21 Javascript Es6 Class Constants
Javascript ES6 gave developers the ability to build complex classes and keep up with the demands of the modern web. This article describes how to create Javascript classes. It also explains the current level of support for Javascript classes across various browsers and what this means for cross-browser compatibility. Jul 20, 2021 - Constants are block-scoped, much like variables declared using the let keyword. The value of a constant can't be changed through reassignment, and it can't be redeclared.
Javascript Es6 Let And Const Javascript Es6 Includes Two
One of the most important parts of JavaScript ES6 is the import and export statements. It lets you move JavaScript classes, methods, components, constants, and any other variables from one ...
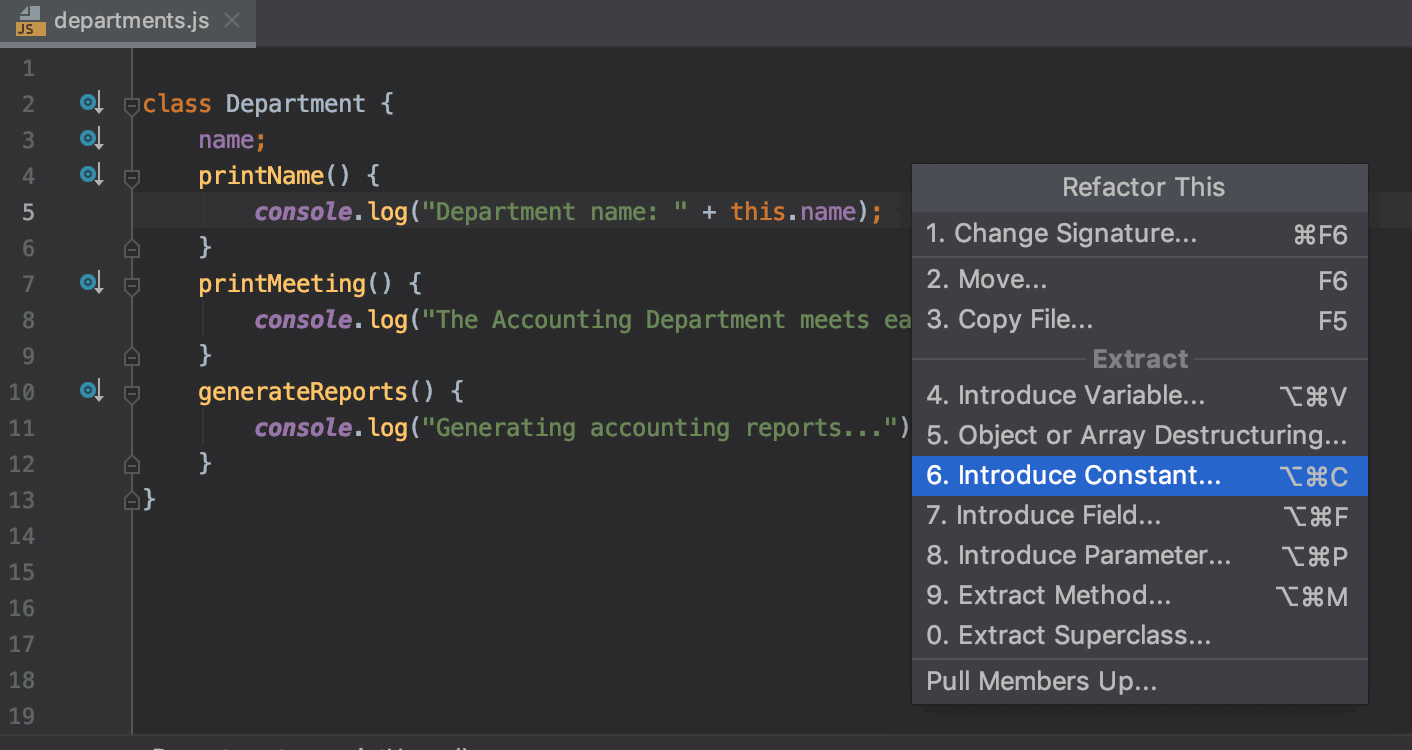
Javascript es6 class constants. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics. Introduction to the JavaScript const keyword ES6 provides a new way of declaring a constant by using the const keyword. The const keyword creates a read-only reference to a value. const CONSTANT_NAME = value; May 04, 2016 -
9/10/2020 · Const Declaration Keyword has been introduced in ES6 to bring the ability to declare variables with constant values which can be set only within the declaration. Const are constant values … Nov 23, 2018 - ES6 is the latest stable implementation of ECMAScript, the open standard on which JavaScript is based. It adds a number of new features to the language including an official module system, block-scoped variables and constants, arrow functions, and numerous other new keywords, syntaxes, and ... Basically the import and export syntax is used everywhere where we write JavaScript and then transcompile and bundle it to "old-school" javascript. But the time when it can only be used in conjunction with compilers like Babel is over. Meanwhile Node.js also supports the so-called ES6 modules, and in the browser we can use them if we want to.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Javascript ES6 Previous Next ... JavaScript const. The const keyword allows you to declare a constant (a JavaScript variable with a constant value). Constants are similar to let variables, except that the value cannot be changed. ... JavaScript Classes are templates for JavaScript Objects. ES6 gives a new way to declare a constant using the const keyword. The keyword const creates a read-only reference to the value. There are some properties of the const that are as follows:
ES6 (ECMAScript2015) là một bản nâng cấp lớn cho JavaScript. Trong bài viết này, chúng ta sẽ tìm hiểu về class es6 để hiểu sâu hơn những cụm từ hay những khái niệm như class, object, static properties, constructor, inheritance, super và extends trong JavaScript. Javascript ES6 classes have explicit constructors, may have class properties, and may have static methods, which are associated with the class rather than an instance of that class. Although the syntax for simple constructors is not dramatically different in ES6, the additional capabilities of ES6 classes are a major functional addition. Yes, ES6 classes do obscure the true nature of JavaScript inheritance. There is an unfortunate disconnect between what a class looks like (its syntax) and how it behaves (its semantics): It looks like an object, but it is a function. My preference would have been for classes to be constructor ...
Oct 03, 2020 - The constructor property returns the constructor function for all JavaScript variables. ... how to Create a class called Person which defines the generic data and functionality of a human with multiple interests in javascript Jun 04, 2018 - They enhance JavaScript by providing a way to define block-scope variables and constants. This article is one of many covering new features of JavaScript introduced with ES6, including Map and WeakMap, Set and WeakSet, new methods available for String, Number, and Array, and the new syntax ... The ability to create constant variables is something javascript was missing for a long time. With ES6 you'll be able to create constants and make sure its value won't be changed during the application execution. The syntax for creating constants is as follow: const SERVER_URL = "".
I want to implement constants in a class, because that's where it makes sense to locate them in the code. So far, I have been implementing the following workaround with static methods: class MyCl... May 04, 2016 - ES6 brings classes to JavaScript, but some things are not immediately obvious or entirely supported. For instance, there is no obvious way to create static class constants. ES6 classes brings a new syntax for getters and setters on object properties Get and set allows us to run code on the reading or writing of a property. ES5 had getters and setters as well but was not widely used because of older IE browsers. ES5 getters and setters did not have as nice of a syntax that ES6 brings us.
How do declare constant class members in JS? : javascript, ES6 brings classes to JavaScript, but some things are not immediately obvious or entirely supported. For instance, there is no obvious way to The static keyword defines a static method for a class. Static methods aren't called on instances ... Modern JavaScript - Imports, Exports, Let, Const, and Promises in ES6+. Over the past few years, there have been many updates to the JavaScript language. And these updates are very useful if you want to improve your coding. Keeping abreast of the newest developments in the language is really important. It can help you get a higher paying job ... Jan 16, 2020 - Some time ago, you could read about our top 10 ES6 features (if you haven’t yet, check it out here). There is no doubt that many fantastic things were introduced in this (and all subsequent) versions…
Jul 09, 2021 - Constants are variables whose values can’t be changed. These are useful for creating immutable touchpoints for a program, used to enhance readability and maintainability. Javascript uses the const keyword to denote constants. In this blog entry we’ll cover the basics as well as how constants ... 22/5/2021 · class Person { //A popular JS convention is to capitalize the class name // The constructor method with the properties inside it constructor(firstName, lastName) { this.firstName = firstName; this.lastName = lastName; } // A method that returns the fullname of the person fullName() { return `${this.firstName} ${this.lastName}`; } } To follow this ES6 tutorial, you should have a good knowledge of JavaScript up to ES5. Section 1. New ES6 syntax. let - declare block-scoped variables using the let keyword. const - define constants using the const keyword. Default function parameters - learn how to set the default value for parameters of a function.
With ES2015 (ES6), with get built-in support for modules in JavaScript. Like with CommonJS, each file is its own module. To make objects, functions, classes or variables available to the outside world it's as simple as exporting them and then importing them where needed in other files. Angular 2 makes heavy use of ES6 modules, so the syntax ... JavaScript does not have the concept of privacy in terms of properties or methods. Although a leading underscore is a common convention to mean private , in fact, these properties are fully public, and as such, are part of the public API contract. In this example, the hi property is assigned a function. When it is invoked off of a Person object, the keyword this will correspond to the newly constructed Person object.. Although methods can be defined this way, this approach does have a downside. Every time an instance of Person is created, a new function is defined and assigned to the hi property of that object.
See how cleaner and more concise your JavaScript code can look and start coding in ES6 now !! "A good programming language is a conceptual universe for thinking about programming." — Alan J. Perlis ... Support for constants (also known as "immutable variables"), i.e., variables which cannot ... ES6 In Depth is a series on new features being added to the JavaScript programming language in the 6th Edition of the ECMAScript standard, ES6 for short.. The feature I'd like to talk about today is at once humble and startlingly ambitious. When Brendan Eich designed the first version of JavaScript back in 1995, he got plenty of things wrong, including things that have been part of the ... Active Oldest Votes. 1. For backwards compatibility, I would create a read-only property of the encapsulating object, which could be the global object: Object.defineProperty (this, 'CONST', { value: 123, writable: false }); See: Object.defineProperty - writable attribute. Otherwise, ES6 has a const keyword.
Also, const will allow you to do this: const foo = {}; foo.bar = 2; IMO const is another case of people trying to shove unnecessary Java principles into JavaScript when no one really needs it (like 'new' or 'this'). At the end of the day do you really need it? 17/9/2015 · 2. You can create a way to define static constants on a class using an odd feature of ES6 classes. Since statics are inherited by their subclasses, you can do the following: const withConsts = (map, BaseClass = Object) => { class ConstClass extends BaseClass { } Object.keys(map).forEach(key => { Object.defineProperty(ConstClass, key, { ... ES6 Classes in JavaScript tutorial. Another fantastic feature that ES6 offers is the ability to create classes within JavaScript, technically, Classes were introduced in ES5 and were introduced to make it easier to use JavaScript already existing 'prototype-based inheritance mechanism. However, this may have been introduced but it is not 100% ...
JavaScript Constants. The const keyword was also introduced in the ES6(ES2015) version to create constants. For example, const x = 5; Once a constant is initialized, we cannot change its value. const x = 5; x = 10; // Error! constant cannot be changed. console.log(x) Simply, a constant is a type of variable whose value cannot be changed. Public static fields. Public static fields are useful when you want a field to exist only once per class, not on every class instance you create. This is useful for caches, fixed-configuration, or any other data you don't need to be replicated across instances. Public static fields are declared using the static keyword. May 13, 2017 - I decided to make a quick post today about an elegant solution for describing constant variables in your applications. I’ve had a quick look around the web and I haven’t seen any mature, organic…
En Javascript se utiliza una sintaxis muy similar a otros lenguajes como, por ejemplo, Java. Declarar una clase es tan sencillo como escribir lo siguiente: // Declaración de una clase class Animal {} // Crear o instanciar un objeto const pato = new Animal (); El nombre elegido debería hacer referencia a la información que va a contener dicha ... JavaScript. Const. The const keyword was introduced in ES6 (2015). Variables defined with const cannot be Redeclared. Variables defined with const cannot be Reassigned. Variables defined with const have Block Scope. 17/8/2020 · Class expression: const A = class{} ... In JavaScript, classes inherit from other classes using the keyword extends. When a class extends another class, it uses prototype-based inheritance. ... But, before you go using ES6 classes in your React application, ...
Jul 20, 2021 - The static keyword defines a static method or property for a class. Neither static methods nor static properties can be called on instances of the class. Instead, they're called on the class itself. JavaScript is an implementation of that language. Es6 brings many promising features like arrow function, template string, const, let and many more. Let's start with easy to understand features and then move on to more complex features. const and let. const (Constants) are block scoped ( only available in local scope). JavaScript ES6 (also known as ECMAScript 2015 or ECMAScript 6) is the newer version of JavaScript that was introduced in 2015. ECMAScript is the standard that JavaScript programming language uses. ECMAScript provides the specification on how JavaScript programming language should work. This tutorial provides a brief summary of commonly used ...
ES6 classes give us a convenient syntax for defining the state and behavior of objects that will represent our concepts. ES6 classes make our code safer by guaranteeing that an initialization... Aug 12, 2019 - Many JavaScript style guides suggest capitalizing constant names. Personally, I rarely see this convention used where I thought it should be. This was because my definition of a constant was a bit off. I decided to do a bit of digging and become a bit more familiar with this convention. ES6 Syntax and Feature Overview. Clear descriptions and comparisons of ES5 and ES6 features. ECMAScript 2015, also known as ES6, introduced many changes to JavaScript. Here is an overview of some of the most common features and syntactical differences, with comparisons to ES5 where applicable. Note: A commonly accepted practice is to use const ...
Difference Between Static and Const in JavaScript with javascript tutorial, introduction, javascript oops, application of javascript, loop, variable, objects, map, typedarray etc.
Web Tools Platform 3 8 News The Eclipse Foundation
Variables And Assignment Javascript For Impatient
9 Es6 Features Every Javascript Developer Should Know By
5 Javascript Bad Parts That Are Fixed In Es6
Introduction To Es6 Main Concepts
Jsdoc Doesn T Generete Doc For Es6 Functions Like Export
Javascript Es6 Write Less Do More By Saidhayani We Ve
5 Tips To Organize Your Javascript Code Without A Framework
Introduction To Es6 Main Concepts
Introduction To Es6 Main Concepts
Es6 Es7 Es8 Amp Writing Modern Javascript Pt1 Scope Let
16 2 Const Topics Of Javascript Es6
The Most Useful Features In The Latest Javascript Since Es6
Fullstack React React Createclass Vs Es6 Class Components
Javascript Es6 5 New Abstractions To Improve Your Code
Making Sense Of Es6 Class Confusion Toptal
Es6 Cheat Sheet By Arrow96 Download Free From Cheatography
Refactoring Javascript Webstorm
Introduction To Babel Javascript Compiler For Es6 Pubnub
Javascript Es6 Tutorial A Complete Crash Course On Modern Js
0 Response to "21 Javascript Es6 Class Constants"
Post a Comment