28 Javascript Import Js File
// File 1: file-one.js // Declare and export some stuff export const transpiler = 'Babel' export const language = 'JavaScript' export const syntax = 'jsx' export const framework = 'React' // File 2: file-two.js // Import modules exported from file-one.js // and rename some of those imports // Note: line breaks are again just for readability ... A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Javascript Intellisense Issues
One of the major differences between require() and import() is that require() can be called from anywhere inside the program whereas import() cannot be called conditionally, it always runs at the beginning of the file. To use the require() statement, a module must be saved with .js extension as opposed to .mjs when the import() statement is used.
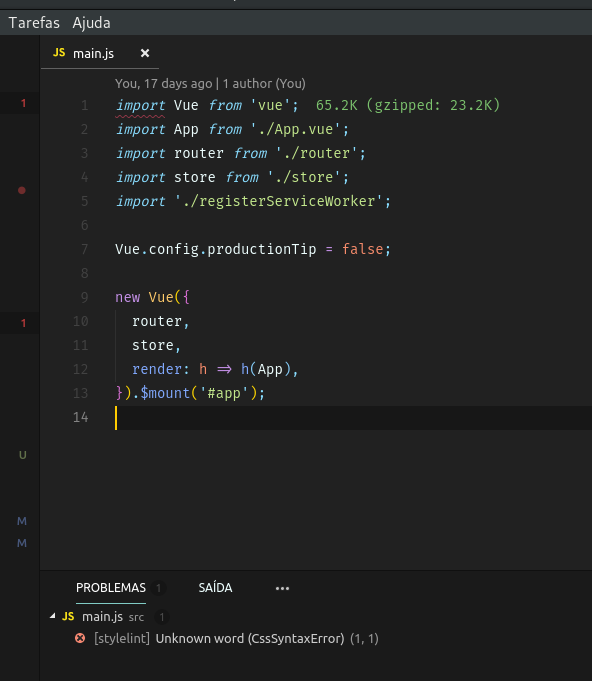
Javascript import js file. 16/4/2020 · Until some years ago, it was not possible to import one js file or block of code from one file into another but since 2015, ES6 has introduced useful ES6 modules. With the module standard, we can use import and export keyword and syntax given to include and export the module in JS respectively. js file import jquery; javascript how add jquery library in js file; import jquery in javascript; js file wit jquery; run jquery in js file; insert jquery from js; import jquery in js; html will not acticate jquery in js file; require jquery in file; import jquery into js file; jquery in js file; require jquery in js file; include jquery js ... The import directive loads the module by path ./sayHi.js relative to the current file, and assigns exported function sayHi to the corresponding variable.. Let's run the example in-browser. As modules support special keywords and features, we must tell the browser that a script should be treated as a module, by using the attribute <script type="module">.
Let's say the above file is saved with the name getDate.js Now if we want to import the above function in another Javascript file in the same folder we can write the code as: Question about importing js file in typescript fashion #2712. ericlu88 opened this issue on Apr 10, 2015 · 7 comments. Labels. Question. Comments. mhegazy added the Question label on Apr 10, 2015. danquirk closed this on Apr 10, 2015. In the example below, the default export with a named export is imported: // main.js import { default as Site, welcome } from './site.js'; new Site ('W3Docs'); Finally, if you try to import everything * as an object, the default property will be exactly the default export, like here:
Export default. In practice, there are mainly two kinds of modules. Modules that contain a library, pack of functions, like say.js above.; Modules that declare a single entity, e.g. a module user.js exports only class User.; Mostly, the second approach is preferred, so that every "thing" resides in its own module. Javascript Import Javascript Import statement is used to import bindings that are exported by another module. Using the Javascript import, the code is easier to manage when it is small and bite-size chunks. This is the thinking behind keeping functions to only one task or having files contain only a few or one component at a time. The module1.js JavaScript file is imported using the src attribute of script tag within the "head" section of the HTML file. Since the JavaScript file is imported, the contents are accessible within the HTML file. We create a button which when clicked triggers the JavaScript function.
Same issue here. Adding a .js in the import inside a TypeScript file does allow to compile it with the TypeScript compiler and will output files with working ESM imports.. However, when I add .js extensions on the imports, I can't get testing working. Tried with Mocha and Jest, but no luck so far: they complain the files with .js extension don't exist, which is correct, those files don't exist ... Consider the open source LWC recipes. First you need to install jQuery in lwc web-app using npm install jquery.OR any other library you want to use. After installation, you will see the file in node_modules folder. Now, you just need to get that library variable wherever you need. 2) The correct method. 2.1) - Create A New XMLHttpRequest. 3) Usage. 3.1) - Parse JSON string into object. In this tutorial, we will see how to Load JSON file locally using pure Javascript. the proper way to include or load the JSON file in a web application, Instead of using JQuery we'll The correct method using XMLHttpRequest.
5/5/2019 · The require statement was used for the last thousand years and people seemed to be happy about them. Except when they weren’t, and used use or some other freakish statements to reference functions in other files.. Once JS is referenced using require you can use functions/methods within them in more than one way (incl. this singleton pattern which you shouldn’t use). JavaScript Files JavaScript files are not HTML files or CSS files. Always end with the js extension; Only include JavaScript; It's customary to put all JavaScript files in a folder called js on websites, like so: Simple Demo of Including JavaScript. Here's a very simple demonstration of how to include an external JavaScript file into an HTML ... How to import local json file data to my JavaScript variable? Javascript Web Development Object Oriented Programming We have an employee.json file in a directory, within the same directory we have a js file, in which we want to import the content of the json file.
You use the import statement, followed by a comma-separated list of the features you want to import wrapped in curly braces, followed by the keyword from, followed by the path to the module file — a path relative to the site root, which for our basic-modules example would be /js-examples/modules/basic-modules. Either you import the code from an external JS file, or you embed the JavaScript code right into your HTML page. Both approaches will get the job done, but you should try to favor importing your code from an external file, as it's best to separate your HTML code from your JavaScript code for easy readability. Since ECMAScript 6 (or ES6) you can use the export or import statement in a JavaScript file to export or import variables, functions, classes or any other entity to/from other JS files. For example, let's suppose you've two JavaScript files named "main.js" and "app.js" and you want to export some variables and functions from "main.js" to "app.js".
The import and export statements are one of the most crucial features of JavaScript ES6. It allows you to import and export JavaScript classes, functions, components, constants, any other variables between one JavaScript file and another. This feature allows you to organize your JavaScript code into small, bite-size files. Import an entire module's contents This inserts myModule into the current scope, containing all the exports from the module in the file located in /modules/my-module.js. import * as myModule from '/modules/my-module.js'; Here, accessing the exports means using the module name ("myModule" in this case) as a namespace. Method 2: Using ES6 import module (Web Runtime Environment only) If we want to access the json file while running JavaScript in browser, we can use the ES6 import syntax to do that.
When you import.js file in your html the order is very important and you can think that the previous \<script> tags are like imports. For example if you script requires jQuery you will need to import first jQuery library and then your script: <script src= "jquery-3.3.1.min.js" ></script> <script src="your-script.js"></script> When a JavaScript file is imported this way, it is imported with a qualifier. The functions in that file are then accessible from the importing script via the qualifier (that is, as Qualifier.functionName (params)). Sometimes it is desirable to have the functions made available in the importing context without needing to … In order to include these variables and functions in another file, say main.js, you can use the import keyword as: // import the variables and function from module.js import { message, number, multiplyNumbers } from './modules.js'; console.log(message); // hello world console.log(number); // 10 console.log(multiplyNumbers(3, 4)); // 12 console.log(multiplyNumbers(5, 8)); // 40
This is because we are going to create an index.js file where all of the files in our folder will be imported and exported again. Using the example of our components folder, let's create a file in components/index.js. Next, we will use an uncommon feature of the export statement to be able to export from another file: 31/7/2019 · Output: Concatenate files Example: Here importing multiple JavaScript files into a single JavaScript file and calling that master JavaScript file from a function. External JavaScript file named as “main.js”. // This alert will export in the main file. alert ("Hello Geeks") External JavaScript file … Tip: To check if a JavaScript file is part of JavaScript project, just open the file in VS Code and run the JavaScript: Go to Project Configuration command. This command opens the jsconfig.json that references the JavaScript file. A notification is shown if the file is not part of any jsconfig.json project.
3/6/2009 · So all you would need to do to import JavaScript is: $.import_js('/path_to_project/scripts/somefunctions.js'); I also made a simple test for this at Example. It includes a main.js file in the main HTML and then the script in main.js uses $.import_js() to import an additional file called included.js, which defines this function:
Using External Js Files In Angular Franco Morales
Code Using Function From Another File Without Import Export
How To Include Javascript Code In Html Page The Engineering
Calling Functions From Another File In Node Js James D
How To Add External Css Amp Javascript File In Angular Project
Import Js File To Another Js File Code Example
Omnis Technical Notes Using The Javascript Worker Object
One Js File Inside Use Export Function And Module Exports
Add Custom Javascript Into Your Wordpress Site
Replacing A Javascript File In A Test Script
Create And Link An External Javascript File
Javascript Import How To Import Modules In Javascript
How To Use An Es6 Import In Node Js Geeksforgeeks
How To Import Multiple Elements From A Javascript File
Dynamic Import Of Javascript Module From Stream Stack Overflow
Pdf Automated Refactoring Of Client Side Javascript Code To
How To Call External Javascript Function Which Is In Separate
Fix Unknown Word Error In Javascript Files Issue 3434
Js Introduction Finereport Help Document
Building Your First React App React Is An Open Source
Import One Js File Into Another In The Plain Js Web Design
How To Import A Typedef From One File To Another In Jsdoc
Node Js Upload Import Excel File Data Into Database Bezkoder
Dynamic Import Of Javascript Module From Stream Stack Overflow
How To Include Javascript Code In Html Page The Engineering
Loading Script Files Dynamically
0 Response to "28 Javascript Import Js File"
Post a Comment