33 How To Concatenate Two Strings Javascript
Join two strings: let str1 = "Hello "; let str2 = "world!"; let res = str1.concat(str2); Try it Yourself ». More "Try it Yourself" examples below. The concat method accepts the string as an argument and returns the new string by concatenating existing string with an argument.
Javascript String Concat How To Concat String In Javascript
29/7/2019 · There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them. The + Operator. The same + operator you use for adding two numbers can be used to concatenate two strings. const str = 'Hello' + ' ' + 'World'; str; // 'Hello World' You can also use +=, where a += b is a shorthand for a = a + b.
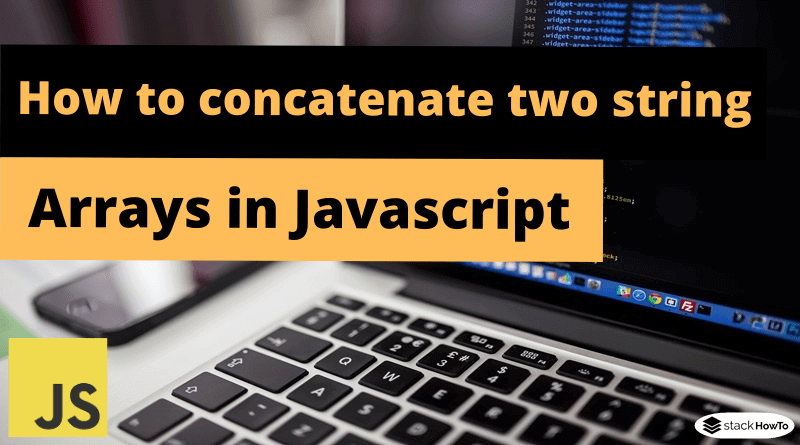
How to concatenate two strings javascript. Concatenate strings in Javascript with a separator Earlier, when we used the join() and concat() methods, we found out that the resulting string has a separator with every value. The separator was a comma that is the default separator of these two methods. JavaScript add strings with + operator. The easiest way to concatenate strings is to use the + or + = operator. The + operator is used to add numbers and strings; in programming, we say that operators are overloaded. let a = 'old' ; let b = ' tree' ; let c = a + b; console .log (c); In the example, we add two strings with the + opeartor. How to join two strings in JavaScript Find out how to combine strings using JavaScript. Published Sep 22, 2019. JavaScript, like any good language, has the ability to join 2 (or more, of course) strings. ... which returns a new string concatenating the one you call this method on, with the argument of the method: const fullname = name. concat ...
24/1/2018 · To concatenate multiple string variables, use the JavaScript concatenation operator. 10/2/2020 · JavaScript offers us three different ways to concatenate or joining strings. The first way is using the plus + operator to join two strings. The second way is using the string interpolation with the help of es6 string literals. The third way is using the += operator to assign a string to the existing string. The concat() function concatenates the string arguments to the calling string and returns a new string. Changes to the original string or the returned string don't affect the other. If the arguments are not of the type string, they are converted to string values before concatenating.
The concat () method is used to join two or more strings to form a new string. This is done by joining one or more strings to the calling string. This method returns a new string containing the text of the joined strings without changing the existing strings. It was introduced in ECMAScript 1. Syntax. Addition assignment ( +=) - MDN. JavaScript provides ways to combine two or more strings into a single string, and 0:00. it's something you'll likely do a lot and programming. 0:05. For example, you might collect the user's first name as one string and 0:08. their last name as a separate string. 0:11. JavaScript String object has a built-in concat () method. As the name suggests, this method joins or merges two or more strings. The concat () method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings.
The concat method creates a new array consisting of the elements in the object on which it is called, followed in order by, for each argument, the elements of that argument (if the argument is an array) or the argument itself (if the argument is not an array). It does not recurse into nested array arguments. The concat method does not alter this or any of the arrays provided as arguments but ... String Concatenation in Java In java, string concatenation forms a new string that is the combination of multiple strings. There are two ways to concat string in java: By + (string concatenation) operator 19/4/2019 · Let’s create two strings and assign two string values to them and create a 3rd variable to concatenate the two strings. var a = "Hello"; var b = "World"; var c = a + b; document.write(c); Now, if we reverse the strings, var c = b + a; This would be the output. So, the result of the concatenation of two strings is based on how the strings are arranged around the concatenation operator as well.
str.concat () function is used to join two or more strings together in JavaScript. For just two strings, you can append as follows: Basically, you can think of it as concatenating str2 to str1. The left-hand side is getting the right-hand side added to it. Appending two or more strings to another This video details three different ways that you can combine multiple strings and variables with string values in JavaScript.Code GIST: https://gist.github.c...
# 4 Ways to Combine Strings in JavaScript. Here are 4 ways to combine strings in JavaScript. My favorite way is using Template Strings. Why? Because it's more readable, no backslash to escape quotes, no awkward empty space separator, and no more messy plus operators ๐ JavaScript String concat() Method. The JavaScript string concat() method combines two or more strings and returns a new string. This method doesn't make any change in the original string. Syntax. The concat() method is represented by the following syntax: In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string.
21/5/2013 · const numStrings = 100; const strings = [...new Array(numStrings)].map(() => Math.random().toString(36).substring(6)); const concatReduce = (strs) => strs.reduce((a, b) => a + b); const concatLoop = (strs) => { let result = '' for (let i = 0; i < strings.length; i++) { result += strings[i]; } return result; } // Case 1: 52,570 ops/s concatLoop(strings); // Case 2: 96,450 ops/s concatReduce(strings) // Case 3: 138,020 ops/s strings.join('') // Case 4: 169,520 ops/s ''.concat(...strings) How to concatenate two string arrays in Javascript. Y ou can easily create a string by concatenating the elements of an array using JavaScript's join () method. The join () method also lets you specify a separator to separate array elements. The default separator is a comma (,). The JavaScript concat () method merges the contents of two or more strings. You can also use the + concatenation operator to merge multiple strings. The concatenation operator is preferred in most cases as it is more concise. Concatenation is a technique used in programming to merge two or more strings into one value.
The concat () accepts a varied number of string arguments and returns a new string containing the combined string arguments. If you pass non-string arguments into the concat (), it will convert these arguments to a string before concatenating. Merge Strings in JavaScript Let's begin by merging strings together. Put the concat () method after the string variable as a "dot function". Then pass a comma-separated list of strings to add inside the () (parenthesis) of the concat () method. How to concatenate two strings javascript. Concatenate Strings In Php Tutorial Wtmatter The concat method doesn't modify the string in place const str3=str1. In this Java split string by delimiter case, the separator is a comma (,) and the result of the Java split string by comma operation will give you an array split Understanding Merge Sort ...
Using Javascript built-in concat () method, we can join two or more strings. The concat () method does not change the existing strings and returns a new string that results from concatenates the string arguments to the original string. See the Pen JavaScript - Concatenate two strings except their first character - basic-ex-61 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: Write a JavaScript program to create a new string without the first and last character of a given string.
Javascript Lesson 22 Concat Method In Javascript Geeksread
Strings Concatenation In C Javatpoint
Basics Of Javascript Part 5 String Functions
Python Concatenate Strings Step By Step Guide Career Karma
Javascript Cut String If Too Long Code Example
Concatenate Char To String In Java
Ms Excel How To Concatenate Strings Together Ws Vba
Mysql Concat Function W3resource
Basic C Program To Concatenate Strings Using Pointer C
C Program To Concatenate Two Strings Without Using Strcat
How To Concatenate Two Strings In Javascript The Complete
3 Ways To Concatenate Strings In Javascript Mastering Js
Splitting Concatenating And Joining Strings In Python
4 Ways To Combine Strings In Javascript Samanthaming Com
Python Program To Concatenate Two Strings
String Concatenation And Format String Vulnerabilities
How To Concatenate Strings In C A Five Minute Guide
How To Concatenate Two String Arrays In Javascript Stackhowto
Concatenate Strings C Slide Share
C Program To Concatenate Two Strings Without Using Strcat
Sql Server Concatenate Operations With Sql Plus And Sql
Javascript Join Two Strings Example Of Concat And
Javascript Tutorial 2 Concatenation How To Join Two Strings Together In Javascript
Javascript Concat String Does Not Work As Expected Stack
How To Create Multi Line String With Template Literals In
How To Do Text Concatenation In Storyline Javascript Light
The Problem Of String Concatenation And Format String
How To Concatenate Two Strings In Javascript The Complete
String Concatenation And Format String Vulnerabilities
5 Ways Of Python String Concatenation With 6 Examples
0 Response to "33 How To Concatenate Two Strings Javascript"
Post a Comment