20 How To Use Regex Validation In Javascript
If there is a matching value, the index value of the string will be returned; if there is no matching value, it will return - 1. JavaScript also provides regexp objects to create and use regular expressions. Jul 01, 2020 - Javascript Form validation is used for validate the user's input data for any registration form, contact form etc. In this tutorial we are showing a form with a submit button. Before submitting data javascript validate the filed and give suggestion as well
How To Check Password Validation Dynamically Using Jquery
JavaScript Form Validation: Removing Spaces and Dashes. Some undesired spaces and dashes from the user input can be removed by using the string object replace() method. The regular expression is used to find the characters and then replace them with empty spaces. Example: JavaScript Form Validation: Removing Spaces
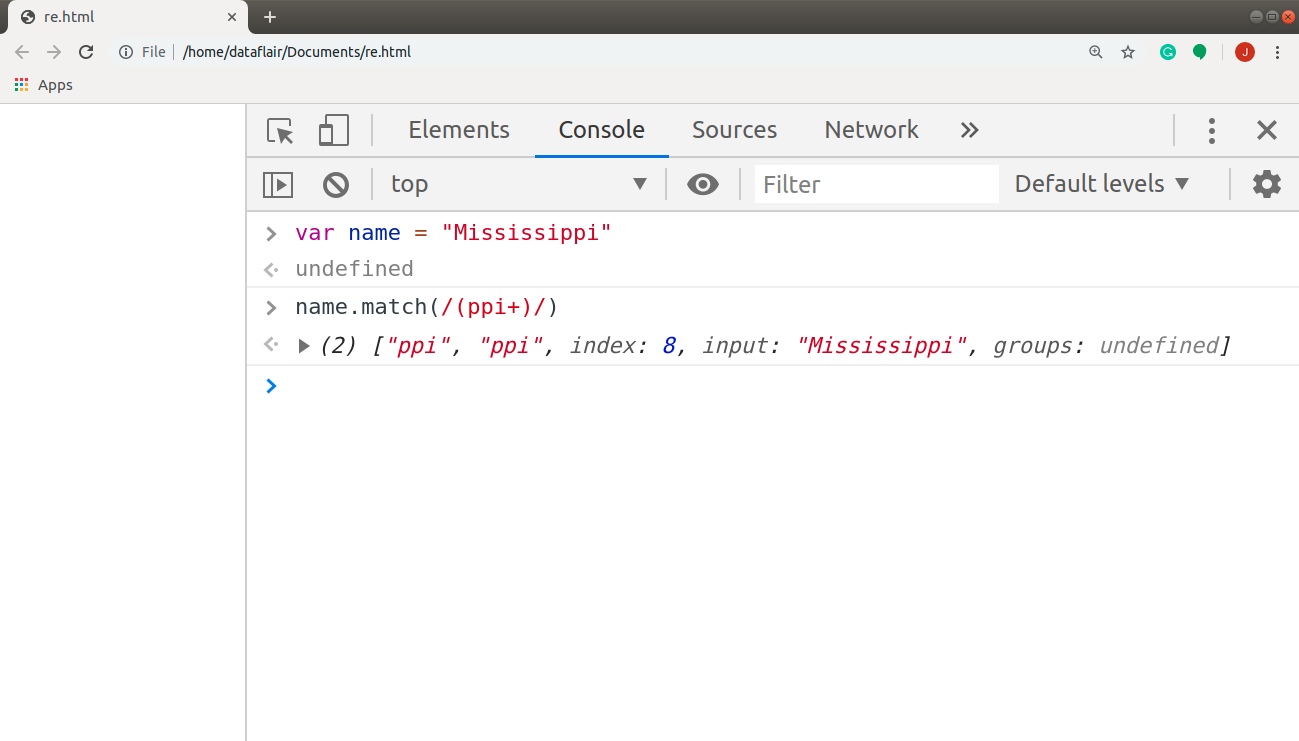
How to use regex validation in javascript. Sep 21, 2017 - Example of how to use the JavaScript RegExp Object. Test your regular expressions online in your web browser. Jul 29, 2021 - Javascript function to validate an email. Also discussed an email structure, example of valid email and invalid email. To validate the said format we use the regular expression ^[A-Za-z]\w{7,15}$, where \w matches any word character (alphanumeric) including the underscore (equivalent to [A-Za-z0-9_]). Next the match() method of string object is used to match the said regular expression against the input value. Here is the complete web document. HTML Code:
Using a JavaScript function, we can validate a field in our ASP.NET page. In this blog, let us see how to do that. ... We can use Regular Expression server-side as well as client-side. Here, I will show you how to use the server-side code. The below Namespace is used for Regular Expression. The / at both ends mark the start and end of the regex The ^ and $ at the ends is to check the full string than for partial matches \d* looks for multiple occurrences of number charcters You do not need to check for both \d as well as [0-9] as they both do the same - i.e. match numbers. The Button has been assigned an OnClick event handler which calls the ValidateEmail JavaScript function. When the Submit Button is clicked, the Email Address in the TextBox will be validated using JavaScript and Regular Expression (Regex) and if the Email Address is invalid, the error message will be displayed next to the TextBox.
Mar 26, 2021 - Check out our JavaScript Regex Cheatsheet for more examples of regular expressions and tips on how to use them. ... While the required and placeholder attributes are not necessarily related to validation, they can be used to improve the user experience when someone is filling out a form. As expected, the library has a couple of core validation methods like isPossible() and isValid(). They offer a handy use of regular expressions that check whether the phone number is entered correctly. There is also a React adaptation of that library with front-end components such as country flags included. Validating a Number Aug 26, 2020 - We have to test all criteria, but using regex you can simplify it. Regular expressions are valid in most string functions, but you should check the prototype of the function if you want to be sure. One such method is the Replace method. Let's learn more about it below. ... JavaScript has a number ...
Sep 18, 2006 - Discover how regular expressions offer a simple and elegant way to manipulate text within JavaScript. Tony Patton also explores how JavaScript's regular expression simplifies data validation. URL regex validation JavaScript | Example code Posted June 9, 2021 June 9, 2021 by Rohit URL regex validation is the best way to check if a string is a valid URL or not. Given an email id and the task is to validate the email id is valid or not. The validation of email is done with the help of Regular Expressions. Approach 1: RegExp - It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.)
In this article, you will learn how to validate the URL in javascript. There are various ways to validate the URL. In this article, we validate the URL using regular expressions. I found these two regexes helpful for me. form validation javascript form validation using regular expression In this tutorial you will see how to use regular expressions to validate. Through a list of examples , we will build a script to validate phone numbers , UK postal codes, along with more examples. Here Mudassar Ahmed Khan has explained with an example, how to implement Indian PAN Card Number validation using Regular Expression (Regex) in JavaScript. The PAN Card Number entered in the TextBox will be validated on Button click using Regular Expression (Regex) in JavaScript. TAGs: JavaScript, Regular Expressions, TextBox, Button
Match the given string with the Regex. In java, this can be done using Pattern.matcher (). Return true if the string matches with the given regex, else return false. Below is the implementation of the above approach: import java.util.regex.*; // Function to validate the password. // Regex to check valid password. 19/8/2021 · All regular expressions in JavaScript are placed between two forward slashes, like so: /your regex/. The expression in this case is: [a-zA-Z] . The brackets and hyphen denote a range of characters. Their syntax is cryptic, and the programming interface JavaScript provides for them is clumsy. But they are a powerful tool for inspecting and processing strings. Properly understanding regular expressions will make you a more effective programmer. ... A regular expression is a type of object. It can be either constructed with the RegExp ...
Form validation using regex in JavaScript with Bootstrap is a script that shows how to validate form fields in your BS5 project using custom Regex. bootstrap regex validation, validate input with regex javascript, regex input validation, bootstrap 5 form validation, perfect javascript form validation using regular expression How to make use of it: If using the RegExp constructor with a string literal, remember that the backslash is an escape in string literals, so to use it in the regular expression, you need to escape it at the string literal level. /a\*b/ and new RegExp("a\\*b") create the same expression, which searches for "a" followed by a literal "*" followed by "b". Feb 26, 2020 - JavaScript exercises, practice and solution: Write a pattern that matches e-mail addresses.
Given string str, the task is to check whether the given string is valid GUID (Globally Unique Identifier) or not by using Regular Expression. The valid GUID (Globally Unique Identifier) must specify the following conditions: . It should be a 128-bit number. It should be 36 characters (32 hexadecimal characters and 4 hyphens) long. It should be displayed in five groups separated by hyphens (-). Edit the Expression & Text to see matches. Roll over matches or the expression for details. PCRE & JavaScript flavors of RegEx are supported. Validate your expression with Tests mode. The side bar includes a Cheatsheet, full Reference, and Help. You can also Save & Share with the Community, ... I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or
Validate Date Using Regular Expressions in JavaScript. Regular expression is a great way of validating date. The only issue that many people face when it comes to regular expressions is the difficulty in understanding them as they contain various symbols and numbers. Each symbol or expression in a regular expression has its own meaning. Mar 06, 2020 - JavaScript’s implementation of Regex is useful for a range of string validation, formatting and iteration techniques. This article acts as an introduction to using regular expressions in JavaScript… The REGEX (Regular Expression) is the easiest way to validate Full Name (first name + last name) format in JavaScript. In the following code snippet, we will show you how to validate the first and last name with Regular Expression using JavaScript. test() - This function is used to perform a regular expression match in JavaScript.
Sep 11, 2018 - In this tutorial we will be discussing how to use regular expression in JavaScript. Regular expression is the most important part in form validations and it is widely used for search, replace and web crawling systems. A regular expression is also known as RegEx. @kommradHomer -- a "regex invalid" address is almost always valid, because whatever regex you use to validate an email address is almost certainly wrong and will exclude valid email addresses. An email address is name_part@domain_part and practically anything, including an @ , is valid in the name_part; The address foo@bar@machine.subdomain ... In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String
22/3/2011 · Here we create a RegExp pattern and use the match to validate it. Javascript validation check for a word. This type of javascript validation will check if the entered text contains a particular word round brackets are used to find out complete words When a regex has the global flag set, test () will advance the lastIndex of the regex. (RegExp.prototype.exec () also advances the lastIndex property.) Further calls to test (str) will resume searching str starting from lastIndex. The lastIndex property will continue to increase each time test () returns true. 8/3/2012 · The following regex is fine for your first name spec: var nameRegex = /^ [a-zA-Z\-]+$/; Adding digits for your username check is straightforward: var usernameRegex = /^ [a-zA-Z0-9]+$/; Note: There are many ways to write regular expressions. I've chosen to provide a version that matches what you started with.
Apr 16, 2020 - The goal of the function is to return true if the given code begins with a 1, 2, or 3 and returns false otherwise. Recently one of my Twitter followers asked me how they might validate password strength using regular expressions (RegEx) in their code. Regular expressions via Wikipedia: A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. Aug 14, 2015 - I'm attempting to validate a field name to match a certain format in JavaScript using Regular Expressions. I need the string inputted to resemble this: word\word\word So anything inputted can't be...
22/5/2019 · Given a URL, the task is to validate it. Here we are going to declare a URL valid or Invalid by matching it with the RegExp by using JavaScript. We’re going to discuss a few methods. Example 1: This example validates the URL = ‘https://www.geeksforgeeks ’ by using Regular Expression. Email input field validation is mandatory before submitting the HTML form. Email validation helps to check if a user submitted a valid email address or not. Using regular expression in JavaScript, you can easily validate the email address before submitting the form. We'll use the regular expression for validate an email address in JavaScript. Sep 27, 2018 - Our regex actually does a more accurate job than the HTML filtering built into the browser. ... If your app has a server, the server needs to validate the email as well, because you can never trust client code, and also JavaScript might be disabled on the user browser.
Javascript example to validate decimal numbers using regular expression example check if input has a decimal value validation to check two digit after …
How To Use Asp Net Regularexpressionvalidator On A Textbox
Phone Number Verification Without Regular Expression
Validate Form Using Javascript Regular Expressions Without
How To Use Regular Expressions Regex In Testim
Javascript Regular Expression How To Create Amp Write Them In
Form Input Validation Using Only Html5 And Regex
Laravel 5 4 Validation With Regex Stack Overflow
Basic Regex In Javascript For Beginners Dev Community
Beginning To Use Regex In Javascript By Cristina Murillo
How To Check Password Validation Dynamically Using Jquery
A Guide To Javascript Regular Expressions
Regex A Simple Example On How To Use Regex For Validation In
Java Email Validation Email Regex Java Journaldev
Javascript Regular Expression How To Create Amp Write Them In
How To Validate Url Using Regular Expression In Javascript
The Regex Table Variable In Google Tag Manager Simo Ahava S
Javascript Regular Expressions The Animated Guide 2021
Javascript Regular Expression How To Create Amp Write Them In
Javascript Form Validation Using Regular Expressions
0 Response to "20 How To Use Regex Validation In Javascript"
Post a Comment