25 Use Node Module In Javascript
If it's using EcmaScript module format (ESM), so not in your case, you can import with import * as DeviceDetector from "device-detector-js". The syntax you are trying isn't valid in either case. See here about how to tell Node that your code is using EcmaScript module format, if you wish to do that. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Why Use Node Js In Ecommerce Development
Never reference node_modules. New JavaScript developers may think OK, I will use the versioned dependency like this: # Use `npm run …` instead $ ./node_modules/.bin/tap Just use an npm script as described above. The effect is the same. I've also seen references to node_modules when importing modules. Don't.
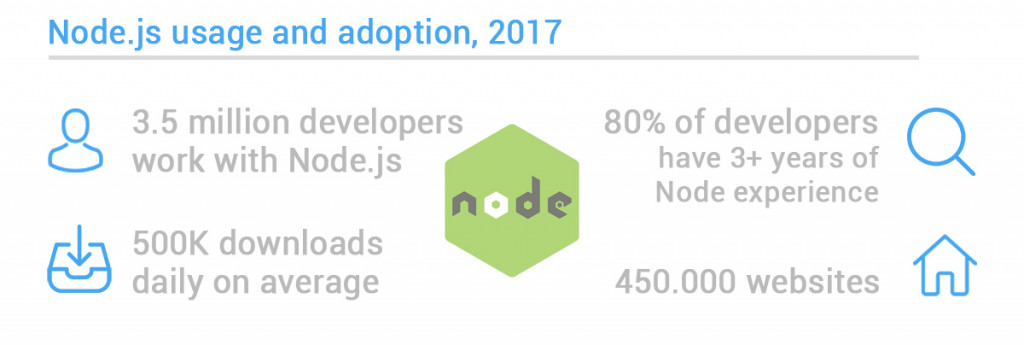
Use node module in javascript. Using the esm module. Installation npm install esm. Now try executing the program written in the index.js file earlier by typing node -r esm index.js in the terminal. Another way to use the esm module is by creating another file say server.js that loads esm before the actual application. In the server.js file write the below code Node uses its own module system called CommonJS, while browsers support ECMAScript (ES). This has forced front-end web developers to use build tools called bundlers in order to convert JavaScript from CommonJS to ES. Snowpack is a lightweight, easy-to-use JavaScript build tool designed for front-end developers. I have found it to work ... May 04, 2021 - Today, thanks to CommonJS, we have JavaScript in server-side applications, command line tools, desktop GUI-based and hybrid applications (Titanium, Adobe AIR, etc.), and more. By all means, every time you use a require(), you’re in fact using the implementation of CommonJS modules, which comes within Node...
The module.exports is a special object which is included in every JavaScript file in the Node.js application by default. The module is a variable that represents the current module, and exports is an object that will be exposed as a module. So, whatever you assign to module.exports will be ... The Asynchronous Module Definition (AMD) format is used in browsers and uses a define function to define modules. The CommonJS (CJS) format is used in Node.js and uses require and module.exports to... Yes Node does. That loader uses the CommonJS module format. The CommonJS module format is non-optimal for the browser, and I do not agree with some of the trade-offs made in the CommonJS module format. By using RequireJS on the server, you can use one format for all your modules, whether they ...
Mar 30, 2016 - For example, you may use the process module instantly (without any require(…)), as in Node. ... There are, however, some small changes between Node's and node-webkit's API. (See “Changes related to node” for details.) ... If a 3rd party module is written totally in JavaScript (i.e. does ... Node uses two core modules for managing module dependencies: The require module, which appears to be available on the global scope — no need to require ('require'). The module module, which also appears to be available on the global scope — no need to require ('module'). The crypto module is a wrapper for OpenSSL cryptographic functions. It supports calculating hashes, authentication with HMAC, ciphers, and more! The crypto module is mostly useful as a tool for implementing cryptographic protocols such as TLS and https.For most users, the built-in tls module and https module should more than suffice. However, for the user that only wants to use small parts of ...
Node.js organizes this complexity using modules, which are any single JavaScript files containing functions or objects that can be used by other programs or modules. A collection of one or more modules is commonly referred to as a package, and these packages are themselves organized by package managers. Let's use the built-in OS module to get some information about your computer's operating system: In your command line, open the Node.js CLI. You'll see the > prompt letting you know you're using Node.js after entering: node. To identify the operating system you are currently using (which should return a response letting you know that you're on Windows), enter: os.platform() The import directive loads the module by path ./sayHi.js relative to the current file, and assigns exported function sayHi to the corresponding variable.. Let's run the example in-browser. As modules support special keywords and features, we must tell the browser that a script should be treated as a module, by using the attribute <script type="module">.
Using rust modules for front-end (plain JavaScript) Building wasm from rust We wont be able to use the rust file or the output binary directly onto the web project because JavaScript doesn't ... In this post we take a look at how to use custom nodejs packages with AWS Lambda, including building and packaging native nodejs modules for use in your Lambda functions. To do the steps below, you'll need an EC2 instance or a similar machine running Amazon Linux with nodejs installed. Using Existing JavaScript Packages with Lambda Dec 14, 2015 - The idea of writing JavaScript modules isn't new, but with the arrival of ES6 and the industry settling on npm as the preferred package manager for JavaScript, we're starting to see many devs migrate away from the above workflow and standardizing on using ES6 and npm. Hold on. `npm`? Isn't that for Node...
A simple introduction to Node.JS modules by example. Node.js is a collection of many open-source libraries. Node.js uses Google's open-source V8 engine as the default JavaScript engine which executes the JavaScript code. The V8 engine is written in... As per above syntax, specify the module name in the require() function. The require() function will return an object, function, property or any other JavaScript type, depending on what the specified module returns. The following example demonstrates how to use Node.js http module to create ...
Node.js 12 introduced support for the import statement behind a --experimental-modules flag and a package.json configuration option.Node.js 14 removes the need for the --experimental-modules flag, but you still need to configure your package.json.Here's how you can use ES6 imports in Node. Setup. Suppose you have two JavaScript files: index.js and test.js. ... Browserify lets you require('modules') in the browser by bundling up all of your dependencies. ... First install node, which ships with npm. Then do: ... Browsers don't have the require method defined, but Node.js does. With Browserify you can write code that uses require in the same way that ... The default format of modules in Node.js is the CommonJS. To make Node.js understand ES modules format, you have to explicitly make so. Node.js uses ECMAScript modules format if: The module's file extension is.mjs
If you need a version of Node.js/npm that is not already installed on the agent: In the pipeline, select Tasks, choose the phase that runs your build tasks, and then select + to add a new task to that phase.. In the task catalog, find and add the Node Tool Installer task.. Select the task and specify the version of the Node.js runtime that you want to install. Feb 28, 2021 - There is plenty more there to keep you busy and blow your mind. Bonus points for the first person who can tell me what ‘NODE_MODULE_CONTEXTS’ is and why it was added. [1] The module._compile method is only used for running JavaScript files. JSON files are simply parsed and returned via ... Aug 26, 2011 - Deprecated. In the past, this list has been used to load non-JavaScript modules into Node.js by compiling them on-demand. However, in practice, there are much better ways to do this, such as loading modules via some other Node.js program, or compiling them to JavaScript ahead of time.
defaultExport. Name that will refer to the default export from the module. module-name. The module to import from. This is often a relative or absolute path name to the .js file containing the module. Certain bundlers may permit or require the use of the extension; check your environment. Oct 05, 2020 - Documentation for the npm registry, website, and command-line interface Node.js has a built-in module called HTTP, which allows Node.js to transfer data over the Hyper Text Transfer Protocol (HTTP). To include the HTTP module, use the require () method: var http = require ('http'); Node.js as a Web Server
Consider modules to be the same as JavaScript libraries. A set of functions you want to include in your application. ... Node.js has a set of built-in modules which you can use without any further installation. In Node.js, a module is a collection of JavaScript functions and objects that can be used by external applications. Describing a piece of code as a module refers less to what the code is and more to what it does—any Node.js file or collection of files can be considered a module if its functions and data are made usable to external programs. Sep 28, 2020 - In this topic, you will learn how to use the JavaScript Module Design Pattern to reduce the chance that your code will conflict with other scripts on your web page. ... In JavaScript, when you define a variable using the var element, it is scoped within the function that it is defined.
In Node.js, the module system has come a long way from its earlier adoption of CommonJS. Today, ECMAScript modules (ES modules), which are now stable and fit for production use, are the official standard for packaging code for reuse in both client- and server-side JavaScript. Jul 20, 2021 - Fast forward a few years and we ... as JavaScript being used in other contexts (Node.js, for example). It has therefore made sense in recent years to start thinking about providing mechanisms for splitting JavaScript programs up into separate modules that can be imported when ... Jul 13, 2020 - So, while learning a JavaScript framework the knowledge of npm would be really useful to learn it in an easier manner. In this series of articles, we aimed to cover the following topics: ... NPM is the default package manager for node. It is used to install, share, and manage javascript packages ...
Summary: in this tutorial, you will learn about the Node.js http module and how to use it to create a simple HTTP server.. Introduction to the Node.js HTTP module. The http module is a core module of Node designed to support many features of the HTTP protocol.. The following example shows how to use the http module:. First, create a new file called server.js and include the http module by ... Aug 30, 2020 - Thank you, but I need to use module system in my project. – Rkcoder Aug 30 '20 at 9:36 ... Not the answer you're looking for? Browse other questions tagged javascript html module or ask your own question. So type the following command. It will install the modules inside the node_modules folder. Now, write the following code inside the start.js file. We will run this file, and it will allow us to run the import-export statement in the app.js file. The next step is to write the following code inside the data.js file.
For Node.js, this process of code sharing - both within individual projects and in external npm dependencies - is facilitated using module.exports or exports. How Node Modules Work How do we use module exports to plug an external module, or sensibly break our project down into multiple files (modules)? Node.js is a serverside application where you run javascript on the server. What you want to do is use the require function on the client. Your best bet is to just write the require method yourself or use any of the other implementations that use a different syntax like requireJS. To use the file system module in your Node.js projects, you'll first need to import this module. In Node.js, the file system module is represented by the acronym fs. So by simply passing fs to the required function (as shown in the code above), you now have access to the file system module.
Node.js treats JavaScript code as CommonJS modules by default. Authors can tell Node.js to treat JavaScript code as ECMAScript modules via the .mjs file extension, the package.json "type" field, or the --input-type flag. See Modules: Packages for more details. ESM is the standard JavaScript module system (ESM is a shorcut for JavaScript Modules, and is also called ESM, or EcmaScript modules, whereby "EcmaScript" is the official name for the JavaScript language). ESM is the "newer" module system, and is supposed to be a replacement for the regular Node.js module system, which is CommonJS (CJS ... Create a working directory for developing your Node.js module. Name this directory awsnodesample. Note that the directory must be created in a location that can be updated by applications. For example, in Windows, do not create the directory under "C:\Program Files". Install Node.js.
10 Best Practices To Containerize Node Js Web Applications
5 Major Companies That Use Node Js And Why By Daniel
Why Use Nodejs 10 Reason To Select Node Web Development
How To Use An Es6 Import In Node Js Geeksforgeeks
Build Node Js Apps With Visual Studio Code
Pros And Cons Of Node Js Web App Development Altexsoft
Why Use Node Js Onestop Devshop Saas Company
What Is Node Js A Comprehensive Guide
Import Validator Or Install Node Modules In Node Js Codez Up
Use Node Js For Backend When Why And Top Reasons Of Using
Creating A Secure Rest Api In Node Js Toptal
Advantages Amp Disadvantages Of Node Js Why To Use Node Js
Why Use Node Js 7 Examples Of Popular Node Js Apps Tsh Io
Node Js Creating And Using Modules Parallelcodes
Step By Step Guide To Use Es6 Modules Effectively With Node Js
Node Js What Is It Best Used For Railsware Blog
This Is What Node Js Is Used For Survey Results Risingstack
What Is Nodejs Why We Use Node Js What We Can Do With Node
Node Js Use Cases When Is It Worth Considering For Web App
Build Node Js Apps With Visual Studio Code
How Enterprises Benefit From Node Js Risingstack
0 Response to "25 Use Node Module In Javascript"
Post a Comment