31 Check Null Input Javascript
Checking if an input is empty with JavaScript 19th Dec 2018. Last week, I shared how to check if an input is empty with CSS. Today, let’s talk about the same thing, but with JavaScript. It’s much simpler. Here’s what we’re building: Events to validate the input The preferred way to check if something is null is by using the strict equality operator(===). function isNull(input) { return input === null; } Make sure not to use the == by mistake. Using the == in place of === will result in misleading type detection. How to Detect an Array in JavaScript
Javascript Check Value Is Null Or Empty Code Example
7/11/2017 · See code below. I declared a variable called empqty, which should be the name of the field to check if there are values, if the data is empty (null) then the tabular report should hide columns 7 and 8. Any assistance would be greatly appreciated! Thank you! <script language="javascript" type="text/javascript"> var stl='none';
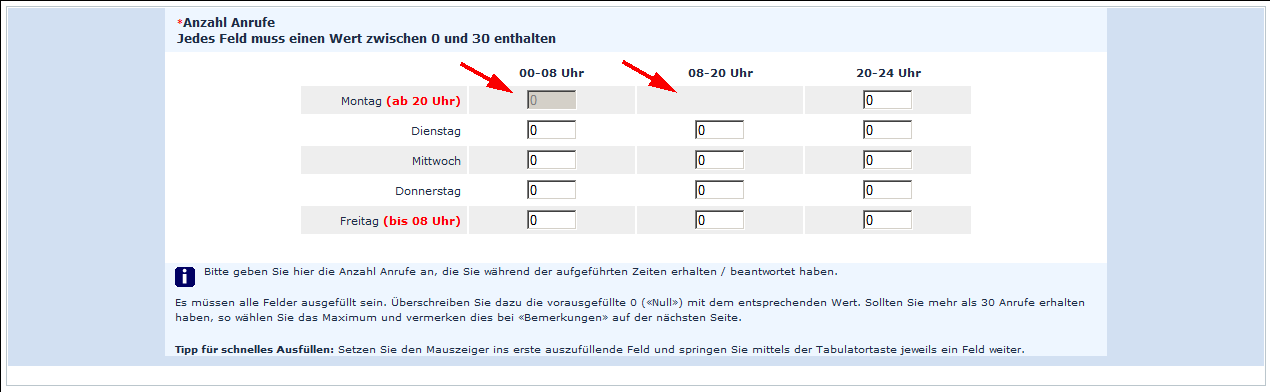
Check null input javascript. Step 2) Add JavaScript: If an input field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted: The variable is neither undefined nor null The variable is neither undefined nor null The variable is undefined or null The variable is undefined or null. The typeof operator for undefined value returns undefined. Hence, you can check the undefined value using typeof operator. Also, null values are checked using the === operator. The following types are considered as false. Null. Undefined. NaN. Empty string ("") Zero (0) So, we first check the type of a variable, and then if the value is of the above type, it has a false value. You put the value in the condition of the if statement. Based on the result, further actions are taken.
30/6/2013 · That was true in certain old browsers (except you want element.selectedIndex not element.selecedValue), but I think you'll find every modern browser lets you use .value directly on the select element to get the selected item. – nnnnnn Jul 1 '13 at 11:20 First run of function is to check if b is an array or not, if not then early exit the function. next step is to compute array difference from [null,'0','0.0',false,undefined,''] and from array b. if b is an empty array predefined conditions will stand else it will remove matching values. conditions = [predefined set] - [to be excluded set ... The value null is written with a literal: null.null is not an identifier for a property of the global object, like undefined can be. Instead, null expresses a lack of identification, indicating that a variable points to no object.In APIs, null is often retrieved in a place where an object can be expected but no object is relevant.
The value of your input is going to be the string "null", not a real null value. Add quotes around your nulls.. Also, accessing inputs via just their ids is bad practice, and I'm not sure if it's even cross-browser compatible.Use the document.getElementById function to find your elements instead.. Finally, (unless my memory fails me) the value property of select elements isn't supported on all ... If the name is empty: After submitting the form: Simplified with 3 Different Methods: Method-1 : Using === operator. Using === operator we will check the string is empty or not. If empty then it will return "Empty String" and if the string is not empty it will return "Not Empty String". To check if the accept checkbox is checked, you use the following code: const cb = document .getElementById ( 'accept' ); console .log (cb.checked); Code language: JavaScript (javascript) Additionally, you can use use the querySelector () to check if the :checked selector does not return null Like this:
Javascript function to check whether a field is empty or not. At first the function required () will accept the HTML input value through inputtx parameter. After that length property of string, object is used to get the length of the said parameter. If the length of value.inputtx is 0 then it returns false otherwise true. @HunanRostomyan Good question, and honestly, no, I do not think that there is. You are most likely safe enough using variable === null which I just tested here in this JSFiddle.The reason I also used ` && typeof variable === 'object'` was not only to illustrate the interesting fact that a null value is a typeof object, but also to keep with the flow of the other checks. 16/7/2020 · JavaScript isNull | How to Check for null. The value null represents the object value or variable value is don’t have any value (“No value”). There is no isNull function in JavaScript script to find without value objects. However you can build your own isNull () function with some logics.
JavaScript HTML Check Multiple Input TextBox/Text Field. JavaScript HTML Check Multiple Input Text Field ตัวอย่างนี้จะเป็นการใช้ JavaScript ควบคุม การตรวจสอบค่าว่างของ Text Field กรณีที่ช่องกรอกข้อมูลมี ... The if condition will execute if my_var is any value other than a null i.e . If my_var is undefined then the condition will execute. If my_var is 0 then the condition will execute. If my_var is " (empty string) then the condition will execute. … This condition will check the exact value of the variable whether it is null or not. Example 2: Vanilla JavaScript is not a new framework or library. It's just regular, plain JavaScript without the use of a library like Lodash or jQuery. # A. Empty Object Check in Newer Browsers. We can use the built-in Object.keys method to check for an empty object.
In JavaScript, one of the everyday tasks while validating data is to ensure that a variable, meant to be string, obtains a valid value. This snippet will guide you in finding the ways of checking whether the string is empty, undefined, or null. Here we go. If you want to check whether the string is empty/null/undefined, use the following code: To check all array values for null or undefined values: ... ajax android angular api button c++ class database date dynamic exception file function html http image input java javascript jquery json laravel list mysql object oop ph php phplaravel phpmysql phpphp post python sed select spring sql string text time url view windows wordpress xml. JavaScript Object.is() method to check null value. Javascript Object is() an inbuilt method that checks whether two values are the same value. The ES2015 method Object.is() differs from the strict === and loose == equality operators in how it checks for NaN and negative zero -0 values. To check null values, the behavior of Object.is() is the ...
Validation is more commonly applied to a form's submit handler since a form can be submitted without clicking the submit button. Comparing the value of the control with "" (empty string) should work, however the value might be whitespace so you might want to remove that first, e.g. To check for null values in JavaScript, you need to check the variable value with null. Use the JavaScript strict equality operator to achieve this. Example. You can try to run the following code to check whether the value of the variable is null or not − 4/4/2020 · how to check if input is null in javascript; checking if an input's value is not blank js; how to get input is empty; why input field is empty javascript; why is the value if my input null; check if input is empty vanilla js; check if any value in input is null; es6 check if input is empty; check textbox empty javascript; if $_input is empty ...
16/10/2019 · Given an HTML document containing input element and the task is to check whether an input element is empty or not with the help of JavaScript. Approach 1: Use element.files.length property to check file is selected or not. If element.files.length property returns 0 then the file is not selected otherwise file is selected. 34 Check If Input Is Null Javascript. Written By Joan A Anderson Tuesday, August 10, 2021 Add Comment. Edit. Check if input is null javascript. At Least One Checkbox Must Be Selected Checked Tech Notes. Understanding Null Undefined And Nan By Kuba Michalski. How To Check For An Object In Javascript Object Null Check. JavaScript HTML Check Multiple Input TextBox/Text Field: ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ...
C hecking for null is a common task that every JavaScript developer has to perform at some point or another. The typeof keyword returns "object" for null, so that means a little bit more effort is required. Comparisons can be made: null === null to check strictly for null or null == undefined to check loosely for either null or undefined. 13/11/2019 · When you’re dealing with user input, ... To avoid that trap, don’t use null in JavaScript. If you want special cases for uninitialized or empty values, state machines are a better bet. JavaScript includes two additional primitive type values - null and undefined, that can be assigned to a variable that has special meaning. null. You can assign null to a variable to denote that currently that variable does not have any value but it will have later on. A null means absence of a value.
Javascript Jeff Kemp On Oracle
How To Set Textbox Value Null In Javascript Code Example
How To Validate Phone Number Input In Html And Javascript
Test An Azure Stream Analytics Job With Sample Data
Powershell Null Comparison Demystified Rencore
How To Check An Empty Undefined Null String In Javascript Quora
How To Check For An Object In Javascript Object Null Check
Javascript The Curious Case Of Null Gt 0 By Abinav Seelan
How To Use Empty String With Javascript Function Code Example
Workarounds Manipulating A Survey At Runtime Using
How To Set The Input Type Date To Null After Submitting The
Javascript Jeff Kemp On Oracle
How To Write Functional Tests In React Part 1 By Jeffrey
How To Check If A String Is Empty Null Undefined In
Introduction To Hypothesis Testing In R Learn Every Concept
Getting Started Validating Form Input
Radio Button With V Model And Value Is Not Always Checked
How To Check A Radio Button Using Javascript Javatpoint
How To Check Empty Undefined Null String In Javascript
Shiny Write Error Messages For Your Ui With Validate
How To Check Null Condition Help Uipath Community Forum
How Can I Check For An Empty Undefined Null String In
Java67 Right Way To Check If String Is Empty In Java
Javascript Jeff Kemp On Oracle
Checking If A Variable Exists In Dmn Juel Dmn Engine
What Went Wrong Troubleshooting Javascript Learn Web
Javascript Check Input For Empty
0 Response to "31 Check Null Input Javascript"
Post a Comment