29 How To Get Textbox Value Using Class Name In Javascript
The default behavior of a submit button obvious – clicking it submits the contents of a form to the server. This seems quite straightforward. So, what could possibly go wrong? Well, what if the user double clicks on the submit button rather than clicking it just the once? 13/1/2020 · JavaScript Get Element By id, name, class, tag value. Javascript access the dom elements by id, class, name, tag, attribute and it’s valued. Here you will learn how to get HTML elements values, attributes by getElementById (), getElementsByClassName (), getElementByName (), getElementsByTagName ().
JavaScript Form Validation. HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted:
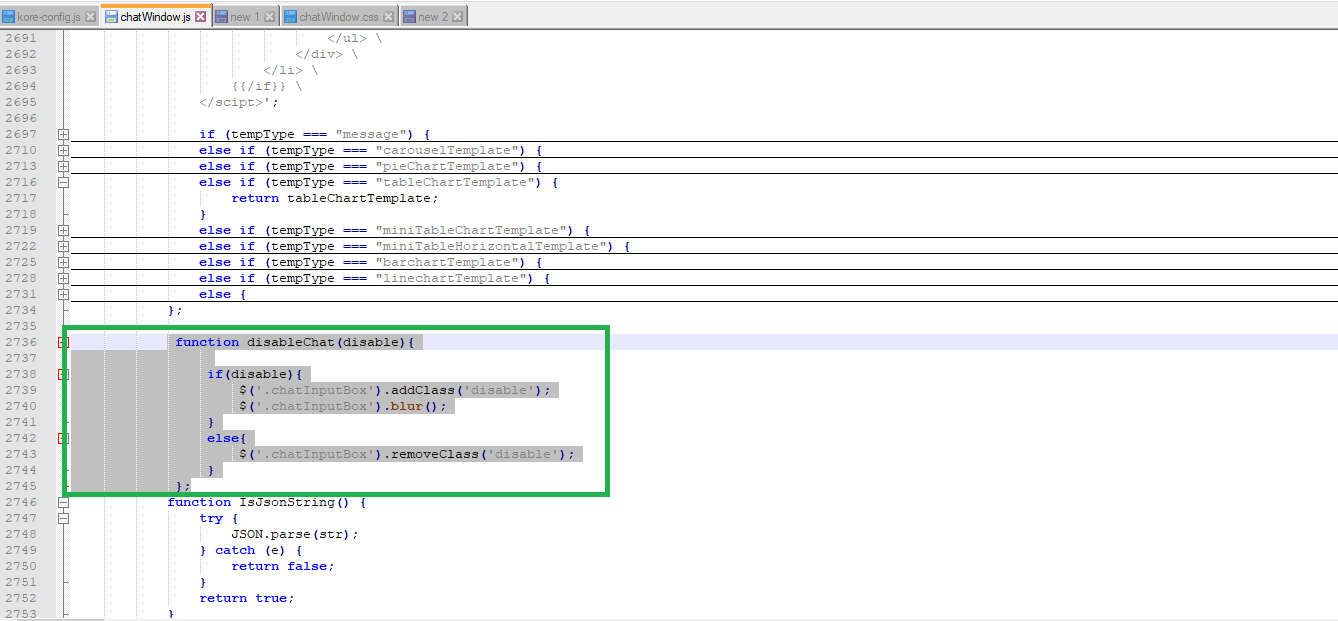
How to get textbox value using class name in javascript. To get the state of a checkbox, whether checked or unchecked, you follow these steps: First, select the checkbox using the selecting DOM methods such as getElementById () or querySelector (). Then, access the checked property of the checkbox element. If its checked property is true, then the checkbox is checked; otherwise, it is not. We can get the value of text input field using various methods in script. There is a text value property which can set and return the value of the value attribute of a text field. Also we can use jquery val () method inside script to get or set the value of text input field. Using text value property: But here in Javascript, the syntax is quite different than the Selenium webdriver. To enter the text into a textbox using javascript, we have two ways: FindElement (Javascript) + EnterText (Javascript) FindElement (WebDriver) + EnterText (Javascript)
10/1/2017 · You can easily get textbox or form elements value using javascript. You can use one of the the following method to get the textbox value. document.getElementById; document.getElmentsByTagName; document.getElementsByName; document.getElementsByClassName; document.reg_frm.fname //where reg_frm is your form name; fname is … Definition and Usage. The name attribute specifies the name of an <input> element. The name attribute is used to reference elements in a JavaScript, or to reference form data after a form is submitted. Note: Only form elements with a name attribute will have their values passed when submitting a form. 19/12/2012 · First if you have all the textbox in a div then you get all the textbox value using children function like this, JavaScript. Copy Code. function GetTextBoxValueOne () { $ ( "#divAllTextBox" ).children ( "input:text" ).each ( function () { alert ($ ( this ).val ()); }); }
This problem can be solved by using input tags having the same "name" attribute value that can group multiple values stored under one name which could later be accessed by using that name. To access all the values entered in input fields use the following method: var input = document.getElementsByName ('array []'); But how do you get CSS values in JavaScript? Turns out, there are two possible ways, depending on whether you're trying to get inline styles or computed styles. Getting inline styles Mar 04, 2016 - Free source code and tutorials for Software developers and Architects.; Updated: 13 Apr 2017
Definition and Usage. The getElementsByName() method returns a collection of all elements in the document with the specified name (the value of the name attribute), as an HTMLCollection object.. The HTMLCollection object represents a collection of nodes. The nodes can be accessed by index numbers. To get the closest input value from clicked element with jQuery, follow the steps −. You need to use closest to find the closest ancestor matching the given. Now, find the input with the given name using the attribute equals selector. The last step is to use val () metjod to retrieve the value of the input. Let's first see how to add jQuery ... 21/3/2013 · I need a function to get the value of the name attribute of each element with class ... I was wondering if using pure Javascript would be faster, or if there is any other ... I tried but couldn't get it to work. I used the same for loop and document.getElementsByClassName('class')[i].name \$\endgroup\$ – Alex Mar 20 '13 at 22:45 ...
Java User Input. The Scanner class is used to get user input, and it is found in the java.util package.. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read Strings: jQuery val () method is used to get the value of an element. This function is used to set or return the value. Return value gives the value attribute of the first element. In case of the set value, it sets the value of the attribute for all elements. This element is mostly used with HTML forms. Example 1: This example is implemented where a ... driver.findElement (By.id ("txtSearchText")).sendKeys ("Selenium"); We can also perform web operations like entering text to the edit box with Javascript Executor in Selenium. We shall use the executeScript method and pass argument index.value='<value to be entered>' and webelement as arguments to the method.
In this tutorial, we are going to find out, that javascript get multiple elements and how to get multiple elements by id using js.Sometimes we need to get the value of the same ID of the multiple elements.But We cannot use the ID to get multiple elements because ID is only used to get the First Element if the same ID is declared to the multiple elements. Jan 02, 2020 - jQuery is a Javascript framework which can simplify coding Javascript for a website and removes a lot of cross browser compatibility issues. This post looks at how to get and set the values of HTML form elements with jQuery. Dec 17, 2015 - This code selects an element with a class of "myCssClass". Since any number of elements can have the same class, this expression will select any number of elements. A jQuery object containing the selected element can be assigned to a JavaScript variable like normal:
Write it Correctly. We have two examples of get input value JavaScript usage. The first one holds a code which will return the value of the property. JavaScript get value of input: textObject.value. The second example can be used for setting the value of the property. As you can see, it contains a value called text, indicating the value of the ... Here, we are defining a function addNumbers () which is getting the value of first input using parseInt (document.getElementById ("value1").value); and second input using parseInt (document.getElementById ("value2").value); values are getting stored in variables val1 and val2. Variable ansD is getting initialized with the element having id ... Description: Selects elements that have the specified attribute with a value ending exactly with a given string. The comparison is case sensitive · attribute: An attribute name
How to assign value to textbox control present in iframe using javascript TextBox value not assigning to variable in asp textbox value empty check without page reload in javascript. To get the value of the text input element, we can use the value property of the text input object: text_val = oText.value; As an example, if we have the following text input element: Next Recommended Reading Get And Set Variable Values From One JavaScript File To Another JavaScript File Using Local Storage LATEST BLOGS Authorization Attribute In ASP.NET Core Web API
In typescript component code, using native html API, document.getElementById returns HTMLInputElement and return value property. getElementById is to select the html element with id selector in javascript this.myusername = (<HTMLInputElement>document.getElementById ("username")).value; formControlName to read input text element on button click The method.getElementsByClassName () returns an array-like object of elements. You need to access an element in the object rather than the object itself (which is why you were seeing [object HTMLInputElement]). For instance, the first object's value property: The getElementsByClassName () method returns a collection of an element's child elements with the specified class name, as a NodeList object. The NodeList object represents a collection of nodes. The nodes can be accessed by index numbers. The index starts at 0.
The prompt () method lets you open a client-side window and take input from a user. For instance, maybe you want the user to enter a first and last name. Normally, you can use an HTML form for user input, but you might need to get the information before sending the form back to your server for processing. There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let’s show you each of them separately and point the differences. The first method uses document.getElementById ('textboxId').value to get the value of the box: 18/7/2020 · In this tutorial, we are going to learn about how to get the value of a input textbox using JavaScript. Consider we have an HTML input field or textbox like this. < input id = " country " type = " text " placeholder = " Country " >
How it works: First, select the Rate button by its id btnRate using the getElementById () method. Second, hook a click event to the Rate button so that when the button is clicked, an anonymous function is executed. Third, call the getElementsByName () in the click event handler to select all radio buttons that have the name rate. This tutorial, Explains about, how to get input values of different HTML form elements using jQuery as follows: we have taken three form elements in our example - input field, radio button and a textarea. To get value of input field. Usually a label would have a value assigned while designing the web page, denoting a name or product description etc. We can set the value of the label dynamically using JavaScript, jQuery or using Asp.Net code behind procedures. Here, I'll show you how you can do this using plain JavaScript.
I am not perfect in Javascript.. I want to show total sum of values entered in qty input boxes in next input box named total without refreshing page. Can anyone will help me to figure it out..? He... Set the value property: textObject.value = text. Property Values: text: It specifies the value of input text field. attributeValue: This parameter is required. It specifies the value of the attribute to add. setAttribute method. This method adds the specified attribute to an element, and set it's specified value. Aug 01, 2019 - DOM: Get Elements by ID, Tag, Name, Class, CSS Selector · By Xah Lee. Date: 2010-10-24. Last updated: 2020-09-07. Here's how to get element in a HTML. ... Return the current script element. [see DOM: Get Current Script Element] Get Element by Matching the Value of the “id” Attribute
JS is a shortcut / short name of Javascript. What is the use of JavaScript (JS)? JS is used for interacting and manipulation with DOM (Document Object Model) elements. Use document.getElementsByClassName ('class_name') [whole_number].value which returns a Live HTMLCollection For example, document.getElementsByClassName ("searchField").value; if this is the first textbox in your page. Method 3: Use document.getElementsByTagName ('tag_name') [whole_number].value which also returns a live HTMLCollection Mar 24, 2014 - hi everyone I have an element like this : ... <...
Dec 04, 2011 - I need to change the class of the input based on text value. When the value is empty it should be default, when there is a value it should change the class to inputtext. .inputno...
Xpath In Selenium Webdriver Tutorial How To Find Xpath
Form Components Form Io Documentation
Javascript Set Input Value By Class Name Change Value
How To Locate Web Element Which Has Multiple Class Names
How To Get Html Textbox Value In Asp Net Dotnettec
Textbox Html Helper In Mvc Application Dot Net Tutorials
Disable Chat Text Box Field For List Entities How To S
Why I Can T Get The Value Of Input In Modal Using Jquery
How To Get Textboxes Values In Mvc4 Created By Jquery
Contact Form 7 Definitive Guide 2021 Supporthost
How To Type Retrieve And Clear Values From The Text Box And
5 Filling In Web Forms Web Scraping Using Selenium Python
How To Get The Value Of Text Input Field Using Javascript
Solved Extracting Value From Field In Form To Be Used In
Enter Only Numbers In Textbox Using Javascript Example
Text Fields Adobe Xd Plugin Reference
Console Utilities Api Reference Chrome Developers
Get Value In Other Text Box By User Inputs In Textbox By
How To Get Previous Textbox Value When Click On Lt A Gt Stack
Javascript Adding A Class Name To The Element Geeksforgeeks
Javascript Preprocessor And Javascript Postprocessor
How To Get Entered Text From Textbox When Value Is Empty In
How To Get The Value Of Text Input Field Using Javascript
Styling Components Styling And Themes Flow Vaadin 14 Docs
Angular 4 Ways To Fetch The Value Of Input In The Controller
How To Disable A Form Field To Prevent User Input
Javascript Basic Calculate Multiplication And Division Of
0 Response to "29 How To Get Textbox Value Using Class Name In Javascript"
Post a Comment