26 Javascript Check If Null
Answer: Use the equality operator ( ==) In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null is a special assignment value, which can be assigned to a ... Unless you want to specifically check for undefined or null. In which case val != null will return true for undefined or null but not for other falsey values like 0 . - Eric H Mar 8 '19 at 20:52
How Can I Determine If A Variable Is Undefined Or Null
javascript check if input value is null or empty; javascript check if input value is null; verify that input is not empty; javascipt function inut is not em; javascript if field blank; how to check if input field is empty javascript; js empty validation; js code that if filed is has text promot thank you; input value is filled then enter in ...
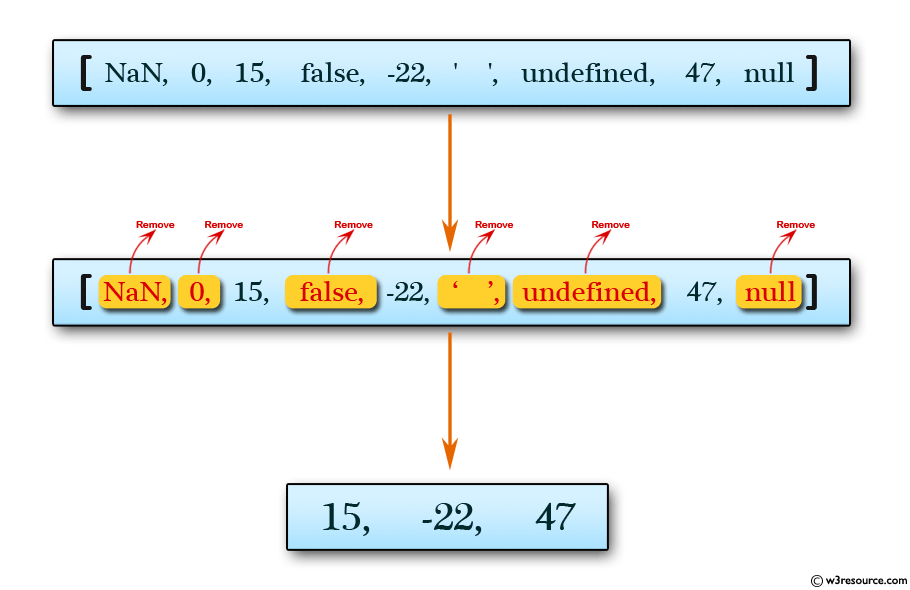
Javascript check if null. Javascript - checking a lookup value for null failing. ... Problem is when the retrieved value is null, I am checking if the value is null or not before proceeding further but it ignores the if condition and enters the loop even though the value is null. Country is a lookup field. Can someone please guide me through this? (use === null to check only for nulls values, and == null to check for null and undefined) console.log(myVar == null ? myVar.myProp : 'fallBackValue'); in some cases, like yours, when your variable is supposed to hold an object, you can simply use the fact that any object is truthy whereas null and undefined are falsy values : 27/11/2017 · javascript check if null or white space or no space . Posted by: admin November 27, 2017 Leave a comment. Questions: I need to check to see if a var is null or has any empty spaces or for that matter just blank (“”) I have the following but not working:
The value null is written with a literal: null.null is not an identifier for a property of the global object, like undefined can be. Instead, null expresses a lack of identification, indicating that a variable points to no object.In APIs, null is often retrieved in a place where an object can be expected but no object is relevant. To check for null values in JavaScript, you need to check the variable value with null. Use the JavaScript strict equality operator to achieve this. Example. You can try to run the following code to check whether the value of the variable is null or not − By using the ?. operator instead of just ., JavaScript knows to implicitly check to be sure obj.first is not null or undefined before attempting to access obj.first.second. If obj.first is null or undefined, the expression automatically short-circuits, returning undefined.
Check for NaN with self-equality. "NaN, and only NaN, will compare unequal to itself.". — MDN Docs. In JavaScript, the best way to check for NaN is by checking for self-equality using either ... One aspect of JavaScript development that many developers struggle with is dealing with optional values. What are the best strategies to minimize errors caused by values that could be null… Checking for the null values before executing the code limits a number of errors. To check the Null values in JavaScript, do the following: 1.First declare the variable. Type the below lines into your JavaScript code: var myVa = null; This will allocate memory and will define the variable. 2. Evaluate if the variable is null.
20/9/2013 · Hi there , I have used follwing code to check for null values of field : <script> function validateInput(ReceiveInputIDCompany){var inputValueCompany = document.getElementById(ReceiveInputIDCompany).value; There are already many answers exist to check for undefined, null, empty or blank variable in JavaScript and jQuery still sometimes they mislead if not understood well. So this article is a straightforward manner to determine if a variable is undefined or has null/empty value or whatsoever. Check undefined, null, empty or blank variable in ... In JavaScript, one of the everyday tasks while validating data is to ensure that a variable, meant to be string, obtains a valid value. This snippet will guide you in finding the ways of checking whether the string is empty, undefined, or null. Here we go. If you want to check whether the string is empty/null/undefined, use the following code:
This check is actually not safe. If myvar is 0, false or any other falsy value the test will fail (if you only intend to check for not null). So only use this to check for not null if the variable can never have another falsy value. - Werzi2001 Sep 19 '13 at 16:03 JavaScript checking null | Using equality operator. Posted July 13, 2020. May 15, 2021. by Rohit. In JS null value means no value or absence of a value. Checking null in Javascript is easy, you can use the equality operator == or strict equality operator === (also called identity operator). Check if JavaScript array is empty, null or undefined in 4 ways June 21, 2020 It is very easy to check if JavaScript array or object is empty but might need additional checks if you want to also check for null or undefined.
C hecking for null is a common task that every JavaScript developer has to perform at some point or another. The typeof keyword returns "object" for null, so that means a little bit more effort is required. Comparisons can be made: null === null to check strictly for null or null == undefined to check loosely for either null or undefined. There are 7 falsy values in JavaScript - false, 0, 0n, '', null, undefined and NaN. A nullish value consists of either null or undefined. This post will provide several alternatives to check for nullish values in JavaScript. To check for null variables, you can use a strict equality operator (===) to compare the variable with null. The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. This can be contrasted with the logical OR (||) operator, which returns the right-hand side operand if the left operand is any falsy value, not only null or undefined.
how to do null check in javascript; js check string empty null undefined; javascript check if null or empty; typescript check for null or undefined but not zero; checking if a variable is empty javascript; object.isnotnull javascript; javascript vail not noll or undefined; return false if not defined js; typescript return value or empty if null In JavaScript null is "nothing". It is supposed to be something that doesn't exist. It is supposed to be something that doesn't exist. Unfortunately, in JavaScript, the data type of null is an object. Using null parameters in JavaScript allows programmers to define a variable without assigning a value to it. Null values are used in simple variables like integers and strings, and they are used for objects on a Web page. However, null values become troublesome for calculations or object manipulation.
Get code examples like"javascript check if undefined or null or empty string". Write more code and save time using our ready-made code examples. JavaScript isNull | How to Check for null. The value null represents the object value or variable value is don't have any value ("No value"). There is no isNull function in JavaScript script to find without value objects. However you can build your own isNull () function with some logics. In newer JavaScript standards like ES5 and ES6 you can just say. > Boolean (0) //false > Boolean (null) //false > Boolean (undefined) //false. all return false, which is similar to Python's check of empty variables. So if you want to write conditional logic around a variable, just say.
JavaScript Object.is() method to check null value. Javascript Object is() an inbuilt method that checks whether two values are the same value. The ES2015 method Object.is() differs from the strict === and loose == equality operators in how it checks for NaN and negative zero -0 values. To check null values, the behavior of Object.is() is the ... The variable is neither undefined nor null The variable is neither undefined nor null The variable is undefined or null The variable is undefined or null. The typeof operator for undefined value returns undefined. Hence, you can check the undefined value using typeof operator. Also, null values are checked using the === operator. @HunanRostomyan Good question, and honestly, no, I do not think that there is. You are most likely safe enough using variable === null which I just tested here in this JSFiddle.The reason I also used ` && typeof variable === 'object'` was not only to illustrate the interesting fact that a null value is a typeof object, but also to keep with the flow of the other checks.
17/11/2019 · To check if a string is empty or null or undefined in Javascript use the following js code snippet. var emptyString; if ... If the string is not undefined or null and if you want to check for empty string in Javascript we can use the length property of the string prototype as shown below. The if condition will execute if my_var is any value other than a null i.e . If my_var is undefined then the condition will execute. If my_var is 0 then the condition will execute. If my_var is " (empty string) then the condition will execute. … This condition will check the exact value of the variable whether it is null or not. Example 2:
How To Check For Null In Javascript By Dr Derek Austin
How To Check For An Object In Javascript Object Null Check
How To Check For An Undefined Or Null Variable In Javascript
How To Check An Object Is Empty Using Javascript Geeksforgeeks
How Can I Check For An Empty Undefined Null String In
How To Check Empty Undefined Null String In Javascript
Mysql Is Null Amp Is Not Null Tutorial With Examples
Concise Way To Check If Value Null Compiled Into More
How To Check For Empty Undefined Null String In Javascript
Javascript Check If Empty Code Example
Sql Query To Select All If Parameter Is Empty Or Null
How To Check If A Variable Is Of Type Object In Javascript
Javascript Array Remove Null 0 Blank False Undefined And
How To Check Null In Javascript Stackover Q Amp A
How To Check For An Object In Javascript Object Null Check
How To Check Null Value In Odata Filter Of Get Items Action
How To Check For Null In Javascript By Dr Derek Austin
How To Check For Null In Javascript By Dr Derek Austin
Javascript Array Remove Null 0 Blank False Undefined And
How To Check Null In Java With Pictures Wikihow
How Can I Check For An Empty Undefined Null String In
Sql Null Functions Isnull Ifnull Combine Amp Nullif
How To Check Null In Java With Pictures Wikihow
Check If Javascript Array Is Empty Null Or Undefined In 4 Ways
0 Response to "26 Javascript Check If Null"
Post a Comment