26 How To Add Elements To Array In Javascript
Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function to calculate the sum of values in an array. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
5 Ways To Add An Element At The Last Index Of The Array In
Jul 21, 2019 - A tutorial on how to use `splice()` to add and remove elements from JavaScript arrays
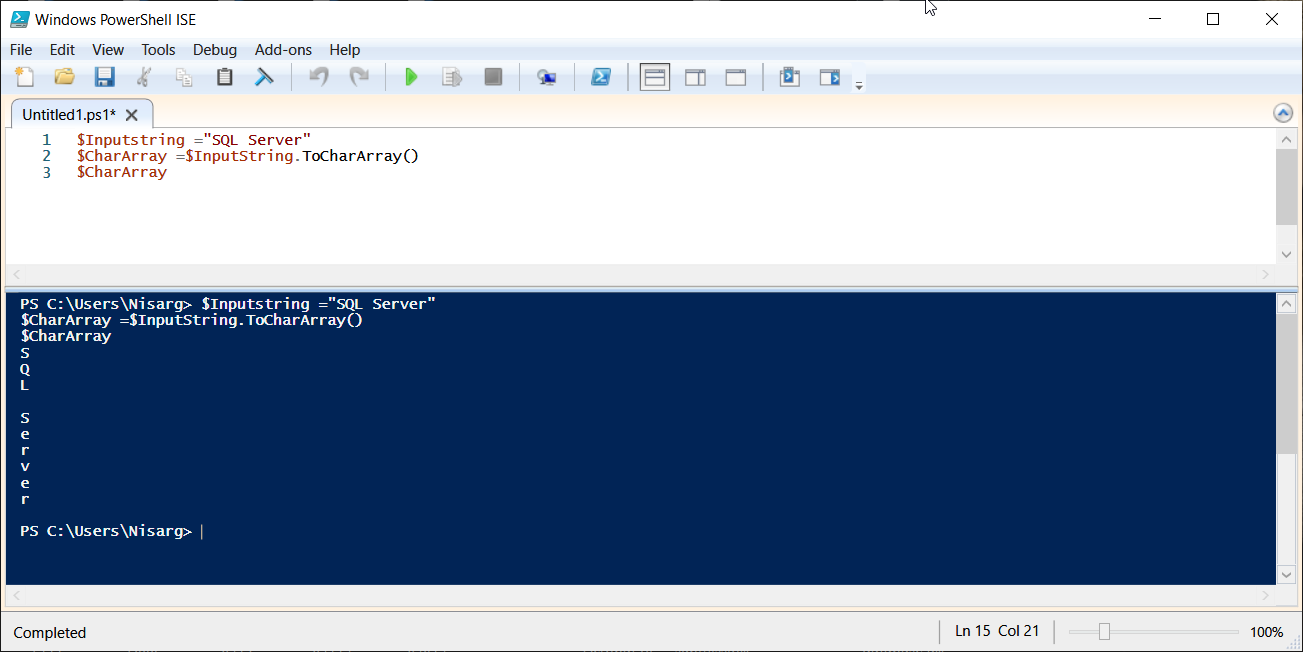
How to add elements to array in javascript. javascript has two inbuilt array methods name unshift() and push(), which is used to add elements or items at first or beginning and last or ending of an array in javascript. This tutorial has the purpose to explain how to add an element at the beginning and ending of an array in javascript using these unshift and push js methods. Get code examples like"how to add multiple elements to A new array javascript". Write more code and save time using our ready-made code examples. Using Array.unshift () The easiest way to add elements at the beginning of an array is to use unshift () method.
Jul 20, 2021 - The concat() method is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array. See the Pen JavaScript: Add items in a blank array and display the items - array-ex-13 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: Write a JavaScript program to compute the sum and product of an array of integers. In JavaScript, by using push () method we can add an element/elemets to the end of an array. EXAMPLE: Adding element to the end of an array. In the above code snippet we have given Id as " myId "to the second <p> element in the HTML code. There is a function myFunction () in the <script> block which is connected to the onclick of the HTML ...
Turning a 2D array into a sparse array of arrays in JavaScript; How to add new value to an existing array in JavaScript? How to compare two arrays in JavaScript and make a new one of true and false? JavaScript; Can we convert two arrays into one JavaScript object? Add two consecutive elements from the original array and display the result in a ... 5 Way to Append Item to Array in JavaScript Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍 18/6/2021 · JavaScript arrays have 3 methods for adding an element to an array: push () adds to the end of the array. unshift () adds to the beginning of the array. splice () adds to the middle of the array. Below are examples of using push (), unshift (), and splice ().
The JavaScript Array.splice () method is used to change the contents of an array by removing existing elements and optionally adding new elements. This method accepts three parameters. The first parameter determines the index at which you want to insert the new element or elements. The second parameter determines the number of elements that you ... The javaScript push() method is used to add new elements to the end of an array. Note: javaScript push() array method changes the length of the given array. Here you will learn the following: How to add the single item of the array? How to add the multiple items of the array? How to push array into array in javaScript? 1 week ago - The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. To access part of an array without modifying it, see slice().
2/8/2019 · // create javascript array let array = [1,2] // add element to array array[array.length] = 3 // add element to array array[array.length] = 4 console.log(array) // result => [1, 2, 3, 4] So in the above example, instead of specifying a constant number we define an Array.length because array size increase as … Sounds like your array elements are Strings, try to convert them to Number when adding: var total = 0; for (var i=0; i<10; i++) { total += +myArray [i]; } Note that I use the unary plus operator ( +myArray [i] ), this is one common way to make sure you are adding up numbers, not concatenating strings. Share. Arrays are considered similar to objects. The main similarity is that both can end with a comma. One of the most crucial advantages of an array is that it can contain multiple elements on the contrary with other variables. An element inside an array can be of a different type, such as string, boolean, object, and even other arrays.
Mar 02, 2017 - If you have just one element to add, you can also try the length method, e.g. array[aray.length] = 'test';. ... Not the answer you're looking for? Browse other questions tagged javascript arrays append or ask your own question. The Push Method The first and probably the most common JavaScript array method you will encounter is push (). The push () method is used for adding an element to the end of an array. Let's say you have an array of elements, each element being a string representing a task you need to accomplish. There are several methods for adding new elements to a JavaScript array. Let's define them. Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) ... The push() method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to ...
The size of the array cannot be changed dynamically in Java, as it is done in C/C++. Hence in order to add an element in the array, one of the following methods can be done: By creating a new array: Create a new array of size n+1, where n is the size of the original array. Add the n elements of the original array in this array. One of the most frequently used javascript methods has to be push. It does a simple thing. Push -> Adds an element to the end of the array. Couldn't be any simpler right. Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array #2 unshift - Insert an element at the beginning of the array #3 spread operator - Adding elements to an array using the new ES6 spread operator
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: JavaScript: Add an element to the end of an array. This is a short tutorial on how to add an element to the end of a JavaScript array. In the example below, we will create a JavaScript array and then append new elements onto the end of it by using the Array.prototype.push() method. In the above program, the splice () method is used to add a new element to an array. In the splice () method, The first argument is the index of an array where you want to add an element. The second argument is the number of elements that you want to remove from the index element.
Last Updated : 06 Mar, 2019. There are several methods for adding new elements to a JavaScript array. push (): The push () method will add an element to the end of an array, while its twin function, the pop () method, will remove an element from the end of the array. If you need to add an element or multiple elements to the end of an array, the ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. To add guitar to the end of the instruments array, type the array's name 1:51. followed by a period, the word push, and a set of parentheses. 1:56. Inside the parentheses, 2:01. you include the element or value you wish to add to the end of the array. 2:02. You can think of the push method as pushing an item 2:08.
Dec 11, 2020 - An array is a linear data structure and arguably one of the most popular data structures used in Computer Science. Modifying an array is a commonly encountered operation. Here, we will discuss how to add an element in any position of an array in JavaScript. An element can be added to an array ... JS this Keyword JS Debugging JS ... number in JavaScript Calculate days between two dates in JavaScript JavaScript String trim() JavaScript timer Remove elements from array JavaScript localStorage JavaScript offsetHeight Confirm password validation Static vs Const How to ... Use forEach function avaialable on array to do it. array.forEach(function(i) { sum += i; }); By this you need not have to worry about the length or terminating conditions for the loop.
The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: How to use JavaScript push, concat, unshift, and splice methods to add elements to the end, beginning, and middle of an array. 1 week ago - Javascript array push() an built-in method that adds a new item to the end of an array and returns the new length of an array.
1 week ago - The push() method adds one or more elements to the end of an array and returns the new length of the array. In the same directory where the JavaScript file is present create the file named code.json. After creating the file to make the work easier add the following code to it: { "notes": [] } In the above code, we create a key called notes which is an array. The Main JavaScript Code. In the upcoming code, we will keep adding elements to the array. HTML Javascript Programming Scripts Adding an element to an array can be done using different functions for different positions. Adding an element at the end of the array This can be accomplished using the push method.
1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Jan 16, 2016 - Given an array [1, 2, 3, 4], how can I find the sum of its elements? (In this case, the sum would be 10.) I thought $.each might be useful, but I'm not sure how to implement it. In the above example, we accessed the array at position 1 of the nestedArray variable, then the item at position 0 in the inner array. Adding an Item to an Array. In our seaCreatures variable we had five items, which consisted of the indices from 0 to 4. If we want to add a new item to the array, we can assign a value to the next index.
You want to explicitly add it at a particular place of the array. That place is called the index. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. To perform this operation you will use the splice () method of an array. There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end.
How To Add Object In Array Using Javascript Javatpoint
Javascript Array Push How To Add Element In Array
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Add Items In A Blank Array And Display The
5 Ways To Add Elements To Javascript Array Simple Examples
How To Add Value To An Array Using Javascript With Source
4 Ways To Convert String To Character Array In Javascript
Create An Array And Populate It With Values In Javascript
5 Ways To Add Elements To Javascript Array Simple Examples
How To Use Bash Array In A Shell Script Scripting Tutorial
Insert An Element In Specific Index In Javascript Array By
Concat In Javascript To Add Or Join Two Or More Arrays Or
Js Add Element To Array Middle Code Example
Using Powershell To Split A String Into An Array
Python List Append How To Add An Element To An Array
How To Append Element To Array In Javascript Tecadmin
How To Append An Item To An Array In Javascript
Javascript Array Insert How To Add To An Array With The
C Program To Insert An Element In An Array
How To Declare An Empty Array In Javascript Quora
Adding And Removing Items From A Powershell Array Jonathan
Adding Elements To Array In Javascript Tech Funda
Javascript Array A Complete Guide For Beginners Dataflair
How To Copy Elements Of One Array To Another In C Codespeedy
How To Add Object In Array Using Javascript Javatpoint
0 Response to "26 How To Add Elements To Array In Javascript"
Post a Comment