20 How To Check Radio Button Value In Javascript
Use document.getElementById ('id').checked method to check whether the element with selected id is check or not. If it is checked then display its corresponding result otherwise check the next statement. If no one radio button is selected then it returns 'No one selected'. I googled "get radio button value javascript" and quickly found this javascript - How to get value of selected radio button? - Stack Overflow Please do basic research before asking a question, if that link doesn't help you there are many more if you google yourself.
Vue Js Radio Button Onchange Event Example Tuts Make
Button Style: Check. Radio Button Choice: Yes (this is the export value I believe though I don't quite know how to use it properly) neither are checked by default but if one is checked I would like to have a variable set to the text "Approved" and if the other radio button is checked I would like to set the variable to contain "Disapproved"
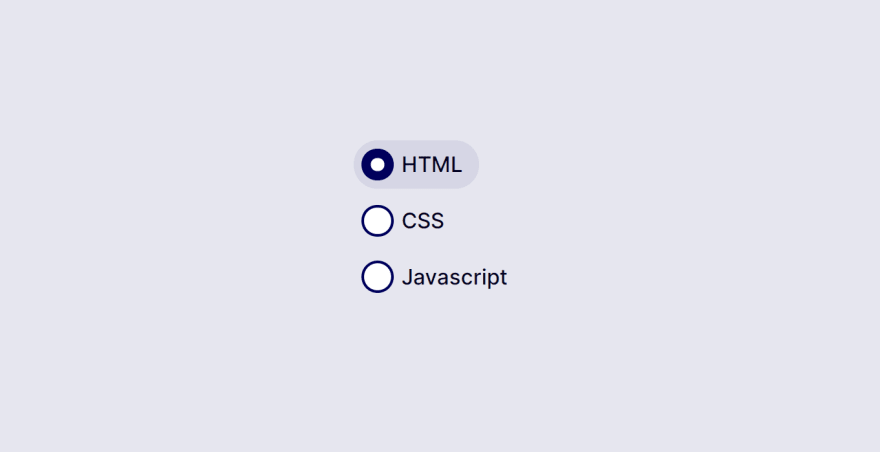
How to check radio button value in javascript. Join Stack Overflow to learn, share knowledge, and build your career. When the above form is submitted with a radio button selected, the form's data includes an entry in the form contact=value.For example, if the user clicks on the "Phone" radio button then submits the form, the form's data will include the line contact=phone.. If you omit the value attribute in the HTML, the submitted form data assigns the value on to the group. radio button checked event using javascript. ... Similarly, "document.getElementById" method is used for retrieving the value from HTML. Radio button checked and unchecked event using jQuery. Suppose we have an HTML form which has a number of radio button choices options as: LG;
Check and un-check a specific radio button: function check() { document.getElementById("red").checked = true; ... Specifies whether a radio button should be checked or not. true - The radio button is checked ... Return Value: A Boolean, returns true if the radio button is checked, and false if the radio button is not checked: More Examples ... Apr 05, 2013 - Javascript getElementById() works for a text box but doesn't work with radio buttons or drop-down selectors ... Is there a JavaScript function that determines which radio input is checked and alerts with the value of that radio input? To get the value of selected radio button, a user-defined function can be created that gets all the radio buttons with the name attribute and finds the radio button selected using the checked property. The checked property returns True if the radio button is selected and False otherwise.
However, it involves us knowing the value we want to set upfront, it might be that we want to somehow get a reference to a radio button and then set that radio button to checked. Using the "checked" property. Now let's look at the DOM Node level method of checking a radio button - the checked property. Jul 15, 2021 - Summary: in this tutorial, you will learn how to use JavaScript to check which radio button in a radio group is checked. Radio buttons allow you to select only one of a predefined set of mutually exclusive options. To create a radio button you use the ... <form> <input type="radio" name="choice" value... How to select a radio button by default in JavaScript? Javascript Object Oriented Programming Front End Technology To select a radio button by default we have to check it as true. If it is checked as true then by default it will be autofocused.
Input Radio checked Property. Check and un-check a specific radio button: function check () {. Find out if a radio button is checked or not: getElementById ("myRadio"). Use a radio button to convert text in an input field to uppercase: getElementById ("fname"). Several radio buttons in a form: var coffee = document. In this article, we will learn how to check or uncheck a radio button using jquery or javascript. There are two ways to check or uncheck the radio button dynamically using jquery or javascript. The first way is to use the prop () function and the second is to use the attr () function of jquery. Here are the examples to check or uncheck the ... The prop () method is used to check or uncheck a checkbox, such as on click of a button. The method requires jQuery 1.6+ versions. The prop () method has an advantage over the.attr () method when setting disabled and checked. The method manipulates the checked property and sets it to true or false based on whether you want to check or uncheck it.
This post will discuss how to get the value of the selected radio button in JavaScript and jQuery. To get the selected radio button, you can use the :checked CSS pseudo-class selector, representing any radio (<input type="radio">) that is checked. We can use this in many ways with jQuery and plain JavaScript. 1. Use following multiple radio button validation example to validate a radio button group in JavaScript. Choose the program which best match with your application. A radio button contains more than one radio buttons. Simply array of radio buttons. All radio buttons have the same name but different ids and values. However, we have to write the JavaScript code to get the value of the checked radio button, which we will see in the chapter below: Check the radio button is selected or not There are two ways in JavaScript to check the marked radio button or to identify which radio button is selected. JavaScript offers two DOM methods for this.
On this page we describe and demonstrate how to use JavaScript to get the value of the selected radio button in a group. We have defined the following getRadioVal function which returns the value of the selected radio button, or undefined if no radio button is checked: <!--Check selected Radio Button value using JavaScript.--> <html> <head> <title> Check selected Radio Button value using JavaScript. </title> <script type="text/javascript"> function checkGender () { if (document.getElementById ('radioMale').checked) alert ("You have selected Male."); else alert ("You have selected Female." Compile how-to-check-a-radio-button-using-javascript1 Example: Online Editor, jQuery and Bootstrap technologies with this online compiler, it helps you learn better the web technology.
The type of the field is determined by the value of the type attribute. One of the input types is the radio, which creates a radio button. When one radio button is chosen, all others will be disabled. Radio buttons are presented in radio groups, which is a collection of radio buttons describing a collection of related options. You can simply use the jQuery :checked selector in combination with the val() method to find the value of the selected radio button inside a group. In this post, I will share with you an example of how to check the radio button based on selected values using jquery. Maybe you have a task that needs to check the radio button on a selected value using a select box or even a button. To proceed kindly check the example code below.
Before submitting the value of the radio button group, it is required to check whether the user selects one radio button in each group. Because it's always a good idea to validate the value in the client before sending data to the server side. Using jQuery you can easily check if radio is checked. If a radio button is in checked state when the form is submitted, the name of the radio button is sent along with the value of the value property (if the radio button is not checked, no information is sent). Tip: Define different values for radio buttons in the same group, to identify (on the server side) which one was checked. You should always use the label tag with radio buttons. This signals to the user that the whole boxed area may be clicked to set the radio button, rather than just the tiny circle. If you are using a scripting language like PHP, ColdFusion, or ASP, then write a function that prints your radio buttons from an array.
Check Radio Button Using JavaScript Select the Radio Button Using ID One of the best possible ways to preselect a radio button is to refer to it by its ID. To select the DOM element using ID, we add the prefix # to the ID and set the checked value as true. value="cc" will be checked by default, if you remove the "checked" non of the boxes will be checked when the form is first loaded. document.teenageMutant.ninjaTurtles.checked=true; now value="aa" is checked. The other radio check boxes are unchecked. In order to uncheck a radio button, there is a lot of methods available, but we are going to see the most preferred methods. Example 1: This example unchecks the radio button by using Javascript checked property. The JavaScript Radio checked property is used to set or return the checked state of an Input Radio Button.
The <input type="radio"> defines a radio button. Radio buttons are normally presented in radio groups (a collection of radio buttons describing a set of related options). Only one radio button in a group can be selected at the same time. Note: The radio group must have share the same name (the value of the name attribute) to be treated as a group. Jun 17, 2020 - button, examples, input, property, radio, JavaScript articles on Yogesh Chauhan's Programming tips and tutorials Blog. This may seem silly and downright stupid but I can't seem to figure out how to check the value of a radio button group in my HTML form via JavaScript. I have the following code: <input type="ra...
We obtain a reference to the group of radio buttons using the name of the group, and then iterate over all the radio button objects to test which one of them is checked.
How To Know Which Radio Button Is Selected Using Jquery
Validate Radio Button Is Chosen Stack Overflow
Radio Button Is Checked But Display Not Check Wordpress
10 Radio Button Css Style Examples Dev Community
Lwc How To Get Selected Radio Button Value On Handle Change
Get Selected Radio Button S Text In C Windows App Techbrij
Angular Bootstrap Radio Button Bootstrap 4 Amp Material Design
Get Radio Button Value Using Angularjs Pakainfo
Get Value From Multiple Radio Button In Javascript Code Example
How To Check A Radio Button Using Javascript Javatpoint
How To Determine Which Radio Button Is Selected Learn Web
How To Tick A Radio Button Based On Another Value In The Same
Angular 12 Dynamic Radio List Get Selected Value On Change
React Radio Button Tutorial With Example Positronx Io
Clicking A Radio Button Web Testing Katalon Community
Angular 12 Dynamic Radio List Get Selected Value On Change
Javascript How To Tell Which Radio Button Is Checked
Php 8 Radio Buttons Get Selected Value Add Custom Styling
0 Response to "20 How To Check Radio Button Value In Javascript"
Post a Comment