21 Javascript Random Number In Range
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript has built-in methods to generate a single, random number. You can leverage this feature to create your own method generating a random number in a range between two other numbers.
The random number is the number generated by the computer system of any such devices which we cannot predict. Developers or the coder use this random number generation to fulfill their business needs. Programmatically, this number can be bounded within the specified range or without putting any boundaries of the range.
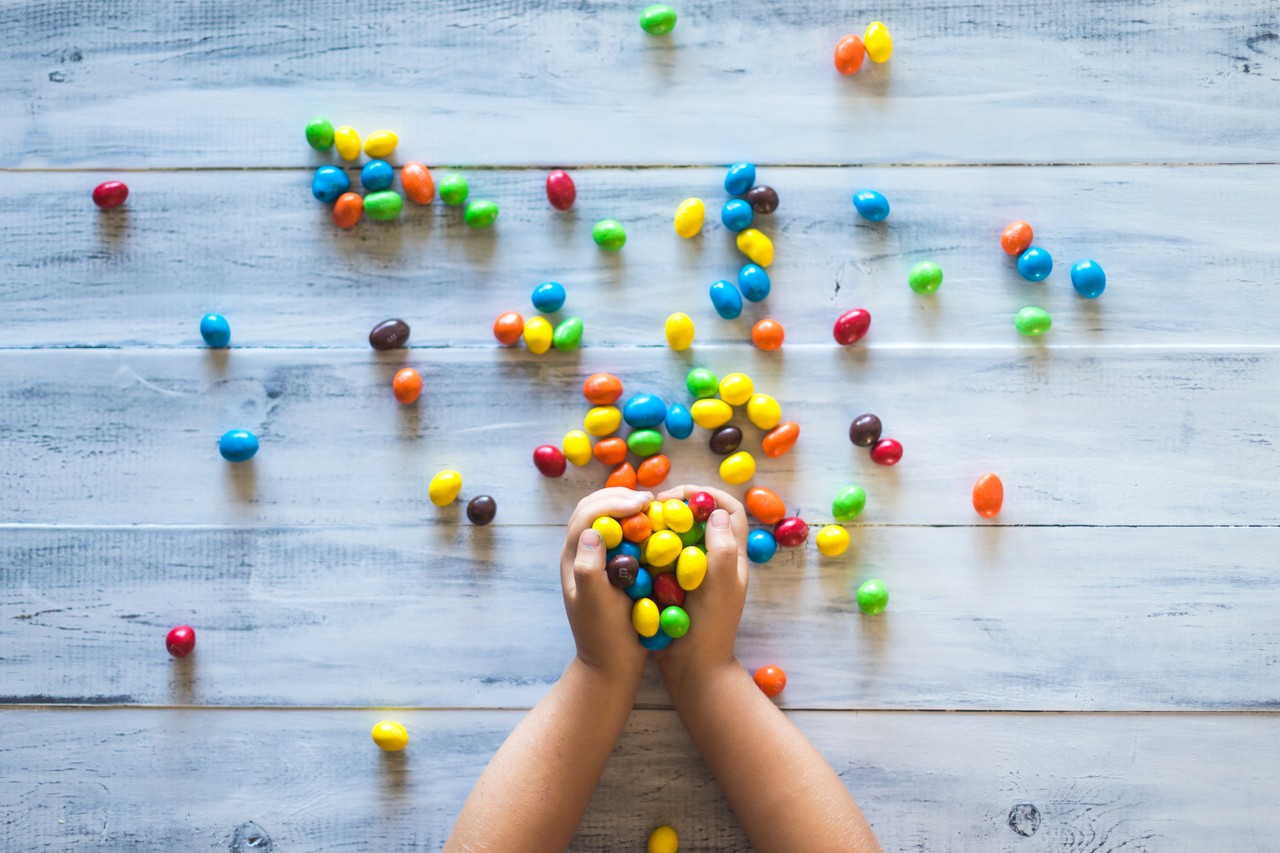
Javascript random number in range. 18/9/2020 · Generating range of numbers 1…n in SAP HANA; Generating a range of numbers in MySQL? Generating a random number that is divisible by n in JavaScript; Armstrong numbers between a range - JavaScript; Generating random hex color in JavaScript; Generating Random Prime Number in JavaScript; Get Random value from a range of numbers in JavaScript ... how to do a random number generator in js. C1 = Math.floor (Math.random () * 100 + 1); random number between 0 and 2 javascript. javascript random number between 1 and 300. random number in jquery. generate random number between 1 and 100 javas. generate random number between 1 and 100 javascript. rand function js. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
17/7/2021 · See the Pen javascript-basic-exercise-1-34 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript program to get a random number in the specified range. Next: Write a JavaScript program to get an array of given n random integers in the specified range. Nov 19, 2020 - Whether for performance testing or any other purpose, it is sometimes useful to generate pseudorandom numbers. Jul 20, 2021 - Note that as numbers in JavaScript are IEEE 754 floating point numbers with round-to-nearest-even behavior, the ranges claimed for the functions below (excluding the one for Math.random() itself) aren't exact. If extremely large bounds are chosen (2^53 or higher), it's possible in extremely ...
May 17, 2020 - Generate a random number in a range. The way we all wish Math.random worked. 29/11/2019 · randomRange should use both myMax and myMin, and return a random number in your range. You cannot pass the test if you are only re-using the function ourRandomRange inside your randomRange formula. You need to write your own formula that uses the variables myMax and myMin . 22/4/2019 · This article describes how to generate a random number using JavaScript. Method 1: Using Math.random() function: The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range. Syntax: Math.random();
Learn how to make a random number in JavaScript: Using Math.random() How to choose numbers up to a given number (e.g. up to 100) A way to randomly generate two numbers in a range (e.g. 32-50) How to improve randomness with Crypto.getRandomValues() Aug 28, 2018 - A short guide to .ceil, .floor, and .round. “Using Math.random() in JavaScript” is published by Joe Cardillo. A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded):
Random Number between Range in JavaScript December 28, 2020 Red Stapler 0 Math.random () is a function that returns a pseudo-random floating numbers between 0 and 1 (0 is inclusive, 1 is exclusive) We usually multiply the result with other numbers to scale the randomized value. 2. Generating Random Numbers in a Range. 2.1. Math.random. Math.random gives a random double value that is greater than or equal to 0.0 and less than 1.0. Let's use the Math.random method to generate a random number in a given range [min, max): public int getRandomNumber(int min, int max) { return ( int) ( (Math.random () * (max - min)) + min); } In JavaScript, you can use the Math. random () function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive). If you want to get a random number between 0 and 20, just multiply the results of Math.random () by 20: To generate a random whole number, you can use the following Math methods along with Math.random ():
Algorithm to Generate Random Number in a Specified Range in JavaScript { { % step %}} Generate a number between the range 0 and 1 and store it in a variable named rand. Calculate the total range range as max-min+1. Apr 16, 2021 - The solution that Javascript, and ... generation. Javascript random numbers start from a hidden internal value called a “seed.” The seed is a starting point for a hidden sequence of numbers that are uniformly distributed throughout their possible range.... Random Number from range in JavaScript. GitHub Gist: instantly share code, notes, and snippets.
JavaScript fundamental (ES6 Syntax): Exercise-33 with Solution. Write a JavaScript program to get a random number in the specified range. Use Math.random() to generate a random value, map it to the desired range using multiplication. Sample Solution: JavaScript Code: * Returns a random integer between min (inclusive) and max (inclusive). * The value is no lower than min (or the next integer greater than min * if min isn't an integer) and no greater than max (or the next integer * Using Math.round() will give you a non-uniform distribution! function getRandomInt(min, max) { 1. Get a random number between two numbers including min and max numbers. By the use of the below method, you will get the random number between two numbers including min and max values. function getRandomNumber(min, max) { return Math.floor(Math.random() * (max - min + 1) + min); } getRandomNumber(0, 50); Copy.
Generate Random Whole Numbers within a Range Instead of generating a random number between zero and a given number like we did before, we can generate a random number that falls within a range of two specific numbers. To do this, we'll define a minimum number min and a maximum number max. Here's the formula we'll use. Take a moment to read it and try to understand what this code is doing ... If the random number obtained like this is less than the lastRandom, ... Browse other questions tagged javascript random or ask your own question. ... Iterator producing unique random numbers in a specified range. 4. Testing random number generator function. 6. range of random number in js generate random no 100 to 1 in javascript and show it to a div get random number between 1 to 100 in javascript to generate random number between 0 and 6 in javascript
Generating Random Numbers in JavaScript Math.random () in JavaScript generates a floating-point (decimal) random number between 0 and 1 (inclusive of 0, but not 1). Let's check this out by calling: console.log (Math.random ()) Get code examples like"javascript get random number in range". Write more code and save time using our ready-made code examples. JavaScript: get a random number from a specific range - random.js
Apr 29, 2020 - Generate integers, floats and BigInts within a certain range. Here, we can see that the random value produced by Math.random() is scaled by a factor of the difference of the numbers. Then it is floored using Math.floor() to make it an integer. Finally, it is added to the smaller number to produce a random number between the given range. java random number between 1000 and 9999 - How do I generate random integers within a specific range in Java? use-strict - strict type checking javascript - What does "use strict" do in JavaScript, and what is the reasoning behind it?
Sep 07, 2020 - The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive).This random number can then be scaled according to the desired range. 1/4/2021 · “The Math.random () function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range.” — MDN Web Docs Below is a function that finds a random number between two arguments (min and max): Apr 01, 2018 - For many situations ranging from coin toss operations to procedural animations, you will want to work with numbers whose values are a bit unpredictable. You will want to work with numbers that are random. Generating a random number is easy. It is built-in to JavaScript and exposed via the infamous ...
24/3/2020 · Math.random() Mathis Javascript’s math object, which is just a storage bin for various mathematical functions. For example, Math.PI returns pi, and Math.round(x) rounds x to the nearest integer. Math.random() gives us a decimal value between 0 and 1. Let’s try: Math.random() // 0.0860233629852345 Math.random() // 0.8732168857142002 Math.random() // 0.3644885309435295 Math.random() // … Courtesy of IonuÈ› G. Stan here.. What I want to know is if you can generate a better random number in a range using crypto.getRandomValues() instead of Math.random(). I would like to be able to generate a number between 0 and 10 inclusive, or 0 - 1, or even 10 - 5000 inclusive. Tweet A formula for generating a random number within a given range using JavaScript is as follows: Math.floor (Math.random () * (UpperRange - LowerRange + 1)) + LowerRange; So to generate a random number between 25 and 75, the equation would be
In this article, we have learned to get random numbers in Javascript and tried several techniques to get the desired results. We have also learned to make a custom function in which we can provide the range of numbers and get the random numbers between that ranges. Math.random() returns a random floating-point number ranging from 0 to less than 1 (inclusive of 0 and exclusive of 1) The above program will show an integer output between min (inclusive) to max (inclusive).
Generate Random Number In Range In Javascript
How To Obtain Random Numbers Within A Range Using Javascript
Random Colors Generating Random Color With Single Line Of Js
How To Select A Random Element From Array In Javascript
Create A Random Number Generator With Javascript Orangeable
Javascript Fundamental Es6 Syntax Get A Random Number In
Creating A Random Number Inside A Range In Javascript Awsm
Generate A Random Number In Range With Javascript Node Js
Random Number Generator And Checker
Java Generate Random Integers In A Range Mkyong Com
Generating Random Numbers In Javascript
Github D3 D3 Random Generate Random Numbers From Various
Javascript Math Random Method Explained
Generate Random Integer Between Two Numbers Using Javascript
How To Get A Number Of Random Elements From An Array Stack
Generate Random Number With Less Codepad
Random Number In Javascript Practical Example
Generate Random Numbers In Javascript Using Crypto Without
React Native Generate Random Number Between 1 To 100 In
Javascript Random Number Generator Coding Commanders
0 Response to "21 Javascript Random Number In Range"
Post a Comment