27 Read Json String Javascript
"How can I read given JSON String in JavaScript". The verb "read" is a bit ambiguous, but if you wanted to evaluate it, you can use. JSON.parse() You need to encapsulate the JSON in a quote that isn't used within your JSON to actually parse it, but looking at your code, there is no easy way to do it.. My advice to you is - That JSON at some ... JSON.stringify() converts a value to JSON notation representing it: If the value has a toJSON() method, it's responsible to define what data will be serialized.; Boolean, Number, and String objects are converted to the corresponding primitive values during stringification, in accord with the traditional conversion semantics.; undefined, Functions, and Symbols are not valid JSON values.
Json Basics For Beginners With Example Exercises
1 week ago - The JSON.parse() method parses a JSON string, constructing the JavaScript value or object described by the string. An optional reviver function can be provided to perform a transformation on the resulting object before it is returned.
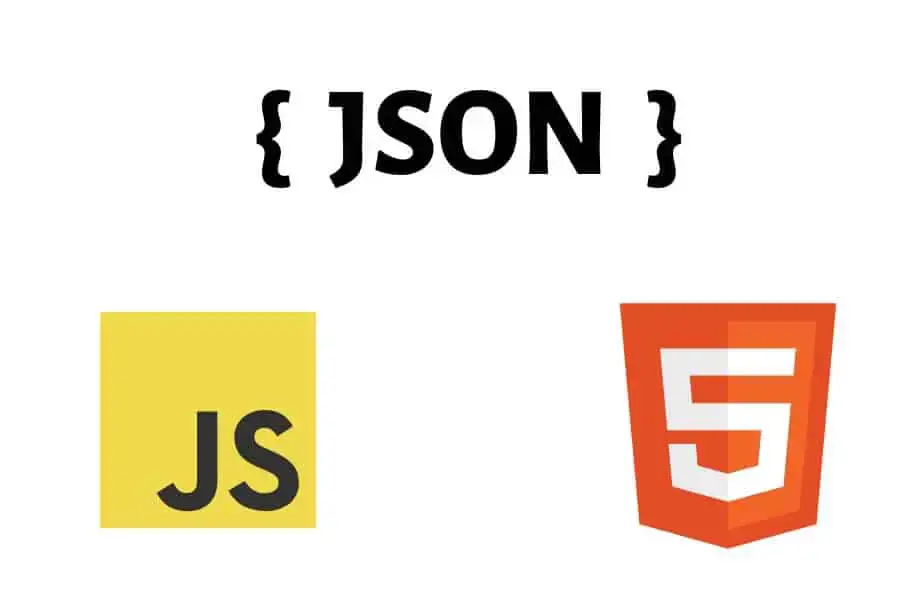
Read json string javascript. Feb 15, 2021 - JavaScript JSON.parse tutorial shows how to parse JSON strings into JavaScript objects. ... JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write and for machines to parse and generate. The official Internet media type for JSON is ... Sep 19, 2020 - A quick reference for the parse and stringify methods of the JSON object. For more information, see Supported collection types in System.Text.Json.. You can implement custom converters to handle additional types or to provide functionality that isn't supported by the built-in converters.. How to read JSON as .NET objects (deserialize) To deserialize from a string or a file, call the JsonSerializer.Deserialize method.. The following example shows how to deserialize a ...
The JSON.parse () method takes a JSON string and transforms it into a JavaScript object. Parsing means to divide into grammatical parts and identify the parts and their relations to each other. After parsing the string from web server, it becomes a JavaScript object and then you can access the data. Here is an example of JSON.parse (): JavaScript Objects. Because JSON syntax is derived from JavaScript object notation, very little extra software is needed to work with JSON within JavaScript. With JavaScript you can create an object and assign data to it, like this: Dec 12, 2013 - suppose instead of phoneNumber its a 0 and inside this 0 property type home and number. now how can u get property inside it. im working on a javascript file and i get error message Unexpected number. ... how to read a .json file in .js file… the json file will includes data in format like ...
Javascript has provided JSON.parse() method to convert a JSON into an object. Once JSON is parsed we can able to access the elements in the JSON. syntax var obj = JSON.parse(JSON); It takes a JSON and parses it into an object so as to access the elements in the provided JSON. Example-1 string Convert a String Back to an Object in JavaScript. To convert a string back into a JavaScript object, use the JSON.parse() method like this: var obj = {'foo': 'bar'}; var string = JSON. stringify (obj); var obj2 = JSON. parse (string) console. log (obj2. foo); bar An Alternative way of Converting to Strings. Another way to convert a thing ... When receiving data from a web server, the data is always a string. Parse the data with JSON.parse(), and the data becomes a JavaScript object.
May 18, 2020 - In this tutorial, we’re going to learn about JSON. We will cover JSON structure, different data types and how to use JSON inside JavaScript.JSON is... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. By using our site, you acknowledge that you have read and understand our Cookie Policy, Privacy Policy, and our Terms of Service. ... Stack Overflow for Teams is a private, secure spot for you and your coworkers to find and share information. ... Closed 5 years ago. I want to parse a JSON string in JavaScript...
A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse (), and the data becomes a JavaScript object. Example - Parsing JSON JSON.parse() converts any JSON String passed into the function, to a JSON object. For better understanding, press F12 to open the Inspect Element of your browser, and go to the console to write the following commands:. var response = '{"result":true,"count":1}'; // Sample JSON object (string form) JSON.parse(response); // Converts passed string to a JSON object. Nov 17, 2011 - Given a string of JSON data, how can I safely turn that string into a JavaScript object? Obviously I can do this unsafely with something like: var obj = eval("(" + json + ')'); but that leaves me
JSON is a text-based data format following JavaScript object syntax, which was popularized by Douglas Crockford. Even though it closely resembles JavaScript object literal syntax, it can be used independently from JavaScript, and many programming environments feature the ability to read (parse) and generate JSON. The JSON.parse () method parses a string and returns a JavaScript object. The string has to be written in JSON format. The JSON.parse () method can optionally transform the result with a function. JavaScript generally used to create, use, consume JSON type data. JSON.parse() is a function that is used to parse JSON structured data. Example JSON Data. During the JSON parse tutorial, we will use the following example JSON data which contains different types like string, integer, date, array, etc.
Learn JavaScript - Read file as string. Get monthly updates about new articles, cheatsheets, and tricks. This will encode the object to a string in both the web browser and Node.js. In order to get a valid string of JSON, you need to pass the JavaScript object to the JSON.stringify method. As you can ... Use the JavaScript function JSON.stringify () to convert it into a string. const myJSON = JSON.stringify(arr); The result will be a string following the JSON notation. myJSON is now a string, and ready to be sent to a server:
Aug 22, 2020 - Description: Takes a well-formed JSON string and returns the resulting JavaScript value. ... The JSON string to parse. Aug 18, 2020 - There are so many programming languages, and every language has its own features. But all of them have one thing in common: they process data. From a simple calculator to supercomputers, they all work on data. It's the same with humans: there are so many countries, so many cultures, and JSON stands for JavaScript Object Notation. JSON is a human-readable data format commonly used to exchange data between web browser, clients and server. Most of the modern APIs using JSON formats as output. That's why the JSON is becoming popular data format for the API's output.
The JSON Format Evaluates to JavaScript Objects The JSON format is syntactically identical to the code for creating JavaScript objects. Because of this similarity, a JavaScript program can easily convert JSON data into native JavaScript objects. In JavaScript, you can easily parse JSON data received from the web server using the JSON.parse() method. This method parses a JSON string and constructs the JavaScript value or object described by the string. If the given string is not valid JSON, you will get a syntax error. Feb 19, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
You can use the JSON.parse () method in JavaScript, to convert a JSON string into a JSON object. Convert String to JSON Using json.stringify () Jul 27, 2020 - How to decode and encode JSON data in JavaScript. You will learn how to encode and decode JSON data in JavaScript with the help of an example. Aug 21, 2018 - JSON, or JavaScript Object Notation, is a minified , readable format for structuring data. It is used primarily to transmit data between a server and web application, as an alternative to XML ... The two important parts that make up JSON are keys and values. Together they make a key/value pair. Key: A key is always a string ...
As the name suggests, JSON.parse () takes a JSON string and parses it into a JavaScript object literal or array. Like with the require method above, fs.readFileSync () is a synchronous method, meaning it could cause your program to slow down if it's reading a large file, JSON or otherwise. JSON's format is derived from JavaScript object syntax, but it is entirely text-based. It is a key-value data format that is typically rendered in curly braces. When you're working with JSON, you'll likely see JSON objects in a.json file, but they can also exist as a JSON object or string within the context of a program. In 2019 Having a string literal with JSON in it, and then calling JSON.parse, is faster than having an object literal in raw JavaScript. So For large object literals, keep them at the top-level (not in a nested function) and use JSON.parse on a string literal.
Reading the stream. Now you've got your reader attached, you can read data chunks out of the stream using the ReadableStreamDefaultReader.read () method. This reads one chunk out of the stream, which you can then do anything you like with. For example, our Simple stream pump example goes on to enqueue each chunk in a new, custom ... To parse JSON strings use the native JSON.parse method instead. Passing in a malformed JSON string results in a JavaScript exception being thrown. For example, the following are all invalid JSON strings: "{test: 1}" (test does not have double quotes around it). "{'test': 1}" ('test' is using single quotes instead of double quotes). Nov 19, 2020 - JSON.stringify() takes a JavaScript object and then transforms it into a JSON string.
The eval () function in JavaScript is used to take an expression and return the string. As a result, it can be used to convert the string into JSON. The string or an expression can be the value of eval (), and even if you pass multiple statements as an expression, the result will still work. The simple syntax for using eval () is as follows: 1 ... And in the same directory (folder), we have a JavaScript file index.js. Our task is to access the content of the json file through the JavaScript file. Method 1: Using require module (NodeJS environment only) We can use the require module to access the json file if we are running our JavaScript file in NodeJS environment. 1 week ago - The json parse() is a built-in JavaScript function that converts text into an object. The json parse() function is converting json string to object. The parse() method is synchronous, so the more the JSON data is big, the more time your program execution will be blocked until the JSON is finished ...
JSON parsing is the process of converting a JSON object in text format to a Javascript object that can be used inside a program. In Javascript, the standard way to do this is by using the method JSON.parse(), as the Javascript standard specifies.. Using JSON.parse() Javascript programs can read JSON objects from a variety of sources, but the most common sources are databases or REST APIs. In JavaScript, the JSON object is used to parse a JSON string. This method is only available in modern browsers (IE8+, Firefox 3.5+, etc). When a valid JSON string is parsed, the result is a JavaScript object, array or other value. See an example below (this example uses the native JSON object). My changes are commented in CAPITALS: function Foo(obj) // CONSTRUCTOR CAN BE OVERLOADED WITH AN OBJECT { this.a = 3; this.b = 2; this.test = function() {return this.a*this.b;}; // IF AN OBJECT WAS PASSED THEN INITIALISE PROPERTIES FROM THAT OBJECT for (var prop in obj) this[prop] = obj[prop]; } var fooObj = new Foo(); alert ...
How To Read Json Object From File In Java Crunchify
Parse Json And Store Json Data Using Node Js Codez Up
Json Tutorial Learn How To Use Json With Javascript
The Json Query Function To Extract Objects From Json Data
Ansible Read Json File Json File Parsing Devops Junction
5 Ways To Convert A Value To String In Javascript
How To Fetch And Display Json Data In Html Using Javascript
Json Tutorial Learn How To Use Json With Javascript
Convert Java Object To Json String Using Jackson Api
Read Write A Raw Json Array Like Json And Map Like Json
Json Load In Python Geeksforgeeks
What Is A Json File Example Javascript Code
Javascript Convert Json Object To Object String Tuts Make
Working With Json Data In Python
Python Json Encode Dumps Decode Loads Amp Read Json File
Working With Json Learn Web Development Mdn
Javascript To Format Json String In Net View Stack Overflow
Extract Scalar Values From Json Data Using Json Value
Two Ways To Use Gson For Json In Java
Json Tutorial Learn How To Use Json With Javascript
Parse Json And Store Json Data Using Node Js Codez Up
3 Ways To Convert Datatable To Json String In Asp Net C
Jquery Convert Json String To Array Sitepoint
0 Response to "27 Read Json String Javascript"
Post a Comment