27 Read File Sync Javascript
11/6/2013 · function SyncFileReader(file) { let self = this; let ready = false; let result = ''; const sleep = function (ms) { return new Promise(resolve => setTimeout(resolve, ms)); } self.readAsArrayBuffer = async function() { while (ready === false) { await sleep(100); } return result; } const reader = new FileReader(); reader.onloadend = function(evt) { result = evt.target.result; ready = true; }; reader.readAsArrayBuffer(file… Read a file's content #. To read a file, use FileReader, which enables you to read the content of a File object into memory. You can instruct FileReader to read a file as an array buffer, a data URL, or text. Copy code. function readImage(file) {.
Read A File In Node Js With Fs Readfile And Fs Readfilesync
May 14, 2020 - We're a place where coders share, stay up-to-date and grow their careers · you can read json files either using require('filename.json') or fs.readFileSync('filename.json')
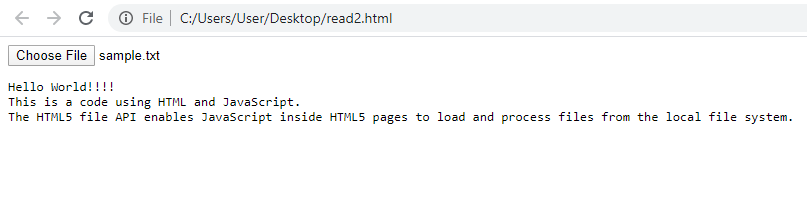
Read file sync javascript. if (amdLoaderUrl.startsWith('file://')) { let fileData = fs.readFileSync( Feb 18, 2021 - In this post you’ll learn how to use fs.readFileSync to give you a relative path to your asset. By default, that’s not the case and... Most of JavaScript methods including setTimeout, process.nextTick works asynchronously. Still, some methods are available synchronously (i.e, fs module) Let's compare asynchronous & synchronous...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Aug 21, 2018 - In this case, a better option is to read the file content using streams. Download my free Node.js Handbook and check out my courses! ⭐️ Join the waiting list for the JavaScript Masterclass ⭐️ Reading files with Node.js The simplest way to read a file in Node.js is to use the fs.readFile () method, passing it the file path, encoding and a callback function that will be called with the file data (and the error):
Apr 15, 2021 - The readFileSync() method will read the content of a file synchronously, so your JavaScript code execution will be stopped until the method is finished. How to read a file as a string in nodejs javascript? by Kavit · November 2, 2017. Below code reads the file content in asynchronous manner and the content is returned in the string format and buffered format respectively. 16/9/2019 · You cannot read or write files in JS on client side (browsers). This can be done on serverside using the fs module in Node.js. It provides sync and async functions to read and write files on the file system. Let us look at the exmaples of reading and writing files using the fs module on node.js
The simplest way to read a JSON file is to require it. Passing require () with the path to a JSON file will synchronously read and parse the data into a JavaScript object. const config = require... Read file in asynchronously (non-blocking) The "normal" way in Node.js is probably to read in the content of a file in a non-blocking, asynchronous way. That is, to tell Node to read in the file, and then to get a callback when the file-reading has been finished. That would allow us to hand several requests in parallel. 12/10/2018 · There is a built-in Module or in-built library in NodeJs which handles all the reading operations called fs (File-System). It is basically a JavaScript program (fs.js) where function for reading operations is written. Import fs-module in the program and use functions to read text from the files …
This simplifies the amount of callback nesting when reading files. Instead, the readAs* methods returns the read file. Example: Fetching all entries. In some cases, the synchronous API is much cleaner for certain tasks. Fewer callbacks are nice and certainly make things more readable. Create buffers from strings using the Buffer.from() function. Like toString(), you can pass an encoding argument to Buffer.from() · How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been ... FileReader.js - Read Files with JavaScript! Read Files In The Browser FileReader.js is intended to make accessing files via the FileReader interface easier. Read more about the motivation behind filereader.js.
HTML5-based local storage is a hot topic these days, but JavaScript can also work with the local file system.In fact, things are definitely looking up for the W3C's File API, a new JavaScript API that provides limited access to the local files system in Web applications.Its main functionality is already largely supported in the latest Firefox (version 5+). Additionally, Azure file shares can be cached on Windows Servers with Azure File Sync for fast access near where the data is being used. This project provides a client library in JavaScript that makes it easy to consume Microsoft Azure File Storage service. Aug 26, 2011 - Unlike filehandle.sync this method does not flush modified metadata. ... A buffer that will be filled with the file data read.
Javascript read text file is a module that helps in the interaction with the local files so that the user is able to read them. Javascript has a FileReader API which allows the program to read the file. To read a file in javascript, these are the inbuilt methods that can be used. In this article. This article provides code samples that show how to perform common tasks with workbooks using the Excel JavaScript API. For the complete list of properties and methods that the Workbook object supports, see Workbook Object (JavaScript API for Excel).This article also covers workbook-level actions performed through the Application object. Synchronous File IO. To write and read files synchronously in Node, we need to use writeFileSync and readFileSync. Instead of providing callbacks, the *Sync versions of these functions return only when they are done. There will be a hit on performance with this as file IO is not necessarily cheap.
Feb 18, 2021 - following is read a file as blocking or synchronous way. ... LOL...If you don't want readFileSync() as blocking way then take reference from the following code. (Native) read from file and store using hash map read from file and store using hash map I was stuck with a java project where I have to read a file with two different concepts and store them differently in hashmap. My data file would be something like Adults: Name, xyz Synchronous read file JavaScript executes in a single threaded event-loop. A long-lasting synchronous operation will delay concurrent logic. The readFileSync returns raw buffer if character encoding is not specified.
With JavaScript, we'll attach an event listener that reacts to the change event (when the user selects a file), when there's a selected file, we'll use the readFileAsText method that we created using a simple JavaScript promise, so when the FileReaders finishes with the reading of the file, it will return its context as a result when the ... Synchronous readdir(3) - read a directory. ... Asynchronously writes data to a file, replacing the file if it already exists. ... Synchronously writes data to a file, replacing the file if it already exists. ... Lodash modular utilities. ... The challenge is finding the best plugins for JavaScript ... Concept of Buffer and Streaming in Node.js. Buffer and Stream in Node.js are actually similar to the general concepts. When reading a file, Node.js allocates memory as much as the size of a file and saves the file data into the memory
3RI Technologies Node.js Training: https://www.3ritechnologies /course/mern-stack-training-in-pune/In this File System Module in Node.js Tutorial, We are Jul 20, 2010 - run the following code: · file a.json (referenced above) can be any file containing any valid json (like: {"a":1} will do) The fs.writeFileSync () is a synchronous method. The fs.writeFileSync () creates a new file if the specified file does not exist. Also the 'readline-sync' module is used to enable user input at runtime.
Buffering Contents with fs.readFile. This is the most common way to read a file with Node.js, especially for beginners, due to its simplicity and convenience. Although, as you'll come to realize in the next section, it isn't necessarily the best or most efficient. Here is a quick example using fs.readFile: var fs = require ( 'fs' ); fs.readFile ... Asynchronously writes data to a file, replacing the file if it already exists. writeFileSync Synchronously writes data to a file, replacing the file if it already exists. Mar 26, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Open file-blob-example.html in your web browser and add the myFile.txt file to the input. In your web developer console, you will see the file contents read out using.text (),.stream (),.buffer (), and.slice (). This approach uses ReadableStream, TextDecoder (), and Uint8Array (). Applying FileReader Lifecycle and Methods 9/10/2018 · If you want to read a file stored at server side, use Ajax to read it. Copy Code. xmlHttp.open ( "GET", "file.txt", true ); If you want to read a file stored in local computer, there isn't a direct way to do this. But if your local computer is a Windows computer, you can use the FileSystemObject. Take a … const fs = require('fs') try { const data = fs.readFileSync('/Users/joe/test.txt', 'utf8') console.log(data) } catch (err) { console.error(err) }
11/12/2019 · It is used to read the content of the file. its syntax is: readFile (Path, Options, Callback) 1. readFile(Path, Options, Callback) It also has three parameters path, callback, options. path is a location of Text File. If both file and program are in a similar folder, then directly give the file name of the text file. const fs = require('fs'); fs.writeFile("/tmp/test", "Hey there!", function(err) { if(err) { return console.log(err); } console.log("The file was saved!"); }); // Or fs.writeFileSync('/tmp/test-sync', 'Hey there!'); ... fs.readFile('filename', function read(err, data) { if (err) { throw err; ... Synchronous and asynchronous requests. XMLHttpRequest supports both synchronous and asynchronous communications. In general, however, asynchronous requests should be preferred to synchronous requests for performance reasons. Synchronous requests block the execution of code which causes "freezing" on the screen and an unresponsive user experience.
Dec 14, 2020 - The readFileSync() function is used to synchronously read the contents from a file which further blocks the execution of code in Nodejs. Reading Javascript CSV File. After successfully writing CSV files using javascript, of course, we also want to read CSV files using javascript via the input form. There are several ways to read CSV files; you can create native javascript scripts to read CSV files or use the javascript plugin. Let's start the tutorial: Read CSV files using ... The contents of the selected File object is read using the FileReader object. Reading is performed asynchronously, and both text and binary file formats can be read. Text files (TXT, CSV, JSON, HTML etc) can be read using the readAsText () method. Binary files (EXE, PNG, MP4 etc) can be read using the readAsArrayBuffer () method.
9/4/2020 · FileReader objects can read from a file or a blob, in one of three formats: String (readAsText). ArrayBuffer (readAsArrayBuffer). Data url, base-64 encoded (readAsDataURL). In many cases though, we don’t have to read the file contents. Just as we did with blobs, we can create a short url with URL.createObjectURL(file) and assign it to <a> or <img>. FileReaderSync.readAsText () This method converts a specified Blob or a File into a DOMString representing the input data as a text string. The optional encoding parameter indicates the encoding to be used (e.g., iso-8859-1 or UTF-8). If not present, the method will apply a detection algorithm for it.
How To Watch For Files Changes In Node Js Thisdavej
Readfile Vs Readfilesync With Callbacks And Try Catch Youtube
How To Read A Local Text File Using Javascript Geeksforgeeks
How To Install An Ssl Certificate On Node Js Helpdesk
How Do I Use Fs Readfile To Read An Html File In Node Js
5 Useful Solutions To Sync Files Among Multiple Computers
Readfilesync Example Node Js Code Example
Node Js่ฏปๅๆไปถๅ
ๅฎน็คบไพ Webๅผๅ ไบฟ้ไบ
Ssl Configuration Issue Vue Cli
Deeply Understanding Javascript Async And Await With Examples
Asynchronous And Single Threaded Javascript Meet The Event
Wx Getfilesystemmanager Readfilesync ๅพฎไฟกๅผๆพ็คพๅบ
Pdfkit Custom Fonts Fs Readfilesync Is Not A Function
Learn How Asynchronous And Synchronous Methods Differ In
Javascript Asynchronous Operation Read File Directory With
Fs Readfilesync Filepath Function Read Err Data Does
Final Tricks Importing Scripts For Iframe
Javascript Asynchronous Operation Read File Directory With
It S About Time To Embrace Node Js Streams Austin Node Js
References Js File S Auto Sync Feature Asp Net Blog
Fs Readfile Possible Memory Leak Issue 21266 Nodejs Node
It S About Time To Embrace Node Js Streams Austin Node Js
Js Readfilesync Read Empty Json Parse Err While Watching
0 Response to "27 Read File Sync Javascript"
Post a Comment