34 Javascript Create New Object From Existing Object
5 ways to get a subset of a object properties in javascript. In this short tutorial, We are going to learn How to create a subset of javascript object with examples. Let's have an javascript object. let user = { id:11, name: "frank", salary: 5000, active: true, roles: [ "admin","hr"] }; Because objects in JavaScript are references values, you can't simply just copy using the =. Here are 3 ways for you to clone an object...
Classes In Javascript Dev Community
The Object.create () method creates a new object, using an existing object as the prototype of the newly created object. To understand the Object.create method, just remember that it takes two parameters. The first parameter is a mandatory object that serves as the prototype of the new object to be created.
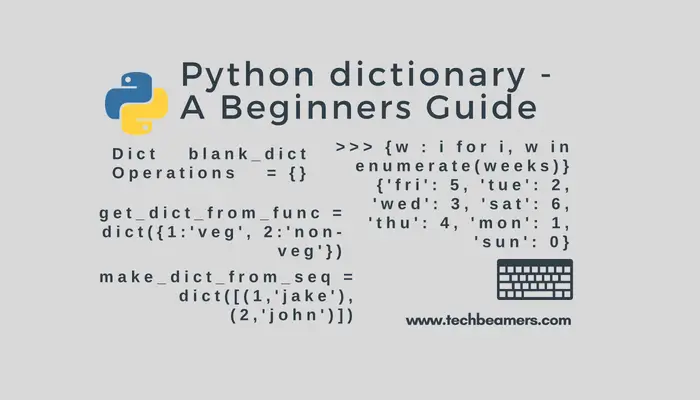
Javascript create new object from existing object. How do I create a new object from an existing object in Javascript , A new object is created that has an exact copy of the values in the original object. But if any of the fields of the object are references to other How to create objects in JavaScript 1. Creating objects using object literal ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Select option(s) that IS NOT an example of deep copying of objects in Javascript. ... In your shallowCopy() function, write an Object.assign() method and pass two arguments to it: A new empty object as the target The source object that you wish to copy ... Property 'forEach' does not exist on type ...
Objects are integral to javascript development and understanding how objects are generated and used is essential. Nov 20, 2018 - Using the spread syntax or Object. ... in JavaScript. Both methodologies can be equivalently used to copy/merge the enumerable properties of an object to another object. Problem with these two approaches is that it will just do the shallow copy. A shallow copy is a bit-wise copy of an object. A new object is created that has an ... Using "Object.create" is the most basic way to create an Inheritance Model in JavaScript. Object.create can be used to create new object using the existing object as a prototype. The existing...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In vanilla JavaScript, there are multiple ways available to combine properties of two objects to create a new object. You can use ES6 methods like Object.assign() and spread operator (...) to perform a shallow merge of two objects. For a deeper merge, you can either write a custom function or use Lodash's merge() method. 6/3/2021 · When you need to append new elements to your JavaScript object variable, you can use either the Object.assign () method or the spread operator. Let me show you an example of using both. First, the Object.assign () method will copy all properties that you defined in an object to another object (also known as source and target objects).
Output: Explanation:In the above code start method was added to the car object and later called by the car.start() and also the stop method was added too after the object was already declared. Creating object with Object.create() method: The Object.create() method creates a new object, using an existing object as the prototype of the newly created object. Jul 10, 2020 - This method is especially useful when you want to create a new object from an already existing object. The Object.create() method takes up to two parameters. The first mandatory parameter is the object that serves as a prototype for the newly created object. The second parameter is an optional ... Javascript answers related to “how to create new object from existing object in javascript without modifying the memory” ... Javascript queries related to “how to create new object from existing object in javascript without modifying the memory”
The Object.create () method The last (but not the least) way to create a JavaScript object is using the Object.create () method. It's a standard method of JavaScript's pre-built Object object type. The Object.create () method allows you to use an existing object literal as the prototype of a new object you create. Apr 22, 2021 - A protip by fr0gs about javascript, programming, and learning. Have you ever wanted to pick properties within an object in javascript and output it straightly as a new object? Sure you probably used lodash before but now you'll be able to do it simply with a…
Code language: CSS (css) In this example, the job and location has the same property country.When we merged these objects, the result object (remoteJob) has the country property with the value from the second object (location).Merge objects using Object.assign() method. The Object.assign() method allows you to copy all enumerable own properties from one or more source objects to a target ... The Object.assign () method only copies enumerable and own properties from a source object to a target object. It uses [ [Get]] on the source and [ [Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties. 21/7/2021 · Write a JavaScript program to create a new object from the specified object, where all the keys are in lowercase. Use Object.keys () and Array.prototype.reduce () to create a new object from the specified object. Convert each key in the original object to lowercase, using String.prototype.toLowerCase ().
Create or Add Dynamic key to Object. So, till this point, we get a rough idea about how we can create and access the objects in javascript. Let's now make it a step further and discuss how we can achieve dynamic key behavior in javascript objects. So, there are 3 ways that can be used to create a Dynamic key to an existing object. 1. person = new Object(); Add a Grepper Answer . Javascript answers related to "how to create new object from existing object in javascript" Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift.
Summary. Objects are assigned and copied by reference. In other words, a variable stores not the "object value", but a "reference" (address in memory) for the value. So copying such a variable or passing it as a function argument copies that reference, not the object itself. All operations via copied references (like adding/removing ... 1. Shallow copy. To shallow copy, an object means to simply create a new object with the exact same set of properties. We call the copy shallow because the properties in the target object can still hold references to those in the source object.. Before we get going with the implementation, however, let's first write some tests, so that later we can check if everything is working as expected. Create a new object on click JavaScript. Create a new Object on Click Javascript . In this Article i will show you how to create a new Object by clicking a Button. After that i will push the newly created Object into an Array. Finally i will demonstrate how to delete the Object from the Array as well.
This article will focus on new object types as well as updated APIs of existing objects in the language. As mentioned in the introduction of the first article ECMAScript 6 - New language improvements in JavaScript, ES6 is designed to fit JavaScript better for writing larger applications. To do so, the language designers added a number of new ... By using Object.create() method, you can create a new object based on any existing object. So in the code above, we simply get the methods on the Book.prototype and assigned them to the prototype property on the Journal constructor. All objects in JavaScript inherit properties and methods from another object called prototype. The prototype property allows us to add new properties and methods to existing object constructors. The new properties are shared among all instances of the specified type, rather than just by one instance of the object.
May 13, 2017 - Quality Weekly Reads About Technology Infiltrating Everything Sep 21, 2020 - Copying objects in JavaScript can be quite daunting especially if you’re new to JavaScript and don’t know your way around the language. Hopefully this article helped you understand and avoid future pitfalls you may encounter copying objects. If you have any library or piece of code that ... So to create a empty file do: var f = new File([""], "filename"); The first argument is the data provided as an array of lines of text; The second argument is the filename ; The third argument looks like: var f = new File([""], "filename.txt", {type: "text/plain", lastModified: date}) It works in FireFox, Chrome and Opera, but not in Safari or ...
Mar 09, 2018 - In this article we will learn multiple ways to create objects in JavaScript like Object Literal, Constructor Function, Object.Create method… 24/8/2017 · const objectConstructor = new Object(); The same data was created using the object constructor method that is initialized with new Object (). Both of these approaches will create an empty object. Using object literals is the more common and preferred method, as it has less potential for inconsistencies and unexpected results. Jul 09, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
For additional guidance on JavaScript in general, you can review our How To Code in JavaScript series. Object.create() The Object.create() method is used to create a new object and link it to the prototype of an existing object. We can create a job object instance, and extend it to a more specific object. 27/8/2021 · How to create an object from two existing objects that are "similar" JavaScript. ... After processing both objects end up populating a new objects with these fields generated dynamically and conditionally depending on the data of the structure and data of the objects above. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
Object.assign () Method. Spread Operator. JSON Methods. Lodash's cloneDeep () Method. Since JavaScript objects are reference types, you can not just use the equal operator ( =) to copy an object. When you create an object in JavaScript, the value is not directory assigned to the variable. Instead, the variable only holds a reference to the value. Often, to create an exact copy of an object in JavaScript, we need to create a new Object from existing objects. There are various methods to achieve this. This article is focused on the different… How do I create a new object from an existing object in Javascript? Ask Question Asked 4 years, 5 months ago. Active 4 years, 5 months ago. ... @T The response is array of objects, I need to extract data and create new array of objects before passing to my component - Rohit Mar 10 '17 at 9:29. basically need to extract values from object ...
The Object.create() method creates a new object, using an existing object as the prototype of the newly created object. Syntax Object . create ( proto ) Object . create ( proto , propertiesObject )
Methods For Deep Cloning Objects In Javascript Logrocket Blog
Object Oriented Programming In Javascript Laptrinhx
Introducing Javascript Ppt Download
Javascript Object Rename Key Stack Overflow
Document Object Model Wikipedia
Creating Objects In Javascript 4 Different Ways Geeksforgeeks
Deep Notes Javascript Javascript Object Oriented And
Object Create To Object Cloning Deep And Shallow Copy Javascript
Adding Extra Object In Existing Object Javascript The
Learn Lightning Create Amp Edit Objects Salesforce Admins
Javascript Array Push How To Add Element In Array
Object Create When And Why To Use Dev Community
Lecture 5 Pdf Science Amp Amp Art Multimedia
Using Javascript Steps In The Trainer
Amazon S3 Batch Operations Aws
A Guide To Object Oriented Programming In Javascript By
4 Ways To Create Powershell Objects Ridicurious Com
How To Append Li To Ul In Javascript Code Example
Python Dictionary Create Append Update Delete Examples
Understanding Classes And Objects
Extending Built In Objects In Javascript
How To Add Object To Built In Object In Javascript Stack
How To Remove A Property From A Javascript Object
4 Different Techniques For Copying Objects In Javascript
New Cross Region Replication For Amazon S3 Aws News Blog
Amazon S3 Features Object Lambda Aws
Creating Objects In Javascript Javascript Is An Object Based
How Can I Add A Key Value Pair To A Javascript Object
Introducing Amazon S3 Object Lambda Use Your Code To
0 Response to "34 Javascript Create New Object From Existing Object"
Post a Comment