31 Javascript Object Of Objects
29/6/2020 · Objects, in JavaScript, is it’s most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript’s primitive data-types(Number, String, Boolean, null, undefined and symbol) in the sense that while these primitive data-types all store a single value each (depending on their types). 10 Javascript Exercises with Objects. Andrei Borisov. May 14, 2020 · 6 min read. Continuing the idea of ten exercises for arrays, I made a collection of tasks for objects. Like the previous one ...
How To Group An Array Of Objects In Javascript By Nikhil
JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through.
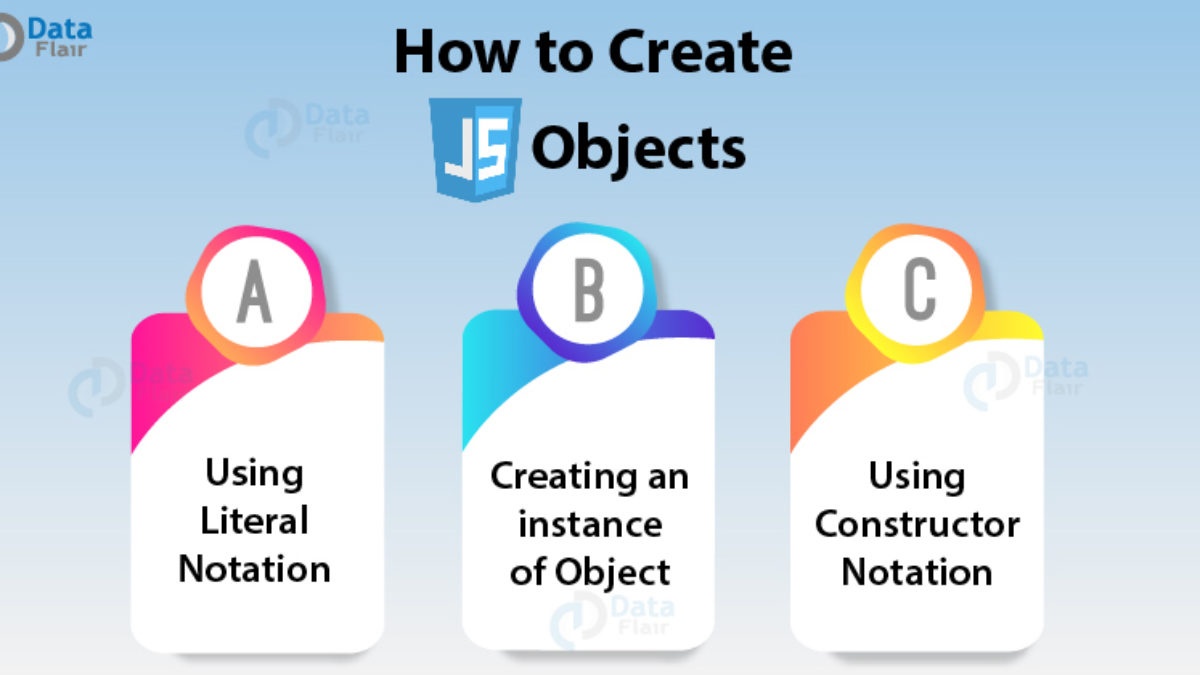
Javascript object of objects. The JavaScript language; Objects: the basics. Objects; Object references and copying; Garbage collection; Object methods, "this" Constructor, operator "new" Optional chaining '?.' Symbol type; Object to primitive conversion; Jun 18, 2014 - You're a little confused about how objects work in JavaScript. The object's reference is the value of the variable. There is no unserialized value. When you create an object, its structure is stored in memory and the variable it was assigned to holds a reference to that structure. Objects. As we know from the chapter Data types, there are eight data types in JavaScript. Seven of them are called "primitive", because their values contain only a single thing (be it a string or a number or whatever). In contrast, objects are used to store keyed collections of various data and more complex entities.
This means that braces have two meanings in JavaScript. At the start of a statement, they start a block of statements. In any other position, they describe an object. Fortunately, it is rarely useful to start a statement with an object in braces, so the ambiguity between these two is not much ... Jan 31, 2021 - In JavaScript, an object is an unordered collection of key-value pairs. Each key-value pair is called a property. The key of a property can be a string and the value of a property can be any valid JavaScript value e.g., a string, a number, an array, and even a function. 5. Write a JavaScript program to get the volume of a Cylinder with four decimal places using object classes. Go to the editor. Volume of a cylinder : V = πr 2 h. where r is the radius and h is the height of the cylinder. Click me to see the solution. 6. Write a Bubble Sort algorithm in JavaScript. Go to the editor.
Methods are actions that can be performed on objects. Object properties can be both primitive values, other objects, and functions. An object method is an object property containing a function definition. JavaScript objects are containers for named values, called properties and methods. 1 week ago - JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined ... JavaScript is an Object Oriented Programming (OOP) language. A programming language can be called object-oriented if it provides four basic capabilities to developers − Encapsulation − the capability to store related information, whether data or methods, together in an object.
JavaScript is designed on a simple object-based paradigm: An object represents a collection of properties; each property is an association between a name/value pair. A value of property can be a function, which is then known as the object’s method. In addition to objects that are predefined ... The Object class represents one of JavaScript's data types. It is used to store various keyed collections and more complex entities. Objects can be created using the Object() constructor or the object initializer / literal syntax. JavaScript Objects A javaScript object is an entity having state and behavior (properties and method). For example: car, pen, bike, chair, glass, keyboard, monitor etc. JavaScript is an object-based language.
A JavaScript object is a variable that can hold many different values. It acts as the container of a set of related values. For example, users of a website, payments in a bank account, or recipes in a cookbook could all be JavaScript objects. In JavaScript, objects can store two kinds of values: 22. You may have as many levels of Object hierarchy as you want, as long you declare an Object as being a property of another parent Object. Pay attention to the commas on each level, that's the tricky part. Don't use commas after the last element on each level: {el1, el2, {el31, el32, el33}, {el41, el42}} The Object.entries() method will only return the object instance's own properties, and not any properties that may be inherited through its prototype. Object.assign() Object.assign() is used to copy values from one object to another. We can create two objects, and merge them with Object.assign().
Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. Objects are same as variables in JavaScript, the only difference is that an object holds multiple values in terms of properties and methods. In JavaScript, an object can be created in two ways: 1) using Object Literal/Initializer Syntax 2) using the Object () Constructor function with the new keyword. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
3 days ago - How TypeScript describes the shapes of JavaScript objects. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript object is unordered collection of properties, where each property has a name and value. A property's value can be a primitive value, object or function. In the last case the property is called a method. You can distinguish three categories of objects: Native objects are instances of object types and objects defined by the ...
JavaScript supports extending data types. JavaScript objects are a great way to define custom data types. An object is an instance which contains a set of key value pairs. Unlike primitive data types, objects can represent multiple or complex values and can change over their life time. Objects in javascript are a group of different data types or objects put together as "key-value" pairs. The "key" part of the object is nothing but the object properties. For example, let us consider we have an object "Student", where its properties are: first_name, last_name, age, student_id, class, etc. 21/11/2020 · Suppose we have an Object of Objects like this −. const obj = { "CAB": { name: 'CBSSP', position: 2 }, "NSG": { name: 'NNSSP', position: 3 }, "EQU": { name: 'SSP', position: 1 } }; We are required to write a JavaScript function that takes in one such array and sorts the sub-objects on the basis of the 'position' property of sub-objects (either in ...
Well, not really. I have simply been withholding information about the way JavaScript objects work. In addition to their set of properties, most objects also have a prototype. A prototype is another object that is used as a fallback source of properties. When an object gets a request for a ... 1 week ago - The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well). Aug 05, 2017 - I'm somewhat new to Javascript, so maybe this is just a noob mistake, but I haven't found anything that specifically helps me while looking around. I'm writing a game, and I'm trying to build an object for the pause menu. One of the things I would like to do is have the buttons on the menu ...
JavaScript functions are a special type of objects, called function objects. A function object includes a string which holds the actual code -- the function body -- of the function. The code is literally just a string. JavaScript object is a non-primitive data-type that allows you to store multiple collections of data. Note: If you are familiar with other programming languages, JavaScript objects are a bit different. You do not need to create classes in order to create objects. Here is an example of a JavaScript object. // object const student = { firstName: 'ram', class: 10 }; 14/5/2020 · Quite literally. JSON stands for JavaScript Object Notation. Creating an object is as simple as this: { "color": "purple", "type": "minivan", "registration": new Date ('2012-02-03'), "capacity": 7 } This object represents a car. There can be many types and colors of cars, each object then represents a specific car.
Object properties are defined as a simple association between name and value. All properties have a name and value is one of the attributes linked with the property, which defines the access granted to the property. Properties refer to the collection of values which are associated with the JavaScript object. 1. Creating objects using object literal syntax. This is really simple. All you have to do is throw your key value pairs separated by ':' inside a set of curly braces ( { }) and your object is ready to be served (or consumed), like below: This is the simplest and most popular way to create objects in JavaScript. 2. Objects in JavaScript are standalone entities that can be likened to objects in real life. For example, a book might be an object which you would describe by the title, author, number of pages, and genre. Similarly, a car might be an object that you would describe by the color, make, model, and horsepower.
The Object.keys () method was introduced in ES6 to make it easier to loop over objects. It takes the object that you want to loop over as an argument and returns an array containing all properties names (or keys). After which you can use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of ... May 16, 2019 - JSON is a textual representation of data, just like XML, YAML, CSV, and others. To work with such data, it first has to be converted to JavaScript data types, i.e. arrays and objects (and how to work with those was just explained). How to parse JSON is explained in the question Parse JSON in ... Dec 24, 2019 - It’s clearly recognizable as to what is happening, so using new Object(), you are really just typing more and (in theory, if not optimized out by the JavaScript engine) doing an unnecessary function call. Also, literal notation creates the object and assigns the property in same line of code ...
Most of the times when we're working with JavaScript, we'll be dealing with nested objects and often we'll be needing to access the innermost nested values safely. Let's take this nested object as an example. const user = {. id: 101, email: 'jack@dev ', personalInfo: {. name: 'Jack', Objects are the standalone entities that hold specific properties and methods. Moreover, the Objects in JavaScript can be created either by directly assigning the literals, using constructors of objects, functions or class, and using prototype method Object.create (). In addition to the above, each object in JavaScript supports specific methods ...
Tools Qa What Are Javascript Objects And Their Useful
What Is An Object How To Javascript Objects Treehouse
Creating A List Of Objects Inside A Javascript Object Stack
A Concise Guide To Javascript Objects
Rewriting Javascript Converting An Array Of Objects To An
Different Ways To Duplicate Objects In Javascript By
Javascript Filter Object How To Filter Objects In Array
Javascript Objects Explore The Different Methods Used To
Getting Started With Javascript Object Scotch Io
How To Merge Amp Concat Objects Using Javascript Object Assign
How To Use Object Destructuring In Javascript
Javascript Objects How To Create Amp Access An Object In
Working With Js Objects And Functions Stack Overflow
Looping Through Array Of Objects Typeerror Cannot Read
Objects In Javascript Geeksforgeeks
Get A Unique List Of Objects In An Array Of Object In
How Can I Remove Properties From Javascript Objects O Reilly
How To Extract The Property Values Of A Javascript Object
Js Array From An Array Like Object Dzone Web Dev
Diving Deeper In Javascripts Objects By Arfat Salman Bits
The Most Common Patterns To Create Objects In Javascript Es5
3 Ways To Clone Objects In Javascript Samanthaming Com
The Most Common Patterns To Create Objects In Javascript Es5
What Is An Object In Javascript Example Codez Up
Seven Ways Of Creating Objects In Javascript For C
Fill Object Of Objects Javascript Code Example
The Chronicles Of Javascript Objects By Arfat Salman Bits
0 Response to "31 Javascript Object Of Objects"
Post a Comment