23 Javascript Check If Number Is Integer
Number.isNaN() is different from the global isNaN() function. The global isNaN() function converts the tested value to a Number, then tests it. Number.isNaN() does not convert the values to a Number, and will not return true for any value that is not of the type Number. Tip: In JavaScript, the value NaN is considered a type of number. javascript check if a value is a integer number; javascript check if variable is number; check if input is a number javascript; integer check in javascript; check if a string contains digits js; js is variable int; how to check if a string is an integer javascript; check if string only contains integer digits numbers javascript; check if ...
How To Find Even Numbers In An Array Using Javascript
Determining the sign of a number is super easy now with ES6's Math.sign! It will indicate whether the number is positive, negative or zero...
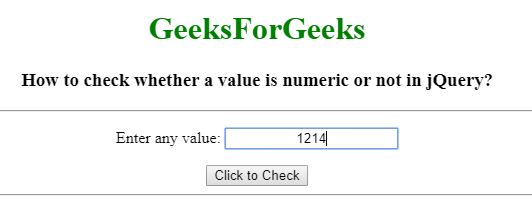
Javascript check if number is integer. JavaScript: Check if a number is a whole number or has a decimal place Last update on February 26 2020 08:09:04 (UTC/GMT +8 hours) JavaScript Math: Exercihse-38 with Solution javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". isNaN () - Stands for "is Not a Number", if variable is not a number, it return true, else return false. typeof - If variable is a number, it will returns a string named "number".
26/5/2014 · Checking via the remainder operator # One can use the remainder operator (%) to express the fact that a number is an integer if the remainder of dividing it by 1 is 0. function isInteger(x) { return x % 1 === 0; } I like this solution, because it is quite self-descriptive. 19/6/2021 · Here we see how to check if a number is an integer in javascript. The Number.isInteger() method in JavaScript is used to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it returns false. Syntax: Number.isInteger(value) Parameters: This method accepts a single parameter value which specifies the number which the user wants to … parseInt (): We can also use the function parseInt () to check if the value is an integer or not, it parses a string argument and returns an integer of the specified radix (the base in mathematical numeral systems). Let's write an example of the same. let num = 500; if (num === parseInt(num, 10))
JavaScript Number isInteger() method. The JavaScript number isInteger() method determines whether the given value is an integer. It returns true if the value is an integer, otherwise it returns false. Syntax. The isInteger() method is represented by the following syntax: Example 6: check to see if number is a decimal javascript · The Number. isInteger() method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false. Example 4: check to see if number is a decimal javascript · The Number. isInteger() method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false.
Output: Yes No Yes No. To check a number is palindrome or not without using any extra space. Method #2:Using string () method. When the number of digits of that number exceeds 10 18, we can't take that number as an integer since the range of long long int doesn't satisfy the given number. The Number.isInteger () method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false. JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... Write a javascript function named is_integer which checks if the passed argument is an integer. You can use any mathematical operator or functions defined in the Math object.
The JavaScript Number.isInteger () method determines whether the passed value is an integer. The syntax of the isInteger () method is: Number.isInteger (value) The isInteger () method is called using the Number class name. Javascript Web Development Front End Technology Object Oriented Programming. Let's say we have the following variables −. var value1 = 10; var value2 = 10.15; Use the Number () condition to check that a number is float or integer −. Number (value) === value && value % 1 !== 0; } Because the parsing functions always convert to string first and then attempt to parse that string, which would be a waste if the value already is a number. ... javascript check if string is integerjavascript is floatjquery is integeris integer javajavascript check if number is decimalnumb...
The return value of text input field is string. First try parseInt () to convert the value to integer. If the type casting fails, it parseInt () will return NaN, so we can use isNaN () to check the result of conversion. The following is demo of above idea: // program to check if a number is a float or integer value function checkNumber(x) { let regexPattern = /^-?[0-9]+$/; // check if the passed number is integer or float let result = regexPattern.test(x); if(result) { console.log(`${x} is an integer.`); } else { console.log(`${x} is a float value.`) } } checkNumber(44); checkNumber(-44); checkNumber(3.4); checkNumber(-3.4); Write a javascript function named ... argument is an integer. You can use any mathematical operator or functions defined in the Math object. ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript documentation to find a method on the built-in Number object that checks if a number ...
Jul 21, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Floor rounds input to the larger number not greater than the input so for example 24.12 results 24 , but 24.12 is not an integer. Try checking if contains . or , (in my country for example decimals are separeted with comma) image. 1009×591 62.1 KB. Jermartyno May 5, 2021, 7:01am #3. Apr 28, 2021 - Another approach is to check for a remainder value when the given number is divided by 1. To validate for numeric value first, place an additional check with unary plus (+) operator, as shown below: ... Alternatively, you can use the JavaScript native parseInt() method, which returns an integer ...
May 21, 2020 - Delphi queries related to “js how to check if number is integer” · how to check in javascript value is integer or not ... Write a javascript function named is_integer which checks if the passed argument is an integer. You can use any mathematical operator or functions defined in the Math ... If the value of a variable is an integer, then the numeric value of it's parseFloat () and parseInt () equivalents will be the same, so: function is_int (value) { if ((parseFloat (value) == parseInt (value)) && !isNaN (value)) { Use the parseInt () Function to Check Whether a Given String Is a Number or Not in JavaScript The parseInt () function parses a string and then returns an integer. It returns NaN when it is unable to extract numbers from the string.
See the Pen javascript-math-exercise-15 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to round a number to a given decimal places. Next: Write a JavaScript function to check whether a variable is numeric or not. Jul 20, 2021 - If the target value is an integer, return true, otherwise return false. If the value is NaN or Infinity, return false. The method will also return true for floating point numbers that can be represented as integer. Nov 19, 2020 - Infinity is indeed a value in JavaScript, representing mathematical infinity (∞). Be prepared for when it pops ups in… ... To check if variable A is an integer I could use the loose equality operator == to see if the parsed value equals itself.
The expression "number" - 32223 results in NaN as happens when you perform a subtraction operation between a string and number. JavaScript typeof Examples. The following code snippet shows the type check result of various values using the typeof operator. JavaScript isInteger () is an inbuilt method that resolves whether the passed value is an integer. The isInteger () method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise, it returns False. The isInteger () method is not supported in Internet Explorer 11 and earlier versions. We have various ways to check if a value is a number. The first is isNaN (), a global variable, assigned to the window object in the browser: const value = 2 isNaN(value) //false isNaN('test') //true isNaN({}) //true isNaN(1.2) //false If isNaN () returns false, the value is a number.
Example 1: javascript is number an integer Number.isInteger(value) //returns a Boolean Example 2: javascript check if a value is a integer number Number.isInteger(12 Write a javascript function named ... argument is an integer. You can use any mathematical operator or functions defined in the Math object. ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript documentation to find a method on the built-in Number object that checks if a number ... 19/6/2020 · Number isinteger JavaScript Method is used to know whether a value an integer or not? You have to pass the value into an isinteger () function and its return boolean value, true if the value is of the type Number. Otherwise, it returns false.
Aug 25, 2020 - JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... how to write a function that checks the number of strings in the string and returns it in javascript In it Number.isInteger () method returns true if the argument is an integer, otherwise returns false. If the value is NaN or Infinity, return false. Note: The method will also return true for floating point numbers that can be represented as integer like 5.0 (as it is exactly equal to 5) MySQL query to fetch records where decimal is a whole number; Check if input is a number or letter in JavaScript? Check if a number is a Krishnamurthy Number or not in C++; Check whether a number is a Fibonacci number or not JavaScript; C# program to check if there are K consecutive 1's in a binary number
2/9/2019 · In this tutorial explain, how you can check if a number is an integer value or not in javascript. You can easily check if a number is an integer or not in javascript using the number.isInteger() function. Number.isInteger() Method. In JavaScript Number.isInteger() method works with numbers, it will check whether a value an integer. This method returns true if the value is of the type Number and an … Sep 04, 2020 - This method is best suited for when you know you have a number and would to check if it is a NaN value, not for general number checking. ... The typeof() function is a global function that accepts a variable or value as an argument and returns a string representation of its type. JavaScript has ...
How To Check If A String Is An Integer In Java Video
Create A Javascript Program That Accepts Integers Chegg Com
How To Check If A Number Is A Float In Python Quora
How To Check If A Value Is A Number In Javascript
Check If A Variable Is An Integer Or A Float In Javascript
Check If Is String Code Example
How To Convert A String To An Integer In Javascript Stack
Convert A Negative Number To A Positive One In Javascript
How To Draw A Flowchart To Check If A Number Is Positive Or
Check Number Integer Or Float In Java
C Program To Check If A Number Is Prime Or Not
How To Check Whether A Value Is Numeric Or Not Using Jquery
Loop To Check If Number In Var Is Positive Or Negative
Javascript Numbers Get Skilled In The Implementation Of Its
How To Determine If A Number Is Odd In Javascript Stack
Python Check Number Odd Or Even Javatpoint
Check If Number Is Even Javascript Code Example
Javascript Math Check Whether A Value Is An Integer Or Not
Javascript Algorithms Integer To Roman Leetcode By
Javascript Basic Check Whether Two Given Integer Values Are
How To Check If A Number Is Integer On C Quora
Really good explanation of the topic. Appreciated.
ReplyDeleteAlso if you want to learn more about javascript i would highly recommend these articles as well.