29 Array Programming Interview Questions In Javascript
Jun 18, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Array Interview Questions (coming soon) Finding the Missing Number from Array. Finding Duplicate Integers in an Array. Finding the Largest and Smallest Number in Unsorted Array. Removing Duplicates from an Array. Reversing an Array. Finding the k -th Smallest Integer in an Unsorted Array.
Snap Interview Questions And Interview Process
Start studying JavaScript Interview Questions. Learn vocabulary, terms, and more with flashcards, games, and other study tools. ... Interpreted programming language. let, var, const. var is function-scoped, let and const are block-scoped. ... Creates a new array with all the elements of the array for which the filtering function returns true ...
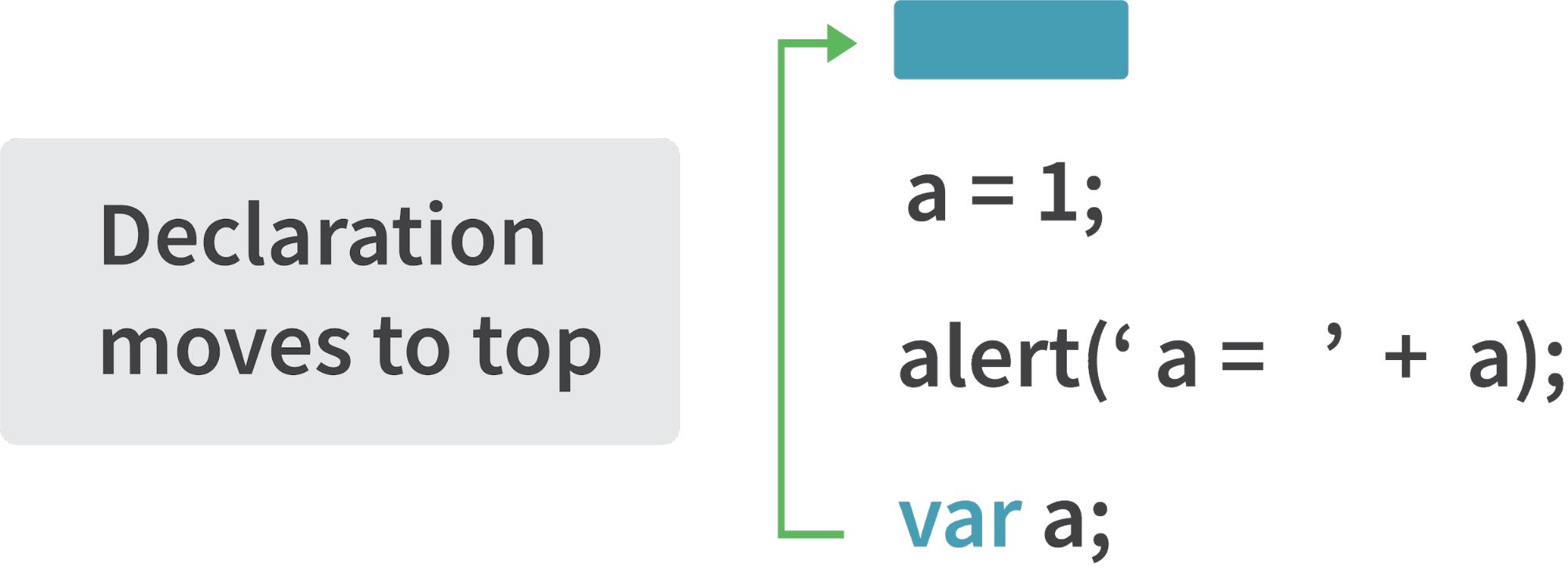
Array programming interview questions in javascript. A selection of questions and answers in regards JavaScript Arrays. - GitHub - jwill9999/JavaScript-Array-Interview-Practice: A selection of questions and answers in regards JavaScript Arrays. Mar 18, 2016 - Interview Algorithm Questions in Javascript() {...} Table of Contents Array Strings Stacks and Queues Recursion Numbers Javascript ... A mostly reasonable collection of technical software development interview questions solved in Javascript in ES5 and ES6 Q 7: True of False: An element in a JavaScript array can be another array. A: True. Hint: JavaScript Array elements can be arrays. Q 8: Given the following line of code, what is the length of the array "foo"? var foo = []; 1. var foo = []; A: 0. Hint: foo is an Array literal, but in this line of code, no elements have been defined.
Given two unsorted arrays, the task is to write a JavaScript program to print the unique (uncommon) elements in the two arrays. Approach: Create an… Read More » Deep, regular learning of JavaScript will improve your coding, and as a positive side effect, interviewing skills. In this post, you will find 7 at first sight simple, but tricky JavaScript interview questions. While at first the questions might seem random, they try to hook into important concepts of JavaScript. Javascript objective interview practice question on arrays. In these objective questions i covered mostly used javascript array methods such push,pop,slice,shift,sort etc. Javascript Interview Questions on Arrays. Question 1. What's the correct syntax for Array Declaration. a) var city = new Array("delhi", "agra", "akot ...
JavaScript Interview Questions: Arrays. Manu Bhardwaj. Follow. Oct 2, 2020 · 2 min read. JavaScript has been the most used programming language for many years now, yet people continue to find it hard to get a grasp of. This article will discuss some of the most frequently asked questions in JavaScript. Array questions are the most asked questions these days. The interviewer wants to know, how efficient code you can write. Therefore practice technical questions on arrays. These array practice questions will help you clear the difficult programming rounds. In other words, these rounds are based on array questions. Jul 07, 2020 - Questions about data structures are very common for coding interviews. Since arrays are so popular in computer programs, you will likely encounter coding problems about arrays in any coding interview. Here are common JavaScript interview questions for arrays that demonstrate your mastery of arrays.
This JavaScript interview questions blog will provide you an in-depth knowledge about JavaScript and prepare you for the interviews in 2021. ... Java is an OOP programming language. JavaScript is an OOP scripting language. ... How to empty an Array in JavaScript? (a) No matter what button the user clicks the number 5 will always be logged to the console. This is because, at the point that the onclick method is invoked (for any of the buttons), the for loop has already completed and the variable i already has a value of 5. (Bonus points for the interviewee if they know enough to talk about how execution contexts, variable objects, activation objects ... This article presents the most common interview questions and popular problems with arrays, two-dimensional arrays, queues, stacks, binary search trees, graphs, etc. To be comfortable with this ...
The javascript engine sees that the method push does not exist on the current array object and therefore, looks for the method push inside the Array prototype and it finds the method. Whenever the property or method is not found on the current object, the javascript engine will always try to look in its prototype and if it still does not exist ... Spread the love Related Posts Append Item to a JavaScript ArrayAppending an item to an array in JavaScript is easy. There're 2 ways to do… JavaScript Interview Questions — Data TypesTo get a job as a front end developer, we need to nail the coding… Cloning Arrays in JavaScriptThere are a few ways to clone an array […] JavaScript Interview Questions. JavaScript interview questions and answers for provides a list of top 20 interview questions. The frequently asked JavaScript interview questions with answers for beginners and professionals are given below. 1) What is JavaScript? JavaScript is a scripting language. It is different from Java language.
Dec 30, 2017 - Home » Content » JavaScript Interview Questions » JavaScript Tech Interview Exercise 1 – Writing an Array Extension ... Get The Course Now! ... Abstraction Algorithms architecture Automated Testing BackboneJs Bower ChaiJs Competence computer science css ES6 events Functional Programming ... 6) What is the default value of Array? Any new Array is always initialized with a default value as follows. For byte, short, int, long - default value is zero (0). For float, double - default value is 0.0. For Boolean - default value is false. For object - default value is null. Live Mock Technical Interview - JavaScript. Question 6 How to empty an array in JavaScript? For instance, var arrayList = ['a', 'b', 'c', 'd', 'e', 'f']; How can we empty the array above? There are a couple ways we can use to empty an array, so let's discuss them all. Method 1 arrayList = [] Above code will set the variable arrayList to a new ...
Jun 04, 2020 - If that character does not match the starting bracket, we tell the program to return false. When we reach the end, any remaining open brackets in the stack will return false. ... A common challenge in a JavaScript interview asks you to remove the first two elements of an array using array ... 38. What will be the console output and why? a is assigned to an object, b is assigned to a using the spread operator, which means a and b are technically the same. c is just an empty object. Using Object.assign (), c is now assigned to a, and later after that, we changed the value of x in a as 2. The console output will be 2,1,1. How to add one new element at end of an array in javascript? Push method adds one or more elements to the end of an array and returns the new length of the array. ... Commonly Asked Java Programming Interview Questions | Set 2. 19, Oct 16. Commonly Asked C++ Interview Questions | Set 2. 18, Dec 15. 10 Most Asked ES6 Interview Questions ...
Stock Span problem. Find a triplet that sum to a given value. Smallest positive missing number. Find the row with maximum number of 1's. Print the matrix in a Spiral manner. Find whether an array is a subset of another array. Implement two Stacks in an array. Majority Element. Wave Array. Feb 22, 2021 - The JavaScript(JS) interviews are not easy. I accept it, you accept it and, everyone does. The number of possibilities of questions could be asked in a JavaScript interview are high. How one will be… A mix of basic and advanced JavaScript interview questions to keep you polymorphic. Non-coding and coding JS interview questions, programming assignments, and math and logic puzzles. The best resources to get your JavaScript skills in top form for the interview. Core advice: Don't just drill JavaScript interview questions.
Arrays In Programming Fundamentals 3. Pointers And Arrays 4. Pointers And 2 D Arrays 5. Array Implementation Details 6. Sorting Algorithms ... SQL Interview Questions; Javascript Interview Questions; MVC Interview Questions; React Interview Questions; jQuery Interview Questions; Angular Interview Questions; A matrix is an array of arrays. Digital images, for example, are essentially matrices, with each pixel being a cell of an array and each array being stacked vertically. That's why this problem is also referred to as rotating an image. Here's an example of a matrix. On the left is an input and on the right is the desired output: 21. Explain array.slice and array.splice in Js. Answer: 1) The slice() method copies a given part of the array and returns it. It does not modify the original array. array.slice(inital,final) 2) Array.splice method is used to remove the items of the selected array and does not form another array. This method modifies the original array.
Tricky JavaScript interview questions. ... The last line answer is 4 because the string is consider as an array of char in javascript. ... This is very novice question of functional programming. Answer: Array is a data structure which store multiple variables of the same type. It can hold primitive types as well as object references. In simple words an array is used to store a collection of data, array is declared like this. dataType [] arrayRefVariable = new dataType [arraySize]; These are some important facts of array. 4) Define let and const keywords. let: The variables declared using let keyword will be mutable, i.e., the values of the variable can be changed. It is similar to var keyword except that it provides block scoping.. const: The variables declared using the const keyword are immutable and block-scoped. The value of the variables cannot be changed or re-assigned if they are declared by using the ...
Because JavaScript is such a small language, yet with incredible complexity, you should be able to ask relatively basic questions and find out if they are really that good based on their answers. For instance, my standard first question to gauge the rest of the interview is: In JavaScript, what is the difference between var x = 1 and x = 1 ... These many questions should be enough but If you need more such coding questions you can take help from books like Cracking The Code Interview, by Gayle Laakmann McDowell which presents 189+ Programming questions and solutions. A good book to prepare for programming job interviews in a short time. This deceptively simple question is designed to test your prospective coder’s awareness of mitigating potential bugs when solving problems. The easiest method would be to set “emptyArray” equal to “[ ]”—which creates a new empty array. However, if the array is referenced anywhere ...
I've carefully gone through over 50 resources, I've been through 10 JavaScript interviews, and I've landed a job at a unicorn startup.. And throughout this entire process, I started to see a pattern in the most frequently asked JS interview questions.. In this article, I have tried to list the concepts which will cover 80% of any good JS interview.. So, if you are prepping for your next JS ... Jun 22, 2014 - javascript interview question, front end interview, front end interview preparation, front end interview questions Mar 12, 2019 - I’ve been conducting interviews for quite some time now . During the last 5 years a lot have evolved in javaScript , but the underlying interview questions remain same. If you master following…
Aug 01, 2021 - Prepare and seize that JS interview with these set of JavaScript Interview Questions and answers. These questions will help you crack upcoming interviews You need to enable JavaScript to run this app 4 days ago - The below list covers all the JavaScript questions for freshers and JavaScript interview questions for professional-level candidates. This JS interview questions guide will help you crack the interview and help you get your dream job for JavaScript Programming.
JavaScript Interview Questions & Answers. 1. What is JavaScript, and how does it work? Ans: JavaScript is a popular client-side and server-side scripting language put into HTML pages Web browsers understand . JavaScript is an Object-Oriented Programming language as well. Javascript Advanced Interview Questions ; Question 18. What Is Javascript Arrays Object? Answer : The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type.
Latest 32 Java 8 Interview Questions Programming Questions
Top Javascript Interview Questions Amp Answers Of 2021
Javascript Reducer A Simple Yet Powerful Array Method
Javascript Interview Questions And Answers 2021 Interviewbit
7 Simple But Tricky Javascript Interview Questions
Javascript Array A Complete Guide For Beginners Dataflair
2021 18 Important Javascript Interview Questions Ngdeveloper
Acing The Javascript Interview Top Questions Explained
Javascript Online Test 20 Questions 35 Mins Tests4geeks
Json Interview Questions And Answers By Online Interview
Javascript Coding Questions And Answers Full Stack Tutorials
Array Data Structure Interview Cake
50 Javascript Interview Questions And Answers In 2021 Updated
Microsoft Software Engineer Interview The Only Post To Read
Find The Second Largest Number Of An Array Js Interview Questions
Javascript Interview Questions Arrays By John Au Yeung
Array Interview Questions In Java For Freshers 2021
Essential Coding Interview Questions With Javascript Part I
15 Best R Programming Interview Questions And Answers
69 Javascript Arrays Interview Questions Solution தம ழ ல Tamil Javascript Course
23 Javascript Interview Questions And Answers
Javascript Interview Questions
100 Python Interview Questions And Answers Updated 2019
A Perfect Guide For Cracking A Javascript Interview A
65 Javascript Interview Questions And Answers The Ultimate
Codingshala Latest Programming Tutorials And Guides
37 Essential Javascript Interview Questions And Answers Toptal
Top 50 Javascript Interview Questions You Must Learn In 2021
0 Response to "29 Array Programming Interview Questions In Javascript"
Post a Comment