20 Assign Callback To Variable Javascript
In this example, the variable i is a global variable. After the loop, its value is 5. When the callback functions are passed to the setTimeout() function executes, they reference the same variable i with the value 5.. In ES5, you can fix this issue by creating another scope so that each callback function references a new variable. In JavaScript, a callback is a function passed into another function as an argument to be executed later. Suppose that you the following numbers array: let numbers = [ 1, 2, 4, 7, 3, 5, 6 ]; Code language: JavaScript (javascript) To find all the odd numbers in the array, you can use the filter () method of the Array object.
Cannot Store Data In Callbacks Dash Plotly Community Forum
To pass variables into callback function, you can use bind(). I will show you how. The dummy function that takes 1 sec to callback. To begin, let's say we have this dummy() function that will do some lengthy processing, and then callback. This is common. For example, a simple http request will use a callback function for the http response.
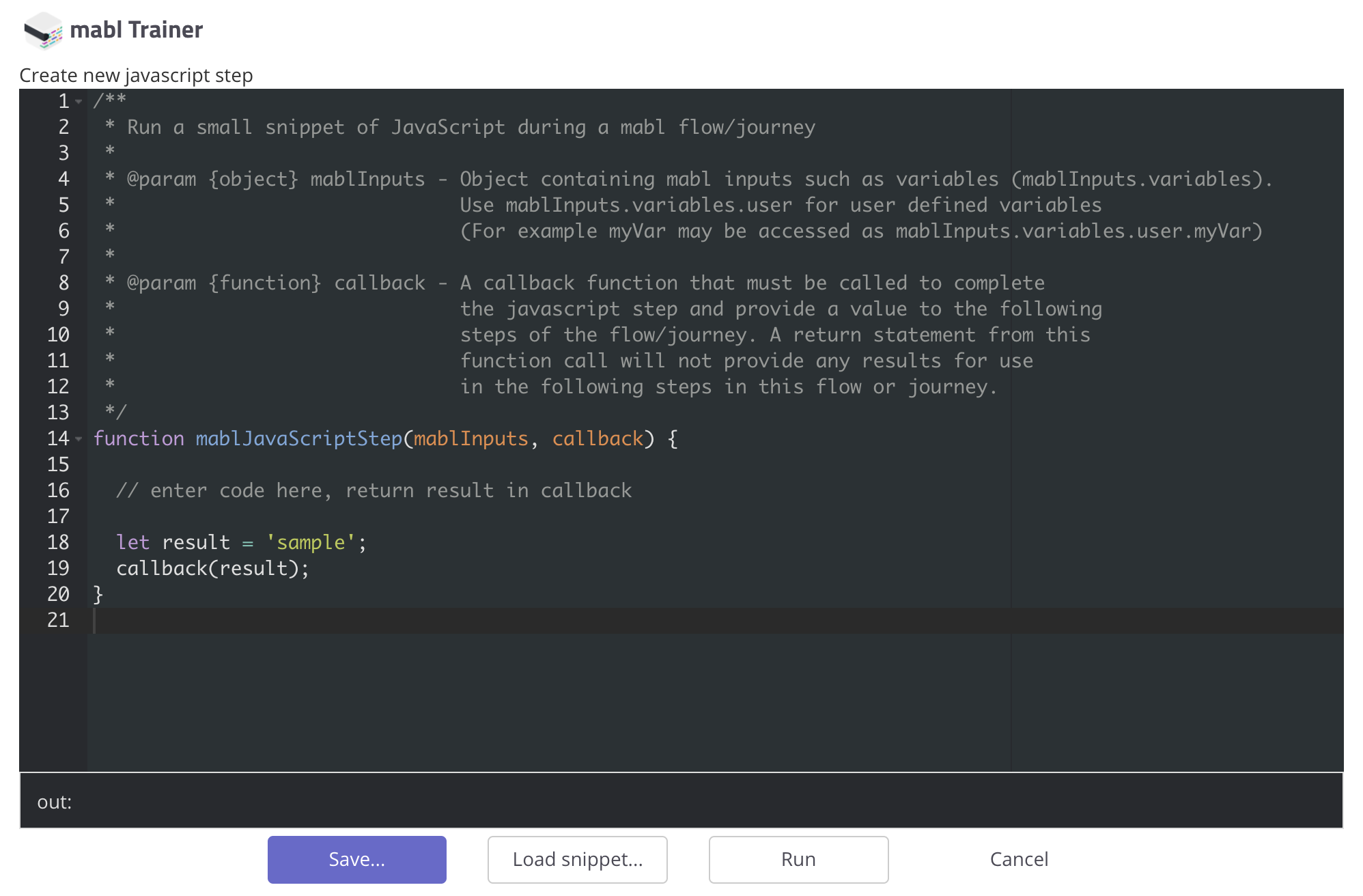
Assign callback to variable javascript. When you define a function this way, the Javascript runtime stores your function in memory and then creates a reference to that function, using the name you've assigned it. That name is then accessible within the current scope. This can be a very convenient way to create a function, but Javascript does not require you to assign a name to a ... JavaScript JavaScript Array Iteration Methods Array Manipulation Remove Array Items with filter ... Assigning the filter callback to a variable. When you assign the callback to a variable, then pass the variable through the filter function, how does the program know that the element refers to the items in the array? I thought the element would ... JavaScript Callbacks. A callback is a function passed as an argument to another function. Using a callback, you could call the calculator function ( myCalculator ) with a callback, and let the calculator function run the callback after the calculation is finished: Example. function myDisplayer (some) {.
I am trying to execute a function which is passed as a variable to my AJAX function. The problem is that the function is executing at the wrong time. The function is assigned to the variable var callback; I could really use some pointers here as I need control of what functions are called after a post request. When assigning a variable value to another variable, you may want to ensure that the source variable is not null, undefined, or empty. You can either write a long if statement with multiple ... How to assign a variable to a callback? I’ve got this function that takes a URL & Callback in: function getMeta (url, callback) { var img = new Image (); img.src = url; img.onload = function () { return callback (this.width, this.height); } } 6. 1. function getMeta(url, callback) {. 2. var img = new Image(); 3.
A JavaScript Function is a JavaScript Variable until it is executed (evaluated). ... very important feature in JavaScript called as callback. ... functions and assigning them to variables sets ... A custom callback function can be created by using the callback keyword as the last parameter. It can then be invoked by calling the callback () function at the end of the function. The typeof operator is optionally used to check if the argument passed is actually a function. Syntax: function processThis (message, callback) {. 2/12/2014 · First example: Instead of using the following: var myVar; setTimeout(function() { myVar = "some value"; }, 0); alert(myVar); You should rather do the following: var myVar; function callback() { alert(myVar); } setTimeout(function() { myVar = "some value"; callback(); }, 0); Instead of this: var myVar; var script = document.createElement("script");
I am trying to assign an ajax response to a variable. How would I go about doing this? @Swoodend is right. You don't want to do this. It's a horrible idea, and you're having a hard time getting it to work because it's the worst way to work with AJAX requests. It'll be easiest for you to use promises, which jQuery has made very convenient. assign callback data to a variable [duplicate] Ask Question Asked 7 years, ... I've been trying to figure out how to set the value from a callback to a variable so that I can call the variable and access the data rather than having to place all my code inside the callback function. ... JavaScript check if variable exists (is defined/initialized ... This can result in an easy-to-make mistake: attempting to assign the return value of a function to another variable, but accidentally assigning the reference to the function. // Example 4 var a = getValue; var b = a; // b is now a reference to getValue. var c = b (); // b is invoked, so c now holds the value returned by getValue (41) function ...
22/9/2013 · How Can I set Global Variable inside Callback function . function getItem(key) { var value=""; window.plugins.applicationPreferences.get(key, function(result) { value=result; }, function(error) {}); return value; } The above code not set value variable with result value ... While JavaScript is asynchronous, it's not multithreaded. In fact, while it's impossible to predict when a callback will be executed, it is guaranteed that a Race Condition will not occur since JavaScript only runs in a single thread. (As a side note, that doesn't mean there isn't a way to run multiple threads in JavaScript. All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
Callback functions work because in JavaScript, every function is an object. This means that we can work with them like any other object. We can assign functions to variables, or pass them as arguments, just like we would with any other value. Hello, I have a problem where I am not able to update a global variable from inside a callback function. Here is my code: // Set global variable. var load_values_from_server = "initial value ... In such a case, you are binding the callback this to the value of the Constructor's this.. Please, take into account that while binding context for jQuery, you can use jQuery.proxy.. The ECMAScript 6 represents arrow functions that can be considered as lambda functions. They don't contain this.The this can be looked up in scope as a regular variable. It means that you are not required to ...
The callback function gets executed (called) inside the higher order function, but not necessarily immediately. It gets "called back" — hence the name — at whatever point serves the code's purpose. Getting REALLY Tricky: 'this' in Callback Functions. Many people find JavaScript callbacks challenging to begin with. Assigning values to variables in the success callback in ajax. Every post and tutorial I can find say the same thing, "you cannot assign a value to a variable in ajax since the ajax completes before the success is called", and therefore you must "do something in the success callback" - what the heck does that mean?? Can someone show me what to ... In JavaScript, a variable stores the data value that can be changed later on. Use the reserved keyword var to declare a variable in JavaScript. Syntax: var < variable-name >; var < variable-name > = < value >; A variable must have a unique name. The following declares a variable. Example: Variable Declaration.
JavaScript global variable tutorial for beginners and professionals with example, declaring javascript global variable within function, internals of global variable in javascript, event, validation, object loop, array, document, tutorial Object.assign() Method Among the Object constructor methods, there is a method Object.assign() which is used to copy the values and properties from one or more source objects to a target object. It invokes getters and setters since it uses both [[Get]] on the source and [[Set]] on the target. Syntax: Above is an example of a callback variable in JavaScript function. "geekOne" accepts an argument and generates an alert with z as the argument. "geekTwo" accepts an argument and a function. "geekTwo" moves the argument it accepted to the function to passed it to. "geekOne" is the callback function in this case.
Back to some JavaScript Basics, Part II. Last week I began a series made to go through some of JavaScript's basics, the quirks. In my previous post, which you can find here, I briefly touched on ... The code i posted is called within a ajax callback which in itself is an async function. Sorry i didn't make this clear, so the code i have above is just called inside the the ajax success callback. Answer: Use the concatenation operator (+) The simple and safest way to use the concatenation operator ( +) to assign or store a bock of HTML code in a JavaScript variable. You should use the single-quotes while stingify the HTML code block, it would make easier to preserve the double-quotes in the actual HTML code.
Why Is My Variable Unaltered After I Modify It Inside Of A
Paho Python Mqtt Client Understanding Callbacks
Angular 4 How To Update Value Of Class Within Callback
How Not To Be Afraid Of Javascript Anymore Neil Kakkar
Concurrency Model And The Event Loop Javascript Mdn
Using Javascript Steps In The Trainer
How To Use React Usememo And Usecallback Hook
Mastering This In Javascript Callbacks And Bind Apply
How To Rewrite A Callback Function In Promise Form And Async
Chapter 5 Functions For The Master Closures And Scopes
How To Access This Inside A Javascript Callback Function
Get More Data About A User With Javascript Variable
Callback Function Javascript Code Example
Async Programming With Javascript Callbacks Promises And
Mastering This In Javascript Callbacks And Bind Apply
Handling Middlewares And Using Callbacks In Expressjs
Mastering This In Javascript Callbacks And Bind Apply
Javascript Asynchronous Programming And Callbacks
The Definition And Use Of Canopen Callback Function
0 Response to "20 Assign Callback To Variable Javascript"
Post a Comment