am working on a form submit button, when it is clicked the first time it is disabled, so multiple submissions cannot occur ... If a function takes a DOM element as a parameter, it can be a string, or a jQuery object , for example. Or why not both? With a small…
Aug 31, 2012 - You’d wrap the snippet around the function you want to check. So say, for example, you have a homepage slider using flexslider or something similar. You might call that function in the global footer or in a JS file that’s shared across pages. Well, if you’re not on the homepage and there is no slider, but you’re calling it in your javascript... Sep 15, 2020 - The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. Jan 04, 2016 - A horrible way of testing if something ... be what is expected. If this.onsubmit were set to the value of 5 or "hello" or anything else in JavaScript that evaluates to true, you are going to get unexpected behavior. – Jason Bunting Apr 28 '09 at 20:22 · The subject of the question asks how to check if something ...
JavaScript Program to Check If a Variable is of Function Type JavaScript Program to Check If a Variable is of Function Type In this example, you will learn to write a JavaScript program that will check if a variable is of function type. To understand this example, you should have the knowledge of the following JavaScript programming topics:
Javascript Mcq Multi Choice Questions Javatpoint
Javascript Localstorage This Tutorial Is Intended As An
Javascript Function Check A Number Is Prime Or Not W3resource
2 Ways To Check If Value Exists In Javascript Object
Checking If An Array Contains A Value In Javascript
How To Check For An Object In Javascript Object Null Check
How Do You Check If An Html Form Checkbox Field Is Checked In
How To Inspect Javascript Function Return Value In Chrome
Should You Use Includes Or Filter To Check If An Array
How To Check If A Value Is A Number In Javascript Anjan Dutta
How To Use If Function In Excel Examples For Text Numbers
Truthy And Falsy Values In Python A Detailed Introduction
Check If A String Contains A Substring In Javascript
Checking If A Key Exists In A Javascript Object Stack Overflow
Javascript Html Form Checking For Non Empty W3resource
Writing Tests Postman Learning Center
3 Ways To Check If An Object Has A Property In Javascript
Identify Functions Using Graphs College Algebra
How Do I Check If An Array Includes A Value In Javascript
Ms Excel How To Use The If Function Ws
Js Check If Array Of Objects Contains Key
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
How To Find Total Sum Of An Array Then Checking If Its Odd Or
How To Check For A Function In Javascript By Dr Derek
Check If An Array Includes A Value Using Javascript
Formula Or Function For If Statement Based On Cell Color
Js Check If Input Is String Code Example
More On Javascript And Usercontrol Hemelix
Solving Everything Be True Freecodecamp Algorithm
Get Started With Debugging Javascript In Microsoft Edge
Javascript Check The Existence Of Variable Geeksforgeeks
2 Ways To Check If Value Exists In Javascript Object
Python Check If Variable Exists In Function Design Corral
Marcus Mengs On Twitter Adding The Realopen Declaration
0 Response to "35 Javascript Check If Value Is Function"
Post a Comment